在Python中开发web报表可以通过使用多种工具和库来实现。常用的方法包括使用Django框架、Flask框架、Pandas库、以及Plotly库等。在这些方法中,使用Django框架和Pandas库是较为常见的方式。Django框架可以帮助快速开发具有丰富功能的web应用,而Pandas库则是处理数据和生成报表的强大工具。下面将详细介绍如何使用Django框架和Pandas库来开发web报表。
一、环境配置与准备
在开始开发之前,首先需要配置开发环境并安装必要的库和工具。
1、安装Python
首先,确保你已经安装了Python。建议使用Python 3.7以上的版本。可以通过命令行运行以下命令来检查Python是否已经安装:
python --version
2、安装Django
Django是一个高级的Python web框架,能够让开发者快速开发web应用。使用pip来安装Django:
pip install django
3、安装Pandas
Pandas是一个强大的数据处理和分析库。使用pip来安装Pandas:
pip install pandas
4、安装其他必要库
根据项目需求,可能还需要安装其他库,比如用于数据可视化的Plotly库:
pip install plotly
二、使用Django创建项目和应用
1、创建Django项目
使用以下命令创建一个新的Django项目:
django-admin startproject report_project
cd report_project
2、创建Django应用
在项目中创建一个新的应用:
python manage.py startapp report_app
3、配置Django应用
在项目的settings.py文件中,添加刚刚创建的应用到INSTALLED_APPS列表中:
INSTALLED_APPS = [
...
'report_app',
]
三、设计报表数据模型
1、定义数据模型
在report_app目录下的models.py文件中,定义报表的数据模型。假设我们要创建一个销售数据报表:
from django.db import models
class SalesReport(models.Model):
date = models.DateField()
product = models.CharField(max_length=100)
quantity = models.IntegerField()
revenue = models.DecimalField(max_digits=10, decimal_places=2)
def __str__(self):
return f"{self.date} - {self.product}"
2、创建数据库
在settings.py文件中配置数据库连接,并运行以下命令来创建数据库表:
python manage.py makemigrations
python manage.py migrate
四、开发报表视图与模板
1、创建报表视图
在report_app目录下的views.py文件中,创建一个视图来处理报表数据的展示:
from django.shortcuts import render
from .models import SalesReport
import pandas as pd
def report_view(request):
sales_data = SalesReport.objects.all()
data = {
'sales_data': sales_data
}
return render(request, 'report_app/report.html', data)
2、创建模板
在report_app目录下的templates/report_app目录中,创建一个report.html模板文件来展示报表数据:
<!DOCTYPE html>
<html>
<head>
<title>Sales Report</title>
</head>
<body>
<h1>Sales Report</h1>
<table border="1">
<tr>
<th>Date</th>
<th>Product</th>
<th>Quantity</th>
<th>Revenue</th>
</tr>
{% for report in sales_data %}
<tr>
<td>{{ report.date }}</td>
<td>{{ report.product }}</td>
<td>{{ report.quantity }}</td>
<td>{{ report.revenue }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
3、配置URL
在report_app目录下的urls.py文件中,配置报表视图的URL:
from django.urls import path
from . import views
urlpatterns = [
path('report/', views.report_view, name='report_view'),
]
在项目的urls.py文件中包含应用的URL配置:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('report_app.urls')),
]
五、数据处理与报表生成
1、使用Pandas处理数据
Pandas可以帮助我们高效地处理和分析数据。在views.py文件中,我们可以使用Pandas来处理数据并生成报表:
import pandas as pd
def report_view(request):
sales_data = SalesReport.objects.all().values()
df = pd.DataFrame(sales_data)
# 数据处理和分析
total_revenue = df['revenue'].sum()
total_quantity = df['quantity'].sum()
data = {
'sales_data': sales_data,
'total_revenue': total_revenue,
'total_quantity': total_quantity,
}
return render(request, 'report_app/report.html', data)
在模板中展示处理后的数据:
<!DOCTYPE html>
<html>
<head>
<title>Sales Report</title>
</head>
<body>
<h1>Sales Report</h1>
<p>Total Revenue: {{ total_revenue }}</p>
<p>Total Quantity: {{ total_quantity }}</p>
<table border="1">
<tr>
<th>Date</th>
<th>Product</th>
<th>Quantity</th>
<th>Revenue</th>
</tr>
{% for report in sales_data %}
<tr>
<td>{{ report.date }}</td>
<td>{{ report.product }}</td>
<td>{{ report.quantity }}</td>
<td>{{ report.revenue }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
2、生成图表
使用Plotly库生成图表,将数据可视化展示在报表中。在views.py文件中,生成图表并将其传递到模板:
import plotly.express as px
def report_view(request):
sales_data = SalesReport.objects.all().values()
df = pd.DataFrame(sales_data)
# 数据处理和分析
total_revenue = df['revenue'].sum()
total_quantity = df['quantity'].sum()
# 生成图表
fig = px.bar(df, x='product', y='revenue', title='Revenue by Product')
chart = fig.to_html(full_html=False)
data = {
'sales_data': sales_data,
'total_revenue': total_revenue,
'total_quantity': total_quantity,
'chart': chart,
}
return render(request, 'report_app/report.html', data)
在模板中嵌入图表:
<!DOCTYPE html>
<html>
<head>
<title>Sales Report</title>
<script src="https://cdn.plot.ly/plotly-latest.min.js"></script>
</head>
<body>
<h1>Sales Report</h1>
<p>Total Revenue: {{ total_revenue }}</p>
<p>Total Quantity: {{ total_quantity }}</p>
{{ chart|safe }}
<table border="1">
<tr>
<th>Date</th>
<th>Product</th>
<th>Quantity</th>
<th>Revenue</th>
</tr>
{% for report in sales_data %}
<tr>
<td>{{ report.date }}</td>
<td>{{ report.product }}</td>
<td>{{ report.quantity }}</td>
<td>{{ report.revenue }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
六、部署与发布
1、配置静态文件
在settings.py文件中配置静态文件目录:
STATIC_URL = '/static/'
STATICFILES_DIRS = [
BASE_DIR / "static",
]
2、使用Gunicorn和Nginx部署
使用Gunicorn作为Wsgi服务器,并使用Nginx作为反向代理服务器来部署Django应用。首先安装Gunicorn:
pip install gunicorn
然后运行Gunicorn:
gunicorn report_project.wsgi:application
配置Nginx来代理Gunicorn,编辑Nginx配置文件:
server {
listen 80;
server_name your_domain;
location / {
proxy_pass http://127.0.0.1:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
重启Nginx服务:
sudo systemctl restart nginx
七、总结
通过以上步骤,我们可以使用Django框架和Pandas库来开发一个功能强大的web报表应用。Django框架提供了强大的web开发工具,Pandas库提供了高效的数据处理和分析功能,结合使用可以快速开发出高质量的web报表应用。此外,可以使用Plotly库进行数据可视化,进一步提升报表的用户体验和数据展示效果。
相关问答FAQs:
如何选择适合的Python框架来开发web报表?
在开发web报表时,选择合适的框架至关重要。常见的Python框架包括Flask、Django和FastAPI。Flask轻量且灵活,非常适合小型项目,Django则提供了完整的功能,适合大型应用,而FastAPI则专注于高性能的API开发。依据项目需求、团队技能和预期的扩展性来选择框架,将有助于提高开发效率。
在Python中如何处理和展示数据以生成报表?
处理和展示数据通常涉及几个步骤。首先,可以使用Pandas库进行数据清洗和处理,Pandas提供了强大的数据框架,方便进行复杂的数据操作。接下来,使用Matplotlib或Seaborn等可视化库将数据可视化,生成图表或图形。最后,将处理后的数据和图形集成到web应用中,使用HTML模板引擎如Jinja2来渲染动态报表。
如何确保开发的web报表具有良好的用户体验?
为了确保web报表的用户体验,设计时应考虑清晰的界面布局和直观的导航。使用响应式设计,使报表在不同设备上都能良好展示。此外,优化加载速度也是提升用户体验的重要因素,可以通过数据分页、懒加载等技术减少初始加载的时间。最后,提供导出功能,如PDF或Excel格式,增加用户的便利性。
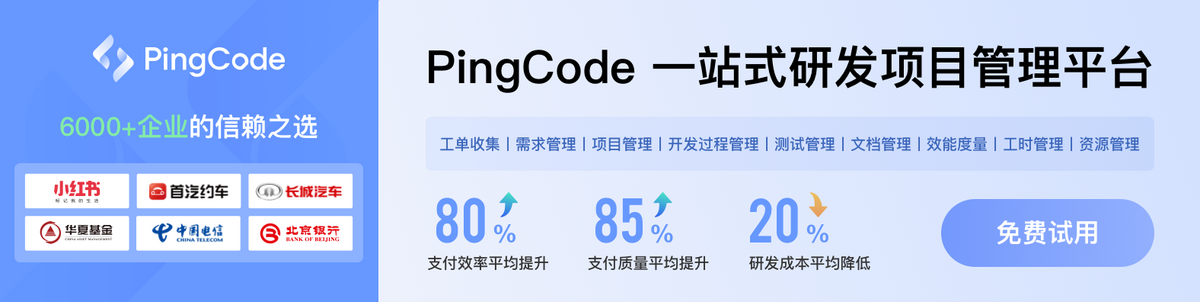