要用Python去除字符串中的一些符号,可以通过使用字符串的内建方法和正则表达式来实现。常见方法包括:使用str.replace()
方法、str.translate()
方法、以及正则表达式中的re.sub()
方法。其中,使用正则表达式的re.sub()
方法是最灵活且高效的。
例如,假设我们想去除字符串中的所有标点符号和特殊字符,只保留字母和数字,可以使用正则表达式来实现。具体而言,可以使用re.sub()
方法来匹配并替换这些符号。下面是一个示例代码:
import re
示例字符串
text = "Hello, world! This is a test string with symbols: @#%&*()"
使用正则表达式去除符号
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text)
在这个示例中,re.sub(r'[^\w\s]', '', text)
表示将所有非字母数字字符和空白字符替换为空字符串,从而去除它们。接下来,我们将详细介绍如何使用上述方法来去除字符串中的符号,并探讨其他相关技术细节。
一、使用str.replace()
方法
1.1、基本用法
str.replace()
方法允许我们替换字符串中的特定子串。例如,如果我们想去除所有逗号,可以这样做:
text = "Hello, world!"
cleaned_text = text.replace(',', '')
print(cleaned_text) # 输出:Hello world!
1.2、替换多个符号
如果我们要替换多个符号,可以嵌套多个replace()
方法:
text = "Hello, world! This is a test string."
cleaned_text = text.replace(',', '').replace('!', '')
print(cleaned_text) # 输出:Hello world This is a test string.
虽然这种方法简单直接,但如果符号列表较长,代码会显得冗长且难以维护。
二、使用str.translate()
方法
2.1、基本用法
str.translate()
方法结合str.maketrans()
方法,可以一次性替换多个字符。首先,创建一个翻译表,然后使用translate()
方法进行替换。例如:
text = "Hello, world! This is a test string."
translation_table = str.maketrans('', '', ',!')
cleaned_text = text.translate(translation_table)
print(cleaned_text) # 输出:Hello world This is a test string
2.2、去除更多符号
我们可以在翻译表中添加更多的符号:
text = "Hello, world! This is a test string with symbols: @#%&*()"
translation_table = str.maketrans('', '', ',!@#%&*()')
cleaned_text = text.translate(translation_table)
print(cleaned_text) # 输出:Hello world This is a test string with symbols
这种方法比replace()
更高效,但不如正则表达式灵活。
三、使用正则表达式(re.sub()
方法)
3.1、基本用法
正则表达式提供了强大的字符串处理能力。我们可以使用re.sub()
方法来匹配和替换字符。例如,去除所有非字母数字字符和空白字符:
import re
text = "Hello, world! This is a test string with symbols: @#%&*()"
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text) # 输出:Hello world This is a test string with symbols
3.2、定制化匹配
我们可以根据需要定制正则表达式。例如,去除所有标点符号:
import re
text = "Hello, world! This is a test string with symbols: @#%&*()"
cleaned_text = re.sub(r'[.,!@#%&*()]', '', text)
print(cleaned_text) # 输出:Hello world This is a test string with symbols
3.3、忽略大小写
如果需要忽略大小写匹配,可以使用re.IGNORECASE
标志:
import re
text = "Hello, World! This is a Test string."
cleaned_text = re.sub(r'[t]', '', text, flags=re.IGNORECASE)
print(cleaned_text) # 输出:Hello, World! his is a es string.
四、综合应用
4.1、去除用户定义的符号
我们可以创建一个函数,允许用户传入要去除的符号列表:
import re
def remove_symbols(text, symbols):
pattern = f"[{re.escape(symbols)}]"
return re.sub(pattern, '', text)
text = "Hello, world! This is a test string with symbols: @#%&*()"
symbols = ",!@#%&*()"
cleaned_text = remove_symbols(text, symbols)
print(cleaned_text) # 输出:Hello world This is a test string with symbols
4.2、处理大文本
对于大文本,使用正则表达式可以提高处理效率。我们可以将文本分块处理,减少内存占用:
import re
def remove_symbols_from_large_text(text, symbols):
pattern = f"[{re.escape(symbols)}]"
cleaned_text = []
chunk_size = 1000 # 定义块大小
for i in range(0, len(text), chunk_size):
chunk = text[i:i + chunk_size]
cleaned_chunk = re.sub(pattern, '', chunk)
cleaned_text.append(cleaned_chunk)
return ''.join(cleaned_text)
large_text = "Hello, world! This is a test string with symbols: @#%&*()" * 1000
symbols = ",!@#%&*()"
cleaned_text = remove_symbols_from_large_text(large_text, symbols)
print(cleaned_text[:100]) # 输出前100个字符以示例
4.3、预处理文本数据
在数据预处理中,去除符号是常见的任务。我们可以将这一步集成到数据清洗流程中:
import pandas as pd
import re
def clean_column(text, symbols):
pattern = f"[{re.escape(symbols)}]"
return re.sub(pattern, '', text)
示例数据框
data = {'text': ["Hello, world!", "Data cleaning is important.", "Symbols: @#%&*()"]}
df = pd.DataFrame(data)
定义要去除的符号
symbols = ",!@#%&*()"
应用到数据框的每一行
df['cleaned_text'] = df['text'].apply(lambda x: clean_column(x, symbols))
print(df)
五、总结
通过本文,我们探讨了多种使用Python去除字符串中符号的方法,包括str.replace()
、str.translate()
和正则表达式re.sub()
。每种方法都有其优点和适用场景:
str.replace()
:适用于替换少量已知符号,代码简单直观。str.translate()
:适用于替换多个已知符号,效率较高。- 正则表达式
re.sub()
:最灵活,适用于复杂的符号替换需求。
在实际应用中,选择合适的方法可以提高代码的可读性和执行效率。希望本文能够帮助你在处理字符串符号时做出最佳选择。
相关问答FAQs:
如何使用Python去除字符串中的特定符号?
要去除字符串中的特定符号,可以使用str.replace()
方法或re
模块的正则表达式。str.replace()
适合简单的替换,而re.sub()
可以处理更复杂的模式。例如,使用re.sub(r'[符号]', '', your_string)
可以删除所有匹配的符号。
Python中有哪些方法可以批量去除字符串中的符号?
批量去除字符串中的符号可以借助string
模块中的string.punctuation
来实现。结合列表解析或join()
方法,可以方便地创建一个新的字符串,只包含所需的字符。例如:
import string
your_string = "Hello, World! @2023"
cleaned_string = ''.join(char for char in your_string if char not in string.punctuation)
如何处理字符串中间隔的符号而不影响其他内容?
在处理字符串时,如果符号之间有空格或其他字符,可以通过使用正则表达式来实现更灵活的匹配。例如,使用re.sub(r'\s*[\符号]\s*', ' ', your_string)
可以将符号和相邻的空格替换为一个空格,从而保持字符串的整洁性。
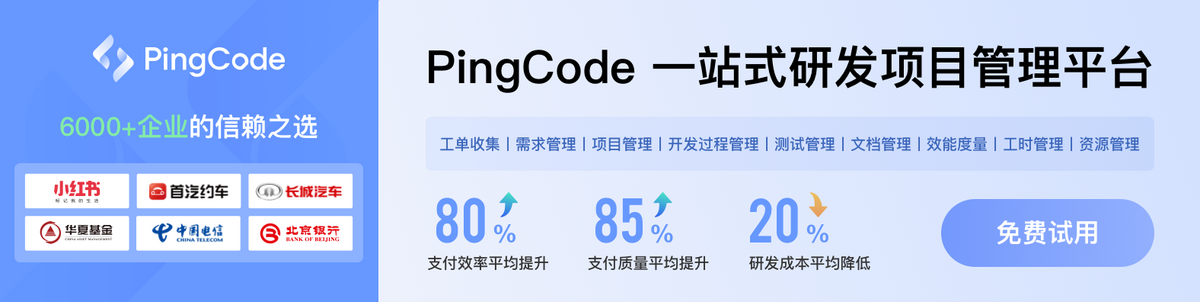