要读取Python代码中的其中一行,可以使用以下方法:使用内置的open
函数打开文件、使用readlines
方法读取所有行、通过行号索引访问特定行。最常用的方法是通过文件操作来读取特定行。接下来,我将详细描述一种方法。
要读取特定行,首先需要打开文件并读取其内容。通过open
函数打开文件,并使用readlines
方法将文件中的所有行读入一个列表中。然后,通过行号索引访问特定行的内容。以下是一个简单的示例代码:
with open('example.py', 'r') as file:
lines = file.readlines()
specific_line = lines[line_number - 1] # 行号从0开始,因此需要减1
print(specific_line)
一、文件操作基础
在Python中,文件操作是一个非常基础但重要的部分。通过文件操作,我们可以读取、写入、修改文件内容。了解文件操作的基础知识对于读取特定行是非常必要的。
1、打开文件
Python内置的open
函数用于打开文件。open
函数有两个主要参数:文件路径和模式。模式决定了文件是以只读、写入、追加还是其他方式打开的。常见的模式包括:
'r'
: 只读模式'w'
: 写入模式'a'
: 追加模式'b'
: 二进制模式
file = open('example.py', 'r')
2、读取文件内容
读取文件内容的方法有多种,包括read
、readline
、readlines
等。每种方法有其独特的用途。
read()
: 读取整个文件内容,返回一个字符串readline()
: 每次调用读取一行,返回一个字符串readlines()
: 读取所有行,返回一个包含每行内容的列表
lines = file.readlines()
3、关闭文件
操作完文件后,必须关闭文件以释放资源。这可以通过close
方法完成。但是,使用with
语句可以自动管理文件资源,无需手动关闭文件。
file.close()
4、使用 with
语句
with
语句是文件操作的推荐方式,能够自动管理文件资源,无需手动关闭文件。
with open('example.py', 'r') as file:
lines = file.readlines()
二、读取特定行的实现
1、基础实现
通过以上基础知识,我们可以实现读取特定行的功能。以下是一个完整的示例代码:
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip() # 去除行末的换行符
else:
return None # 行号超出范围
file_path = 'example.py'
line_number = 3
specific_line = read_specific_line(file_path, line_number)
if specific_line:
print(f"Line {line_number}: {specific_line}")
else:
print(f"Line {line_number} is out of range.")
2、错误处理
在实际应用中,文件操作可能会遇到各种错误,如文件不存在、权限不足等。为了提高代码的鲁棒性,建议添加错误处理。
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip()
else:
return None
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
except PermissionError:
print(f"Error: Permission denied to read the file {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
file_path = 'example.py'
line_number = 3
specific_line = read_specific_line(file_path, line_number)
if specific_line:
print(f"Line {line_number}: {specific_line}")
else:
print(f"Line {line_number} is out of range.")
三、读取大文件的优化
对于大文件,readlines
方法可能会导致内存不足。此时,可以使用readline
方法逐行读取并计数,直到找到特定行。
1、使用readline
方法
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
return None # 行号超出范围
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
except PermissionError:
print(f"Error: Permission denied to read the file {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
file_path = 'example.py'
line_number = 3
specific_line = read_specific_line(file_path, line_number)
if specific_line:
print(f"Line {line_number}: {specific_line}")
else:
print(f"Line {line_number} is out of range.")
2、使用生成器
生成器可以逐行读取文件内容,节省内存资源。以下是使用生成器实现读取特定行的示例:
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
def file_line_generator(file):
while True:
line = file.readline()
if not line:
break
yield line
for current_line_number, line in enumerate(file_line_generator(file), start=1):
if current_line_number == line_number:
return line.strip()
return None
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
except PermissionError:
print(f"Error: Permission denied to read the file {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
file_path = 'example.py'
line_number = 3
specific_line = read_specific_line(file_path, line_number)
if specific_line:
print(f"Line {line_number}: {specific_line}")
else:
print(f"Line {line_number} is out of range.")
四、优化性能和效率
在某些情况下,可能需要进一步优化性能和效率。例如,当需要频繁读取特定行时,可以将文件内容缓存到内存中,以减少I/O操作的次数。
1、缓存文件内容
将文件内容缓存到内存中,可以提高后续读取特定行的效率。
class FileCache:
def __init__(self, file_path):
self.file_path = file_path
self.lines = self._read_file()
def _read_file(self):
try:
with open(self.file_path, 'r') as file:
return file.readlines()
except FileNotFoundError:
print(f"Error: The file {self.file_path} does not exist.")
return []
except PermissionError:
print(f"Error: Permission denied to read the file {self.file_path}.")
return []
except Exception as e:
print(f"An unexpected error occurred: {e}")
return []
def read_specific_line(self, line_number):
if line_number <= len(self.lines):
return self.lines[line_number - 1].strip()
else:
return None
file_cache = FileCache('example.py')
line_number = 3
specific_line = file_cache.read_specific_line(line_number)
if specific_line:
print(f"Line {line_number}: {specific_line}")
else:
print(f"Line {line_number} is out of range.")
2、多线程优化
在某些情况下,多线程读取文件可以提高效率。以下是一个简单的多线程读取示例:
import threading
class FileCache:
def __init__(self, file_path):
self.file_path = file_path
self.lines = self._read_file()
self.lock = threading.Lock()
def _read_file(self):
try:
with open(self.file_path, 'r') as file:
return file.readlines()
except FileNotFoundError:
print(f"Error: The file {self.file_path} does not exist.")
return []
except PermissionError:
print(f"Error: Permission denied to read the file {self.file_path}.")
return []
except Exception as e:
print(f"An unexpected error occurred: {e}")
return []
def read_specific_line(self, line_number):
with self.lock:
if line_number <= len(self.lines):
return self.lines[line_number - 1].strip()
else:
return None
file_cache = FileCache('example.py')
def read_line_in_thread(line_number):
specific_line = file_cache.read_specific_line(line_number)
if specific_line:
print(f"Line {line_number}: {specific_line}")
else:
print(f"Line {line_number} is out of range.")
threads = []
for i in range(1, 6):
thread = threading.Thread(target=read_line_in_thread, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
五、实际应用场景
1、日志文件分析
在实际应用中,读取日志文件的特定行是一个常见需求。通过读取特定行,可以快速定位问题或分析日志内容。
def read_log_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
return None
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
except PermissionError:
print(f"Error: Permission denied to read the file {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
log_file_path = 'system.log'
line_number = 100
log_line = read_log_line(log_file_path, line_number)
if log_line:
print(f"Log Line {line_number}: {log_line}")
else:
print(f"Log Line {line_number} is out of range.")
2、数据处理
在数据处理过程中,可能需要读取文件中的特定行以进行进一步的处理。例如,读取CSV文件的特定行以提取数据。
import csv
def read_csv_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
reader = csv.reader(file)
for current_line_number, row in enumerate(reader, start=1):
if current_line_number == line_number:
return row
return None
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
except PermissionError:
print(f"Error: Permission denied to read the file {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
csv_file_path = 'data.csv'
line_number = 5
csv_line = read_csv_line(csv_file_path, line_number)
if csv_line:
print(f"CSV Line {line_number}: {csv_line}")
else:
print(f"CSV Line {line_number} is out of range.")
通过以上方法和示例,我们可以灵活地读取Python代码中的特定行,并应用于实际的开发和数据处理场景。了解和掌握这些技巧,对于提高代码的效率和鲁棒性是非常有帮助的。
相关问答FAQs:
如何在Python中读取特定行的内容?
在Python中,您可以使用文件的读取方法来获取特定行的内容。例如,您可以将文件中的所有行读取到一个列表中,然后通过索引来访问特定行。以下是一个示例代码:
with open('your_file.py', 'r') as file:
lines = file.readlines()
specific_line = lines[line_number - 1] # line_number是您想要读取的行号
print(specific_line)
这种方法简单且易于理解,适合小文件的读取。
是否可以直接读取大型文件中的某一行而不加载整个文件?
是的,可以使用file.seek()
和file.readline()
方法来高效地读取大型文件中的特定行,而不需要将整个文件加载到内存中。这种方法适用于处理大文件时节省内存。实现方法如下:
with open('your_file.py', 'r') as file:
for current_line_number, line in enumerate(file):
if current_line_number == line_number - 1:
print(line)
break
这种方法会逐行读取文件,直到找到目标行。
在读取代码时,有什么建议以避免错误?
在读取代码文件时,确保处理文件路径和行号的有效性。使用异常处理来捕获潜在的错误,例如文件不存在或行号超出范围。以下是增强版的代码示例:
try:
with open('your_file.py', 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
print(lines[line_number - 1])
else:
print("行号超出范围。")
except FileNotFoundError:
print("文件未找到,请检查路径。")
通过这种方式,可以提高代码的稳定性和用户体验。
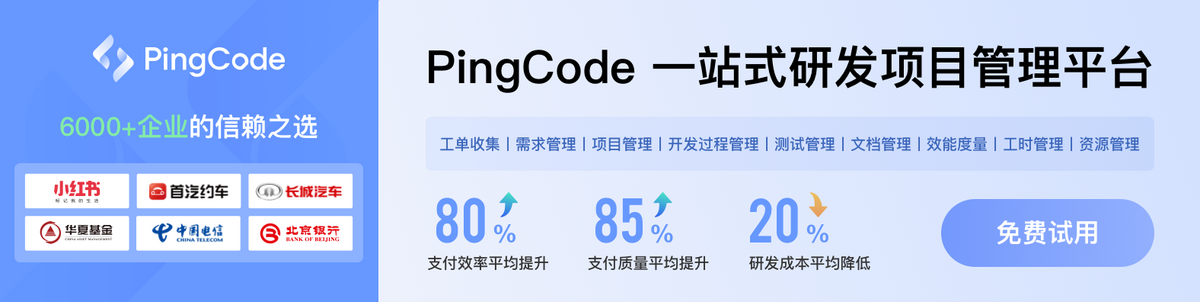