在Python中判断字符串是否包含某个字符,可以使用多种方法:使用in
操作符、find()
方法、index()
方法、count()
方法。 使用in
操作符是最常见且高效的方法,因为它简单直观。下面将详细介绍这些方法,并提供示例代码来帮助你理解和应用。
一、使用 in
操作符
in
操作符是Python中用于检查子字符串是否存在于字符串中的最直接和常用的方法。它返回一个布尔值,表示子字符串是否存在。
string = "Hello, world!"
char = "o"
if char in string:
print(f"The character '{char}' is in the string.")
else:
print(f"The character '{char}' is not in the string.")
在上面的示例中,char
变量包含我们要检查的字符。if char in string:
语句将返回True
或False
,取决于char
是否存在于string
中。
二、使用 find()
方法
find()
方法返回子字符串在字符串中首次出现的索引,如果子字符串不在字符串中,则返回-1
。这是一个用于判断子字符串是否存在的有效方法。
string = "Hello, world!"
char = "o"
index = string.find(char)
if index != -1:
print(f"The character '{char}' is in the string at index {index}.")
else:
print(f"The character '{char}' is not in the string.")
在这个例子中,find()
方法返回字符在字符串中的索引,如果找到则返回其位置,否则返回-1
。
三、使用 index()
方法
index()
方法类似于find()
,但如果子字符串不在字符串中,它会引发一个ValueError
异常。这意味着你需要使用try
和except
块来处理异常。
string = "Hello, world!"
char = "o"
try:
index = string.index(char)
print(f"The character '{char}' is in the string at index {index}.")
except ValueError:
print(f"The character '{char}' is not in the string.")
在这个示例中,index()
方法返回字符在字符串中的索引,如果找不到字符则引发异常并打印相应的消息。
四、使用 count()
方法
count()
方法返回子字符串在字符串中出现的次数。如果次数大于0,则表示子字符串存在。
string = "Hello, world!"
char = "o"
count = string.count(char)
if count > 0:
print(f"The character '{char}' is in the string and appears {count} times.")
else:
print(f"The character '{char}' is not in the string.")
在这个例子中,count()
方法返回字符在字符串中出现的次数,如果次数大于0,则表示字符存在并打印其出现的次数。
五、应用场景和性能比较
不同方法适用于不同的场景:
in
操作符:最常用和最直观的方法,适用于大多数情况。find()
方法:当你需要知道字符的位置但不想处理异常时使用。index()
方法:当你希望直接处理异常情况时使用。count()
方法:当你需要知道字符出现的次数时使用。
在性能方面,in
操作符通常是最快的,因为它是内置的且直接在C语言级别实现。find()
和 index()
方法的性能相似,但 index()
方法会在找不到字符时引发异常。count()
方法会遍历整个字符串,因此在需要频繁检查字符存在时可能效率较低。
六、扩展应用
除了判断单个字符是否存在,这些方法还可以用于判断子字符串是否存在。例如:
string = "Hello, world!"
substring = "world"
if substring in string:
print(f"The substring '{substring}' is in the string.")
else:
print(f"The substring '{substring}' is not in the string.")
这种方法对单个字符和子字符串同样适用。
总结
判断字符串中是否包含某个字符在Python中有多种方法,每种方法都有其适用的场景和优缺点。通过in
操作符、find()
方法、index()
方法、count()
方法,你可以高效地实现这一需求。根据具体的应用场景和性能要求,选择最合适的方法将有助于优化代码的可读性和执行效率。
相关问答FAQs:
如何在Python中检查一个字符串是否包含特定字符?
在Python中,可以使用in
运算符来判断一个字符串是否包含某个字符。例如,如果你想检查字符串my_string
中是否包含字符a
,可以使用如下代码:
if 'a' in my_string:
print("字符串中包含字符 'a'")
这种方法简洁且易于理解,适合初学者使用。
Python中有哪些方法可以查找字符的索引位置?
除了检查字符是否存在,你还可以使用str.find()
或str.index()
方法来找到字符在字符串中的位置。find()
方法返回字符的索引,如果未找到则返回-1
;而index()
方法在未找到字符时会引发异常。使用示例:
index = my_string.find('a')
if index != -1:
print(f"字符 'a' 在字符串中的位置是: {index}")
如何判断多个字符是否存在于字符串中?
如果需要检查多个字符是否都在字符串中,可以使用集合运算或循环。利用集合的交集可以快速判断,如下所示:
my_string = "hello world"
characters_to_check = {'h', 'w', 'o'}
if characters_to_check.issubset(set(my_string)):
print("字符串中包含所有指定字符")
else:
print("字符串中不包含所有指定字符")
这种方法非常高效,可以轻松扩展到任意数量的字符检查。
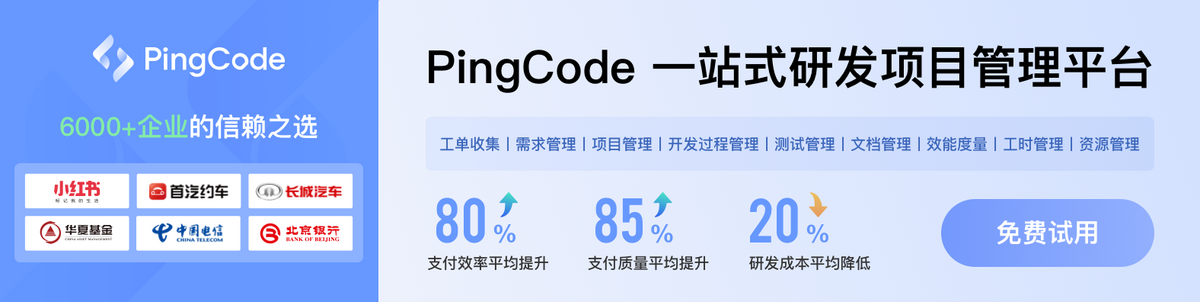