Python 如何在一个图中画多个子图
要在一个图中画多个子图,可以使用 Matplotlib 库、通过 plt.subplots()
函数、创建子图和设置布局。其中,plt.subplots()
函数是最常见的实现方式,它允许你轻松地创建一个包含多个子图的图形窗口。使用 Matplotlib 库可以通过灵活的布局和丰富的绘图功能,实现专业的图形展示效果。下面我们将详细讨论如何使用 Matplotlib 库在一个图中绘制多个子图,并介绍如何通过不同的方法来定制和优化子图的布局。
一、Matplotlib 库概述
Matplotlib 是 Python 中最流行的二维绘图库之一,广泛用于数据可视化。它的功能强大且易于使用,支持多种图表类型,包括折线图、散点图、柱状图、直方图等。Matplotlib 提供了丰富的 API,允许用户高度自定义图表的外观和布局。
1、安装 Matplotlib
在开始使用 Matplotlib 之前,需要确保已安装该库。可以通过以下命令安装:
pip install matplotlib
二、使用 plt.subplots()
创建子图
plt.subplots()
是 Matplotlib 中创建子图的主要方法。它返回一个包含子图的 Figure 对象和一个包含 Axes 对象的数组。通过这种方式,用户可以轻松地在一个图形窗口中绘制多个子图。
1、基本用法
plt.subplots()
函数的基本用法如下:
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
绘制子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].plot([1, 2, 3], [9, 4, 1])
plt.show()
2、自定义子图布局
可以通过 plt.subplots()
函数的参数自定义子图的布局和外观。例如,可以设置子图的尺寸、间距等:
import matplotlib.pyplot as plt
创建 2 行 3 列的子图,设置图形尺寸和间距
fig, axs = plt.subplots(2, 3, figsize=(12, 6), gridspec_kw={'hspace': 0.5, 'wspace': 0.3})
绘制子图
for ax in axs.flat:
ax.plot([1, 2, 3], [1, 2, 3])
plt.show()
三、使用 subplot2grid
方法
subplot2grid
方法允许用户通过网格布局精确控制子图的位置和大小。通过这种方式,可以灵活地创建复杂的子图布局。
1、基本用法
subplot2grid
的基本用法如下:
import matplotlib.pyplot as plt
创建图形
fig = plt.figure()
创建子图,设置子图在网格中的位置和大小
ax1 = plt.subplot2grid((3, 3), (0, 0), colspan=3)
ax2 = plt.subplot2grid((3, 3), (1, 0), colspan=2)
ax3 = plt.subplot2grid((3, 3), (1, 2), rowspan=2)
ax4 = plt.subplot2grid((3, 3), (2, 0))
ax5 = plt.subplot2grid((3, 3), (2, 1))
绘制子图
ax1.plot([1, 2, 3], [1, 2, 3])
ax2.plot([1, 2, 3], [3, 2, 1])
ax3.plot([1, 2, 3], [1, 4, 9])
ax4.plot([1, 2, 3], [9, 4, 1])
ax5.plot([1, 2, 3], [2, 2, 2])
plt.show()
2、自定义网格布局
可以通过 subplot2grid
方法的参数精确控制子图的网格位置和跨度:
import matplotlib.pyplot as plt
创建图形
fig = plt.figure(figsize=(8, 6))
创建子图
ax1 = plt.subplot2grid((4, 4), (0, 0), colspan=4)
ax2 = plt.subplot2grid((4, 4), (1, 0), colspan=3, rowspan=3)
ax3 = plt.subplot2grid((4, 4), (1, 3), rowspan=3)
绘制子图
ax1.plot([1, 2, 3], [1, 2, 3])
ax2.plot([1, 2, 3], [3, 2, 1])
ax3.plot([1, 2, 3], [1, 4, 9])
plt.show()
四、使用 GridSpec
创建复杂布局
GridSpec
是 Matplotlib 提供的一个高级布局管理工具,它允许用户通过网格系统精确控制子图的位置和大小。使用 GridSpec
,可以创建非常复杂的子图布局。
1、基本用法
GridSpec
的基本用法如下:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建图形
fig = plt.figure(figsize=(8, 6))
gs = gridspec.GridSpec(3, 3)
创建子图
ax1 = fig.add_subplot(gs[0, :])
ax2 = fig.add_subplot(gs[1, :-1])
ax3 = fig.add_subplot(gs[1:, -1])
ax4 = fig.add_subplot(gs[-1, 0])
ax5 = fig.add_subplot(gs[-1, -2])
绘制子图
ax1.plot([1, 2, 3], [1, 2, 3])
ax2.plot([1, 2, 3], [3, 2, 1])
ax3.plot([1, 2, 3], [1, 4, 9])
ax4.plot([1, 2, 3], [9, 4, 1])
ax5.plot([1, 2, 3], [2, 2, 2])
plt.show()
2、自定义网格布局
可以通过 GridSpec
的参数自定义网格布局的行列数、间距等:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建图形
fig = plt.figure(figsize=(10, 8))
gs = gridspec.GridSpec(4, 4, hspace=0.5, wspace=0.5)
创建子图
ax1 = fig.add_subplot(gs[0, :])
ax2 = fig.add_subplot(gs[1, :-1])
ax3 = fig.add_subplot(gs[1:, -1])
ax4 = fig.add_subplot(gs[-1, 0])
ax5 = fig.add_subplot(gs[-1, -2])
绘制子图
ax1.plot([1, 2, 3], [1, 2, 3])
ax2.plot([1, 2, 3], [3, 2, 1])
ax3.plot([1, 2, 3], [1, 4, 9])
ax4.plot([1, 2, 3], [9, 4, 1])
ax5.plot([1, 2, 3], [2, 2, 2])
plt.show()
五、为子图添加标题和标签
在创建多个子图时,为每个子图添加标题和标签有助于更好地理解图表内容。可以使用 set_title()
、set_xlabel()
和 set_ylabel()
方法来设置标题和标签。
1、为子图添加标题
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
为每个子图添加标题
axs[0, 0].set_title('Subplot 1')
axs[0, 1].set_title('Subplot 2')
axs[1, 0].set_title('Subplot 3')
axs[1, 1].set_title('Subplot 4')
绘制子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].plot([1, 2, 3], [9, 4, 1])
plt.show()
2、为子图添加标签
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
为每个子图添加标签
axs[0, 0].set_xlabel('X Label 1')
axs[0, 0].set_ylabel('Y Label 1')
axs[0, 1].set_xlabel('X Label 2')
axs[0, 1].set_ylabel('Y Label 2')
axs[1, 0].set_xlabel('X Label 3')
axs[1, 0].set_ylabel('Y Label 3')
axs[1, 1].set_xlabel('X Label 4')
axs[1, 1].set_ylabel('Y Label 4')
绘制子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].plot([1, 2, 3], [9, 4, 1])
plt.show()
六、为子图添加图例
在一个图中绘制多个子图时,添加图例可以帮助区分不同的图表内容。可以使用 legend()
方法为子图添加图例。
1、为子图添加图例
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
绘制子图并添加图例
axs[0, 0].plot([1, 2, 3], [1, 4, 9], label='Line 1')
axs[0, 0].legend()
axs[0, 1].plot([1, 2, 3], [1, 2, 3], label='Line 2')
axs[0, 1].legend()
axs[1, 0].plot([1, 2, 3], [3, 2, 1], label='Line 3')
axs[1, 0].legend()
axs[1, 1].plot([1, 2, 3], [9, 4, 1], label='Line 4')
axs[1, 1].legend()
plt.show()
七、调整子图间距和布局
在创建多个子图时,调整子图间距和布局可以提高图表的可读性。可以使用 subplots_adjust()
方法来调整子图间距。
1、调整子图间距
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
调整子图间距
fig.subplots_adjust(hspace=0.5, wspace=0.5)
绘制子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].plot([1, 2, 3], [9, 4, 1])
plt.show()
八、使用 tight_layout
方法优化布局
tight_layout
方法可以自动调整子图参数,使其适应图形区域,避免子图重叠。
1、使用 tight_layout
方法
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
绘制子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].plot([1, 2, 3], [9, 4, 1])
自动调整子图参数
fig.tight_layout()
plt.show()
九、将子图保存为图像文件
在完成多个子图的创建和调整后,可以将图形保存为图像文件。可以使用 savefig()
方法将图形保存为不同格式的文件。
1、保存图形为图像文件
import matplotlib.pyplot as plt
创建 2 行 2 列的子图
fig, axs = plt.subplots(2, 2)
绘制子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].plot([1, 2, 3], [9, 4, 1])
保存图形为图像文件
fig.savefig('subplots.png')
plt.show()
通过上述方法,可以在一个图中绘制多个子图,并通过自定义布局、添加标题和标签、调整间距、添加图例等方式,使图形更加美观和易于理解。Matplotlib 提供了丰富的功能和灵活的布局选项,适用于各种数据可视化需求。
相关问答FAQs:
如何在Python中创建多个子图?
在Python中,可以使用Matplotlib库轻松创建多个子图。通过调用plt.subplots()
函数,可以指定行数和列数来生成一个包含多个子图的图形。例如,使用fig, axs = plt.subplots(2, 2)
可以创建一个2×2的子图布局。之后,可以通过axs
数组访问每个子图并绘制不同的数据。
使用Seaborn是否可以方便地绘制多个子图?
Seaborn库不仅可以用于绘制美观的统计图形,还可以通过FacetGrid
来轻松创建多个子图。使用FacetGrid
,可以根据某一分类变量将数据分成多个子图,从而展示不同类别的分布情况。这种方法在处理复杂数据时特别有效。
在绘制多个子图时,如何调整子图间距?
在使用Matplotlib绘制多个子图时,可以通过plt.subplots_adjust()
函数来调整子图之间的间距。该函数允许用户设置上下左右的边距参数,比如plt.subplots_adjust(hspace=0.5, wspace=0.5)
可以增大子图之间的垂直和水平间距,使得图形布局更加美观,避免重叠。
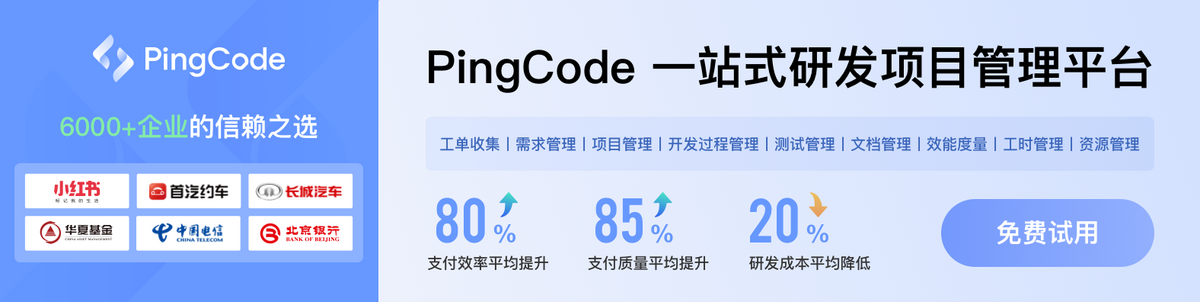