在Python中,要在一行输出到文件夹,可以使用适当的库,如os
和open
函数,来实现文件操作。例如,你可以使用open
函数与写入模式来创建并写入文件,再通过os
模块移动文件到指定目录。具体操作步骤包括:创建文件并写入内容、使用os
模块检查目录存在性及创建目录、移动文件到目标目录。下面详细描述其中的关键步骤。
一、创建文件并写入内容
要在Python中创建文件并写入内容,可以使用open
函数。open
函数可以打开一个文件,并返回一个文件对象。下面是一个简单的例子:
with open('example.txt', 'w') as file:
file.write('This is a test content')
在这个例子中,我们创建了一个名为example.txt
的文件,并写入了字符串'This is a test content'
。with
语句确保文件在处理完后会被正确关闭。
二、使用os
模块检查目录存在性及创建目录
在写入文件后,下一步是检查目标目录是否存在,并在必要时创建目录。os
模块提供了检查和创建目录的函数。下面是一个例子:
import os
directory = 'path/to/directory'
if not os.path.exists(directory):
os.makedirs(directory)
在这个例子中,我们首先检查目录'path/to/directory'
是否存在。如果不存在,我们使用os.makedirs
创建它。
三、移动文件到目标目录
写入内容并确保目标目录存在后,最后一步是将文件移动到目标目录。我们可以使用shutil
模块中的move
函数来完成这一任务:
import shutil
shutil.move('example.txt', 'path/to/directory/example.txt')
这个命令会将example.txt
移动到指定的目录中。
总结
结合以上步骤,我们可以在一行代码中实现文件的创建、写入及移动。以下是一个完整的例子,展示如何在一行代码中实现上述操作:
import os, shutil; open('example.txt', 'w').write('This is a test content'); os.makedirs('path/to/directory', exist_ok=True); shutil.move('example.txt', 'path/to/directory/example.txt')
详细分解各步骤
1、文件创建与内容写入
在这一步,我们使用open
函数以写入模式创建文件,并写入内容:
open('example.txt', 'w').write('This is a test content')
该语句创建了一个名为example.txt
的文件,并写入了字符串'This is a test content'
。使用'w'
模式时,如果文件已存在,将被覆盖。
2、检查并创建目录
为了确保目标目录存在,我们使用os.makedirs
函数:
os.makedirs('path/to/directory', exist_ok=True)
exist_ok=True
参数确保如果目录已经存在,不会引发异常。
3、移动文件
最后一步是移动文件到目标目录,使用shutil.move
函数:
shutil.move('example.txt', 'path/to/directory/example.txt')
该函数将文件移动到指定目录,如果目标目录中已经存在同名文件,将被覆盖。
实际应用场景
在实际应用中,这种方法可以用于多种场景。例如,日志记录、数据导出、自动化任务等。在数据分析和科学计算中,常常需要将中间结果或最终结果输出到文件,并按需组织到指定目录中。
代码优化与扩展
在实际项目中,可以将上述代码封装成一个函数,以便于重复使用和维护。例如:
import os
import shutil
def write_and_move(content, filename, directory):
with open(filename, 'w') as file:
file.write(content)
os.makedirs(directory, exist_ok=True)
shutil.move(filename, os.path.join(directory, filename))
使用该函数
write_and_move('This is a test content', 'example.txt', 'path/to/directory')
这种封装方式使代码更加清晰、可维护,并且易于扩展。
异常处理与健壮性
在实际应用中,除了基本的文件操作外,还需要考虑异常处理,以增强代码的健壮性。例如,处理文件写入失败、目录创建失败等情况:
import os
import shutil
def write_and_move(content, filename, directory):
try:
with open(filename, 'w') as file:
file.write(content)
os.makedirs(directory, exist_ok=True)
shutil.move(filename, os.path.join(directory, filename))
except Exception as e:
print(f'An error occurred: {e}')
使用该函数
write_and_move('This is a test content', 'example.txt', 'path/to/directory')
通过这种方式,代码在遇到异常时可以提供有用的错误信息,帮助调试和解决问题。
性能优化
在处理大量文件时,性能可能成为一个问题。可以考虑使用多线程或多进程来提高效率。例如,使用concurrent.futures
模块来并行处理多个文件:
import os
import shutil
from concurrent.futures import ThreadPoolExecutor
def write_and_move(content, filename, directory):
try:
with open(filename, 'w') as file:
file.write(content)
os.makedirs(directory, exist_ok=True)
shutil.move(filename, os.path.join(directory, filename))
except Exception as e:
print(f'An error occurred: {e}')
使用线程池并行处理
contents = ['content1', 'content2', 'content3']
filenames = ['file1.txt', 'file2.txt', 'file3.txt']
directory = 'path/to/directory'
with ThreadPoolExecutor(max_workers=3) as executor:
executor.map(write_and_move, contents, filenames, [directory] * len(contents))
通过这种方式,可以有效提高处理大量文件的效率。
结语
在Python中,文件操作是常见的任务之一,通过合理使用标准库中的函数和模块,可以高效地完成文件的创建、写入和移动操作。希望本文提供的详细介绍和示例能够帮助你更好地理解和应用这些技术,从而提高编程效率和代码质量。
相关问答FAQs:
如何在Python中实现一行输出到指定文件夹?
在Python中,可以使用内置的open
函数来创建或打开一个文件,并使用write
方法将内容写入文件中。如果你希望将输出写入特定的文件夹,确保在指定文件路径时包含文件夹的完整路径。例如,使用以下代码可以实现:
with open('your_directory/your_file.txt', 'w') as f:
f.write('这是要输出的内容')
在这个示例中,your_directory
是你想要输出文件的文件夹名称,your_file.txt
是你想要创建或写入的文件名。
如何确保输出文件夹存在?
在尝试输出到一个文件夹之前,确认该文件夹已经存在是很重要的。如果文件夹不存在,可以使用os
模块中的makedirs
方法创建它。以下是一个示例代码:
import os
directory = 'your_directory'
if not os.path.exists(directory):
os.makedirs(directory)
with open(os.path.join(directory, 'your_file.txt'), 'w') as f:
f.write('这是要输出的内容')
这个代码片段会检查文件夹是否存在,如果不存在,就会创建它,然后再进行写入操作。
如何处理写入文件时可能出现的错误?
在文件写入过程中,可能会遇到一些常见的错误,如权限问题或路径错误。可以通过使用try-except
块来捕获并处理这些错误。例如:
try:
with open('your_directory/your_file.txt', 'w') as f:
f.write('这是要输出的内容')
except OSError as e:
print(f'写入文件时出现错误: {e}')
这样可以确保在写入文件时,如果发生错误,程序不会崩溃,并且会输出相应的错误信息,便于调试和修复问题。
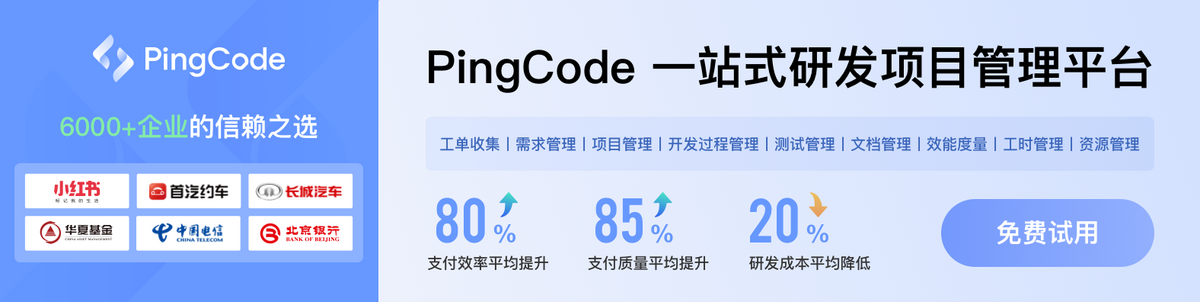