开头段落:
Python判断两个字符串的方法有多种,包括使用运算符、内置函数和外部库。 其中最基本的方法是使用==
运算符进行直接比较。此外,可以使用内置的str
方法如startswith()
和endswith()
来进行部分匹配,或者使用正则表达式进行更复杂的匹配。在特定场景下,还可以利用外部库如difflib
进行字符串相似度比较。这些方法各有优缺点,适用于不同的应用场景。
一、使用运算符进行比较
最直接的方法是使用==
运算符进行字符串比较。这种方法适用于需要判断两个字符串是否完全相同的场景。
1.1、使用==
运算符
str1 = "hello"
str2 = "hello"
if str1 == str2:
print("The strings are equal.")
else:
print("The strings are not equal.")
在上面的示例中,我们直接使用==
运算符比较str1
和str2
。如果两个字符串内容完全相同,则输出“字符串相同”。
1.2、使用!=
运算符
str1 = "hello"
str2 = "world"
if str1 != str2:
print("The strings are not equal.")
else:
print("The strings are equal.")
!=
运算符用于判断两个字符串是否不同。如果两个字符串内容不同,则输出“字符串不同”。
二、使用内置字符串方法
Python 提供了多种内置字符串方法,可以用于部分匹配或者条件判断。
2.1、使用startswith()
方法
startswith()
方法用于判断字符串是否以指定的前缀开头。
str1 = "hello world"
if str1.startswith("hello"):
print("The string starts with 'hello'.")
else:
print("The string does not start with 'hello'.")
2.2、使用endswith()
方法
endswith()
方法用于判断字符串是否以指定的后缀结尾。
str1 = "hello world"
if str1.endswith("world"):
print("The string ends with 'world'.")
else:
print("The string does not end with 'world'.")
三、使用正则表达式进行比较
对于更复杂的匹配需求,可以使用正则表达式。Python 提供了re
模块来处理正则表达式。
3.1、使用re.match()
re.match()
用于从字符串的起始位置匹配正则表达式。
import re
pattern = r"hello"
str1 = "hello world"
if re.match(pattern, str1):
print("The string matches the pattern.")
else:
print("The string does not match the pattern.")
3.2、使用re.search()
re.search()
用于在整个字符串中搜索正则表达式的匹配项。
import re
pattern = r"world"
str1 = "hello world"
if re.search(pattern, str1):
print("The string contains the pattern.")
else:
print("The string does not contain the pattern.")
四、使用外部库进行字符串相似度比较
在某些情况下,我们可能需要判断两个字符串的相似度。Python 提供了difflib
模块,可以计算字符串之间的相似度。
4.1、使用difflib.SequenceMatcher
difflib.SequenceMatcher
用于比较两个字符串的相似度。
import difflib
str1 = "hello world"
str2 = "hello"
ratio = difflib.SequenceMatcher(None, str1, str2).ratio()
print(f"The similarity ratio is {ratio:.2f}")
在上面的示例中,我们使用difflib.SequenceMatcher
计算了str1
和str2
的相似度比率。
五、使用集合操作进行比较
集合操作可以用于判断两个字符串中的字符集合是否相同或存在交集。
5.1、使用集合比较字符集合
str1 = "hello"
str2 = "ehlol"
if set(str1) == set(str2):
print("The strings have the same set of characters.")
else:
print("The strings do not have the same set of characters.")
在这个示例中,我们将字符串转换为集合,并使用==
运算符进行比较。
六、使用排序进行比较
排序是一种判断两个字符串是否由相同字符组成的简便方法。
6.1、使用排序比较
str1 = "hello"
str2 = "ehlol"
if sorted(str1) == sorted(str2):
print("The strings are anagrams.")
else:
print("The strings are not anagrams.")
在这个示例中,我们将字符串进行排序,并使用==
运算符进行比较。如果两个字符串排序后相同,则它们是字母异位词。
七、使用哈希函数进行比较
哈希函数是一种高效的比较方法,适用于大型字符串比较。
7.1、使用hash()
函数
str1 = "hello"
str2 = "hello"
if hash(str1) == hash(str2):
print("The strings have the same hash value.")
else:
print("The strings do not have the same hash value.")
在这个示例中,我们使用hash()
函数计算字符串的哈希值,并进行比较。如果哈希值相同,则字符串相同。
八、使用locale
模块进行比较
在多语言环境中,可以使用locale
模块进行区域感知的字符串比较。
8.1、使用locale.strcoll()
import locale
locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
str1 = "hello"
str2 = "hello"
if locale.strcoll(str1, str2) == 0:
print("The strings are equal according to locale settings.")
else:
print("The strings are not equal according to locale settings.")
在这个示例中,我们使用locale.strcoll()
函数进行字符串比较,考虑到了区域设置。
九、使用unicodedata
模块进行比较
对于包含特殊字符或不同编码的字符串,可以使用unicodedata
模块进行规范化处理。
9.1、使用unicodedata.normalize()
import unicodedata
str1 = "café"
str2 = "café"
if unicodedata.normalize('NFC', str1) == unicodedata.normalize('NFC', str2):
print("The strings are equal after normalization.")
else:
print("The strings are not equal after normalization.")
在这个示例中,我们使用unicodedata.normalize()
函数进行字符串规范化,并进行比较。
十、使用人工智能进行高级比较
在一些高级应用场景中,可以使用机器学习或深度学习模型进行字符串比较。
10.1、使用预训练模型进行相似度计算
from sentence_transformers import SentenceTransformer, util
model = SentenceTransformer('paraphrase-MiniLM-L6-v2')
str1 = "hello world"
str2 = "hello"
embeddings1 = model.encode(str1, convert_to_tensor=True)
embeddings2 = model.encode(str2, convert_to_tensor=True)
cosine_scores = util.pytorch_cos_sim(embeddings1, embeddings2)
print(f"The similarity score is {cosine_scores.item():.2f}")
在这个示例中,我们使用预训练的句子嵌入模型计算字符串的相似度分数。
总结
Python 提供了多种方法来判断两个字符串,包括基本运算符、内置字符串方法、正则表达式、外部库和高级的机器学习方法。根据具体的应用场景选择合适的方法,可以有效解决字符串比较问题。
相关问答FAQs:
如何在Python中比较两个字符串的相等性?
在Python中,可以使用==
运算符来判断两个字符串是否相等。如果两个字符串的内容完全一致,则返回True
,否则返回False
。示例代码如下:
str1 = "Hello"
str2 = "Hello"
are_equal = str1 == str2 # 返回 True
Python中是否区分字符串的大小写?
是的,Python在比较字符串时是区分大小写的。例如,"hello"和"Hello"被认为是不同的字符串。如果需要忽略大小写进行比较,可以使用lower()
或upper()
方法将两个字符串转换为相同的大小写后再进行比较。示例代码如下:
str1 = "hello"
str2 = "Hello"
are_equal = str1.lower() == str2.lower() # 返回 True
如何判断一个字符串是否包含另一个字符串?
在Python中,可以使用in
关键字来判断一个字符串是否包含另一个字符串。这种方式非常简洁易懂。示例代码如下:
str1 = "Hello, World!"
substring = "World"
contains = substring in str1 # 返回 True
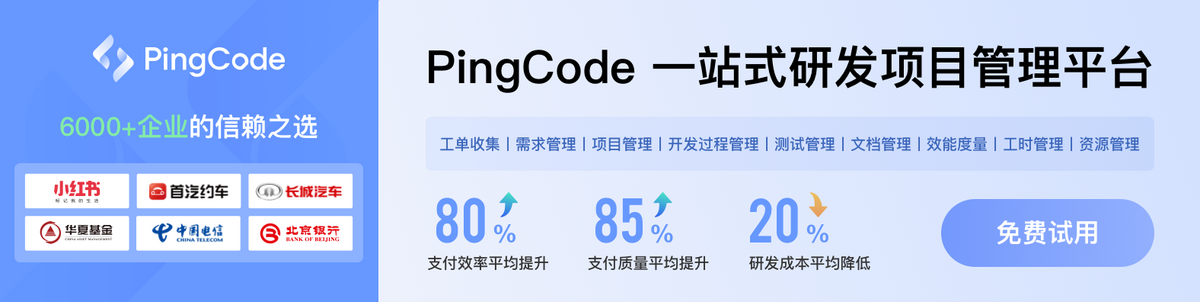