在Python中合并两个HDF5文件的方法包括:使用h5py库、逐个数据集复制、利用并行处理。我们将详细讨论其中的使用h5py库这一方法。
使用h5py库是一种常见且高效的方法。首先,h5py库是一个用于处理HDF5文件的Python库,它提供了对HDF5文件的读写操作。合并HDF5文件的核心步骤包括:打开源文件和目标文件、遍历源文件中的数据集并将其复制到目标文件中。接下来,我们将详细解释这一方法,并提供具体的代码示例。
一、使用h5py库
h5py库是一个用于处理HDF5文件的Python库,能够方便地进行文件的读写操作。首先,确保你已经安装了h5py库。如果没有安装,可以通过以下命令进行安装:
pip install h5py
然后,我们可以开始编写代码来合并两个HDF5文件。以下是一个具体的示例代码:
import h5py
def copy_dataset(source_file, target_file, dataset_name):
with h5py.File(source_file, 'r') as src:
with h5py.File(target_file, 'a') as tgt:
src.copy(dataset_name, tgt)
def merge_hdf5_files(source_files, target_file):
for source_file in source_files:
with h5py.File(source_file, 'r') as src:
for dataset_name in src.keys():
copy_dataset(source_file, target_file, dataset_name)
source_files = ['source1.h5', 'source2.h5']
target_file = 'merged.h5'
merge_hdf5_files(source_files, target_file)
二、逐个数据集复制
在合并HDF5文件时,我们需要确保目标文件中没有重复的数据集名称。因此,在复制数据集时,可以对数据集名称进行检查和处理,以防止名称冲突。
import h5py
def copy_dataset(source_file, target_file, dataset_name):
with h5py.File(source_file, 'r') as src:
with h5py.File(target_file, 'a') as tgt:
if dataset_name in tgt:
new_name = dataset_name + '_copy'
while new_name in tgt:
new_name += '_copy'
src.copy(dataset_name, tgt, new_name)
else:
src.copy(dataset_name, tgt)
def merge_hdf5_files(source_files, target_file):
for source_file in source_files:
with h5py.File(source_file, 'r') as src:
for dataset_name in src.keys():
copy_dataset(source_file, target_file, dataset_name)
source_files = ['source1.h5', 'source2.h5']
target_file = 'merged.h5'
merge_hdf5_files(source_files, target_file)
三、利用并行处理
对于大型HDF5文件,逐个数据集复制可能会花费较长时间。此时,可以考虑利用并行处理来加速文件合并。Python的multiprocessing库提供了简单易用的并行处理功能。
import h5py
from multiprocessing import Pool
def copy_dataset(args):
source_file, target_file, dataset_name = args
with h5py.File(source_file, 'r') as src:
with h5py.File(target_file, 'a') as tgt:
if dataset_name in tgt:
new_name = dataset_name + '_copy'
while new_name in tgt:
new_name += '_copy'
src.copy(dataset_name, tgt, new_name)
else:
src.copy(dataset_name, tgt)
def merge_hdf5_files(source_files, target_file):
with Pool() as pool:
tasks = []
for source_file in source_files:
with h5py.File(source_file, 'r') as src:
for dataset_name in src.keys():
tasks.append((source_file, target_file, dataset_name))
pool.map(copy_dataset, tasks)
source_files = ['source1.h5', 'source2.h5']
target_file = 'merged.h5'
merge_hdf5_files(source_files, target_file)
四、错误处理和日志记录
在实际应用中,文件操作可能会遇到各种问题,例如文件不存在、读取错误等。为了提高代码的鲁棒性,我们可以添加错误处理和日志记录功能。
import h5py
import logging
from multiprocessing import Pool
logging.basicConfig(level=logging.INFO)
def copy_dataset(args):
source_file, target_file, dataset_name = args
try:
with h5py.File(source_file, 'r') as src:
with h5py.File(target_file, 'a') as tgt:
if dataset_name in tgt:
new_name = dataset_name + '_copy'
while new_name in tgt:
new_name += '_copy'
src.copy(dataset_name, tgt, new_name)
else:
src.copy(dataset_name, tgt)
logging.info(f'Successfully copied {dataset_name} from {source_file} to {target_file}')
except Exception as e:
logging.error(f'Error copying {dataset_name} from {source_file} to {target_file}: {e}')
def merge_hdf5_files(source_files, target_file):
with Pool() as pool:
tasks = []
for source_file in source_files:
with h5py.File(source_file, 'r') as src:
for dataset_name in src.keys():
tasks.append((source_file, target_file, dataset_name))
pool.map(copy_dataset, tasks)
source_files = ['source1.h5', 'source2.h5']
target_file = 'merged.h5'
merge_hdf5_files(source_files, target_file)
五、总结
合并HDF5文件在数据处理和分析中是一个常见的需求。使用Python的h5py库,我们可以方便地进行HDF5文件的读写和合并。通过逐个数据集复制的方法,我们可以确保数据集名称不冲突,并且可以利用并行处理来加速文件合并过程。最后,通过添加错误处理和日志记录功能,可以提高代码的鲁棒性和可维护性。
希望本篇文章能帮助你更好地理解和实现Python中合并HDF5文件的方法。如果你有任何问题或建议,欢迎留言讨论。
相关问答FAQs:
如何在Python中读取HDF5文件的内容?
在Python中,可以使用h5py
库来读取HDF5文件的内容。首先,您需要安装h5py
库,使用pip install h5py
命令进行安装。然后,您可以使用以下代码来打开文件并读取数据:
import h5py
with h5py.File('filename.h5', 'r') as file:
# 打印文件中的所有数据集和组
print(list(file.keys()))
# 读取特定数据集
data = file['dataset_name'][:]
这种方式可以帮助您理解HDF5文件中的结构和数据。
合并HDF5文件时需要注意哪些事项?
在合并HDF5文件时,确保两个文件中的数据集结构相似是至关重要的。如果数据集的维度或类型不匹配,可能会导致合并失败。此外,您还需要考虑合并后的数据集命名,以避免命名冲突。为此,可以使用h5py
库中的方法来检查数据集的属性和形状。
如何在合并后验证数据完整性?
合并HDF5文件后,验证数据完整性非常重要。可以通过读取合并后的数据集并与原始数据集进行比较来实现。这可以通过以下步骤完成:
- 读取每个原始文件的相应数据集。
- 读取合并后的数据集。
- 比较数据的形状和内容,确保它们相同。
例如,可以使用NumPy库的np.array_equal()
函数来比较两个数组是否相等。这样可以确保合并操作没有丢失或更改数据。
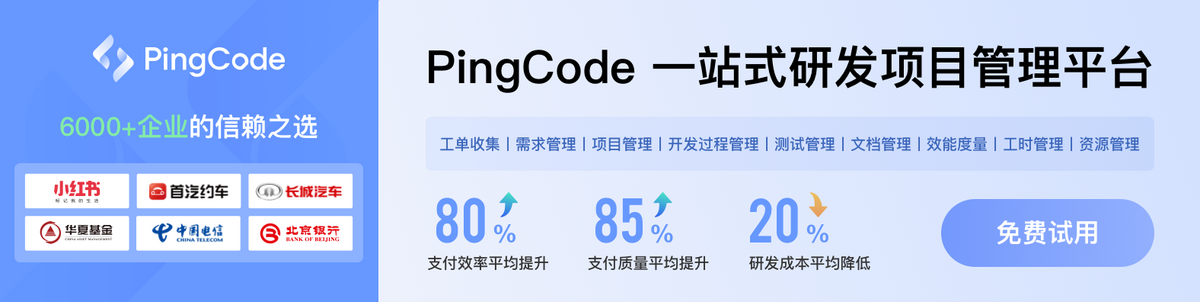