Python如何统计一个文件行数据类型
在Python中,统计一个文件的行数据类型可以通过读取文件、逐行解析、利用正则表达式识别数据类型来实现。这里的数据类型可以包括整数、浮点数、字符串等。首先,我们需要打开文件并逐行读取,然后对每一行进行解析,并统计每种数据类型的出现次数。接下来,我们将具体探讨如何实现这些步骤。
一、读取文件并逐行解析
在Python中,读取文件并逐行解析是非常简单的。我们可以使用open()
函数打开文件,使用readlines()
方法逐行读取内容。以下是一个基本的示例:
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
在这个函数中,with open(file_path, 'r') as file
确保文件在读取后会被自动关闭,而file.readlines()
则返回一个包含文件中所有行的列表。
二、使用正则表达式识别数据类型
为了识别每行的数据类型,我们可以使用Python的re
模块。以下是一些常见的数据类型及其正则表达式:
- 整数:
^\d+$
- 浮点数:
^\d+\.\d+$
- 字符串:
^[A-Za-z]+$
我们可以编写一个函数来匹配每一行的内容并确定它的类型:
import re
def determine_data_type(line):
if re.match(r'^\d+$', line):
return 'integer'
elif re.match(r'^\d+\.\d+$', line):
return 'float'
elif re.match(r'^[A-Za-z]+$', line):
return 'string'
else:
return 'unknown'
三、统计每种数据类型的出现次数
我们可以使用一个字典来存储每种数据类型的出现次数。以下是一个完整的示例程序:
def count_data_types(file_path):
lines = read_file(file_path)
data_type_counts = {'integer': 0, 'float': 0, 'string': 0, 'unknown': 0}
for line in lines:
line = line.strip()
data_type = determine_data_type(line)
data_type_counts[data_type] += 1
return data_type_counts
在这个程序中,我们首先读取文件的所有行,然后使用determine_data_type
函数确定每一行的数据类型,最后更新data_type_counts
字典。
四、处理不同的数据格式
在实际应用中,文件中的数据行可能包含不同的格式,例如带有空格、特殊字符或混合数据类型。我们需要增强我们的程序来处理这些情况。
1、忽略空行和空格
我们可以在读取每一行后使用strip()
方法去除空格,并跳过空行:
for line in lines:
line = line.strip()
if not line:
continue
data_type = determine_data_type(line)
data_type_counts[data_type] += 1
2、处理包含特殊字符的字符串
如果文件中的字符串可能包含特殊字符,我们需要修改正则表达式以包括这些字符:
def determine_data_type(line):
if re.match(r'^\d+$', line):
return 'integer'
elif re.match(r'^\d+\.\d+$', line):
return 'float'
elif re.match(r'^[A-Za-z\s\W]+$', line):
return 'string'
else:
return 'unknown'
3、处理混合数据类型
对于包含混合数据类型的行,我们可以使用更加复杂的逻辑来解析每一行,并分别统计每种类型的出现次数。例如,如果一行包含多个数据项,我们可以使用split()
方法将其拆分为多个部分,然后分别进行类型判断:
def count_data_types(file_path):
lines = read_file(file_path)
data_type_counts = {'integer': 0, 'float': 0, 'string': 0, 'unknown': 0}
for line in lines:
line = line.strip()
if not line:
continue
items = line.split()
for item in items:
data_type = determine_data_type(item)
data_type_counts[data_type] += 1
return data_type_counts
五、输出结果并进行可视化
在统计完所有数据类型后,我们可以将结果输出到控制台,或者使用Python的绘图库进行可视化。
1、输出结果到控制台
我们可以简单地打印data_type_counts
字典:
def print_data_type_counts(data_type_counts):
for data_type, count in data_type_counts.items():
print(f"{data_type}: {count}")
2、使用Matplotlib进行可视化
我们可以使用matplotlib
库将统计结果绘制成柱状图:
import matplotlib.pyplot as plt
def visualize_data_type_counts(data_type_counts):
data_types = list(data_type_counts.keys())
counts = list(data_type_counts.values())
plt.bar(data_types, counts)
plt.xlabel('Data Type')
plt.ylabel('Count')
plt.title('Data Type Counts')
plt.show()
六、完整代码示例
以下是一个完整的示例代码,结合了上述所有步骤:
import re
import matplotlib.pyplot as plt
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
def determine_data_type(line):
if re.match(r'^\d+$', line):
return 'integer'
elif re.match(r'^\d+\.\d+$', line):
return 'float'
elif re.match(r'^[A-Za-z\s\W]+$', line):
return 'string'
else:
return 'unknown'
def count_data_types(file_path):
lines = read_file(file_path)
data_type_counts = {'integer': 0, 'float': 0, 'string': 0, 'unknown': 0}
for line in lines:
line = line.strip()
if not line:
continue
items = line.split()
for item in items:
data_type = determine_data_type(item)
data_type_counts[data_type] += 1
return data_type_counts
def print_data_type_counts(data_type_counts):
for data_type, count in data_type_counts.items():
print(f"{data_type}: {count}")
def visualize_data_type_counts(data_type_counts):
data_types = list(data_type_counts.keys())
counts = list(data_type_counts.values())
plt.bar(data_types, counts)
plt.xlabel('Data Type')
plt.ylabel('Count')
plt.title('Data Type Counts')
plt.show()
if __name__ == "__main__":
file_path = 'data.txt'
data_type_counts = count_data_types(file_path)
print_data_type_counts(data_type_counts)
visualize_data_type_counts(data_type_counts)
通过以上代码,我们可以读取文件,统计每种数据类型的出现次数,并将结果输出到控制台或进行可视化。这种方法不仅高效,而且易于扩展,可以处理不同的数据格式和复杂的文件结构。
相关问答FAQs:
如何在Python中读取文件并统计行数?
在Python中,可以使用内置的open()
函数打开文件,然后通过循环读取每一行来统计行数。示例代码如下:
with open('yourfile.txt', 'r') as file:
line_count = sum(1 for line in file)
print(f'文件的行数是:{line_count}')
此代码将打开指定的文件,并逐行读取,计算总行数。
如何判断文件中每行的数据类型?
在读取文件的每一行时,可以使用try-except
结构来判断数据类型。常见的数据类型包括整数、浮点数和字符串。可以通过以下示例代码实现:
def detect_type(line):
try:
int(line)
return '整数'
except ValueError:
try:
float(line)
return '浮点数'
except ValueError:
return '字符串'
with open('yourfile.txt', 'r') as file:
for line in file:
print(f'行内容: {line.strip()},数据类型: {detect_type(line.strip())}')
这段代码将读取文件的每一行,并输出每行的内容及其对应的数据类型。
如何在统计行数据类型时排除空行或注释行?
在处理文件时,常常需要忽略空行或以特定字符开头的注释行。可以通过简单的条件判断来实现:
with open('yourfile.txt', 'r') as file:
for line in file:
stripped_line = line.strip()
if not stripped_line or stripped_line.startswith('#'):
continue
print(f'行内容: {stripped_line},数据类型: {detect_type(stripped_line)}')
在这段代码中,只有非空且不以‘#’开头的行会被处理,从而避免了对空行和注释行的统计。
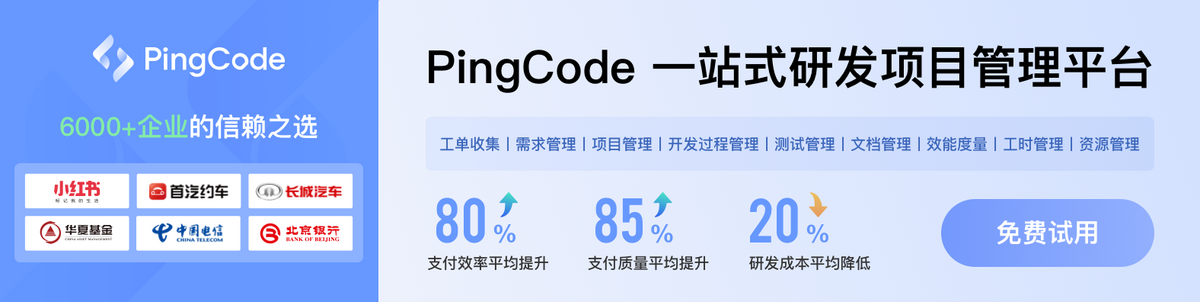