Python中删除文件中的某一行的方法有:使用临时文件替换原文件、读取文件内容并重写文件、使用文件行号指定删除等。 其中,使用临时文件替换原文件是一种常见且安全的方法,能够有效避免意外删除数据。下面将详细介绍这种方法。
一、使用临时文件替换原文件
在处理文件时,最常见的方法之一是创建一个临时文件,在这个临时文件中写入需要保留的内容,最后用临时文件替换原文件。具体步骤如下:
- 读取原文件内容:首先,将原文件的内容逐行读取到内存中。
- 写入临时文件:在写入临时文件的过程中,跳过需要删除的行。
- 替换原文件:将临时文件重命名为原文件名,完成替换。
通过这种方法,可以有效避免直接操作原文件可能带来的风险。以下是一个完整的Python代码示例:
import os
def delete_line_from_file(file_path, line_number):
"""
删除文件中指定行号的行。
:param file_path: 文件路径
:param line_number: 要删除的行号(从1开始)
"""
temp_file_path = file_path + '.tmp'
with open(file_path, 'r') as read_file, open(temp_file_path, 'w') as write_file:
current_line = 1
for line in read_file:
if current_line != line_number:
write_file.write(line)
current_line += 1
os.replace(temp_file_path, file_path)
示例用法
delete_line_from_file('example.txt', 3)
二、读取文件内容并重写文件
另一种方法是直接读取文件内容到内存中,将需要保留的内容存储在列表中,然后重写文件。这种方法适用于文件较小的情况,因为它需要将文件内容全部加载到内存中。
- 读取文件内容到列表:将文件内容逐行读取到列表中。
- 删除指定行:根据行号删除指定行。
- 重写文件:将修改后的内容写回文件。
以下是代码示例:
def delete_line_from_file_in_memory(file_path, line_number):
"""
在内存中删除文件中指定行号的行,然后重写文件。
:param file_path: 文件路径
:param line_number: 要删除的行号(从1开始)
"""
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number > len(lines) or line_number < 1:
raise IndexError("行号超出范围")
del lines[line_number - 1]
with open(file_path, 'w') as file:
file.writelines(lines)
示例用法
delete_line_from_file_in_memory('example.txt', 3)
三、使用文件行号指定删除
在某些情况下,您可能希望通过特定的标识符或内容来定位要删除的行,而不是通过行号。这种方法可以通过以下步骤实现:
- 读取文件内容到列表:将文件内容逐行读取到列表中。
- 查找并删除指定行:通过特定标识符或内容查找并删除行。
- 重写文件:将修改后的内容写回文件。
以下是代码示例:
def delete_line_by_content(file_path, target_content):
"""
根据内容删除文件中的行。
:param file_path: 文件路径
:param target_content: 要删除行的内容
"""
with open(file_path, 'r') as file:
lines = file.readlines()
lines = [line for line in lines if target_content not in line]
with open(file_path, 'w') as file:
file.writelines(lines)
示例用法
delete_line_by_content('example.txt', '要删除的内容')
四、处理大文件和性能优化
当文件较大时,上述方法可能会遇到性能瓶颈。为了优化性能,可以考虑以下方法:
- 逐行处理文件:避免将整个文件内容加载到内存中,逐行处理文件内容,可以有效减少内存占用。
- 使用生成器:在读取文件时使用生成器,可以节省内存。
- 批量处理:如果需要删除多行,可以一次性读取和写入多个行,减少文件操作次数。
以下是逐行处理文件的代码示例:
import os
def delete_lines_from_large_file(file_path, line_numbers):
"""
删除大文件中指定行号的行。
:param file_path: 文件路径
:param line_numbers: 要删除的行号列表(从1开始)
"""
temp_file_path = file_path + '.tmp'
line_numbers = set(line_numbers)
with open(file_path, 'r') as read_file, open(temp_file_path, 'w') as write_file:
current_line = 1
for line in read_file:
if current_line not in line_numbers:
write_file.write(line)
current_line += 1
os.replace(temp_file_path, file_path)
示例用法
delete_lines_from_large_file('example.txt', [3, 5, 7])
五、错误处理和异常捕获
在实际应用中,处理文件操作时应考虑到可能出现的各种错误,如文件不存在、权限不足等。因此,添加错误处理和异常捕获是非常重要的。以下是改进后的代码示例:
import os
def delete_line_with_error_handling(file_path, line_number):
"""
带错误处理的删除文件中指定行号的行。
:param file_path: 文件路径
:param line_number: 要删除的行号(从1开始)
"""
try:
if not os.path.exists(file_path):
raise FileNotFoundError(f"文件 {file_path} 不存在")
temp_file_path = file_path + '.tmp'
with open(file_path, 'r') as read_file, open(temp_file_path, 'w') as write_file:
current_line = 1
for line in read_file:
if current_line != line_number:
write_file.write(line)
current_line += 1
os.replace(temp_file_path, file_path)
except FileNotFoundError as e:
print(e)
except PermissionError as e:
print(f"没有权限访问文件 {file_path}: {e}")
except Exception as e:
print(f"处理文件 {file_path} 时发生错误: {e}")
示例用法
delete_line_with_error_handling('example.txt', 3)
六、多平台兼容性
文件操作在不同操作系统上的行为可能有所不同,因此编写代码时应考虑多平台兼容性。例如,路径分隔符在Windows和Linux上不同,可以使用os.path
模块来处理路径问题。
以下是改进后的代码示例:
import os
def delete_line_cross_platform(file_path, line_number):
"""
支持跨平台的删除文件中指定行号的行。
:param file_path: 文件路径
:param line_number: 要删除的行号(从1开始)
"""
try:
if not os.path.exists(file_path):
raise FileNotFoundError(f"文件 {file_path} 不存在")
temp_file_path = os.path.join(os.path.dirname(file_path), 'temp_' + os.path.basename(file_path))
with open(file_path, 'r') as read_file, open(temp_file_path, 'w') as write_file:
current_line = 1
for line in read_file:
if current_line != line_number:
write_file.write(line)
current_line += 1
os.replace(temp_file_path, file_path)
except FileNotFoundError as e:
print(e)
except PermissionError as e:
print(f"没有权限访问文件 {file_path}: {e}")
except Exception as e:
print(f"处理文件 {file_path} 时发生错误: {e}")
示例用法
delete_line_cross_platform('example.txt', 3)
七、总结
在Python中删除文件中的某一行可以通过多种方法实现,常见的有使用临时文件替换原文件、读取文件内容并重写文件、使用文件行号指定删除等。每种方法都有其适用的场景和优缺点。在实际应用中,需要根据具体情况选择合适的方法,并注意处理可能出现的错误和异常,以确保代码的健壮性和可维护性。
相关问答FAQs:
如何在Python中删除文件中的特定行?
在Python中,可以通过读取文件的所有行,将不需要删除的行写入一个新的文件,或者直接在原文件中进行操作来删除特定行。常用的方法是使用open()
函数配合readlines()
或fileinput
模块。示例代码如下:
with open('example.txt', 'r') as file:
lines = file.readlines()
with open('example.txt', 'w') as file:
for line in lines:
if line.strip("\n") != "要删除的行内容":
file.write(line)
这种方式可以有效地删除指定内容的行。
使用Python删除文件中的空行有什么简单方法?
删除空行可以通过读取文件并检查每一行是否为空来实现。可以使用strip()
函数来判断行是否只有空格。以下是一个示例代码:
with open('example.txt', 'r') as file:
lines = file.readlines()
with open('example.txt', 'w') as file:
for line in lines:
if line.strip():
file.write(line)
这种方法可以快速清理文件中的空行。
在Python中可以通过哪些方法删除文件中的多行?
删除多行可以使用条件语句来判断哪些行需要被删除。可以将要删除的行的索引存储在一个列表中,然后在重写文件时跳过这些索引。示例代码如下:
lines_to_delete = [1, 3, 5] # 删除第2、4、6行
with open('example.txt', 'r') as file:
lines = file.readlines()
with open('example.txt', 'w') as file:
for index, line in enumerate(lines):
if index not in lines_to_delete:
file.write(line)
这种方法灵活且高效,适合处理需要删除多行的情况。
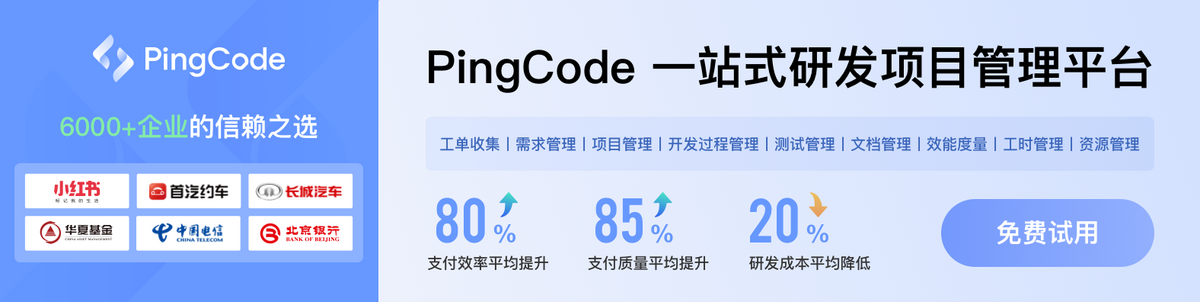