Python画八分之一圆的方法有很多种,其中常见的方法包括使用Matplotlib、Pygame、Turtle等图形库。Matplotlib是一个强大的绘图库,特别适合数据可视化;Pygame更适合游戏开发和交互式图形界面;Turtle则是一个简单的绘图工具,适合教学和初学者使用。下面将详细介绍如何使用这些库来绘制八分之一圆。
一、使用Matplotlib绘制八分之一圆
Matplotlib是Python中最常用的绘图库之一,它提供了丰富的绘图功能,能够满足大多数绘图需求。以下是使用Matplotlib绘制八分之一圆的详细步骤。
1. 安装Matplotlib
在开始绘制之前,需要确保已经安装了Matplotlib库。如果没有安装,可以通过以下命令进行安装:
pip install matplotlib
2. 导入必要的库
在绘制之前,需要导入必要的库:
import matplotlib.pyplot as plt
import numpy as np
3. 生成数据
使用numpy
生成八分之一圆的数据点:
theta = np.linspace(0, np.pi/4, 100) # 生成0到π/4之间的100个点
r = 1 # 半径为1
x = r * np.cos(theta)
y = r * np.sin(theta)
4. 绘制八分之一圆
使用matplotlib.pyplot
进行绘制:
plt.plot(x, y)
plt.title('One-Eighth Circle')
plt.xlabel('x')
plt.ylabel('y')
plt.axis('equal')
plt.grid(True)
plt.show()
二、使用Pygame绘制八分之一圆
Pygame是一个跨平台的Python模块,专为电子游戏设计,但也能很好地用于图形绘制。以下是使用Pygame绘制八分之一圆的详细步骤。
1. 安装Pygame
首先,确保已经安装了Pygame库。如果没有安装,可以通过以下命令进行安装:
pip install pygame
2. 导入必要的库
在绘制之前,需要导入必要的库:
import pygame
import math
3. 初始化Pygame
在绘制之前需要初始化Pygame:
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption('One-Eighth Circle')
4. 绘制八分之一圆
使用Pygame的绘图功能进行绘制:
def draw_eighth_circle(screen, center, radius, color):
for angle in range(0, 46): # 0到45度
x = center[0] + int(radius * math.cos(math.radians(angle)))
y = center[1] - int(radius * math.sin(math.radians(angle)))
screen.set_at((x, y), color)
center = (200, 200)
radius = 100
color = (255, 0, 0) # 红色
screen.fill((255, 255, 255)) # 白色背景
draw_eighth_circle(screen, center, radius, color)
pygame.display.flip()
5. 事件循环
确保Pygame窗口保持打开:
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
三、使用Turtle绘制八分之一圆
Turtle是一个简单的绘图工具,非常适合教学和初学者使用。以下是使用Turtle绘制八分之一圆的详细步骤。
1. 导入必要的库
Turtle库是Python标准库的一部分,无需安装,直接导入即可:
import turtle
2. 初始化Turtle
在绘制之前需要初始化Turtle:
window = turtle.Screen()
window.setup(width=400, height=400)
t = turtle.Turtle()
3. 绘制八分之一圆
使用Turtle的绘图功能进行绘制:
t.penup()
t.goto(0, 0)
t.pendown()
t.setheading(0) # 设置初始方向为0度
t.circle(100, 45) # 半径为100,绘制45度的圆弧
4. 保持窗口打开
确保Turtle窗口保持打开:
turtle.done()
通过上述三种方法中的任意一种,您都可以成功绘制一个八分之一圆。每种方法都有其独特的优点和适用场景,您可以根据实际需求选择合适的方法进行绘制。
相关问答FAQs:
如何使用Python绘制八分之一圆的代码示例?
使用Python可以借助Matplotlib库来绘制八分之一圆。以下是一个简单的代码示例:
import matplotlib.pyplot as plt
import numpy as np
theta = np.linspace(0, np.pi/4, 100) # 生成0到π/4之间的100个点
x = np.cos(theta) # 计算x坐标
y = np.sin(theta) # 计算y坐标
plt.plot(x, y) # 绘制八分之一圆
plt.fill(x, y, alpha=0.5) # 填充颜色
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.gca().set_aspect('equal') # 设置坐标轴比例
plt.title('八分之一圆的图形')
plt.show()
这段代码会生成一个八分之一圆的图形,你可以根据需要调整参数。
在绘制八分之一圆时,我需要考虑哪些参数?
在绘制八分之一圆时,主要考虑的参数包括圆的半径、起始角度和结束角度。半径决定了圆的大小,起始角度和结束角度则定义了八分之一圆的范围。在Matplotlib中,可以通过np.linspace
函数来生成圆弧的角度点,然后用三角函数计算相应的x和y坐标。
可以用哪些其他库来绘制八分之一圆?
除了Matplotlib,Python中还有其他库可以用于绘制八分之一圆。例如,使用Pygame库可以创建图形界面并绘制八分之一圆。另一个选择是使用Tkinter库,结合Canvas组件,可以实现图形的绘制。不同的库有不同的特点,可以根据项目需求进行选择。
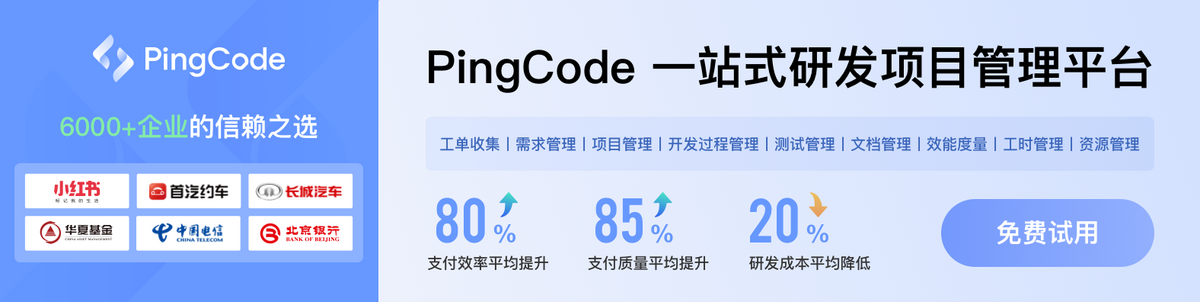