Python 字符串去重数据库的方法:使用集合数据结构、使用字典数据结构、使用 pandas 库、使用 SQL 语句。本文将详细介绍其中的使用集合数据结构方法。集合是一种无序且不重复的元素集合,非常适合用于去重操作。通过将字符串拆分为单个字符或单词,然后存入集合,可以轻松去除重复项。接下来,我们将从多方面进行深入探讨。
一、使用集合数据结构
集合(set)是 Python 提供的一种内置数据结构,它具有无序且不重复的特性,非常适合用来进行去重操作。
1、基本原理
在 Python 中,集合是一种包含不重复元素的数据结构。可以通过将字符串拆分成单个字符或单词,然后将这些字符或单词添加到集合中,自动去除重复项。
def remove_duplicates(input_string):
unique_chars = set(input_string)
return ''.join(unique_chars)
2、代码示例
2.1、去除重复字符
我们可以通过将字符串转换为集合,然后再转换为字符串,来去除字符串中的重复字符。
input_string = "aabbccdd"
unique_string = ''.join(set(input_string))
print(unique_string) # 输出:abcd
2.2、去除重复单词
如果我们需要去除字符串中的重复单词,可以先将字符串拆分为单词列表,然后使用集合进行去重。
input_string = "this is a test test string"
words = input_string.split()
unique_words = list(set(words))
unique_string = ' '.join(unique_words)
print(unique_string) # 输出:this is a test string
3、优缺点分析
3.1、优点
- 简单易用:集合操作简单,代码简洁明了。
- 高效:集合的查找和插入操作都是 O(1) 的时间复杂度。
3.2、缺点
- 无序性:集合是无序的,不能保证去重后的元素顺序与原始字符串顺序一致。
- 不适合大文本:对于非常大的文本,内存消耗可能较高。
二、使用字典数据结构
字典(dict)是另一种可以用来去重的 Python 内置数据结构。与集合不同,字典还可以存储每个元素的频率或其他相关信息。
1、基本原理
通过将字符串拆分为单个字符或单词,然后将这些字符或单词作为字典的键,自动去除重复项。
def remove_duplicates(input_string):
unique_chars = dict.fromkeys(input_string)
return ''.join(unique_chars)
2、代码示例
2.1、去除重复字符
与集合类似,我们可以通过字典来去除字符串中的重复字符。
input_string = "aabbccdd"
unique_string = ''.join(dict.fromkeys(input_string))
print(unique_string) # 输出:abcd
2.2、去除重复单词
字典也可以用来去除字符串中的重复单词。
input_string = "this is a test test string"
words = input_string.split()
unique_words = list(dict.fromkeys(words))
unique_string = ' '.join(unique_words)
print(unique_string) # 输出:this is a test string
3、优缺点分析
3.1、优点
- 顺序保留:在 Python 3.7 及以上版本中,字典是有序的,可以保留插入顺序。
- 高效:字典的查找和插入操作都是 O(1) 的时间复杂度。
3.2、缺点
- 内存消耗:字典的内存消耗比集合稍高。
- 复杂性增加:相比集合,字典的操作稍复杂一些。
三、使用 pandas 库
pandas 是一个强大的数据处理库,提供了丰富的数据操作功能。我们也可以利用 pandas 库来进行字符串去重操作。
1、基本原理
通过将字符串转换为 pandas 的 Series 对象,然后使用 pandas 提供的去重函数。
import pandas as pd
def remove_duplicates(input_string):
words = input_string.split()
unique_words = pd.Series(words).drop_duplicates().tolist()
return ' '.join(unique_words)
2、代码示例
2.1、去除重复字符
虽然 pandas 更适合处理数据帧,但也可以用来处理简单的字符串去重操作。
import pandas as pd
input_string = "aabbccdd"
chars = list(input_string)
unique_chars = pd.Series(chars).drop_duplicates().tolist()
unique_string = ''.join(unique_chars)
print(unique_string) # 输出:abcd
2.2、去除重复单词
pandas 在处理复杂文本时非常方便,可以轻松去除重复单词。
import pandas as pd
input_string = "this is a test test string"
words = input_string.split()
unique_words = pd.Series(words).drop_duplicates().tolist()
unique_string = ' '.join(unique_words)
print(unique_string) # 输出:this is a test string
3、优缺点分析
3.1、优点
- 功能强大:pandas 提供了丰富的数据处理功能,可以应对复杂的数据处理需求。
- 易于扩展:可以结合其他 pandas 函数进行更多的数据操作。
3.2、缺点
- 依赖性:需要安装 pandas 库,增加了额外的依赖。
- 性能开销:对于简单的去重操作,性能不如集合和字典。
四、使用 SQL 语句
对于存储在数据库中的字符串数据,可以使用 SQL 语句来进行去重操作。通过 SELECT DISTINCT 语句,可以轻松去除重复项。
1、基本原理
通过 SQL 语句进行查询,使用 DISTINCT 关键字去除重复项。
SELECT DISTINCT column_name
FROM table_name;
2、代码示例
2.1、去除重复字符
虽然 SQL 主要用于结构化数据查询,但我们也可以将字符串数据存入数据库,通过 SQL 进行去重。
import sqlite3
def remove_duplicates_from_db(input_string):
conn = sqlite3.connect(':memory:')
c = conn.cursor()
c.execute('CREATE TABLE chars (char TEXT)')
c.executemany('INSERT INTO chars (char) VALUES (?)', [(char,) for char in input_string])
c.execute('SELECT DISTINCT char FROM chars')
unique_chars = ''.join(row[0] for row in c.fetchall())
conn.close()
return unique_chars
input_string = "aabbccdd"
unique_string = remove_duplicates_from_db(input_string)
print(unique_string) # 输出:abcd
2.2、去除重复单词
对于存储在数据库中的文本数据,可以通过 SQL 语句进行去重。
import sqlite3
def remove_duplicates_from_db(input_string):
conn = sqlite3.connect(':memory:')
c = conn.cursor()
c.execute('CREATE TABLE words (word TEXT)')
words = input_string.split()
c.executemany('INSERT INTO words (word) VALUES (?)', [(word,) for word in words])
c.execute('SELECT DISTINCT word FROM words')
unique_words = ' '.join(row[0] for row in c.fetchall())
conn.close()
return unique_words
input_string = "this is a test test string"
unique_string = remove_duplicates_from_db(input_string)
print(unique_string) # 输出:this is a test string
3、优缺点分析
3.1、优点
- 适用于大数据:对于大规模数据集,SQL 查询非常高效。
- 功能强大:可以结合其他 SQL 语句进行复杂的数据操作。
3.2、缺点
- 复杂性:需要掌握 SQL 语法,增加了学习成本。
- 依赖数据库:需要配置和管理数据库,增加了系统复杂性。
结论
去除字符串中的重复项是一个常见的数据处理需求,Python 提供了多种方法来实现这一目标,包括集合、字典、pandas 库和 SQL 语句。每种方法都有其优缺点,选择哪种方法取决于具体的应用场景和需求。
使用集合数据结构:简单易用,适合小规模数据去重。
使用字典数据结构:保留顺序,适合需要顺序保留的场景。
使用 pandas 库:功能强大,适合复杂数据处理需求。
使用 SQL 语句:高效处理大规模数据,适合数据库存储的数据去重。
通过上述方法,你可以根据具体需求选择最适合的方法来去除字符串中的重复项,提高数据处理效率。
相关问答FAQs:
如何在Python中去除字符串中的重复字符并存入数据库?
在Python中,可以使用集合(set)来去除字符串中的重复字符。将字符串转为集合后,再将其转换回字符串,便可得到去重后的结果。之后,可以使用数据库连接库(如SQLite、MySQL等)将结果存入数据库。示例代码如下:
import sqlite3
def remove_duplicates(input_string):
return ''.join(set(input_string))
# 示例字符串
original_string = "hello world"
unique_string = remove_duplicates(original_string)
# 数据库连接
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute("CREATE TABLE IF NOT EXISTS strings (unique_string TEXT)")
c.execute("INSERT INTO strings (unique_string) VALUES (?)", (unique_string,))
conn.commit()
conn.close()
如何从数据库中提取字符串并去除重复字符?
可以通过Python的数据库连接库,从数据库中提取字符串数据,然后使用相同的去重方法进行处理。提取数据后,利用前面提到的去重函数可以得到结果。示例代码如下:
# 数据库连接
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute("SELECT unique_string FROM strings")
rows = c.fetchall()
for row in rows:
print(remove_duplicates(row[0]))
conn.close()
去重后的字符串如何进行其他操作,例如计数或分析?
去重后的字符串可以进行各种操作,例如字母计数、词频分析等。可以使用Python的内置函数和库,如collections.Counter来实现。例如,可以统计去重字符串中的各个字符出现的次数。示例代码如下:
from collections import Counter
unique_counts = Counter(unique_string)
print(unique_counts)
通过这些操作,可以更深入地分析处理后的字符串数据。
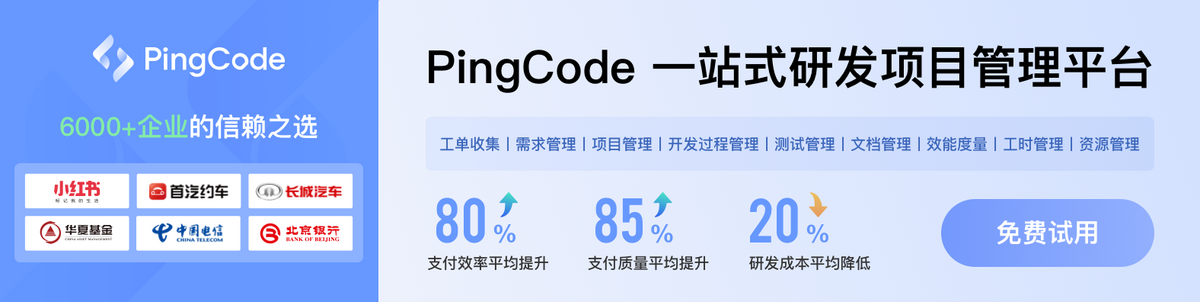