Python将输出结果保存到文件夹的方法包括使用文件写入操作、日志记录工具、序列化工具、以及数据库等。 其中,最常用的方法是使用文件写入操作。接下来,我将详细描述如何使用Python将输出结果保存到文件夹,并且探讨其他方法的使用情景和优缺点。
一、使用文件写入操作
1.1、基础文件写入
在Python中,最基本的文件写入操作是通过内置的 open()
函数和 write()
方法完成的。以下是一个简单的例子:
# 打开文件(如果文件不存在则创建文件)
with open('output.txt', 'w') as file:
# 写入内容
file.write('This is a test output.\n')
file.write('Output can be written line by line.\n')
在这个例子中,我们使用 with
语句来确保文件在操作完成后被正确关闭。文件模式 'w'
表示写入模式,如果文件已经存在,它将被覆盖。如果我们希望追加内容,可以使用 'a'
模式。
1.2、写入多行数据
如果需要写入多行数据,可以使用以下方法:
data = ['Line 1', 'Line 2', 'Line 3']
with open('output.txt', 'w') as file:
for line in data:
file.write(line + '\n')
或者使用 writelines()
方法:
data = ['Line 1\n', 'Line 2\n', 'Line 3\n']
with open('output.txt', 'w') as file:
file.writelines(data)
1.3、保存复杂数据结构
对于更复杂的数据结构(如列表、字典),可以使用 json
模块进行序列化:
import json
data = {
'name': 'John',
'age': 30,
'city': 'New York'
}
with open('output.json', 'w') as file:
json.dump(data, file)
二、使用日志记录工具
2.1、基础日志记录
Python提供了强大的 logging
模块,用于记录程序运行时的日志信息。以下是一个基本的日志记录示例:
import logging
logging.basicConfig(filename='app.log', filemode='w', format='%(name)s - %(levelname)s - %(message)s')
logger = logging.getLogger('example_logger')
logger.warning('This is a warning message')
logger.error('This is an error message')
2.2、高级日志记录
对于更高级的日志记录需求,可以配置多个日志处理器和格式器:
import logging
创建日志记录器
logger = logging.getLogger('example_logger')
logger.setLevel(logging.DEBUG)
创建文件处理器
file_handler = logging.FileHandler('app.log')
file_handler.setLevel(logging.DEBUG)
创建控制台处理器
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.ERROR)
创建格式器并将其添加到处理器中
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
file_handler.setFormatter(formatter)
console_handler.setFormatter(formatter)
将处理器添加到记录器中
logger.addHandler(file_handler)
logger.addHandler(console_handler)
记录示例日志
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
三、使用序列化工具
3.1、Pickle模块
pickle
模块可以将Python对象序列化为二进制格式,并将其写入文件:
import pickle
data = {'name': 'John', 'age': 30, 'city': 'New York'}
with open('data.pkl', 'wb') as file:
pickle.dump(data, file)
要从文件中加载数据,可以使用 pickle.load()
方法:
with open('data.pkl', 'rb') as file:
loaded_data = pickle.load(file)
print(loaded_data)
3.2、CSV模块
对于表格数据,csv
模块提供了方便的读写方法:
import csv
data = [
['Name', 'Age', 'City'],
['John', 30, 'New York'],
['Anna', 22, 'London'],
['Mike', 32, 'San Francisco']
]
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
四、使用数据库
4.1、SQLite数据库
Python内置的 sqlite3
模块提供了一个轻量级的嵌入式数据库:
import sqlite3
连接到数据库(如果数据库不存在则创建数据库)
conn = sqlite3.connect('example.db')
c = conn.cursor()
创建表
c.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER, city TEXT)''')
插入数据
c.execute("INSERT INTO users (name, age, city) VALUES ('John', 30, 'New York')")
c.execute("INSERT INTO users (name, age, city) VALUES ('Anna', 22, 'London')")
c.execute("INSERT INTO users (name, age, city) VALUES ('Mike', 32, 'San Francisco')")
提交事务
conn.commit()
查询数据
c.execute('SELECT * FROM users')
print(c.fetchall())
关闭连接
conn.close()
五、总结
通过本文详细介绍的几种方法,我们可以灵活地选择适合自己的方式将Python输出结果保存到文件夹中。使用文件写入操作是最直接和常用的方法,适合保存简单的文本和数据。使用日志记录工具则适用于需要记录程序运行状态和错误信息的场景。使用序列化工具可以方便地保存和恢复复杂的Python对象,而使用数据库则适合处理大量结构化数据。
每种方法都有其独特的优势和适用情景,选择合适的方法可以显著提高数据处理和存储的效率。在实际应用中,我们往往需要结合多种方法,以满足不同的需求。希望这篇文章能帮助你更好地理解和运用这些技术,将Python输出结果保存到文件夹中。
相关问答FAQs:
如何在Python中将输出结果保存为文本文件?
在Python中,您可以使用内置的open()
函数将输出结果保存到文本文件中。通过指定文件名和模式(例如“w”代表写入模式),您可以将输出内容写入文件。示例如下:
with open('output.txt', 'w') as file:
file.write('这是要保存的输出结果')
这样,内容就会被保存到当前目录下的output.txt
文件中。
如何将Python程序的输出保存为CSV文件?
如果您需要将输出结果保存为CSV格式,可以使用csv
模块。通过创建一个csv.writer
对象,您可以轻松地将数据写入CSV文件。示例代码如下:
import csv
data = [['名称', '年龄'], ['Alice', 30], ['Bob', 25]]
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
这段代码将创建一个output.csv
文件,并将数据以表格形式保存。
如何将Python中的输出结果保存为JSON格式?
若要将输出结果保存为JSON格式,可以使用json
模块。通过json.dump()
方法,可以将Python对象直接保存为JSON文件。以下是示例:
import json
data = {'name': 'Alice', 'age': 30}
with open('output.json', 'w') as file:
json.dump(data, file)
执行后,output.json
文件中将包含格式化的JSON数据,便于存储和传输。
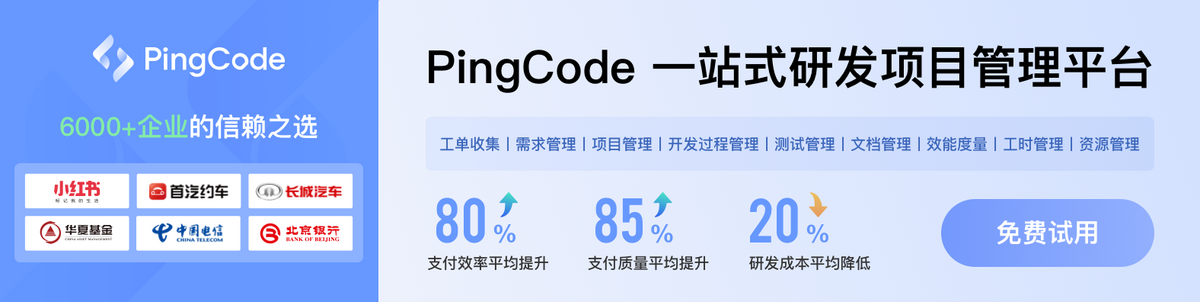