通过Python可以让三维图动起来的方式有很多,主要包括:使用Matplotlib、利用Mayavi、借助Plotly、以及通过Pygame进行3D动画开发。其中,Matplotlib 是最常用的,它提供了强大的2D和3D绘图功能,并且具有良好的社区支持。Mayavi 则适合处理更复杂的3D数据和动画。Plotly 是一个交互式绘图库,适合用于展示动态数据。Pygame 则是一个用于开发游戏的库,也可以用来进行3D动画的开发。下面将详细介绍如何使用这些工具来实现三维图的动画。
一、使用Matplotlib实现三维图动起来
Matplotlib 是一个广泛使用的绘图库,支持生成各种静态、动态、交互式的图表。通过结合 matplotlib.animation
模块,可以实现三维图的动画效果。
1、基本使用方法
要创建一个基本的3D动画,首先需要导入相关库:
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
from matplotlib.animation import FuncAnimation
接下来,设置图形的基本框架:
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X<strong>2 + Y</strong>2))
surface = ax.plot_surface(X, Y, Z, cmap='viridis')
为了使图形动起来,需要定义一个更新函数:
def update(frame):
ax.clear()
Z = np.sin(np.sqrt(X<strong>2 + Y</strong>2) + frame / 10.0)
ax.plot_surface(X, Y, Z, cmap='viridis')
然后,使用 FuncAnimation
创建动画:
ani = FuncAnimation(fig, update, frames=100, interval=50)
plt.show()
2、细节优化
为了使动画更流畅,可以调整帧的数量和间隔时间。还可以添加颜色渐变、光照效果等来增强视觉效果。例如,使用 plot_trisurf
创建网格图:
triangles = ax.plot_trisurf(X.flatten(), Y.flatten(), Z.flatten(), cmap='viridis')
二、利用Mayavi实现复杂三维动画
Mayavi 是一个强大的3D数据可视化工具,适用于处理复杂的三维数据和动画。
1、安装和基本使用
首先需要安装 Mayavi:
pip install mayavi
然后,导入相关库并创建一个基本的三维图形:
from mayavi import mlab
import numpy as np
x, y = np.mgrid[-10:10:100j, -10:10:100j]
z = np.sin(x*y)
mlab.surf(x, y, z, colormap='cool')
mlab.show()
2、添加动画效果
Mayavi 提供了多种方式来实现动画效果,可以通过 mlab.animate
装饰器来实现:
@mlab.animate
def anim():
f = mlab.gcf()
while True:
x, y = np.mgrid[-10:10:100j, -10:10:100j]
z = np.sin(x*y + f.scene.time)
s.mlab_source.scalars = z
f.scene.time += 0.1
yield
anim()
mlab.show()
三、借助Plotly创建交互式三维动画
Plotly 是一个强大的绘图库,支持创建高度交互的图表,包括三维图形和动画。
1、基本使用
首先安装 Plotly:
pip install plotly
然后,创建基本的三维图形:
import plotly.graph_objs as go
import numpy as np
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X<strong>2 + Y</strong>2))
fig = go.Figure(data=[go.Surface(z=Z, x=X, y=Y)])
fig.show()
2、添加动画效果
Plotly 支持通过 frames
参数添加动画效果:
frames = [go.Frame(data=[go.Surface(z=np.sin(np.sqrt(X<strong>2 + Y</strong>2) + k / 10.0))])
for k in range(100)]
fig.frames = frames
fig.update_layout(
updatemenus=[{
'buttons': [{'args': [None, {'frame': {'duration': 50, 'redraw': True}, 'fromcurrent': True}],
'label': 'Play',
'method': 'animate'}]
}]
)
fig.show()
四、通过Pygame进行3D动画开发
Pygame 是一个用于开发游戏的库,也可以用来进行3D动画的开发。
1、安装和基本使用
首先安装 Pygame:
pip install pygame
然后,设置基本的3D动画框架:
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *
vertices = [(1, -1, -1), (1, 1, -1), (-1, 1, -1), (-1, -1, -1), (1, -1, 1), (1, 1, 1), (-1, -1, 1), (-1, 1, 1)]
edges = [(0, 1), (1, 2), (2, 3), (3, 0), (4, 5), (5, 6), (6, 7), (7, 4), (0, 4), (1, 5), (2, 6), (3, 7)]
def draw_cube():
glBegin(GL_LINES)
for edge in edges:
for vertex in edge:
glVertex3fv(vertices[vertex])
glEnd()
pygame.init()
display = (800, 600)
pygame.display.set_mode(display, DOUBLEBUF | OPENGL)
gluPerspective(45, (display[0] / display[1]), 0.1, 50.0)
glTranslatef(0.0, 0.0, -5)
glRotatef(25, 2, 1, 0)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
glRotatef(1, 3, 1, 1)
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
draw_cube()
pygame.display.flip()
pygame.time.wait(10)
结论
通过上述介绍,可以看出使用Python实现三维图的动画有多种方式。Matplotlib 适合简单的3D动画,Mayavi 适合复杂的3D数据可视化,Plotly 适合交互式图表,而Pygame 则适合游戏开发和更高级的3D动画。根据具体需求选择合适的工具,可以更有效地实现三维图的动画效果。
相关问答FAQs:
如何在Python中创建动态的三维图?
在Python中,可以使用Matplotlib库的mpl_toolkits.mplot3d
模块来创建三维图。通过结合FuncAnimation
函数,可以让三维图动起来。你需要设置一个更新函数来改变图形的数据点,并在每一帧中重新绘制图形。确保安装了必要的库,如Matplotlib和NumPy,来支持动态更新。
使用哪些库可以实现三维动画效果?
除了Matplotlib,Python中还有其他库可以实现三维动画效果。例如,Mayavi和Plotly都是非常强大的可视化工具,支持创建动态三维图。Mayavi适合进行科学计算和可视化,而Plotly则更适合创建交互式图表。选择合适的库取决于你的具体需求和项目复杂度。
如何提高三维动画的性能?
为了提高三维动画的性能,可以考虑以下几个方面:优化数据处理,减少每帧绘制的点数;使用更高效的绘图库,如VisPy或PyOpenGL;在创建动画时,尽量使用矢量图形而不是位图,避免每帧都进行重绘。此外,调整动画的帧率也可以有效提升性能,确保流畅的用户体验。
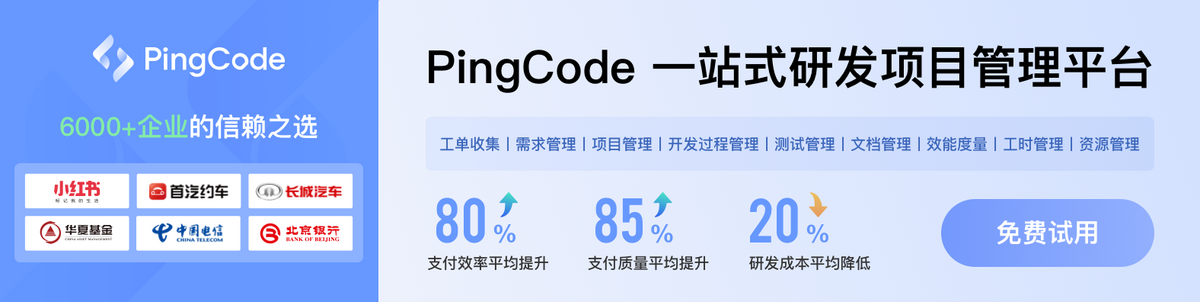