通过Python遍历一个文件中的所有图像
核心观点:使用os模块获取文件列表、使用Pillow或OpenCV库读取图像、利用循环结构遍历文件、检查文件格式过滤非图像文件。我们将详细描述如何利用Python的os模块获取文件列表,并结合Pillow或OpenCV库读取图像文件。在具体实现过程中,需要特别注意文件格式的检查,以确保只处理图像文件。
一、使用os模块获取文件列表
os模块是Python标准库中用于与操作系统交互的模块。它提供了丰富的方法来操作文件和目录。我们可以利用os.listdir()
方法来获取指定目录下的所有文件名,并结合os.path
模块来检查文件类型和路径。
import os
def get_all_files_in_directory(directory):
files = os.listdir(directory)
return [os.path.join(directory, file) for file in files]
在上述代码中,os.listdir(directory)
返回指定目录下的所有文件和文件夹名。通过列表推导式结合os.path.join(directory, file)
,我们将这些文件名转换为完整的文件路径。
二、使用Pillow或OpenCV库读取图像
1. 使用Pillow库
Pillow(PIL的一个分支)是Python Imaging Library的一个分支,提供了强大的图像处理功能。我们可以使用Pillow库中的Image.open()
方法来读取图像文件。
from PIL import Image
def read_image_with_pillow(image_path):
try:
img = Image.open(image_path)
img.verify() # 验证文件是否为图像
return img
except (IOError, SyntaxError) as e:
print(f"File {image_path} is not an image.")
return None
上述代码中,Image.open(image_path)
尝试打开指定路径的图像文件,img.verify()
用于验证文件是否为有效的图像。
2. 使用OpenCV库
OpenCV是一个开源的计算机视觉库,广泛用于图像处理和计算机视觉领域。我们可以使用OpenCV库中的cv2.imread()
方法来读取图像文件。
import cv2
def read_image_with_opencv(image_path):
img = cv2.imread(image_path)
if img is None:
print(f"File {image_path} is not an image.")
return img
在上述代码中,cv2.imread(image_path)
尝试读取图像文件,如果返回值为None
,则说明文件不是有效的图像。
三、利用循环结构遍历文件
在获取目录下所有文件的列表并确定读取图像的方法后,我们可以利用for循环遍历这些文件,并读取图像文件。
def process_all_images_in_directory(directory, use_pillow=True):
files = get_all_files_in_directory(directory)
for file in files:
if use_pillow:
img = read_image_with_pillow(file)
else:
img = read_image_with_opencv(file)
if img is not None:
# 在这里处理图像文件
print(f"Processing image: {file}")
在上述代码中,process_all_images_in_directory()
函数接受一个目录路径和一个布尔参数use_pillow
,通过遍历目录下的所有文件,逐个读取图像文件并进行处理。
四、检查文件格式过滤非图像文件
为了确保只处理图像文件,我们需要检查文件的扩展名。常见的图像文件扩展名包括.jpg
、.jpeg
、.png
、.bmp
等。
def is_image_file(file_path):
image_extensions = ('.jpg', '.jpeg', '.png', '.bmp', '.gif', '.tiff')
return file_path.lower().endswith(image_extensions)
在上述代码中,is_image_file()
函数接受一个文件路径作为参数,并根据文件扩展名判断该文件是否为图像文件。
五、综合实现遍历图像文件的完整代码
结合上述各个步骤,我们可以实现一个完整的Python脚本,用于遍历指定目录下的所有图像文件并进行处理。
import os
from PIL import Image
import cv2
def get_all_files_in_directory(directory):
files = os.listdir(directory)
return [os.path.join(directory, file) for file in files]
def read_image_with_pillow(image_path):
try:
img = Image.open(image_path)
img.verify()
return img
except (IOError, SyntaxError) as e:
print(f"File {image_path} is not an image.")
return None
def read_image_with_opencv(image_path):
img = cv2.imread(image_path)
if img is None:
print(f"File {image_path} is not an image.")
return img
def is_image_file(file_path):
image_extensions = ('.jpg', '.jpeg', '.png', '.bmp', '.gif', '.tiff')
return file_path.lower().endswith(image_extensions)
def process_all_images_in_directory(directory, use_pillow=True):
files = get_all_files_in_directory(directory)
for file in files:
if is_image_file(file):
if use_pillow:
img = read_image_with_pillow(file)
else:
img = read_image_with_opencv(file)
if img is not None:
# 在这里处理图像文件
print(f"Processing image: {file}")
示例调用
directory = "path/to/your/images"
process_all_images_in_directory(directory, use_pillow=True)
在上述完整代码中,我们结合os模块获取文件列表、Pillow或OpenCV库读取图像文件,并通过循环结构遍历文件,同时使用文件格式检查函数过滤非图像文件,实现了遍历指定目录下所有图像文件的功能。
六、优化和扩展
1. 批量处理图像
在实际应用中,我们可能需要对图像进行批量处理。例如,调整图像大小、转换图像格式、应用滤镜等。我们可以在读取图像后,调用相应的图像处理函数。
def resize_image(img, size=(128, 128)):
return img.resize(size)
def convert_image_to_grayscale(img):
return img.convert('L')
def process_all_images_in_directory(directory, use_pillow=True):
files = get_all_files_in_directory(directory)
for file in files:
if is_image_file(file):
if use_pillow:
img = read_image_with_pillow(file)
if img is not None:
img = resize_image(img)
img = convert_image_to_grayscale(img)
img.show()
else:
img = read_image_with_opencv(file)
if img is not None:
img = cv2.resize(img, (128, 128))
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imshow('Processed Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
在上述代码中,我们添加了图像处理函数resize_image()
和convert_image_to_grayscale()
,并在读取图像后调用这些函数进行图像处理。
2. 多线程或多进程处理
对于大量图像文件,单线程处理可能效率较低。我们可以利用多线程或多进程技术提高处理效率。
import threading
def process_image_thread(file, use_pillow):
if use_pillow:
img = read_image_with_pillow(file)
if img is not None:
img = resize_image(img)
img = convert_image_to_grayscale(img)
img.show()
else:
img = read_image_with_opencv(file)
if img is not None:
img = cv2.resize(img, (128, 128))
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imshow('Processed Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
def process_all_images_in_directory(directory, use_pillow=True):
files = get_all_files_in_directory(directory)
threads = []
for file in files:
if is_image_file(file):
t = threading.Thread(target=process_image_thread, args=(file, use_pillow))
threads.append(t)
t.start()
for t in threads:
t.join()
在上述代码中,我们使用Python的threading
模块创建多个线程并发处理图像文件。
七、总结
本文介绍了如何利用Python遍历一个文件中的所有图像,主要分为以下几个步骤:
- 使用os模块获取文件列表:通过
os.listdir()
方法获取指定目录下的所有文件,并结合os.path.join()
获取完整路径。 - 使用Pillow或OpenCV库读取图像:分别介绍了如何使用Pillow和OpenCV库读取图像文件,并验证文件是否为有效的图像。
- 利用循环结构遍历文件:通过for循环遍历文件列表,并调用相应的图像读取方法。
- 检查文件格式过滤非图像文件:通过检查文件扩展名,确保只处理图像文件。
- 综合实现遍历图像文件的完整代码:结合上述各个步骤,提供了一个完整的Python脚本,实现遍历指定目录下所有图像文件的功能。
- 优化和扩展:介绍了批量处理图像和多线程处理的优化方法。
通过本文的介绍,读者可以掌握如何利用Python遍历一个文件中的所有图像,并进行相应的图像处理操作。
相关问答FAQs:
如何在Python中识别文件中的图像格式?
在遍历文件中的图像时,首先需要确定文件支持的图像格式。常见的格式包括JPEG、PNG、GIF等。可以使用Python的imghdr
模块来检测文件类型,或使用PIL
库(Pillow)来打开文件并检查其格式。这将帮助您筛选出文件夹中包含的图像。
如何使用Python遍历指定文件夹中的所有图像文件?
可以使用os
模块和glob
模块来遍历指定文件夹中的图像文件。利用os.listdir()
方法可以列出文件夹内所有文件,而glob.glob()
则可以通过指定图像文件的扩展名(如*.jpg
或*.png
)来筛选出图像文件。通过循环遍历这些文件名,可以轻松处理每个图像。
在遍历图像文件时,如何处理可能出现的错误?
在处理图像文件时,可能会遇到文件损坏或无效格式等问题。建议使用try...except
语句来捕获异常,例如在打开图像时可能引发的IOError
。这样可以确保程序不会因为单个文件的问题而中断,从而提高代码的健壮性和用户体验。
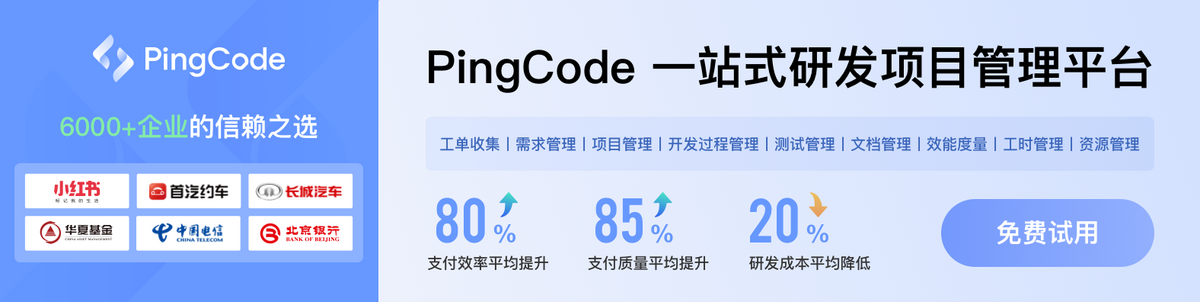