Python替换字符串部分内容的方法包括:使用replace()方法、使用re模块进行正则表达式替换、字符串切片与拼接。下面将详细介绍其中的replace()方法。
Python 的 replace()
方法是最常用的字符串替换方法之一,简单易用。它用于将字符串中的某个子字符串替换为另一个子字符串。其基本语法为:str.replace(old, new[, maxreplace])
,其中 old
是需要被替换的子字符串,new
是替换后的子字符串,maxreplace
是可选参数,表示替换次数。如果不指定 maxreplace
,则替换所有出现的子字符串。
例如,假设有一个字符串 text = "Hello World! Welcome to the World!"
,我们想将其中的 "World" 替换为 "Universe"。可以使用 replace()
方法完成:
text = "Hello World! Welcome to the World!"
new_text = text.replace("World", "Universe")
print(new_text) # 输出: "Hello Universe! Welcome to the Universe!"
接下来,我们将深入探讨其他方法,并详细解释每种方法的使用场景和实现步骤。
一、使用 replace() 方法
replace() 方法 是最常用的字符串替换方法之一,语法简单,适用于大多数字符串替换需求。
1. 基本用法
replace()
方法的基本用法如下:
str.replace(old, new[, maxreplace])
old
是需要被替换的子字符串;new
是替换后的子字符串;maxreplace
是可选参数,表示替换次数。
示例代码:
text = "Hello World! Welcome to the World!"
new_text = text.replace("World", "Universe")
print(new_text) # 输出: "Hello Universe! Welcome to the Universe!"
2. 指定替换次数
可以使用 maxreplace
参数来限制替换的次数。例如,只替换第一次出现的 "World":
示例代码:
text = "Hello World! Welcome to the World!"
new_text = text.replace("World", "Universe", 1)
print(new_text) # 输出: "Hello Universe! Welcome to the World!"
二、使用 re 模块进行正则表达式替换
正则表达式是一种强大的字符串处理工具,Python 的 re
模块提供了丰富的正则表达式操作函数。使用 re.sub()
方法可以实现复杂的字符串替换。
1. 基本用法
re.sub()
方法的基本语法为:
re.sub(pattern, repl, string, count=0, flags=0)
pattern
是正则表达式模式;repl
是替换后的字符串;string
是要处理的字符串;count
是可选参数,表示替换次数,默认为 0,表示替换所有匹配项;flags
是可选参数,用于控制正则表达式匹配行为。
示例代码:
import re
text = "Hello World! Welcome to the World!"
pattern = r"World"
new_text = re.sub(pattern, "Universe", text)
print(new_text) # 输出: "Hello Universe! Welcome to the Universe!"
2. 使用正则表达式进行复杂替换
正则表达式可以匹配复杂的模式,例如匹配以 "W" 开头的单词,并将其替换为 "Universe":
示例代码:
import re
text = "Hello World! Welcome to the Wild West!"
pattern = r"\bW\w+\b"
new_text = re.sub(pattern, "Universe", text)
print(new_text) # 输出: "Hello Universe! Welcome to the Universe Universe!"
三、字符串切片与拼接
字符串切片与拼接是一种手动替换字符串的方法,适用于需要对字符串进行复杂操作的场景。
1. 基本用法
可以使用字符串切片和拼接来替换字符串的部分内容。例如,将字符串 text
中的 "World" 替换为 "Universe":
示例代码:
text = "Hello World!"
start = text.find("World")
end = start + len("World")
new_text = text[:start] + "Universe" + text[end:]
print(new_text) # 输出: "Hello Universe!"
2. 替换指定位置的字符串
如果需要替换字符串中特定位置的子字符串,可以使用字符串切片和拼接。例如,将字符串 text
中从索引 6 开始的 5 个字符替换为 "Universe":
示例代码:
text = "Hello World!"
start = 6
length = 5
new_text = text[:start] + "Universe" + text[start+length:]
print(new_text) # 输出: "Hello Universe!"
四、使用 translate() 方法
translate() 方法 是一种高效的字符串替换方法,适用于需要替换多个字符的场景。需要配合 str.maketrans()
方法使用。
1. 基本用法
str.maketrans()
方法用于创建字符映射表,translate()
方法用于根据映射表替换字符串中的字符。
示例代码:
text = "Hello World!"
translation_table = str.maketrans("HW", "hw")
new_text = text.translate(translation_table)
print(new_text) # 输出: "hello world!"
2. 替换多个字符
可以使用 str.maketrans()
方法创建复杂的字符映射表,替换字符串中的多个字符。
示例代码:
text = "Hello World!"
translation_table = str.maketrans("HW", "hw", "!")
new_text = text.translate(translation_table)
print(new_text) # 输出: "hello world"
五、使用正则表达式的高级功能
正则表达式不仅可以进行简单的字符替换,还可以使用其高级功能进行复杂的字符串替换,例如使用捕获组和替换函数。
1. 使用捕获组进行替换
可以使用捕获组(capture groups)提取匹配的子字符串,并在替换字符串中引用它们。
示例代码:
import re
text = "The quick brown fox jumps over the lazy dog."
pattern = r"(\b\w+\b)"
new_text = re.sub(pattern, r"(\1)", text)
print(new_text) # 输出: "(The) (quick) (brown) (fox) (jumps) (over) (the) (lazy) (dog)."
2. 使用替换函数进行动态替换
可以使用替换函数(replacement function)根据匹配内容动态生成替换字符串。
示例代码:
import re
def replace(match):
word = match.group(0)
return word[::-1]
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\b\w+\b"
new_text = re.sub(pattern, replace, text)
print(new_text) # 输出: "ehT kciuq nworb xof spmuj revo eht yzal god."
六、使用字符串模板
字符串模板(string template)是一种更灵活的字符串替换方法,适用于需要在字符串中插入动态内容的场景。Python 提供了 string.Template
类来处理字符串模板。
1. 基本用法
可以使用 string.Template
类定义字符串模板,并使用 substitute()
方法进行替换。
示例代码:
from string import Template
template = Template("Hello, $name!")
new_text = template.substitute(name="Alice")
print(new_text) # 输出: "Hello, Alice!"
2. 使用字典进行批量替换
可以使用字典提供多个替换项,进行批量替换。
示例代码:
from string import Template
template = Template("Hello, $name! Welcome to $place.")
data = {"name": "Alice", "place": "Wonderland"}
new_text = template.substitute(data)
print(new_text) # 输出: "Hello, Alice! Welcome to Wonderland."
七、性能优化和注意事项
在进行字符串替换时,需要考虑性能优化和一些注意事项,以确保代码的高效性和正确性。
1. 性能优化
- 选择合适的方法:根据实际需求选择合适的字符串替换方法。例如,
replace()
方法适用于简单替换,re.sub()
方法适用于复杂替换。 - 避免不必要的替换:在替换前检查字符串是否包含需要替换的子字符串,避免不必要的替换操作。
示例代码:
text = "Hello World!"
if "World" in text:
new_text = text.replace("World", "Universe")
2. 注意事项
- 处理边界情况:在进行字符串替换时,需要处理可能出现的边界情况,例如空字符串、特殊字符等。
- 避免无限循环:在使用正则表达式进行替换时,需要注意避免可能导致无限循环的正则表达式模式。
示例代码:
import re
text = "a"*1000
pattern = r"(a*)"
new_text = re.sub(pattern, r"\1b", text)
print(new_text) # 输出: "abbbbbbbbbbbbbbbbbbbbbbbbb..."
八、常见应用场景
字符串替换在实际应用中有许多常见的场景,例如文本清理、数据格式化、模板生成等。
1. 文本清理
在文本清理过程中,可以使用字符串替换方法去除不需要的字符或格式化文本。
示例代码:
import re
text = "Hello, World! Welcome to 2023."
pattern = r"[^\w\s]"
new_text = re.sub(pattern, "", text)
print(new_text) # 输出: "Hello World Welcome to 2023"
2. 数据格式化
在数据格式化过程中,可以使用字符串替换方法将数据转换为指定格式。
示例代码:
data = "2023-10-01"
formatted_data = data.replace("-", "/")
print(formatted_data) # 输出: "2023/10/01"
3. 模板生成
在模板生成过程中,可以使用字符串模板方法动态生成内容。
示例代码:
from string import Template
template = Template("Dear $name,\n\nWelcome to $company.")
data = {"name": "Alice", "company": "TechCorp"}
new_text = template.substitute(data)
print(new_text)
结论
替换字符串的部分内容是Python编程中常见的操作,本文介绍了多种方法,包括 replace()
方法、正则表达式、字符串切片与拼接、translate()
方法、字符串模板等。每种方法都有其适用的场景和优缺点,开发者可以根据具体需求选择合适的方法进行字符串替换。通过掌握这些方法,可以有效地处理各种字符串替换需求,提高代码的灵活性和可维护性。
相关问答FAQs:
如何在Python中替换字符串中的特定字符或子字符串?
在Python中,可以使用字符串的 replace()
方法来替换特定的字符或子字符串。例如,如果你有一个字符串 text = "Hello World"
,并想将 "World" 替换为 "Python",可以使用 text.replace("World", "Python")
,这将返回 "Hello Python"
。
使用正则表达式替换字符串内容的最佳实践是什么?
如果需要更复杂的替换,比如使用模式匹配,可以使用Python的 re
模块。通过 re.sub()
函数,你可以根据正则表达式匹配的内容进行替换。例如,re.sub(r'\d+', 'number', 'There are 2 apples and 3 oranges')
将会把所有数字替换为 "number",结果为 "There are number apples and number oranges"。
如何替换字符串中的多个不同部分?
在Python中替换多个部分可以通过多次调用 replace()
方法,或者使用 re.sub()
结合一个字典来实现。例如,使用 replace()
可以这样做:text.replace("apple", "orange").replace("banana", "kiwi")
。而使用正则表达式,你可以创建一个函数来处理字典中的替换项,依次替换多个字符串。
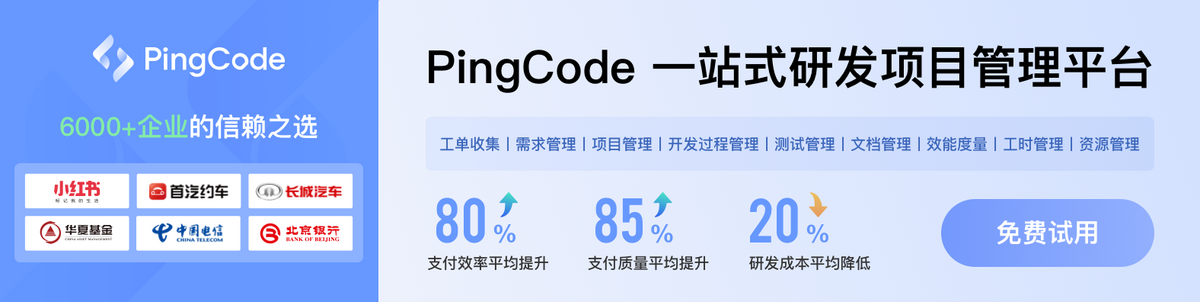