格式化操作符在Python中是通过占位符、格式说明符、字符串模板、函数和方法的组合来工作的。 这些工具和技术使得在Python中动态生成和处理字符串变得非常方便。占位符是最基本的格式化工具,通常是以百分号(%)作为标记。格式说明符则可以具体规定数据的格式,如整数、浮点数、字符串等。字符串模板和format函数进一步增强了格式化的灵活性和可读性。f-string(格式化字符串字面值)是Python 3.6引入的一种新的格式化方式,它提供了更简洁和直观的语法。下面将详细解释这些概念及其使用方法。
一、占位符与格式说明符
占位符是最基本的格式化工具,通常使用百分号(%)作为标记。格式说明符则决定了插入数据的类型和格式。
1. 占位符
在Python中,最常见的占位符有以下几种:
%d
:用于整数%f
:用于浮点数%s
:用于字符串
示例:
name = "John"
age = 30
print("My name is %s and I am %d years old." % (name, age))
2. 格式说明符
格式说明符可以进一步指定数据的显示格式,如小数点精度、宽度等。
示例:
pi = 3.141592653589793
print("Pi to three decimal places: %.3f" % pi)
二、字符串模板
字符串模板提供了一种更灵活的字符串格式化方式。它们使用string.Template
类,并用${}
作为占位符。
1. 使用字符串模板
示例:
from string import Template
template = Template("Hello, my name is ${name} and I am ${age} years old.")
result = template.substitute(name="John", age=30)
print(result)
三、format方法
format
方法是Python 3中引入的一种更强大、更灵活的字符串格式化方式。它支持位置参数和关键字参数,并允许更复杂的格式化操作。
1. 位置参数
示例:
print("My name is {} and I am {} years old.".format("John", 30))
2. 关键字参数
示例:
print("My name is {name} and I am {age} years old.".format(name="John", age=30))
四、f-string(格式化字符串字面值)
f-string是Python 3.6引入的格式化方式,它提供了最简洁和直观的语法。可以在字符串中直接嵌入变量和表达式。
1. 基本用法
示例:
name = "John"
age = 30
print(f"My name is {name} and I am {age} years old.")
2. 表达式
示例:
x = 10
y = 5
print(f"The sum of {x} and {y} is {x + y}.")
五、深入理解和高级用法
通过以上四种方式的基本用法,我们已经了解了Python中格式化操作符的基本工作原理。接下来,我们将深入探讨它们的高级用法和一些具体的应用场景。
一、占位符与格式说明符的高级用法
占位符和格式说明符不仅可以进行简单的数据插入,还可以进行更复杂的格式控制,如对齐、填充、精度设置等。
1. 对齐与填充
可以在格式说明符中指定对齐方式和填充字符。
示例:
name = "John"
print("My name is %-10s is awesome!" % name) # 左对齐
print("My name is %10s is awesome!" % name) # 右对齐
2. 精度与宽度
可以指定浮点数的精度和显示宽度。
示例:
pi = 3.141592653589793
print("Pi to three decimal places: %10.3f" % pi)
二、字符串模板的高级用法
字符串模板在处理复杂字符串时非常有用,特别是在需要动态生成字符串的场景中。
1. 使用safe_substitute避免KeyError
在使用substitute
方法时,如果缺少某些占位符,会抛出KeyError
。使用safe_substitute
可以避免这个问题。
示例:
template = Template("Hello, my name is ${name} and I am ${age} years old.")
result = template.safe_substitute(name="John")
print(result) # 输出:Hello, my name is John and I am ${age} years old.
三、format方法的高级用法
format
方法支持更复杂的格式化操作,如嵌套字段、字典参数、对象属性等。
1. 嵌套字段
可以在格式字符串中嵌套字段,以实现更复杂的格式化。
示例:
person = {'name': 'John', 'age': 30}
print("My name is {0[name]} and I am {0[age]} years old.".format(person))
2. 对象属性
可以通过format
方法访问对象的属性。
示例:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("John", 30)
print("My name is {0.name} and I am {0.age} years old.".format(person))
四、f-string的高级用法
f-string不仅可以嵌入变量,还可以嵌入表达式和函数调用。
1. 表达式与函数调用
在f-string中,可以直接嵌入表达式和函数调用,极大地提高了灵活性和可读性。
示例:
x = 10
y = 5
print(f"The sum of {x} and {y} is {x + y}.")
print(f"The product of {x} and {y} is {x * y}.")
print(f"The maximum of {x} and {y} is {max(x, y)}.")
2. 多行f-string
f-string也支持多行字符串,这在处理复杂文本时非常有用。
示例:
name = "John"
age = 30
message = (
f"My name is {name} and I am {age} years old.\n"
f"I love programming in Python!"
)
print(message)
五、性能与最佳实践
在处理大量字符串时,性能是一个需要考虑的重要因素。不同的格式化方式在性能上有不同的表现。
1. 性能比较
根据经验,f-string通常是最快的格式化方式,其次是format
方法,而占位符(%)和字符串模板则相对较慢。
示例:
import timeit
name = "John"
age = 30
f-string
time_fstring = timeit.timeit('f"My name is {name} and I am {age} years old."', globals=globals())
format
time_format = timeit.timeit('"My name is {} and I am {} years old.".format(name, age)', globals=globals())
占位符
time_placeholder = timeit.timeit('"My name is %s and I am %d years old." % (name, age)', globals=globals())
字符串模板
from string import Template
template = Template("My name is ${name} and I am ${age} years old.")
time_template = timeit.timeit('template.substitute(name="John", age=30)', globals=globals())
print(f"f-string: {time_fstring}")
print(f"format: {time_format}")
print(f"占位符: {time_placeholder}")
print(f"字符串模板: {time_template}")
2. 最佳实践
根据不同的应用场景选择最合适的格式化方式是最佳实践。例如,在需要高性能的场景中,优先使用f-string;在需要灵活格式化的场景中,使用format
方法;在处理复杂模板时,使用字符串模板。
总结:
通过本文,我们详细探讨了Python中格式化操作符的工作原理和具体使用方法。占位符、格式说明符、字符串模板、format方法、f-string各有其优缺点和适用场景。掌握这些工具和技术,不仅可以提高代码的可读性和灵活性,还可以在不同场景中选择最合适的解决方案。希望这些内容对您深入理解和应用Python的字符串格式化有所帮助。
相关问答FAQs:
格式化操作符在Python中有哪些类型?
格式化操作符主要有两个:%
和 format()
。%
是一种传统的格式化方式,类似于C语言中的printf函数。通过在字符串中使用占位符(如%s
、%d
等),可以将变量的值插入到指定位置。而format()
方法则提供了更灵活的格式化选项,可以通过花括号 {}
来定义占位符,并允许更复杂的格式设置。
如何使用格式化操作符将多个变量插入到字符串中?
在使用%
操作符时,可以将多个变量放入一个元组中,例如:"Hello, %s. You are %d years old." % (name, age)
。对于format()
方法,可以在花括号中使用索引,像这样:"Hello, {}. You are {} years old.".format(name, age)
。这两种方法都能有效地将多个变量插入到字符串中,保持代码的清晰和可读。
在Python中,何时应该使用格式化操作符而不是其他字符串格式化方法?
虽然%
操作符和format()
方法都可以实现字符串格式化,但在Python 3.6及以后的版本中,f-string(格式化字符串字面量)更为推荐,因为它提供了更简洁的语法和更好的性能。如果你的代码需要兼容较早版本的Python,或是需要使用较为复杂的格式化选项,那么format()
方法会是一个不错的选择。对于简单的格式化需求,%
操作符仍然是可用的,但建议逐步过渡到更新的格式化方法。
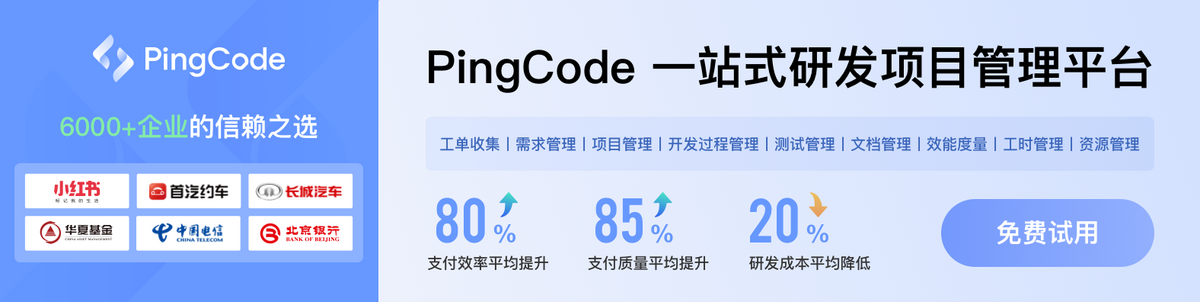