在Python中,增减时间戳的天数可以通过使用datetime
模块实现。具体方法包括使用timedelta
对象、处理时间戳格式、以及考虑时区问题。接下来,我们将详细讨论这些方法,并通过代码示例展示如何在时间戳的基础上增减天数。
一、使用datetime
模块的基础知识
datetime
模块是Python标准库中的一个模块,提供了处理日期和时间的各种函数。它包含以下几个主要的类:
datetime.date
:表示日期(年、月、日)。datetime.time
:表示时间(时、分、秒、微秒)。datetime.datetime
:表示日期和时间。datetime.timedelta
:表示时间间隔,即两个日期或时间之间的差异。
使用这些类,我们可以方便地进行日期和时间的计算。
二、timedelta
对象的使用
timedelta
对象用于表示两个日期或时间之间的时间差。我们可以通过创建一个timedelta
对象并将其添加到一个日期时间对象中来增减天数。
from datetime import datetime, timedelta
当前时间戳
current_timestamp = datetime.now()
print(f"当前时间戳: {current_timestamp}")
增加7天
new_timestamp = current_timestamp + timedelta(days=7)
print(f"增加7天后的时间戳: {new_timestamp}")
减少7天
new_timestamp = current_timestamp - timedelta(days=7)
print(f"减少7天后的时间戳: {new_timestamp}")
三、处理时间戳格式
时间戳通常表示为一个浮点数,表示从1970年1月1日(Unix纪元)以来的秒数。我们可以使用datetime.fromtimestamp
和datetime.timestamp
方法来处理时间戳。
import time
当前时间戳
current_timestamp = time.time()
print(f"当前Unix时间戳: {current_timestamp}")
将时间戳转换为datetime对象
current_datetime = datetime.fromtimestamp(current_timestamp)
print(f"时间戳转换为datetime对象: {current_datetime}")
增加7天
new_datetime = current_datetime + timedelta(days=7)
new_timestamp = new_datetime.timestamp()
print(f"增加7天后的Unix时间戳: {new_timestamp}")
减少7天
new_datetime = current_datetime - timedelta(days=7)
new_timestamp = new_datetime.timestamp()
print(f"减少7天后的Unix时间戳: {new_timestamp}")
四、考虑时区问题
在处理时间戳和日期时间对象时,时区问题是一个需要注意的重要方面。Python的pytz
库提供了时区支持,允许我们在不同的时区之间进行转换。
from datetime import datetime, timedelta
import pytz
当前时间戳
current_timestamp = datetime.now(pytz.utc)
print(f"当前UTC时间戳: {current_timestamp}")
增加7天
new_timestamp = current_timestamp + timedelta(days=7)
print(f"增加7天后的UTC时间戳: {new_timestamp}")
将UTC时间转换为本地时区时间
local_timezone = pytz.timezone('Asia/Shanghai')
local_timestamp = new_timestamp.astimezone(local_timezone)
print(f"增加7天后的本地时间戳: {local_timestamp}")
减少7天
new_timestamp = current_timestamp - timedelta(days=7)
print(f"减少7天后的UTC时间戳: {new_timestamp}")
将UTC时间转换为本地时区时间
local_timestamp = new_timestamp.astimezone(local_timezone)
print(f"减少7天后的本地时间戳: {local_timestamp}")
五、总结
在Python中处理时间戳和日期时间的增减天数操作主要通过datetime
模块完成。使用timedelta
对象可以方便地进行日期和时间的计算,而处理时间戳格式和时区转换可以确保我们的时间计算在不同环境下都能正确执行。通过以上方法,我们可以轻松地在时间戳的基础上增减天数,满足各种应用需求。
相关问答FAQs:
如何使用Python将时间戳转换为可读的日期格式?
在Python中,可以使用datetime
模块将时间戳转换为可读的日期格式。具体方法是使用datetime.fromtimestamp()
函数。例如:
import datetime
timestamp = 1633072800 # 示例时间戳
date_time = datetime.datetime.fromtimestamp(timestamp)
print(date_time.strftime('%Y-%m-%d %H:%M:%S')) # 输出可读日期格式
这样可以将时间戳转换为"年-月-日 时:分:秒"的格式。
如何在Python中处理时间戳的时区问题?
时间戳通常是基于UTC时间的。在处理时区时,可以使用pytz
库配合datetime
模块进行转换。首先,确保安装了pytz
库,然后可以这样做:
import datetime
import pytz
timestamp = 1633072800
utc_time = datetime.datetime.fromtimestamp(timestamp, pytz.utc)
local_time = utc_time.astimezone(pytz.timezone('Asia/Shanghai')) # 转换为上海时区
print(local_time.strftime('%Y-%m-%d %H:%M:%S'))
这样可以确保你得到正确的本地时间。
Python中如何进行时间戳的运算,增减天数?
在Python中,可以通过timedelta
类来增减时间。例如,要在一个时间戳的基础上增加5天,可以这样处理:
import datetime
timestamp = 1633072800 # 示例时间戳
date_time = datetime.datetime.fromtimestamp(timestamp)
new_date_time = date_time + datetime.timedelta(days=5) # 增加5天
new_timestamp = int(new_date_time.timestamp()) # 转换回时间戳
print(new_timestamp)
同样的方式可以用来减去天数,只需将days
参数设置为负数即可。
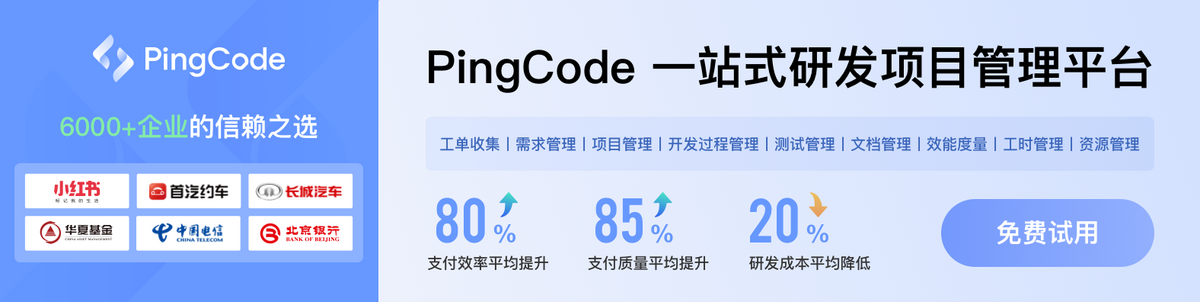