在Python中移除字符串中指定字符串的方法有多种,常见的方法包括使用replace()方法、正则表达式、split()和join()方法以及自定义函数。其中,最常用和简便的方法是使用replace()方法。这种方法不仅直观易懂,而且能够处理大多数字符串操作需求。下面我们将详细介绍这些方法的具体使用和适用场景。
一、使用replace()方法
replace()方法是Python中处理字符串替换的常用方法。它的基本语法是:
str.replace(old, new[, count])
其中,old
是要被替换的子字符串,new
是替换后的子字符串,count
是可选参数,表示替换的次数。
示例代码
original_str = "Hello, world! Hello, everyone!"
modified_str = original_str.replace("Hello", "")
print(modified_str) # 输出: ", world! , everyone!"
在这个例子中,replace("Hello", "")
将字符串中的所有"Hello"替换为空字符串,从而实现了移除"Hello"的目的。
使用场景
replace()方法适用于大多数简单的字符串替换和移除任务,尤其是当目标子字符串明确且不涉及复杂匹配规则时。
二、使用正则表达式
正则表达式(Regular Expressions,简称regex)是处理字符串的强大工具,特别适用于复杂的字符串匹配和替换任务。在Python中,正则表达式由re模块提供支持。
示例代码
import re
original_str = "Hello, world! Hello, everyone!"
pattern = "Hello"
modified_str = re.sub(pattern, "", original_str)
print(modified_str) # 输出: ", world! , everyone!"
在这个例子中,re.sub(pattern, "", original_str)
使用正则表达式将所有匹配pattern
的子字符串替换为空字符串,从而实现移除目的。
使用场景
正则表达式适用于需要复杂匹配规则的字符串操作,比如匹配特定模式的字符串、忽略大小写等。
三、使用split()和join()方法
split()方法将字符串按照指定的分隔符拆分成列表,join()方法将列表中的元素连接成一个新的字符串。通过将字符串拆分、过滤并重新连接,可以实现移除指定子字符串的目的。
示例代码
original_str = "Hello, world! Hello, everyone!"
split_str = original_str.split("Hello")
modified_str = "".join(split_str)
print(modified_str) # 输出: ", world! , everyone!"
在这个例子中,split("Hello")
将字符串按照"Hello"拆分成列表,join(split_str)
将列表元素重新连接成一个新的字符串,从而实现移除目的。
使用场景
split()和join()方法适用于需要对字符串进行复杂处理或多步处理的场景。
四、自定义函数
在某些特殊情况下,可能需要自定义函数来处理字符串移除任务。这种方法灵活性高,可以根据具体需求进行定制。
示例代码
def remove_substring(original_str, substring):
result = ""
i = 0
while i < len(original_str):
if original_str[i:i+len(substring)] == substring:
i += len(substring)
else:
result += original_str[i]
i += 1
return result
original_str = "Hello, world! Hello, everyone!"
modified_str = remove_substring(original_str, "Hello")
print(modified_str) # 输出: ", world! , everyone!"
在这个例子中,自定义函数remove_substring
通过遍历字符串并逐字符比较的方式移除指定子字符串。
使用场景
自定义函数适用于需要高度定制化字符串处理逻辑的场景。
五、性能比较
在实际应用中,不同方法的性能可能会有所差异。一般来说,replace()方法的性能较好,适合大多数场景。正则表达式的性能可能较差,但适用于复杂匹配任务。split()和join()方法以及自定义函数的性能取决于具体实现和应用场景。
性能测试
import time
测试数据
original_str = "Hello, world! " * 1000
测试replace方法
start_time = time.time()
modified_str = original_str.replace("Hello", "")
end_time = time.time()
print("replace方法耗时:", end_time - start_time)
测试正则表达式方法
start_time = time.time()
modified_str = re.sub("Hello", "", original_str)
end_time = time.time()
print("正则表达式方法耗时:", end_time - start_time)
测试split和join方法
start_time = time.time()
split_str = original_str.split("Hello")
modified_str = "".join(split_str)
end_time = time.time()
print("split和join方法耗时:", end_time - start_time)
测试自定义函数方法
start_time = time.time()
modified_str = remove_substring(original_str, "Hello")
end_time = time.time()
print("自定义函数方法耗时:", end_time - start_time)
通过上述性能测试,可以根据具体场景选择最合适的方法。
六、总结
在Python中移除字符串中指定字符串的方法多种多样,常见的包括replace()方法、正则表达式、split()和join()方法以及自定义函数。其中,replace()方法最为简单和直观,适用于大多数场景;正则表达式适用于复杂匹配任务;split()和join()方法适用于多步处理任务;自定义函数适用于高度定制化需求。根据具体应用场景选择合适的方法,可以有效提高代码的可读性和性能。
无论选择哪种方法,都应注意代码的可读性和维护性,尽量避免过于复杂的实现,以便在后续维护过程中能够快速理解和修改代码。
相关问答FAQs:
如何在Python中移除字符串中的多个指定子字符串?
在Python中,可以使用str.replace()
方法循环遍历要移除的多个子字符串,或使用正则表达式的re.sub()
函数。示例代码如下:
import re
original_string = "Hello, this is a sample string."
to_remove = ["Hello", "sample"]
# 使用replace循环移除
for item in to_remove:
original_string = original_string.replace(item, "")
print(original_string.strip()) # 输出: "this is a string."
# 使用正则表达式移除
pattern = '|'.join(map(re.escape, to_remove))
new_string = re.sub(pattern, '', original_string).strip()
print(new_string) # 输出: "this is a string."
在Python中移除字符串时,是否会影响原字符串?
在Python中,字符串是不可变的。这意味着移除操作不会改变原始字符串,而是返回一个新的字符串。你可以将新字符串赋值给一个变量,以保留更改。例如:
new_string = original_string.replace("sample", "")
在此示例中,original_string
保持不变,而new_string
包含已移除子字符串的版本。
有没有简便的方法可以移除字符串中的特定字符或子字符串?
可以使用列表推导式与join()
方法结合来简化移除字符串中的特定字符或子字符串的过程。例如:
original_string = "Hello, World!"
to_remove = "lo"
# 移除指定字符
new_string = ''.join(char for char in original_string if char not in to_remove)
print(new_string) # 输出: "He, Wr!"
这种方法可以快速地排除字符串中的任何字符,而不需要使用复杂的循环或正则表达式。
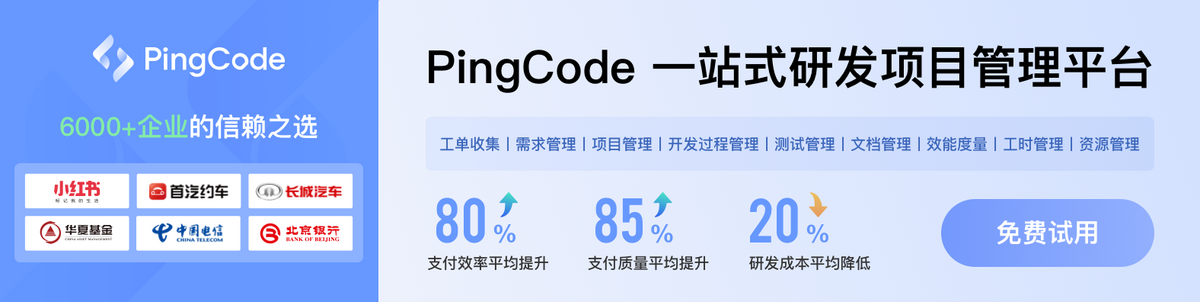