在 Python 中,可以通过多种方法给所有子图都添加网格线。最常用的方法是使用 Matplotlib 库。首先,使用 plt.subplots
创建多个子图,然后使用 ax.grid(True)
对每个子图添加网格线。这种方法简单高效,适用于大多数情景。
例如,在创建多个子图并添加网格线时,可以使用以下代码:
import matplotlib.pyplot as plt
创建2x2的子图
fig, axes = plt.subplots(2, 2)
遍历每个子图并添加网格线
for ax in axes.flatten():
ax.grid(True)
plt.show()
通过这种方法,可以快速为每个子图添加网格线,使得数据的可视化效果更加清晰。
一、如何使用 Matplotlib 创建子图
Matplotlib 是 Python 中最流行的数据可视化库之一,支持创建各种类型的图表。通过 plt.subplots
函数,可以方便地创建多个子图。plt.subplots
返回一个包含 Figure 对象和一组 Axes 对象的元组。
import matplotlib.pyplot as plt
创建2x2的子图
fig, axes = plt.subplots(2, 2)
添加数据到子图
axes[0, 0].plot([1, 2, 3], [4, 5, 6])
axes[0, 1].plot([1, 2, 3], [6, 5, 4])
axes[1, 0].plot([1, 2, 3], [4, 6, 5])
axes[1, 1].plot([1, 2, 3], [5, 4, 6])
显示图表
plt.show()
二、为每个子图添加网格线
在创建子图之后,可以使用 ax.grid(True)
为每个子图添加网格线。这可以通过遍历 axes
数组来实现。
import matplotlib.pyplot as plt
创建2x2的子图
fig, axes = plt.subplots(2, 2)
添加数据到子图
axes[0, 0].plot([1, 2, 3], [4, 5, 6])
axes[0, 1].plot([1, 2, 3], [6, 5, 4])
axes[1, 0].plot([1, 2, 3], [4, 6, 5])
axes[1, 1].plot([1, 2, 3], [5, 4, 6])
遍历每个子图并添加网格线
for ax in axes.flatten():
ax.grid(True)
显示图表
plt.show()
三、网格线的自定义设置
除了简单地打开网格线外,Matplotlib 还允许对网格线进行详细的自定义设置。这包括设置网格线的颜色、线型、宽度等。
import matplotlib.pyplot as plt
创建2x2的子图
fig, axes = plt.subplots(2, 2)
添加数据到子图
axes[0, 0].plot([1, 2, 3], [4, 5, 6])
axes[0, 1].plot([1, 2, 3], [6, 5, 4])
axes[1, 0].plot([1, 2, 3], [4, 6, 5])
axes[1, 1].plot([1, 2, 3], [5, 4, 6])
自定义网格线
for ax in axes.flatten():
ax.grid(True, which='both', color='gray', linestyle='--', linewidth=0.5)
显示图表
plt.show()
四、通过 rcParams 设置全局网格线
如果希望所有图表默认都带有网格线,可以通过 Matplotlib 的全局参数设置 rcParams
来实现。这样,无需在每个子图中单独设置网格线。
import matplotlib.pyplot as plt
设置全局网格线
plt.rcParams['axes.grid'] = True
plt.rcParams['grid.color'] = 'gray'
plt.rcParams['grid.linestyle'] = '--'
plt.rcParams['grid.linewidth'] = 0.5
创建2x2的子图
fig, axes = plt.subplots(2, 2)
添加数据到子图
axes[0, 0].plot([1, 2, 3], [4, 5, 6])
axes[0, 1].plot([1, 2, 3], [6, 5, 4])
axes[1, 0].plot([1, 2, 3], [4, 6, 5])
axes[1, 1].plot([1, 2, 3], [5, 4, 6])
显示图表
plt.show()
五、使用 seaborn 添加网格线
Seaborn 是基于 Matplotlib 的高级可视化库,它默认带有美观的网格线设置。如果使用 Seaborn 创建图表,可以更方便地添加和自定义网格线。
import seaborn as sns
import matplotlib.pyplot as plt
设置Seaborn样式
sns.set(style="whitegrid")
创建2x2的子图
fig, axes = plt.subplots(2, 2)
添加数据到子图
sns.lineplot(x=[1, 2, 3], y=[4, 5, 6], ax=axes[0, 0])
sns.lineplot(x=[1, 2, 3], y=[6, 5, 4], ax=axes[0, 1])
sns.lineplot(x=[1, 2, 3], y=[4, 6, 5], ax=axes[1, 0])
sns.lineplot(x=[1, 2, 3], y=[5, 4, 6], ax=axes[1, 1])
显示图表
plt.show()
六、在面向对象接口中添加网格线
Matplotlib 提供了面向对象的接口,使得创建和管理图表更加灵活和可控。通过创建 Figure 和 Axes 对象,可以更细致地控制图表的各个方面,包括网格线。
import matplotlib.pyplot as plt
创建Figure和Axes对象
fig, ((ax1, ax2), (ax3, ax4)) = plt.subplots(2, 2)
添加数据到子图
ax1.plot([1, 2, 3], [4, 5, 6])
ax2.plot([1, 2, 3], [6, 5, 4])
ax3.plot([1, 2, 3], [4, 6, 5])
ax4.plot([1, 2, 3], [5, 4, 6])
添加网格线
ax1.grid(True)
ax2.grid(True)
ax3.grid(True)
ax4.grid(True)
显示图表
plt.show()
通过以上内容,我们详细介绍了在 Python 中如何给所有子图添加网格线的方法,包括使用 Matplotlib 和 Seaborn 库,以及通过全局设置和面向对象接口进行自定义。希望通过这些方法,可以帮助您更好地进行数据可视化。
相关问答FAQs:
如何在Python中给多个子图添加网格线?
在使用Matplotlib绘制多个子图时,可以通过循环遍历每个子图对象来添加网格线。通常使用plt.subplots()
创建子图,然后在每个子图上调用ax.grid()
方法。这样可以确保每个子图都有相同的网格线样式。
在不同的子图上是否可以自定义网格线样式?
是的,可以为每个子图设置不同的网格线样式。通过在调用ax.grid()
时传入参数,如color
, linestyle
, 和 linewidth
,可以实现这一点。这样可以根据每个子图的需求进行个性化设置,使得数据的可读性更高。
是否可以同时给所有子图添加不同颜色的网格线?
当然可以。通过在循环中为每个子图指定不同的颜色,可以轻松实现这一目标。只需在调用ax.grid()
时设置不同的颜色参数,例如ax.grid(color='red')
或ax.grid(color='blue')
,便可为每个子图添加独特的网格线颜色。这在比较多个数据集时特别有用。
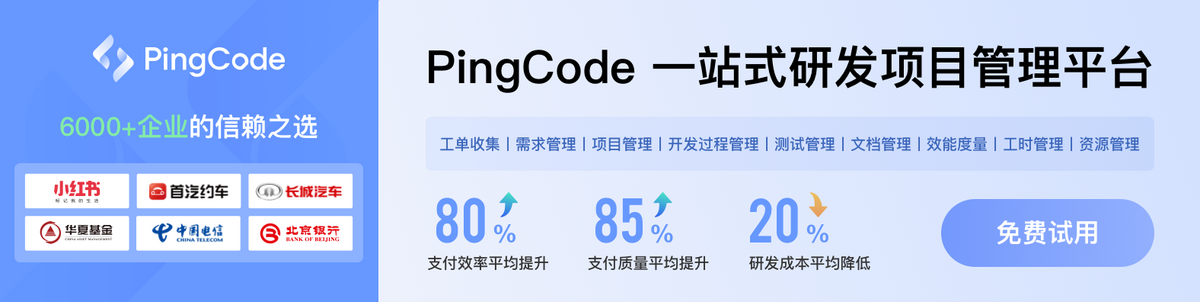