Python读取一个文件夹中全部工作簿的方法包括:使用os模块获取文件路径、使用pandas模块读取Excel工作簿、遍历文件夹中的所有文件、筛选出Excel文件并读取内容。使用os模块、遍历文件夹、筛选Excel文件、使用pandas读取内容。下面将详细描述如何实现这一过程。
一、使用os模块获取文件路径
os模块是Python标准库中的一个模块,主要用于与操作系统进行交互。 通过os模块,可以获取文件夹中的所有文件路径,为后续读取Excel文件做准备。
os模块的常用方法包括:
- os.listdir():返回指定文件夹中的文件和文件夹列表
- os.path.join():将多个路径组合后返回
- os.path.isfile():判断给定路径是否为文件
- os.path.splitext():分离文件名与扩展名
以下是使用os模块获取文件夹中所有文件路径的示例代码:
import os
def get_all_files(folder_path):
all_files = []
for root, dirs, files in os.walk(folder_path):
for file in files:
file_path = os.path.join(root, file)
all_files.append(file_path)
return all_files
folder_path = 'your_folder_path'
all_files = get_all_files(folder_path)
print(all_files)
二、遍历文件夹中的所有文件
在获取了文件夹中所有文件的路径后,我们需要遍历这些文件,筛选出Excel文件。Excel文件通常有两个扩展名:.xls 和 .xlsx。
三、筛选Excel文件
筛选文件的过程可以使用os.path.splitext()方法,该方法将文件名和扩展名分开。通过检查扩展名,可以筛选出Excel文件。
以下是筛选Excel文件的示例代码:
def filter_excel_files(all_files):
excel_files = []
for file in all_files:
if os.path.isfile(file):
ext = os.path.splitext(file)[1]
if ext in ['.xls', '.xlsx']:
excel_files.append(file)
return excel_files
excel_files = filter_excel_files(all_files)
print(excel_files)
四、使用pandas读取Excel文件
pandas是Python中一个强大的数据处理和分析库,能够方便地读取和操作Excel文件。 通过pandas.read_excel()方法,可以读取Excel文件中的数据。
以下是使用pandas读取Excel文件的示例代码:
import pandas as pd
def read_excel_files(excel_files):
data_frames = []
for file in excel_files:
df = pd.read_excel(file)
data_frames.append(df)
return data_frames
data_frames = read_excel_files(excel_files)
for df in data_frames:
print(df.head())
五、综合示例
将以上步骤整合到一个完整的示例中:
import os
import pandas as pd
def get_all_files(folder_path):
all_files = []
for root, dirs, files in os.walk(folder_path):
for file in files:
file_path = os.path.join(root, file)
all_files.append(file_path)
return all_files
def filter_excel_files(all_files):
excel_files = []
for file in all_files:
if os.path.isfile(file):
ext = os.path.splitext(file)[1]
if ext in ['.xls', '.xlsx']:
excel_files.append(file)
return excel_files
def read_excel_files(excel_files):
data_frames = []
for file in excel_files:
df = pd.read_excel(file)
data_frames.append(df)
return data_frames
folder_path = 'your_folder_path'
all_files = get_all_files(folder_path)
excel_files = filter_excel_files(all_files)
data_frames = read_excel_files(excel_files)
for df in data_frames:
print(df.head())
六、处理读取的Excel数据
在读取Excel文件后,可以对数据进行处理和分析。以下是一些常见的数据处理操作:
1、数据清洗
数据清洗是数据处理过程中重要的一步,常见的操作包括处理缺失值、重复值和异常值。
处理缺失值的示例代码:
for df in data_frames:
df.dropna(inplace=True)
print(df.head())
2、数据转换
数据转换是将数据从一种形式转换为另一种形式的过程,常见的操作包括数据类型转换和数据格式转换。
数据类型转换的示例代码:
for df in data_frames:
df['column_name'] = df['column_name'].astype(float)
print(df.head())
3、数据合并
数据合并是将多个数据集合并为一个数据集的过程,常见的操作包括纵向合并和横向合并。
纵向合并的示例代码:
merged_df = pd.concat(data_frames, ignore_index=True)
print(merged_df.head())
4、数据分析
数据分析是从数据中提取有用信息的过程,常见的操作包括描述性统计分析和数据可视化。
描述性统计分析的示例代码:
for df in data_frames:
print(df.describe())
数据可视化的示例代码:
import matplotlib.pyplot as plt
for df in data_frames:
df['column_name'].hist()
plt.show()
七、总结
通过以上步骤,我们可以使用Python读取一个文件夹中全部工作簿,并对数据进行处理和分析。os模块用于获取文件路径、遍历文件夹、筛选Excel文件、pandas模块用于读取和处理Excel数据。这些方法和技巧可以帮助我们高效地处理大量Excel文件,提高工作效率。
相关问答FAQs:
如何使用Python读取特定文件夹中的所有Excel工作簿?
要读取一个文件夹中的所有Excel工作簿,可以使用pandas
库结合os
模块。首先,使用os.listdir()
获取文件夹内所有文件的列表,然后筛选出以.xlsx
或.xls
结尾的文件名。接着,利用pandas.read_excel()
逐个读取工作簿。以下是一个示例代码:
import os
import pandas as pd
folder_path = '你的文件夹路径'
workbooks = [f for f in os.listdir(folder_path) if f.endswith(('.xlsx', '.xls'))]
data_frames = [pd.read_excel(os.path.join(folder_path, wb)) for wb in workbooks]
在读取Excel文件时,如何处理不同工作表的数据?
如果一个Excel文件包含多个工作表,pandas
的read_excel()
函数允许你指定要读取的工作表名称或索引。可以通过sheet_name
参数来实现这一点。如果需要读取所有工作表,可以将sheet_name
设置为None
,这样会返回一个字典,其中键是工作表名称,值是对应的数据框。
data = pd.read_excel('文件路径.xlsx', sheet_name=None)
是否可以并行读取多个工作簿以提高效率?
是的,可以使用concurrent.futures
库实现并行读取多个工作簿。通过创建一个线程池,可以同时读取多个Excel文件,显著减少处理时间。以下是一个示例:
import os
import pandas as pd
from concurrent.futures import ThreadPoolExecutor
def read_workbook(file_path):
return pd.read_excel(file_path)
folder_path = '你的文件夹路径'
workbooks = [os.path.join(folder_path, f) for f in os.listdir(folder_path) if f.endswith(('.xlsx', '.xls'))]
with ThreadPoolExecutor() as executor:
data_frames = list(executor.map(read_workbook, workbooks))
这些方法可以帮助您有效地读取和处理文件夹中的所有工作簿数据。
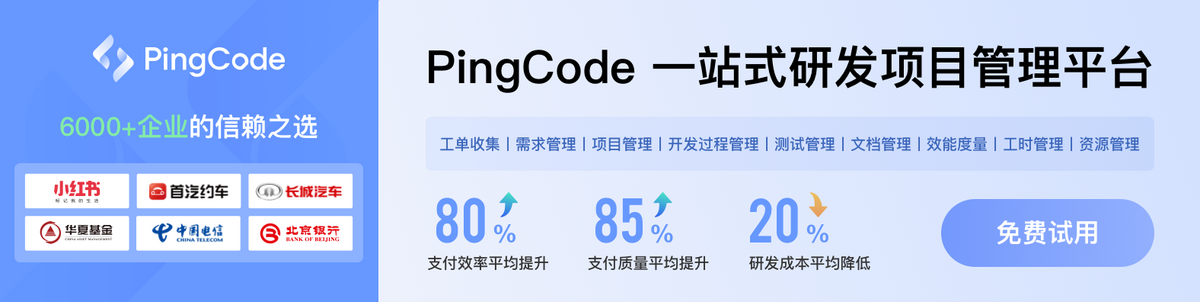