用Python写一个贪吃蛇AI的核心在于:理解游戏规则、选择合适的算法、实现路径规划。 其中,路径规划是最关键的一步,因为它决定了AI蛇的行动路线。本文将详细描述如何从零开始用Python编写一个贪吃蛇AI,涵盖从环境搭建、游戏规则实现、算法选择到具体代码实现的方方面面。
一、环境搭建与游戏规则实现
1、搭建Python开发环境
在开始编写贪吃蛇AI之前,我们需要先搭建Python开发环境。推荐使用Python的最新稳定版本(例如Python 3.9或3.10)。此外,还需要安装一些常用的库,如Pygame用于游戏图形界面,以及Numpy等用于数据处理。
pip install pygame numpy
2、实现基本的贪吃蛇游戏
为了让AI能够在贪吃蛇游戏中运行,我们需要先实现一个基本的贪吃蛇游戏。Pygame是一个不错的选择,因为它简单易用且功能强大。
import pygame
import time
import random
pygame.init()
定义屏幕大小和游戏速度
screen_width, screen_height = 600, 400
game_display = pygame.display.set_mode((screen_width, screen_height))
clock = pygame.time.Clock()
snake_block = 10
snake_speed = 15
定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (213, 50, 80)
green = (0, 255, 0)
显示分数
def display_score(score):
font = pygame.font.SysFont(None, 35)
value = font.render("Score: " + str(score), True, black)
game_display.blit(value, [0, 0])
定义蛇的初始状态
def our_snake(snake_block, snake_list):
for x in snake_list:
pygame.draw.rect(game_display, black, [x[0], x[1], snake_block, snake_block])
定义游戏循环
def game_loop():
game_over = False
game_close = False
x1, y1 = screen_width / 2, screen_height / 2
x1_change, y1_change = 0, 0
snake_list = []
length_of_snake = 1
foodx = round(random.randrange(0, screen_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, screen_height - snake_block) / 10.0) * 10.0
while not game_over:
while game_close:
game_display.fill(white)
font = pygame.font.SysFont(None, 50)
message = font.render("You Lost! Press Q-Quit or C-Play Again", True, red)
game_display.blit(message, [screen_width / 6, screen_height / 3])
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
if event.key == pygame.K_c:
game_loop()
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x1_change = -snake_block
y1_change = 0
elif event.key == pygame.K_RIGHT:
x1_change = snake_block
y1_change = 0
elif event.key == pygame.K_UP:
y1_change = -snake_block
x1_change = 0
elif event.key == pygame.K_DOWN:
y1_change = snake_block
x1_change = 0
if x1 >= screen_width or x1 < 0 or y1 >= screen_height or y1 < 0:
game_close = True
x1 += x1_change
y1 += y1_change
game_display.fill(white)
pygame.draw.rect(game_display, green, [foodx, foody, snake_block, snake_block])
snake_head = []
snake_head.append(x1)
snake_head.append(y1)
snake_list.append(snake_head)
if len(snake_list) > length_of_snake:
del snake_list[0]
for x in snake_list[:-1]:
if x == snake_head:
game_close = True
our_snake(snake_block, snake_list)
display_score(length_of_snake - 1)
pygame.display.update()
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, screen_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, screen_height - snake_block) / 10.0) * 10.0
length_of_snake += 1
clock.tick(snake_speed)
pygame.quit()
quit()
game_loop()
二、选择合适的算法
1、广度优先搜索(BFS)
广度优先搜索是一种图搜索算法,适用于找到最短路径。它会遍历所有可能的路径,找到最短的那条。由于贪吃蛇游戏的复杂性,BFS可能在蛇变长后变得不够高效。
2、深度优先搜索(DFS)
深度优先搜索是一种递归算法,适合找到一条路径,但不一定是最短路径。与BFS相比,DFS的实现更简单,但在复杂的游戏场景中可能会遇到死胡同。
3、A*算法
A算法结合了BFS和DFS的优点,通过启发式函数估计路径成本,能找到最优路径。A算法在贪吃蛇AI中表现出色,能有效避免陷入死胡同。
4、强化学习
强化学习是一种更为复杂的算法,适用于动态调整策略。在贪吃蛇AI中,强化学习可以通过不断学习和调整策略,逐步提高游戏表现。
三、实现路径规划
在选择了合适的算法后,我们需要具体实现路径规划。这里以A*算法为例,详细描述如何实现路径规划。
1、定义节点和启发式函数
在A*算法中,我们需要定义节点和启发式函数。节点表示蛇头的位置,启发式函数用于估计当前节点到目标节点的距离。
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 # 距离起点的实际成本
self.h = 0 # 启发式函数估计的成本
self.f = 0 # 总成本
def heuristic(node, goal):
return abs(node.position[0] - goal.position[0]) + abs(node.position[1] - goal.position[1])
2、实现A*算法
A*算法的核心在于维护一个开放列表和一个闭合列表,不断从开放列表中选择最优节点进行扩展,直到找到目标节点。
def astar(maze, start, end):
start_node = Node(start)
end_node = Node(end)
open_list = []
closed_list = []
open_list.append(start_node)
while open_list:
current_node = min(open_list, key=lambda node: node.f)
open_list.remove(current_node)
closed_list.append(current_node)
if current_node.position == end_node.position:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
children = []
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if node_position[0] > (len(maze) - 1) or node_position[0] < 0 or node_position[1] > (len(maze[0]) - 1) or node_position[1] < 0:
continue
if maze[node_position[0]][node_position[1]] != 0:
continue
new_node = Node(node_position, current_node)
children.append(new_node)
for child in children:
if child in closed_list:
continue
child.g = current_node.g + 1
child.h = heuristic(child, end_node)
child.f = child.g + child.h
if child in open_list:
existing_node = open_list[open_list.index(child)]
if child.g > existing_node.g:
continue
open_list.append(child)
四、将AI集成到贪吃蛇游戏中
1、修改游戏循环
为了让AI控制蛇的移动,我们需要修改游戏循环,使其根据A*算法计算的路径来移动。
def game_loop_with_ai():
game_over = False
game_close = False
x1, y1 = screen_width / 2, screen_height / 2
snake_list = []
length_of_snake = 1
foodx = round(random.randrange(0, screen_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, screen_height - snake_block) / 10.0) * 10.0
while not game_over:
while game_close:
game_display.fill(white)
font = pygame.font.SysFont(None, 50)
message = font.render("You Lost! Press Q-Quit or C-Play Again", True, red)
game_display.blit(message, [screen_width / 6, screen_height / 3])
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
if event.key == pygame.K_c:
game_loop_with_ai()
maze = [[0] * (screen_width // snake_block) for _ in range(screen_height // snake_block)]
for segment in snake_list:
maze[segment[1] // snake_block][segment[0] // snake_block] = 1
start = (y1 // snake_block, x1 // snake_block)
end = (foody // snake_block, foodx // snake_block)
path = astar(maze, start, end)
if path:
next_move = path[1]
y1_change = (next_move[0] - start[0]) * snake_block
x1_change = (next_move[1] - start[1]) * snake_block
if x1 >= screen_width or x1 < 0 or y1 >= screen_height or y1 < 0:
game_close = True
x1 += x1_change
y1 += y1_change
game_display.fill(white)
pygame.draw.rect(game_display, green, [foodx, foody, snake_block, snake_block])
snake_head = []
snake_head.append(x1)
snake_head.append(y1)
snake_list.append(snake_head)
if len(snake_list) > length_of_snake:
del snake_list[0]
for x in snake_list[:-1]:
if x == snake_head:
game_close = True
our_snake(snake_block, snake_list)
display_score(length_of_snake - 1)
pygame.display.update()
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, screen_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, screen_height - snake_block) / 10.0) * 10.0
length_of_snake += 1
clock.tick(snake_speed)
pygame.quit()
quit()
game_loop_with_ai()
五、总结
用Python编写贪吃蛇AI涉及多个步骤,从环境搭建、游戏规则实现、算法选择到路径规划的具体实现。本文详细描述了如何用Pygame实现基本的贪吃蛇游戏,并通过A算法实现AI控制蛇的移动。虽然A算法在贪吃蛇游戏中表现出色,但在实际应用中,还可以结合其他算法和技术,如强化学习,以进一步提高AI的智能水平。通过不断学习和实践,您将能够编写出更智能、更高效的贪吃蛇AI。
相关问答FAQs:
如何开始学习用Python编写贪吃蛇AI?
要学习用Python编写贪吃蛇AI,首先需要掌握Python的基础知识,包括数据结构、函数和类的使用。可以通过在线课程、书籍或者编程社区参与项目来提升技能。理解贪吃蛇游戏的基本规则和逻辑也是非常重要的,可以先从手动编写游戏逻辑开始,再逐步引入AI。
贪吃蛇AI的核心算法是什么?
贪吃蛇AI的核心算法通常包括路径寻找算法,比如A*算法或Dijkstra算法。这些算法帮助AI在游戏中找到最短路径,避免与蛇身碰撞并获取食物。此外,可以使用简单的决策树或神经网络来增强AI的决策能力,使其更智能。
如何测试和优化贪吃蛇AI的表现?
测试贪吃蛇AI的表现可以通过多次运行游戏并记录每局的得分、存活时间等数据。根据这些数据分析AI的决策和反应,识别其弱点。优化时,可以考虑改进算法、调整参数或引入更复杂的策略,以提高AI在不同场景下的适应能力和表现。
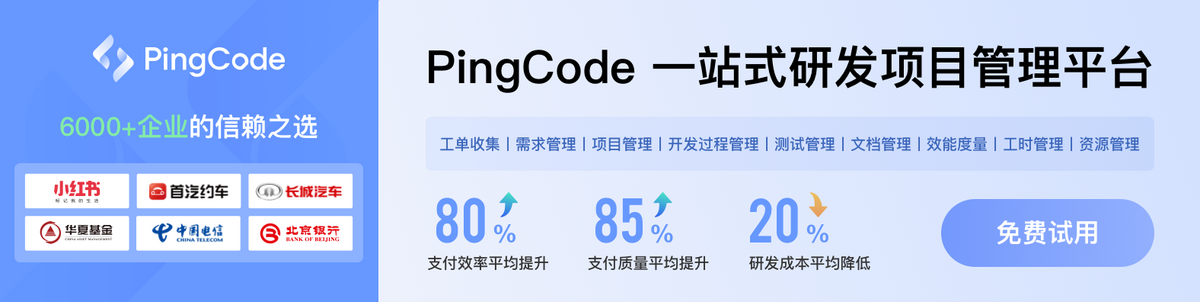