如何用Python写一个中国象棋
使用Python写一个中国象棋程序,关键在于:理解棋盘和棋子的表示、编写棋子移动规则、实现游戏逻辑、设计用户界面。以下将详细描述如何一步步实现这些关键点。
一、理解棋盘和棋子的表示
在实现中国象棋程序之前,首先需要明确棋盘和棋子的表示方式。棋盘由10行9列组成,每个棋子都有不同的种类、阵营和位置属性。可以使用二维数组或列表来表示棋盘,用字典或对象来表示棋子。
棋盘表示
可以使用一个二维列表来表示棋盘,每个元素代表棋盘上的一个位置。初始时,列表中的元素可以为空,表示没有棋子。棋子可以用一个字典来表示,包括种类、阵营和位置等信息。
# 10行9列的棋盘,初始为空
board = [[None for _ in range(9)] for _ in range(10)]
棋子字典,包含种类、阵营和位置
pieces = {
'R_King': {'type': 'King', 'side': 'Red', 'position': (0, 4)},
'B_King': {'type': 'King', 'side': 'Black', 'position': (9, 4)},
# 其他棋子类似表示
}
棋子表示
棋子可以用一个类来表示,包含种类、阵营、位置和移动规则等属性和方法。通过定义不同棋子的类,可以方便地管理和操作棋子。
class Piece:
def __init__(self, type, side, position):
self.type = type
self.side = side
self.position = position
def move(self, new_position):
# 检查移动是否合法
if self.is_valid_move(new_position):
self.position = new_position
return True
return False
def is_valid_move(self, new_position):
# 不同棋子有不同的移动规则
raise NotImplementedError("This method should be implemented by subclasses")
二、编写棋子移动规则
中国象棋的每个棋子都有不同的移动规则,需要为每个棋子编写相应的移动规则。可以通过继承Piece
类来实现不同棋子的移动规则。
例子:将(帅)的移动规则
将(帅)只能在九宫格内移动,每次只能移动一格。
class King(Piece):
def is_valid_move(self, new_position):
x, y = self.position
new_x, new_y = new_position
# 将帅只能在九宫格内
if self.side == 'Red':
if not (0 <= new_x <= 2 and 3 <= new_y <= 5):
return False
else:
if not (7 <= new_x <= 9 and 3 <= new_y <= 5):
return False
# 只能移动一格
if abs(new_x - x) + abs(new_y - y) != 1:
return False
return True
例子:车的移动规则
车可以在直线上任意移动,但不能越过其他棋子。
class Rook(Piece):
def is_valid_move(self, new_position, board):
x, y = self.position
new_x, new_y = new_position
# 车只能直线移动
if x != new_x and y != new_y:
return False
# 检查路径上是否有其他棋子
if x == new_x:
step = 1 if new_y > y else -1
for i in range(y + step, new_y, step):
if board[x][i] is not None:
return False
else:
step = 1 if new_x > x else -1
for i in range(x + step, new_x, step):
if board[i][y] is not None:
return False
return True
三、实现游戏逻辑
实现游戏逻辑需要包括游戏的初始化、棋子的选择和移动、胜负判断等。
初始化游戏
初始化棋盘和棋子,将棋子放置在初始位置。
def initialize_game():
board = [[None for _ in range(9)] for _ in range(10)]
pieces = {
'R_King': King('King', 'Red', (0, 4)),
'B_King': King('King', 'Black', (9, 4)),
# 其他棋子初始化
}
for piece in pieces.values():
x, y = piece.position
board[x][y] = piece
return board, pieces
棋子的选择和移动
实现棋子的选择和移动功能,并检查移动是否合法。
def select_piece(pieces, position):
for piece in pieces.values():
if piece.position == position:
return piece
return None
def move_piece(board, pieces, piece, new_position):
if piece.move(new_position):
x, y = piece.position
new_x, new_y = new_position
# 更新棋盘
board[x][y] = None
board[new_x][new_y] = piece
return True
return False
胜负判断
胜负判断可以通过检查是否有一方的将(帅)被吃掉来实现。
def check_winner(pieces):
red_king_alive = any(piece for piece in pieces.values() if piece.type == 'King' and piece.side == 'Red')
black_king_alive = any(piece for piece in pieces.values() if piece.type == 'King' and piece.side == 'Black')
if not red_king_alive:
return 'Black'
if not black_king_alive:
return 'Red'
return None
四、设计用户界面
用户界面是与用户交互的桥梁,可以使用Python的图形界面库如Tkinter、PyQt等来设计一个简易的用户界面。
使用Tkinter设计用户界面
下面是一个简易的Tkinter用户界面示例,包含棋盘绘制和棋子移动功能。
import tkinter as tk
from tkinter import messagebox
class ChessGUI(tk.Tk):
def __init__(self, board, pieces):
super().__init__()
self.board = board
self.pieces = pieces
self.selected_piece = None
self.canvas = tk.Canvas(self, width=450, height=500)
self.canvas.pack()
self.draw_board()
self.draw_pieces()
self.canvas.bind("<Button-1>", self.on_click)
def draw_board(self):
for i in range(10):
for j in range(9):
x1, y1 = j * 50, i * 50
x2, y2 = x1 + 50, y1 + 50
self.canvas.create_rectangle(x1, y1, x2, y2, outline='black')
def draw_pieces(self):
for piece in self.pieces.values():
x, y = piece.position
x1, y1 = y * 50 + 25, x * 50 + 25
color = 'red' if piece.side == 'Red' else 'black'
self.canvas.create_oval(x1 - 20, y1 - 20, x1 + 20, y1 + 20, fill=color)
self.canvas.create_text(x1, y1, text=piece.type[0], fill='white')
def on_click(self, event):
x, y = event.y // 50, event.x // 50
if self.selected_piece:
if move_piece(self.board, self.pieces, self.selected_piece, (x, y)):
self.draw_board()
self.draw_pieces()
winner = check_winner(self.pieces)
if winner:
messagebox.showinfo("Game Over", f"{winner} wins!")
self.destroy()
self.selected_piece = None
else:
self.selected_piece = select_piece(self.pieces, (x, y))
if __name__ == "__main__":
board, pieces = initialize_game()
app = ChessGUI(board, pieces)
app.mainloop()
总结
通过以上步骤,我们实现了一个简易的中国象棋程序,包含棋盘和棋子的表示、棋子移动规则、游戏逻辑和用户界面。这个程序可以进一步扩展和优化,例如增加更多的棋子、改进移动规则、添加更多的用户界面功能等。希望这篇文章对你实现中国象棋程序有所帮助。
相关问答FAQs:
如何开始用Python编写中国象棋游戏?
要开始编写中国象棋游戏,首先需要了解游戏的基本规则和棋盘布局。可以利用Python的pygame库来创建游戏界面,处理用户输入和棋子移动逻辑。建议先设计一个简单的文本版本,确保游戏逻辑正常后,再逐步添加图形界面。
在Python中实现中国象棋的棋子移动规则时需要注意哪些事项?
实现棋子移动规则时,必须清楚每种棋子的移动方式和限制。例如,车可以横竖移动,马走“日”字等。同时,要考虑到棋子的吃子规则和将军、捉将等特殊情况,以确保游戏的公平性和挑战性。
如何优化用Python编写的中国象棋游戏的性能?
可以通过优化数据结构来提高游戏性能,例如使用字典或集合来存储棋子的位置,减少查找时间。此外,考虑实现棋局的缓存机制,以便在回退或重做操作时提高效率。同时,优化绘图性能,避免不必要的重绘操作,确保游戏流畅运行。
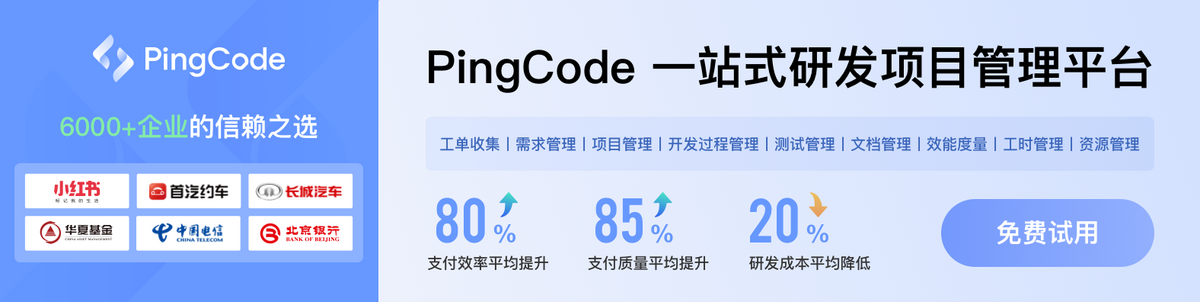