Python比较两个字符是否相同的方法有:使用“==运算符”、使用“ord()函数”、使用“str.casefold()方法”等。其中,使用“==运算符”是最常用且最直接的方法。
在Python中,比较两个字符是否相同最常用的方法是使用“==运算符”。这是一种直接且高效的方法,因为Python中的字符串和字符都是对象,==运算符可以直接比较它们的值。下面将详细介绍使用“==运算符”进行字符比较的方法。
一、使用“==运算符”比较字符
使用“==运算符”是最简单、最直观的方法。它直接比较两个字符的值,判断它们是否相同。如果相同,返回True;否则,返回False。
char1 = 'a'
char2 = 'a'
if char1 == char2:
print("The characters are the same.")
else:
print("The characters are different.")
在上述示例中,变量char1
和char2
都包含字符‘a’,所以char1 == char2
的结果为True,程序将输出“The characters are the same.”。
二、使用“ord()函数”比较字符
ord()
函数用于返回字符对应的ASCII码值。通过比较两个字符的ASCII码值,也可以判断它们是否相同。
char1 = 'a'
char2 = 'b'
if ord(char1) == ord(char2):
print("The characters are the same.")
else:
print("The characters are different.")
在这个示例中,ord(char1)
返回字符‘a’的ASCII码值97,ord(char2)
返回字符‘b’的ASCII码值98。由于97不等于98,程序将输出“The characters are different.”。
三、使用“str.casefold()方法”进行不区分大小写的比较
在某些情况下,可能需要进行不区分大小写的字符比较。这时可以使用str.casefold()
方法,它将字符串全部转换为小写,从而实现不区分大小写的比较。
char1 = 'A'
char2 = 'a'
if char1.casefold() == char2.casefold():
print("The characters are the same (case-insensitive).")
else:
print("The characters are different.")
在上面的示例中,char1.casefold()
和char2.casefold()
都转换为小写‘a’,所以char1.casefold() == char2.casefold()
的结果为True,程序将输出“The characters are the same (case-insensitive).”。
四、使用正则表达式进行字符比较
有时需要更复杂的字符比较,例如比较字符是否符合某种模式。这时可以使用正则表达式模块re
。
import re
char1 = 'a'
pattern = r'a'
if re.fullmatch(pattern, char1):
print("The character matches the pattern.")
else:
print("The character does not match the pattern.")
在这个示例中,变量char1
包含字符‘a’,模式pattern
也是‘a’,所以re.fullmatch(pattern, char1)
的结果为True,程序将输出“The character matches the pattern.”。
五、处理Unicode字符的比较
在处理国际化应用时,经常需要比较Unicode字符。Python对Unicode字符的支持非常好,使用“==运算符”同样适用。
char1 = 'ü'
char2 = 'ü'
if char1 == char2:
print("The Unicode characters are the same.")
else:
print("The Unicode characters are different.")
在这个示例中,两个Unicode字符都是‘ü’,所以char1 == char2
的结果为True,程序将输出“The Unicode characters are the same.”。
六、总结
在Python中比较两个字符是否相同的方法有很多,但最常用的还是使用“==运算符”。其他方法如ord()
函数、str.casefold()
方法和正则表达式等也有其独特的应用场景。
- 使用“==运算符”比较字符:最简单、最常用的方法。
- 使用“ord()函数”比较字符:通过比较字符的ASCII码值判断。
- 使用“str.casefold()方法”进行不区分大小写的比较:适用于需要忽略大小写的场景。
- 使用正则表达式进行字符比较:适用于复杂模式匹配。
- 处理Unicode字符的比较:Python对Unicode支持很好,使用“==运算符”同样适用。
通过灵活运用这些方法,可以满足各种不同需求的字符比较操作。
相关问答FAQs:
如何在Python中判断两个字符是否相等?
在Python中,判断两个字符是否相同可以通过简单的比较操作符==
来实现。例如,您可以使用以下代码:
char1 = 'a'
char2 = 'a'
is_equal = char1 == char2
print(is_equal) # 输出: True
这种方法适用于比较任何两个字符或字符串的相等性。
如果字符的大小写不同,如何比较?
在比较字符时,大小写差异可能导致不相等。如果希望忽略大小写,可以使用lower()
或upper()
方法将字符转换为统一的大小写。例如:
char1 = 'A'
char2 = 'a'
is_equal = char1.lower() == char2.lower()
print(is_equal) # 输出: True
这种方式确保比较时不受字符大小写的影响。
如何处理特殊字符或空格的比较?
在比较字符时,特殊字符或空格可能会影响结果。为了确保字符比较的准确性,可以使用strip()
方法去除空格,并使用replace()
方法处理特殊字符。例如:
char1 = ' Hello '
char2 = 'Hello'
is_equal = char1.strip() == char2
print(is_equal) # 输出: True
这种方法可以确保在比较时忽略多余的空格。
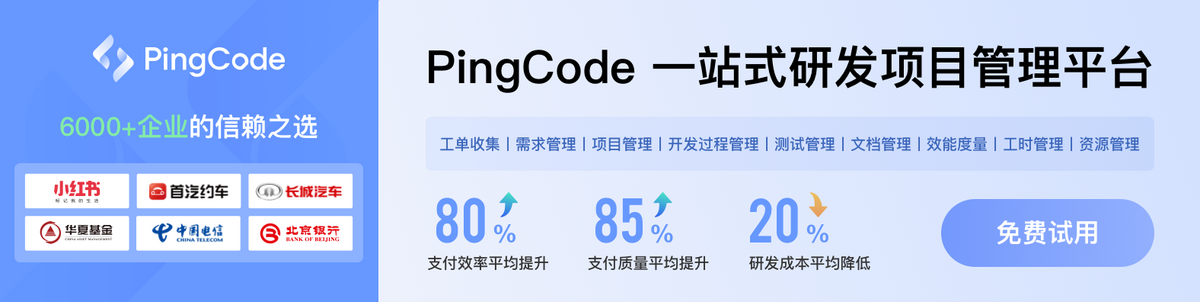