要将Python输出结果写入文本文件,可以使用 open()
函数、 write()
方法或 writelines()
方法, with
语句。本文将详细介绍如何将Python输出结果写入文本文件,并涵盖多种方法和最佳实践。
一、使用open()
函数和write()
方法
Python提供了open()
函数用于打开文件,并返回文件对象。使用write()
方法可以将字符串写入文件。这是最基本的方式之一。
1.1、打开文件
首先,需要用open()
函数打开一个文件。
file = open("output.txt", "w")
在这里,"w"
表示写入模式。如果文件不存在,它将被创建;如果文件存在,它将被覆盖。
1.2、写入内容
使用write()
方法将字符串写入文件。
file.write("Hello, World!")
1.3、关闭文件
写入操作完成后,务必关闭文件以确保数据被正确写入。
file.close()
二、使用with
语句
with
语句是Python中用于处理资源管理的简洁方式。在文件操作中,with
语句确保文件在离开with
块时自动关闭,即使发生异常。
2.1、写入单行内容
with open("output.txt", "w") as file:
file.write("Hello, World!")
2.2、写入多行内容
可以使用write()
方法多次写入。
with open("output.txt", "w") as file:
file.write("Hello, World!\n")
file.write("Python is awesome!\n")
三、使用writelines()
方法
writelines()
方法用于写入一个字符串列表,不会自动添加换行符。
3.1、写入列表内容
lines = ["Hello, World!\n", "Python is awesome!\n"]
with open("output.txt", "w") as file:
file.writelines(lines)
四、格式化输出
有时需要将变量或计算结果写入文件,可以使用字符串格式化。
4.1、使用f-string
name = "John"
age = 30
with open("output.txt", "w") as file:
file.write(f"Name: {name}, Age: {age}")
4.2、使用str.format()
name = "John"
age = 30
with open("output.txt", "w") as file:
file.write("Name: {}, Age: {}".format(name, age))
五、处理异常
在文件操作中,处理异常非常重要,以防止数据丢失或文件损坏。
5.1、使用try-except
try:
with open("output.txt", "w") as file:
file.write("Hello, World!")
except IOError as e:
print(f"An error occurred: {e}")
六、追加写入
有时需要在文件末尾追加内容,可以使用"a"
模式。
6.1、追加内容
with open("output.txt", "a") as file:
file.write("Appending new line.\n")
七、写入JSON格式数据
Python的json
模块可以方便地将Python数据结构写入文件。
7.1、写入JSON
import json
data = {"name": "John", "age": 30}
with open("output.txt", "w") as file:
json.dump(data, file)
八、写入CSV格式数据
使用csv
模块可以将数据写入CSV文件。
8.1、写入CSV
import csv
data = [["Name", "Age"], ["John", 30], ["Jane", 25]]
with open("output.csv", "w", newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
九、写入二进制数据
有时需要写入非文本数据,如图像或音频文件,可以使用"wb"
模式。
9.1、写入二进制数据
binary_data = b'\x00\x01\x02\x03'
with open("output.bin", "wb") as file:
file.write(binary_data)
十、自动生成文件名
为了避免文件覆盖或方便管理,可以自动生成文件名。
10.1、使用时间戳
import time
file_name = f"output_{int(time.time())}.txt"
with open(file_name, "w") as file:
file.write("Hello, World!")
十一、使用上下文管理器
创建自定义上下文管理器,可以在更复杂的场景中管理文件操作。
11.1、自定义上下文管理器
class FileManager:
def __init__(self, file_name, mode):
self.file_name = file_name
self.mode = mode
def __enter__(self):
self.file = open(self.file_name, self.mode)
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
with FileManager("output.txt", "w") as file:
file.write("Hello, World!")
十二、总结
写入文件时,务必注意文件模式、异常处理和资源管理。通过使用with
语句、write()
方法、writelines()
方法以及格式化输出,可以灵活高效地将Python输出结果写入文本文件。无论是处理简单的文本数据还是复杂的二进制数据,Python都提供了丰富的工具和方法来满足需求。
相关问答FAQs:
如何在Python中将输出结果保存到文本文件中?
在Python中,可以使用内置的open()
函数创建或打开一个文本文件,并使用write()
方法将输出结果写入文件。示例代码如下:
with open('output.txt', 'w') as file:
file.write('这是我想要保存的输出结果。\n')
使用with
语句可以确保文件在写入后自动关闭,避免资源泄露。
我可以将多个输出结果写入同一个文本文件吗?
当然可以!只需在打开文件时使用'a'
模式,这样可以追加内容而不覆盖原有的内容。示例代码如下:
with open('output.txt', 'a') as file:
file.write('这是另一个输出结果。\n')
这样,新的输出结果将被添加到文件的末尾。
如何将Python中的打印输出重定向到文本文件?
可以通过重定向sys.stdout
来实现这一点。示例代码如下:
import sys
with open('output.txt', 'w') as file:
sys.stdout = file
print('这个输出将被写入文件而不是控制台。')
sys.stdout = sys.__stdout__ # 恢复默认输出
这种方法使得所有的打印语句都将输出到指定的文件中,而不是控制台。记得在完成后恢复默认输出,以避免后续输出出错。
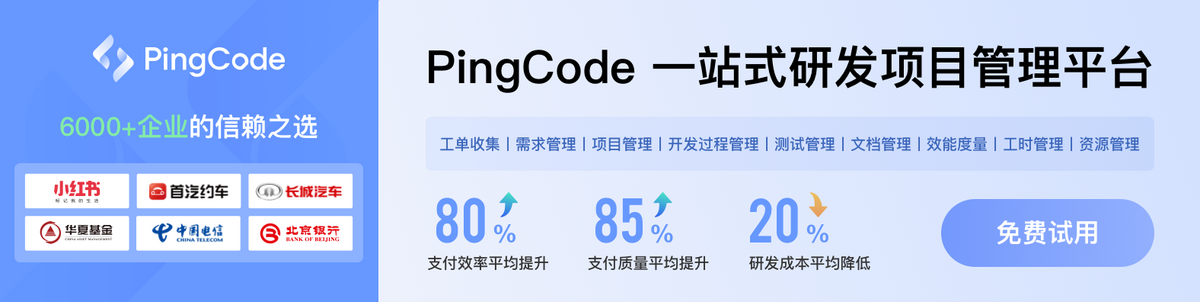