Python中将字符串转换为时间格式的方法主要有:使用datetime模块、利用strptime函数、设置自定义格式、处理时间时区等。 其中,datetime模块是最常用的工具,它提供了丰富的函数和类来处理日期和时间。在本篇文章中,我们将详细介绍这些方法,并提供实际代码示例,帮助你更好地理解和应用。
一、使用datetime模块
Python的datetime模块是处理日期和时间的标准库,提供了多个类和方法来处理时间转换。
1.1 datetime.strptime()
strptime
是datetime模块中的一个函数,它可以将一个时间字符串转换为datetime对象。它需要两个参数:一个是时间字符串,另一个是时间字符串的格式。
from datetime import datetime
date_string = "2023-10-01 12:30:45"
date_format = "%Y-%m-%d %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
print(date_object)
在这个例子中,date_string
是要转换的字符串,date_format
是字符串的格式。%Y
表示四位数的年份,%m
表示月份,%d
表示月份中的一天,%H
表示小时,%M
表示分钟,%S
表示秒。
1.2 datetime.fromisoformat()
fromisoformat
是datetime模块的另一个方法,用于将符合ISO 8601格式的字符串转换为datetime对象。
date_string = "2023-10-01T12:30:45"
date_object = datetime.fromisoformat(date_string)
print(date_object)
这个方法仅适用于符合ISO 8601标准的字符串。
二、利用时间格式化字符串
不同的时间格式化字符串表示不同的时间部分。了解这些格式化字符串可以帮助你更灵活地处理时间转换。
2.1 常用的时间格式化字符串
%Y
:四位数的年份,例如:2023%m
:两位数的月份,例如:01到12%d
:两位数的日期,例如:01到31%H
:两位数的小时(24小时制),例如:00到23%M
:两位数的分钟,例如:00到59%S
:两位数的秒,例如:00到59
2.2 示例代码
from datetime import datetime
date_string = "01-10-2023 12:30:45"
date_format = "%d-%m-%Y %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
print(date_object)
在这个例子中,日期格式是"%d-%m-%Y %H:%M:%S"
,它与上一个例子中的格式不同,但效果是一样的。
三、自定义时间格式
有时候,你需要处理一些不符合常规格式的时间字符串,这时候你可以自定义时间格式。
3.1 自定义格式示例
from datetime import datetime
date_string = "01-Oct-2023 12:30:45"
date_format = "%d-%b-%Y %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
print(date_object)
在这个例子中,%b
表示月份的缩写,如:Jan、Feb、Mar等。
3.2 更复杂的自定义格式
如果时间字符串包含非标准字符或者需要处理不同语言的月份名称,你可以使用正则表达式和locale模块。
import locale
from datetime import datetime
设置区域
locale.setlocale(locale.LC_TIME, 'en_US.UTF-8')
date_string = "01-October-2023 12:30:45"
date_format = "%d-%B-%Y %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
print(date_object)
在这个例子中,%B
表示月份的全称,如:January、February、March等。通过设置区域,你可以处理不同语言的月份名称。
四、处理时间时区
处理时间时区是时间转换中的一个重要方面。datetime模块中的pytz
库可以帮助你处理时区问题。
4.1 安装pytz库
在使用pytz库之前,你需要先安装它:
pip install pytz
4.2 使用pytz处理时区
from datetime import datetime
import pytz
date_string = "2023-10-01 12:30:45"
date_format = "%Y-%m-%d %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
设置时区
timezone = pytz.timezone("America/New_York")
date_object = timezone.localize(date_object)
print(date_object)
在这个例子中,我们将一个时间字符串转换为datetime对象,并将其设置为纽约时区。
4.3 转换时区
你还可以将一个datetime对象从一个时区转换到另一个时区。
from datetime import datetime
import pytz
date_string = "2023-10-01 12:30:45"
date_format = "%Y-%m-%d %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
设置时区
timezone = pytz.timezone("America/New_York")
date_object = timezone.localize(date_object)
转换到另一个时区
new_timezone = pytz.timezone("Asia/Shanghai")
new_date_object = date_object.astimezone(new_timezone)
print(new_date_object)
在这个例子中,我们将一个datetime对象从纽约时区转换到上海时区。
五、处理异常情况
在实际应用中,时间字符串可能不总是符合预期格式。因此,处理异常情况是非常重要的。
5.1 处理无效的时间字符串
你可以使用try-except块来处理无效的时间字符串。
from datetime import datetime
date_string = "invalid date"
date_format = "%Y-%m-%d %H:%M:%S"
try:
date_object = datetime.strptime(date_string, date_format)
print(date_object)
except ValueError:
print("Invalid date format")
在这个例子中,如果时间字符串不符合预期格式,程序会捕获ValueError异常,并输出“Invalid date format”。
5.2 处理不完整的时间字符串
有时候,时间字符串可能缺少某些部分,例如:只包含日期而不包含时间。你可以使用默认值来处理这种情况。
from datetime import datetime
date_string = "2023-10-01"
date_format = "%Y-%m-%d"
try:
date_object = datetime.strptime(date_string, date_format)
print(date_object)
except ValueError:
default_time = "00:00:00"
date_string += f" {default_time}"
date_format += " %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
print(date_object)
在这个例子中,如果时间字符串只包含日期部分,我们将默认时间部分设置为“00:00:00”。
六、总结
在这篇文章中,我们详细介绍了Python中将字符串转换为时间格式的方法,包括使用datetime模块、利用时间格式化字符串、自定义时间格式、处理时间时区和处理异常情况。通过掌握这些方法,你可以更灵活地处理各种时间字符串,从而提高程序的健壮性和可维护性。
希望这篇文章能够帮助你更好地理解和应用Python中的时间转换方法。如果你有任何问题或建议,欢迎在评论区留言。
相关问答FAQs:
如何在Python中将字符串转换为日期时间对象?
在Python中,可以使用datetime
模块来将字符串转换为日期时间对象。通常,使用strptime
方法可以解析字符串并生成相应的日期时间对象。你需要提供字符串和相应的格式。例如,datetime.strptime('2023-10-01 14:30', '%Y-%m-%d %H:%M')
将返回一个代表2023年10月1日14时30分的日期时间对象。
字符串转换时,如何处理不同的时间格式?
当处理不同的时间格式时,必须确保格式字符串与输入字符串的格式匹配。例如,'01/10/2023'
需要使用'%d/%m/%Y'
作为格式字符串。使用strptime
时,确保根据实际字符串格式指定正确的格式符号,以便成功转换。
如果字符串格式不正确,如何处理错误?
在转换字符串时,可能会遇到格式不匹配的情况。为了安全地处理这些错误,可以使用try
和except
语句来捕获ValueError
异常。当输入字符串无法转换时,可以通过异常处理机制返回友好的错误提示或进行相应的处理。这样可以确保程序的稳定性。
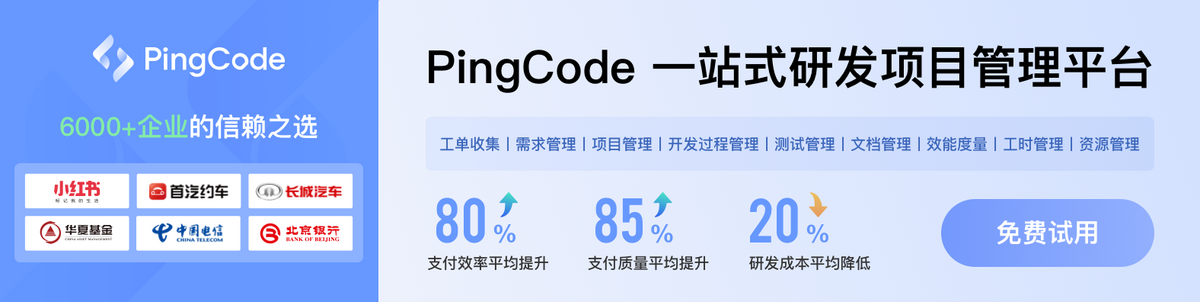