在Python中通过使用Matplotlib将横坐标改成年月,可以通过设置日期格式化器来实现。你需要导入相关的日期处理模块,并在绘图时指定日期格式。这可以通过使用matplotlib.dates
模块中的DateFormatter
和AutoDateLocator
来完成。
一、导入必要的库
在开始绘图之前,首先需要导入必要的库。除了Matplotlib,我们还需要pandas
来处理日期数据。
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import pandas as pd
from datetime import datetime
二、生成示例数据
为了演示如何将横坐标改成年月,我们需要生成一些示例数据。假设我们有一组日期和对应的值。
# 生成日期范围
dates = pd.date_range(start='2021-01-01', end='2022-01-01', freq='M')
生成对应的值
values = [i for i in range(len(dates))]
三、创建图形并绘制数据
在这一步,我们将创建图形并绘制数据,同时设置横坐标的日期格式。
fig, ax = plt.subplots()
ax.plot(dates, values)
设置日期格式
date_format = mdates.DateFormatter('%Y-%m')
ax.xaxis.set_major_formatter(date_format)
自动调整日期标签的显示
fig.autofmt_xdate()
plt.show()
通过以上步骤,你可以轻松地将横坐标改成年月格式。接下来,我们将详细介绍每个步骤,并探讨一些高级用法和技巧。
一、导入必要的库
在数据可视化的过程中,选择合适的库是非常重要的。Matplotlib是Python中最常用的绘图库之一,具有强大的功能和灵活性。为了处理日期数据,我们还需要导入pandas
和datetime
模块。
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import pandas as pd
from datetime import datetime
Matplotlib的pyplot
模块提供了绘制数据的基本功能,而dates
模块则用于处理日期格式。pandas
是一个强大的数据处理库,可以方便地生成和处理日期数据。datetime
模块提供了操作日期和时间的基本功能。
二、生成示例数据
在这一部分,我们将生成一些示例数据用于演示。假设我们有一组日期数据和对应的值数据。我们可以使用pandas
的date_range
函数生成日期范围。
# 生成日期范围
dates = pd.date_range(start='2021-01-01', end='2022-01-01', freq='M')
生成对应的值
values = [i for i in range(len(dates))]
在上面的代码中,我们生成了从2021年1月1日到2022年1月1日之间的每个月的日期,并为每个日期生成了一个对应的值。
三、创建图形并绘制数据
在生成了数据之后,我们可以使用Matplotlib创建图形并绘制数据。同时,我们需要设置横坐标的日期格式。
fig, ax = plt.subplots()
ax.plot(dates, values)
设置日期格式
date_format = mdates.DateFormatter('%Y-%m')
ax.xaxis.set_major_formatter(date_format)
自动调整日期标签的显示
fig.autofmt_xdate()
plt.show()
在上面的代码中,我们首先使用subplots
函数创建了一个图形和一个子图。然后,我们使用plot
函数绘制了日期和对应的值。接下来,我们使用DateFormatter
函数设置了日期格式为年-月
。最后,我们使用autofmt_xdate
函数自动调整日期标签的显示,以避免标签重叠。
四、处理不同时间间隔的数据
在实际应用中,你可能会遇到不同时间间隔的数据,例如每天、每周或每年。你可以根据需要调整日期格式和时间间隔。
1、每天的数据
如果你有每天的数据,可以将日期格式设置为年-月-日
。
dates = pd.date_range(start='2021-01-01', end='2021-01-31', freq='D')
values = [i for i in range(len(dates))]
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m-%d')
ax.xaxis.set_major_formatter(date_format)
fig.autofmt_xdate()
plt.show()
2、每周的数据
如果你有每周的数据,可以将日期格式设置为年-月-日
。
dates = pd.date_range(start='2021-01-01', end='2021-03-01', freq='W')
values = [i for i in range(len(dates))]
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m-%d')
ax.xaxis.set_major_formatter(date_format)
fig.autofmt_xdate()
plt.show()
3、每年的数据
如果你有每年的数据,可以将日期格式设置为年
。
dates = pd.date_range(start='2021-01-01', end='2025-01-01', freq='Y')
values = [i for i in range(len(dates))]
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y')
ax.xaxis.set_major_formatter(date_format)
fig.autofmt_xdate()
plt.show()
五、定制化日期标签
为了使图形更加美观和易读,你可以对日期标签进行定制化。下面是一些常用的定制化技巧。
1、旋转日期标签
如果日期标签过长,可以通过旋转日期标签来避免重叠。
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m')
ax.xaxis.set_major_formatter(date_format)
旋转日期标签
plt.xticks(rotation=45)
plt.show()
2、设置日期标签的字体大小
为了使日期标签更清晰,可以调整字体大小。
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m')
ax.xaxis.set_major_formatter(date_format)
设置日期标签的字体大小
plt.xticks(fontsize=12)
plt.show()
3、设置主刻度和次刻度
你可以设置主要和次要的日期刻度,以便更好地显示时间轴。
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m')
ax.xaxis.set_major_formatter(date_format)
设置主要和次要的日期刻度
ax.xaxis.set_major_locator(mdates.MonthLocator())
ax.xaxis.set_minor_locator(mdates.WeekdayLocator())
plt.show()
六、处理时间序列数据中的缺失值
在实际应用中,时间序列数据中可能会存在缺失值。处理这些缺失值是数据可视化的重要步骤。
1、插值法填补缺失值
插值法是一种常用的填补缺失值的方法。你可以使用pandas
的interpolate
函数进行插值。
import numpy as np
生成包含缺失值的数据
dates = pd.date_range(start='2021-01-01', end='2021-01-31', freq='D')
values = [i if i % 5 != 0 else np.nan for i in range(len(dates))]
使用插值法填补缺失值
values = pd.Series(values).interpolate()
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m-%d')
ax.xaxis.set_major_formatter(date_format)
fig.autofmt_xdate()
plt.show()
2、前向填补和后向填补
前向填补和后向填补是另一种常用的填补缺失值的方法。你可以使用pandas
的fillna
函数进行填补。
# 生成包含缺失值的数据
dates = pd.date_range(start='2021-01-01', end='2021-01-31', freq='D')
values = [i if i % 5 != 0 else np.nan for i in range(len(dates))]
使用前向填补和后向填补
values = pd.Series(values).fillna(method='ffill').fillna(method='bfill')
fig, ax = plt.subplots()
ax.plot(dates, values)
date_format = mdates.DateFormatter('%Y-%m-%d')
ax.xaxis.set_major_formatter(date_format)
fig.autofmt_xdate()
plt.show()
七、总结
在这篇文章中,我们详细介绍了如何在Python中使用Matplotlib将横坐标改成年月格式。通过导入必要的库、生成示例数据、创建图形并绘制数据,我们可以轻松地实现这一功能。我们还探讨了如何处理不同时间间隔的数据、定制化日期标签以及处理时间序列数据中的缺失值。
希望这篇文章能帮助你更好地理解和应用Matplotlib的日期处理功能,为你的数据可视化工作提供有力支持。
相关问答FAQs:
如何在Python中将横坐标格式化为年月?
在Python中,可以使用Matplotlib库来绘制图形并自定义横坐标。如果您希望将横坐标显示为年月格式,可以使用mdates
模块来处理日期数据。具体步骤包括将日期数据转换为datetime
对象,然后使用mdates.DateFormatter
指定格式。例如,可以使用mdates.DateFormatter('%Y-%m')
来设置显示为“年-月”格式。
在绘制图形时,如何确保横坐标的日期顺序正确?
为了确保横坐标的日期顺序正确,建议在数据准备阶段使用pandas
库将日期数据进行排序。将日期列转换为datetime
类型后,使用sort_values()
方法对数据进行排序,确保绘图时横坐标按照时间顺序排列。此外,在使用Matplotlib绘图时,调用plt.xticks()
来设置刻度和标签,以确保显示的日期格式符合您的需求。
是否可以在Python中使用其他库绘制横坐标为年月的图形?
除了Matplotlib,Python中还有其他库可以绘制图形并自定义横坐标格式。例如,Seaborn是基于Matplotlib的高级接口,允许更简单的图形绘制,同时保持横坐标的日期格式化功能。Plotly也是一个非常流行的库,支持交互式图形,您可以轻松设置横坐标为年月格式。通过这些库,您可以实现美观且功能丰富的图形展示。
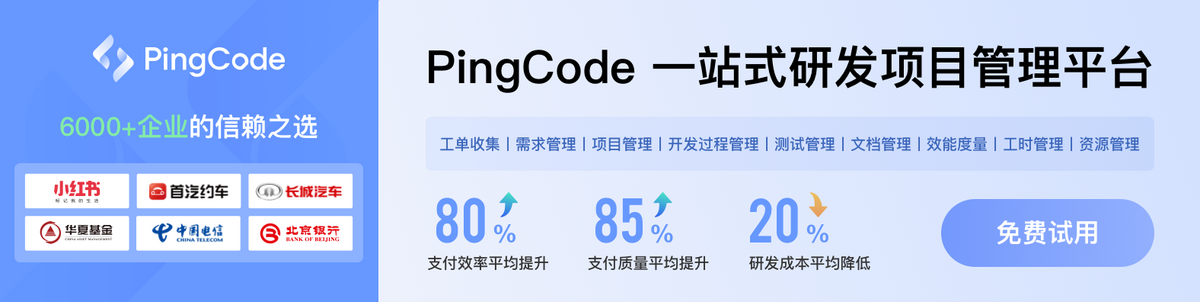