如何用Python算商品销售价格
使用Python计算商品销售价格的关键点包括:正确获取商品原价、应用适当的折扣或增值税、考虑多种商品组合的价格计算。其中,正确获取商品原价是最关键的一步,因为它决定了后续所有计算的基础。在这篇文章中,我们将详细探讨这些关键点,并介绍如何用Python编写代码来实现这些计算。
一、获取商品原价
在计算商品销售价格之前,第一步是获取商品的原价。原价通常是商家设定的初始价格,可能会存在于数据库、电子表格或者其他数据源中。在Python中,我们可以使用多种方法来获取这些数据,比如从CSV文件读取或者通过API获取。
1. 从CSV文件读取商品原价
CSV文件是一种常见的数据存储格式,下面是一个简单的示例:
import csv
def read_prices_from_csv(file_path):
prices = {}
with open(file_path, mode='r') as file:
csv_reader = csv.reader(file)
next(csv_reader) # 跳过表头
for row in csv_reader:
product_name = row[0]
price = float(row[1])
prices[product_name] = price
return prices
假设CSV文件包含两列:商品名称和原价
file_path = 'product_prices.csv'
prices = read_prices_from_csv(file_path)
print(prices)
在这个例子中,我们定义了一个函数 read_prices_from_csv
,它从CSV文件中读取商品名称和原价,并将它们存储在一个字典中。
2. 通过API获取商品原价
有时候商品的原价可能存储在远程服务器上,需要通过API来获取。这时我们可以使用Python的 requests
库:
import requests
def get_prices_from_api(api_url):
response = requests.get(api_url)
data = response.json()
prices = {item['name']: item['price'] for item in data}
return prices
假设API返回一个包含商品名称和原价的JSON数组
api_url = 'https://api.example.com/product_prices'
prices = get_prices_from_api(api_url)
print(prices)
在这个示例中,我们定义了一个函数 get_prices_from_api
,它从API获取商品名称和原价,并将它们存储在一个字典中。
二、应用折扣或增值税
获取了商品原价后,接下来我们需要考虑如何应用折扣或增值税。这一步非常关键,因为不同的商品可能有不同的折扣率或税率。
1. 应用固定折扣
如果所有商品都有一个固定折扣,我们可以很容易地计算折后价格:
def apply_discount(prices, discount_percentage):
discounted_prices = {}
for product, price in prices.items():
discounted_price = price * (1 - discount_percentage / 100)
discounted_prices[product] = discounted_price
return discounted_prices
假设折扣率为10%
discount_percentage = 10
discounted_prices = apply_discount(prices, discount_percentage)
print(discounted_prices)
2. 应用增值税
如果需要应用增值税,我们可以类似地进行计算:
def apply_tax(prices, tax_percentage):
taxed_prices = {}
for product, price in prices.items():
taxed_price = price * (1 + tax_percentage / 100)
taxed_prices[product] = taxed_price
return taxed_prices
假设增值税率为15%
tax_percentage = 15
taxed_prices = apply_tax(prices, tax_percentage)
print(taxed_prices)
三、考虑多种商品组合的价格计算
在实际销售中,消费者通常不会只购买单一商品,因此我们需要考虑多种商品组合的价格计算。这里我们需要处理购物车中商品的总价格计算。
1. 计算购物车总价格
下面是一个简单的示例,计算购物车中所有商品的总价格:
def calculate_total_price(cart, prices):
total_price = 0
for product, quantity in cart.items():
total_price += prices[product] * quantity
return total_price
假设购物车包含商品名称和数量
cart = {'Product A': 2, 'Product B': 3}
total_price = calculate_total_price(cart, discounted_prices)
print(f'Total price: {total_price}')
2. 应用购物车级别的折扣或税率
有时候,折扣或税率是针对整个购物车的,而不是单个商品。这时我们需要在计算总价格后再应用这些折扣或税率:
def apply_cart_discount(total_price, discount_percentage):
return total_price * (1 - discount_percentage / 100)
def apply_cart_tax(total_price, tax_percentage):
return total_price * (1 + tax_percentage / 100)
假设购物车级别的折扣率和税率
cart_discount_percentage = 5
cart_tax_percentage = 10
total_price_with_discount = apply_cart_discount(total_price, cart_discount_percentage)
total_price_with_tax = apply_cart_tax(total_price_with_discount, cart_tax_percentage)
print(f'Total price after discount and tax: {total_price_with_tax}')
四、总结
通过上述步骤,我们可以使用Python有效地计算商品销售价格。关键步骤包括:正确获取商品原价、应用适当的折扣或增值税、考虑多种商品组合的价格计算。每一步都需要仔细处理,以确保最终计算结果的准确性。
使用Python进行这些计算的好处是灵活性和可扩展性。我们可以轻松地调整折扣率、税率,甚至添加更多的计算逻辑,以适应不同的业务需求。
通过合理的编程实践和代码优化,我们可以确保计算过程的高效和准确。这不仅有助于提高业务运营效率,还能增强客户满意度和信任度。
相关问答FAQs:
如何使用Python计算商品的折扣价格?
在Python中,计算商品的折扣价格可以通过简单的数学运算实现。首先,您需要确定原价和折扣率。使用以下公式可以轻松计算折扣后的价格:
original_price = 100 # 原价
discount_rate = 0.2 # 折扣率(20%)
discounted_price = original_price * (1 - discount_rate)
print(discounted_price)
这段代码会输出折扣后的价格。您可以根据需要调整原价和折扣率。
如何在Python中计算商品的税后价格?
要计算商品的税后价格,需要知道商品的原价和税率。通过以下代码可以实现:
original_price = 100 # 原价
tax_rate = 0.1 # 税率(10%)
taxed_price = original_price * (1 + tax_rate)
print(taxed_price)
这将输出包含税费的最终价格。根据具体需求,您可以调整原价和税率。
如何使用Python创建一个商品价格计算器?
您可以创建一个简单的商品价格计算器,用户输入原价、折扣和税率,程序输出最终价格。以下是示例代码:
def calculate_final_price(original_price, discount_rate, tax_rate):
discounted_price = original_price * (1 - discount_rate)
final_price = discounted_price * (1 + tax_rate)
return final_price
# 用户输入
original_price = float(input("请输入商品原价: "))
discount_rate = float(input("请输入折扣率(如20%请输入0.2): "))
tax_rate = float(input("请输入税率(如10%请输入0.1): "))
final_price = calculate_final_price(original_price, discount_rate, tax_rate)
print(f"最终销售价格为: {final_price}")
以上代码将帮助用户根据输入的信息计算出最终销售价格。
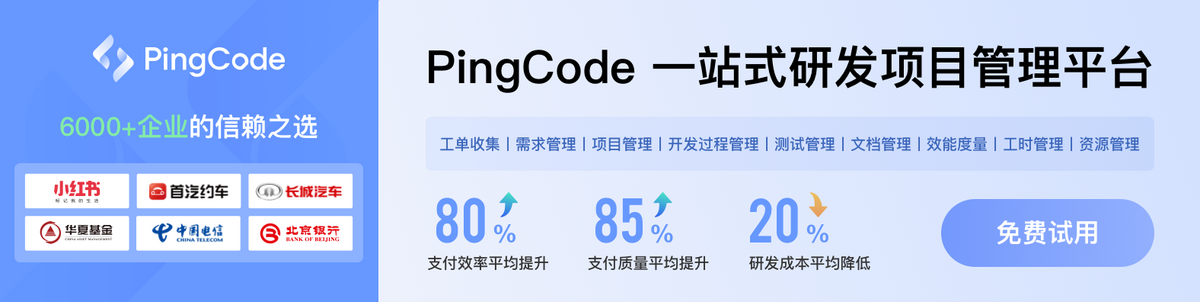