用Python开发一个笔记本程序的关键步骤包括:选择合适的开发框架、设计用户界面、实现基本功能如创建、编辑和删除笔记、以及添加额外功能如搜索和分类。 在这篇文章中,我们将重点讨论如何使用Python和一些常用的库,如Tkinter和SQLite,来开发一个功能完善的笔记本程序。
一、选择合适的开发框架
在开发笔记本程序时,选择一个合适的开发框架至关重要。Python有许多库和框架可以帮助你快速搭建图形用户界面(GUI)。
Tkinter
Tkinter是Python的标准GUI库,几乎所有的Python发行版都包含它。它非常适合用于小型到中型的桌面应用。
- 优点:内置于Python,无需额外安装,易于学习和使用。
- 缺点:对于复杂的界面设计可能显得有些笨拙。
PyQt
PyQt是一个功能强大的库,基于Qt框架,可以用来创建跨平台应用程序。
- 优点:功能强大,支持复杂的界面设计。
- 缺点:需要额外安装,学习曲线较陡。
Kivy
Kivy是一个开源的Python库,用于快速开发多点触摸应用程序。
- 优点:适用于跨平台开发,支持触摸屏设备。
- 缺点:安装和配置可能比较复杂。
二、设计用户界面
设计一个用户友好的界面是开发任何应用程序的关键。对于一个笔记本程序,用户界面应该包括以下几个基本元素:
- 主窗口:显示所有笔记的列表。
- 编辑窗口:用于创建和编辑笔记。
- 搜索栏:用于快速查找特定笔记。
主窗口设计
主窗口是用户打开应用程序后看到的第一个界面,它应该简单明了,方便用户浏览所有笔记。
import tkinter as tk
from tkinter import ttk
class NotebookApp:
def __init__(self, root):
self.root = root
self.root.title("Notebook")
self.tree = ttk.Treeview(root, columns=('Title', 'Date'))
self.tree.heading('#0', text='ID')
self.tree.heading('Title', text='Title')
self.tree.heading('Date', text='Date')
self.tree.pack(fill=tk.BOTH, expand=True)
if __name__ == "__main__":
root = tk.Tk()
app = NotebookApp(root)
root.mainloop()
编辑窗口设计
编辑窗口允许用户创建和编辑笔记。它应该包括一个文本框和保存按钮。
class EditWindow:
def __init__(self, master):
self.top = tk.Toplevel(master)
self.top.title("Edit Note")
self.text = tk.Text(self.top)
self.text.pack(fill=tk.BOTH, expand=True)
self.save_button = tk.Button(self.top, text="Save", command=self.save_note)
self.save_button.pack()
def save_note(self):
# Save note logic here
pass
三、实现基本功能
创建、编辑和删除笔记
实现笔记的创建、编辑和删除功能是笔记本程序的核心功能。我们可以使用SQLite数据库来存储笔记。
import sqlite3
class NotebookApp:
def __init__(self, root):
self.root = root
self.root.title("Notebook")
self.conn = sqlite3.connect('notes.db')
self.cursor = self.conn.cursor()
self.create_table()
self.tree = ttk.Treeview(root, columns=('Title', 'Date'))
self.tree.heading('#0', text='ID')
self.tree.heading('Title', text='Title')
self.tree.heading('Date', text='Date')
self.tree.pack(fill=tk.BOTH, expand=True)
self.load_notes()
def create_table(self):
self.cursor.execute('''CREATE TABLE IF NOT EXISTS notes
(id INTEGER PRIMARY KEY,
title TEXT,
content TEXT,
date TIMESTAMP DEFAULT CURRENT_TIMESTAMP)''')
def load_notes(self):
self.cursor.execute("SELECT id, title, date FROM notes")
for row in self.cursor.fetchall():
self.tree.insert('', 'end', text=row[0], values=(row[1], row[2]))
def save_note_to_db(self, title, content):
self.cursor.execute("INSERT INTO notes (title, content) VALUES (?, ?)", (title, content))
self.conn.commit()
self.load_notes()
def delete_note_from_db(self, note_id):
self.cursor.execute("DELETE FROM notes WHERE id=?", (note_id,))
self.conn.commit()
self.load_notes()
搜索功能
搜索功能可以帮助用户快速找到特定的笔记。可以在主窗口添加一个搜索栏,用户输入关键词后,程序会在数据库中查找匹配的笔记。
class NotebookApp:
def __init__(self, root):
self.root = root
self.root.title("Notebook")
self.conn = sqlite3.connect('notes.db')
self.cursor = self.conn.cursor()
self.create_table()
self.search_var = tk.StringVar()
self.search_bar = tk.Entry(root, textvariable=self.search_var)
self.search_bar.pack()
self.search_button = tk.Button(root, text="Search", command=self.search_notes)
self.search_button.pack()
self.tree = ttk.Treeview(root, columns=('Title', 'Date'))
self.tree.heading('#0', text='ID')
self.tree.heading('Title', text='Title')
self.tree.heading('Date', text='Date')
self.tree.pack(fill=tk.BOTH, expand=True)
self.load_notes()
def search_notes(self):
search_term = self.search_var.get()
self.cursor.execute("SELECT id, title, date FROM notes WHERE title LIKE ?", ('%' + search_term + '%',))
self.tree.delete(*self.tree.get_children())
for row in self.cursor.fetchall():
self.tree.insert('', 'end', text=row[0], values=(row[1], row[2]))
# Other methods remain the same...
四、添加额外功能
分类功能
分类功能可以帮助用户更好地组织笔记。可以在数据库中添加一个分类字段,并在界面上提供分类的选择。
class NotebookApp:
def __init__(self, root):
self.root = root
self.root.title("Notebook")
self.conn = sqlite3.connect('notes.db')
self.cursor = self.conn.cursor()
self.create_table()
self.category_var = tk.StringVar()
self.category_menu = ttk.Combobox(root, textvariable=self.category_var)
self.category_menu['values'] = ('Work', 'Personal', 'Study')
self.category_menu.pack()
self.tree = ttk.Treeview(root, columns=('Title', 'Date', 'Category'))
self.tree.heading('#0', text='ID')
self.tree.heading('Title', text='Title')
self.tree.heading('Date', text='Date')
self.tree.heading('Category', text='Category')
self.tree.pack(fill=tk.BOTH, expand=True)
self.load_notes()
def create_table(self):
self.cursor.execute('''CREATE TABLE IF NOT EXISTS notes
(id INTEGER PRIMARY KEY,
title TEXT,
content TEXT,
date TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
category TEXT)''')
def load_notes(self):
self.cursor.execute("SELECT id, title, date, category FROM notes")
for row in self.cursor.fetchall():
self.tree.insert('', 'end', text=row[0], values=(row[1], row[2], row[3]))
def save_note_to_db(self, title, content, category):
self.cursor.execute("INSERT INTO notes (title, content, category) VALUES (?, ?, ?)", (title, content, category))
self.conn.commit()
self.load_notes()
def delete_note_from_db(self, note_id):
self.cursor.execute("DELETE FROM notes WHERE id=?", (note_id,))
self.conn.commit()
self.load_notes()
五、优化用户体验
自动保存功能
自动保存功能可以防止用户在编辑过程中丢失数据。可以使用线程或定时器来定期保存笔记。
import threading
class EditWindow:
def __init__(self, master):
self.top = tk.Toplevel(master)
self.top.title("Edit Note")
self.text = tk.Text(self.top)
self.text.pack(fill=tk.BOTH, expand=True)
self.save_button = tk.Button(self.top, text="Save", command=self.save_note)
self.save_button.pack()
self.auto_save_thread = threading.Thread(target=self.auto_save)
self.auto_save_thread.start()
def save_note(self):
# Save note logic here
pass
def auto_save(self):
while True:
self.save_note()
time.sleep(60) # Save every 60 seconds
备份与恢复功能
备份与恢复功能可以保护用户数据免受意外丢失。可以定期将数据库备份到一个文件,并提供恢复功能。
import shutil
class NotebookApp:
def __init__(self, root):
self.root = root
self.root.title("Notebook")
self.conn = sqlite3.connect('notes.db')
self.cursor = self.conn.cursor()
self.create_table()
self.backup_button = tk.Button(root, text="Backup", command=self.backup_db)
self.backup_button.pack()
self.restore_button = tk.Button(root, text="Restore", command=self.restore_db)
self.restore_button.pack()
self.tree = ttk.Treeview(root, columns=('Title', 'Date'))
self.tree.heading('#0', text='ID')
self.tree.heading('Title', text='Title')
self.tree.heading('Date', text='Date')
self.tree.pack(fill=tk.BOTH, expand=True)
self.load_notes()
def backup_db(self):
shutil.copy('notes.db', 'notes_backup.db')
def restore_db(self):
shutil.copy('notes_backup.db', 'notes.db')
self.load_notes()
# Other methods remain the same...
六、测试与调试
在开发过程中,测试与调试是必不可少的步骤。可以使用Python的unittest模块来编写单元测试,确保每个功能都能正常工作。
import unittest
class TestNotebookApp(unittest.TestCase):
def setUp(self):
self.app = NotebookApp(tk.Tk())
def test_create_table(self):
self.app.create_table()
self.app.cursor.execute("SELECT name FROM sqlite_master WHERE type='table' AND name='notes'")
self.assertIsNotNone(self.app.cursor.fetchone())
def test_save_note_to_db(self):
self.app.save_note_to_db("Test Note", "This is a test note.", "Work")
self.app.cursor.execute("SELECT * FROM notes WHERE title='Test Note'")
self.assertIsNotNone(self.app.cursor.fetchone())
if __name__ == "__main__":
unittest.main()
七、发布与维护
打包应用
在开发完成后,可以使用pyinstaller等工具将Python脚本打包成可执行文件,方便用户安装和使用。
pyinstaller --onefile notebook.py
维护与更新
发布后,需要定期维护和更新应用,修复已知问题并添加新功能。可以使用版本控制工具如Git来管理代码,并使用CI/CD工具如Travis CI或GitHub Actions来自动化测试和部署过程。
总结
开发一个功能完善的笔记本程序需要考虑多个方面,从选择合适的开发框架、设计用户界面、实现基本功能,到添加额外功能、优化用户体验、测试与调试,最后发布与维护。在整个过程中,代码的可读性和可维护性尤为重要。希望这篇文章能为你提供一些有用的指导,帮助你成功开发自己的笔记本程序。
相关问答FAQs:
如何开始使用Python开发笔记本程序?
要开始使用Python开发笔记本程序,您需要安装Python及其开发环境。推荐使用Anaconda或直接从Python官网安装。接下来,您可以使用文本编辑器或集成开发环境(IDE)如PyCharm或VSCode来编写代码。选择合适的库,如Tkinter或PyQt,以创建用户界面。确保在开发过程中仔细设计程序结构,以便于未来的维护和扩展。
笔记本程序需要哪些基本功能?
一个基本的笔记本程序通常需要具备创建、编辑、保存和删除笔记的功能。用户界面应直观,支持文字格式化、搜索笔记以及分类管理等功能。此外,考虑添加标签功能,以便用户快速查找笔记。同时,支持导入和导出功能将提高用户体验,例如将笔记保存为PDF或文本文件。
如何提高笔记本程序的用户体验?
为了提高用户体验,您可以添加自动保存功能,以防止数据丢失。确保界面设计简洁且易于导航,使用合适的颜色和字体以提升可读性。引入快捷键和工具栏可以加快用户操作。同时,考虑实现云同步功能,使用户能够在不同设备间访问其笔记,增加程序的灵活性和便利性。
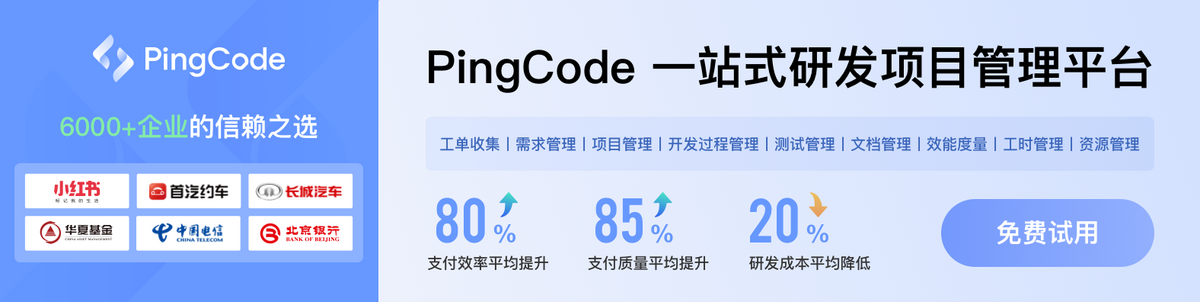