使用Python对文本的某一行进行修改,主要步骤包括:读取文件内容、修改目标行、将内容写回文件。 具体操作包括读取文件内容、定位目标行、修改该行、写回文件。以下将详细描述这些步骤及其实现细节。
一、读取文件内容
在处理文本文件时,首先需要将文件内容读取到内存中。Python提供了多种读取文件的方法,最常用的是使用open()
函数和readlines()
方法。
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
上述函数read_file
接受文件路径作为参数,并返回文件中的所有行内容,以列表形式存储。使用with open
可以确保文件被正确关闭,避免资源泄露。
二、定位目标行
定位目标行是修改文本的关键。可以根据行号或特定的条件(如某个关键词)来定位目标行。
根据行号定位
如果已知要修改的行号,可以直接使用索引访问该行内容。
def modify_line_by_number(lines, line_number, new_content):
if 0 <= line_number < len(lines):
lines[line_number] = new_content + '\n'
else:
print(f"Line number {line_number} is out of range.")
return lines
根据关键词定位
如果需要根据特定关键词来定位行,可以遍历文件内容,找到包含关键词的行。
def modify_line_by_keyword(lines, keyword, new_content):
for i, line in enumerate(lines):
if keyword in line:
lines[i] = new_content + '\n'
break
return lines
三、修改目标行
修改目标行后,我们需要将修改后的内容写回到文件中。使用writelines()
方法可以将整个内容列表写回文件。
def write_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
四、完整示例
将上述步骤整合到一个完整的示例中,展示如何读取文件、修改某一行并写回文件。
def modify_text_file(file_path, line_number=None, keyword=None, new_content=''):
# 读取文件内容
lines = read_file(file_path)
# 修改目标行
if line_number is not None:
lines = modify_line_by_number(lines, line_number, new_content)
elif keyword is not None:
lines = modify_line_by_keyword(lines, keyword, new_content)
else:
print("Either line number or keyword must be provided.")
return
# 写回文件
write_file(file_path, lines)
五、使用示例
以下示例展示了如何使用上述函数来修改文件的特定行。
file_path = 'sample.txt'
根据行号修改
modify_text_file(file_path, line_number=2, new_content="This is the new content for line 3")
根据关键词修改
modify_text_file(file_path, keyword="old_keyword", new_content="This is the new content for the line containing 'old_keyword'")
六、处理异常情况
在实际应用中,还需要处理可能出现的异常情况,例如文件不存在、文件读取失败、行号超出范围等。
import os
def modify_text_file_with_exceptions(file_path, line_number=None, keyword=None, new_content=''):
if not os.path.exists(file_path):
print(f"File {file_path} does not exist.")
return
try:
# 读取文件内容
lines = read_file(file_path)
except Exception as e:
print(f"Failed to read file: {e}")
return
# 修改目标行
if line_number is not None:
lines = modify_line_by_number(lines, line_number, new_content)
elif keyword is not None:
lines = modify_line_by_keyword(lines, keyword, new_content)
else:
print("Either line number or keyword must be provided.")
return
try:
# 写回文件
write_file(file_path, lines)
except Exception as e:
print(f"Failed to write file: {e}")
通过上述步骤,可以全面掌握使用Python对文本文件某一行进行修改的技巧。这些方法不仅适用于简单文本文件的处理,还可以扩展应用到更复杂的数据处理任务中。
七、扩展应用
批量修改
在某些情况下,我们可能需要批量修改文件中的多行内容。例如,替换所有出现特定关键词的行。
def modify_lines_by_keyword(lines, keyword, new_content):
for i, line in enumerate(lines):
if keyword in line:
lines[i] = new_content + '\n'
return lines
正则表达式
使用正则表达式可以实现更复杂的行定位和内容替换。例如,替换所有包含特定模式的行。
import re
def modify_lines_by_regex(lines, pattern, new_content):
for i, line in enumerate(lines):
if re.search(pattern, line):
lines[i] = new_content + '\n'
return lines
多文件处理
如果需要对多个文件进行相同的修改,可以遍历文件目录,批量处理文件。
import glob
def modify_multiple_files(file_pattern, line_number=None, keyword=None, new_content=''):
for file_path in glob.glob(file_pattern):
modify_text_file_with_exceptions(file_path, line_number, keyword, new_content)
八、总结
使用Python对文本某一行进行修改,涉及文件读取、行定位、行修改和文件写回等步骤。通过灵活运用行号、关键词和正则表达式等方法,可以实现多种复杂的文本处理任务。此外,处理异常情况和扩展应用场景(如批量修改和多文件处理)也是提高代码鲁棒性的重要方面。希望通过本文的详细介绍,读者能够掌握并应用这些技巧,解决实际工作中的文本处理需求。
相关问答FAQs:
如何在Python中定位并修改文本文件的特定行?
在Python中,可以使用文件读取和写入的操作来定位并修改文本文件中的特定行。首先读取文件的所有行,然后根据行号进行修改,最后将修改后的内容写回文件。例如,可以使用列表来存储文件的每一行,通过索引来访问并修改特定行,最后将整个列表写入文件。
如果我只想替换文本文件中特定行的部分内容,该怎么做?
如果只需要替换特定行中的部分内容,可以先读取文件中的所有行,找到目标行并在该行中进行字符串的替换操作。完成替换后,将修改后的行写回文件。在Python中,可以使用字符串的replace()
方法来实现这一点。
在Python中修改文本文件时,如何确保数据安全?
在修改文本文件时,为了确保数据安全,建议在进行写操作之前先创建文件的备份。此外,可以使用with
语句来打开文件,这样可以确保文件在操作完成后正确关闭。还可以在修改文件之前先读取并验证内容,确保所做的修改是正确的。
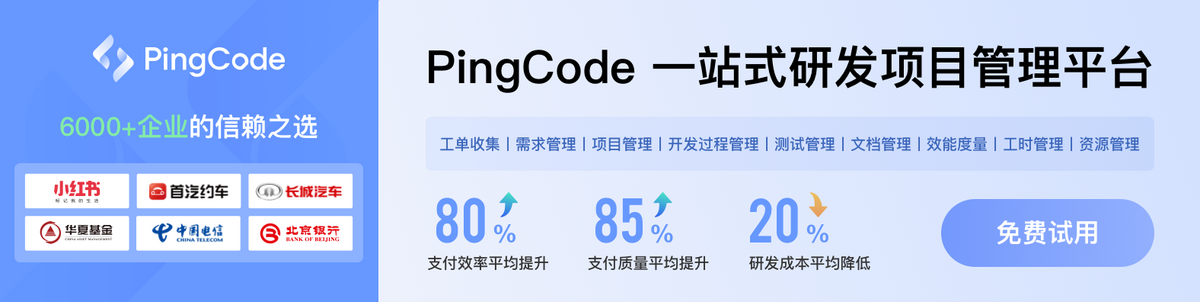