使用Python循环测试每一类别的方法包括:使用for循环、使用while循环、使用列表推导式、利用函数和生成器。 其中,通过for循环可以最简单直接地遍历每个类别,并进行测试。这种方法适用于大多数场景,且代码清晰易懂。下面将详细介绍如何使用Python循环测试每一类别的方法。
一、利用for循环
for循环是Python中最常用的循环结构之一。 它可以轻松地遍历列表、元组、字典等可迭代对象,并对其中的每个元素进行操作。以下是具体的实现方法。
1.1 遍历列表中的每个类别
假设有一个包含多个类别的列表,我们可以使用for循环遍历每个类别,并进行相应的测试。
categories = ['Category1', 'Category2', 'Category3']
for category in categories:
# 进行相应的测试
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
1.2 遍历字典中的每个类别
如果类别数据存储在字典中,我们可以遍历字典的键或值来进行测试。
category_dict = {
'Category1': 'Test1',
'Category2': 'Test2',
'Category3': 'Test3'
}
for category, test in category_dict.items():
print(f'Testing {category} with {test}')
# 此处可添加具体的测试逻辑
二、利用while循环
while循环在某些特定场景下会比for循环更灵活。 特别是当需要基于某些条件动态决定是否继续循环时,while循环是一个很好的选择。
2.1 使用while循环遍历列表
categories = ['Category1', 'Category2', 'Category3']
index = 0
while index < len(categories):
category = categories[index]
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
index += 1
2.2 基于条件的while循环
categories = ['Category1', 'Category2', 'Category3']
index = 0
while index < len(categories):
category = categories[index]
if category != 'Category2': # 假设对某一类别不进行测试
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
index += 1
三、使用列表推导式
列表推导式是一种简洁的语法, 它可以在一行代码中实现对列表中每个元素的操作。虽然它更适合进行简单的变换,但在某些情况下也可以用于测试每个类别。
3.1 简单的列表推导式
categories = ['Category1', 'Category2', 'Category3']
[print(f'Testing {category}') for category in categories]
3.2 带有条件的列表推导式
categories = ['Category1', 'Category2', 'Category3']
[print(f'Testing {category}') for category in categories if category != 'Category2']
四、利用函数和生成器
将逻辑封装在函数中有助于提高代码的可读性和复用性。 生成器则可以在需要时动态生成数据,节省内存。
4.1 使用函数进行测试
def test_category(category):
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
categories = ['Category1', 'Category2', 'Category3']
for category in categories:
test_category(category)
4.2 使用生成器进行测试
def category_generator(categories):
for category in categories:
yield category
categories = ['Category1', 'Category2', 'Category3']
for category in category_generator(categories):
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
五、结合多种方法
在实际开发中,往往需要结合多种方法来满足复杂的需求。以下是一个综合示例,展示如何使用多种方法来进行每一类别的测试。
5.1 综合示例
def test_category(category):
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
def category_generator(categories):
for category in categories:
yield category
categories = ['Category1', 'Category2', 'Category3', 'Category4']
使用for循环和生成器
for category in category_generator(categories):
if category != 'Category3': # 假设对某一类别不进行测试
test_category(category)
六、实战中的应用
在实际项目中,循环测试每一类别的方法可以应用于多个领域。以下是几个常见的应用场景。
6.1 数据分析中的类别遍历
在数据分析中,经常需要对数据集中的每个类别进行统计和分析。可以使用上述方法遍历每个类别,并进行相应的分析操作。
import pandas as pd
假设有一个数据集
data = {
'Category': ['A', 'B', 'A', 'B', 'C', 'A', 'C'],
'Value': [10, 20, 30, 40, 50, 60, 70]
}
df = pd.DataFrame(data)
获取所有类别
categories = df['Category'].unique()
for category in categories:
category_data = df[df['Category'] == category]
print(f'Category: {category}, Mean Value: {category_data['Value'].mean()}')
6.2 Web爬虫中的类别遍历
在Web爬虫中,可以使用循环遍历每个类别的链接,并进行数据抓取。
import requests
from bs4 import BeautifulSoup
categories = ['news', 'sports', 'entertainment']
base_url = 'https://example.com/'
for category in categories:
url = f'{base_url}{category}'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 进行数据抓取
print(f'Fetched data for {category}')
七、优化和性能考量
在处理大规模数据或复杂逻辑时,需要考虑代码的性能和优化。以下是一些常见的优化方法。
7.1 使用多线程或多进程
对于耗时较长的操作,可以使用多线程或多进程来提高效率。
import threading
def test_category(category):
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
categories = ['Category1', 'Category2', 'Category3', 'Category4']
threads = []
for category in categories:
thread = threading.Thread(target=test_category, args=(category,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
7.2 使用批量处理
对于需要频繁读写数据库或文件的操作,可以使用批量处理来减少I/O操作次数。
import sqlite3
def test_category(category):
print(f'Testing {category}')
# 此处可添加具体的测试逻辑
categories = ['Category1', 'Category2', 'Category3', 'Category4']
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS test_results
(category TEXT, result TEXT)''')
results = []
for category in categories:
result = f'Result for {category}'
results.append((category, result))
test_category(category)
批量插入结果
cursor.executemany('INSERT INTO test_results VALUES (?,?)', results)
conn.commit()
conn.close()
八、总结
通过本文的介绍,我们详细探讨了如何使用Python循环测试每一类别的方法。包括使用for循环、while循环、列表推导式、函数和生成器等多种方法,并结合实际应用场景进行了演示。希望这些方法和技巧能够帮助你在实际开发中更高效地处理类似问题。
相关问答FAQs:
如何在Python中实现循环遍历每一类别的测试?
在Python中,可以使用循环结构(如for循环或while循环)来遍历不同类别的数据。首先,确保你的数据已经按照类别进行分类。接着,可以使用for
循环迭代每个类别,并对每个类别执行测试逻辑。例如,可以将每个类别的数据放入一个列表或字典中,然后使用循环进行处理。
在进行类别测试时,如何处理异常情况?
在测试每个类别时,处理异常情况是至关重要的。可以使用try
和except
语句来捕捉可能出现的错误,确保程序能够继续运行而不会中断。例如,在循环中尝试执行测试逻辑,如果发生异常,则记录错误信息并继续处理下一个类别。
如何评估每个类别的测试结果?
评估测试结果可以通过计算准确率、召回率等指标来实现。可以在循环中对每个类别的测试结果进行记录,并在循环结束后汇总这些数据。使用数据分析工具,如Pandas,可以方便地处理和可视化这些结果,帮助你理解每个类别的表现。
如何优化循环测试以提高性能?
针对大规模数据集,优化循环测试的性能非常重要。可以考虑使用多线程或多进程技术来并行处理不同类别的测试。此外,避免在循环内重复计算相同的数据,可以通过预处理来减少不必要的计算,从而提高整体性能。
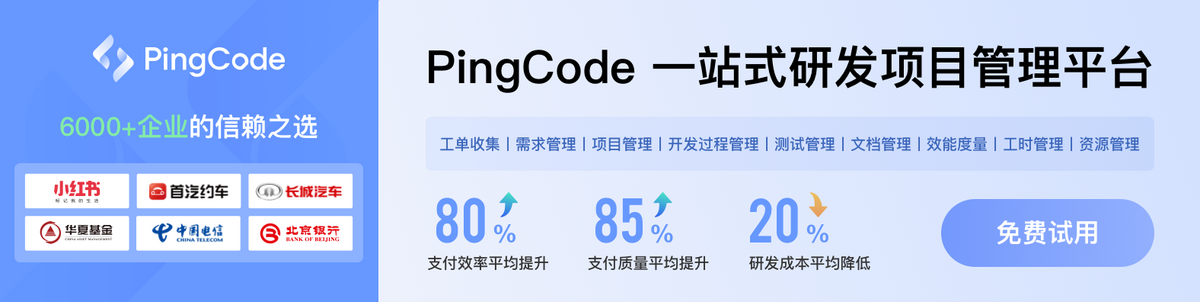