要使用Python爬虫获取天气信息,可以通过使用requests库发送HTTP请求、解析网站内容,使用BeautifulSoup库解析HTML、从公开的天气API获取数据。其中,使用公开的天气API是最为简单和可靠的方式。比如,可以使用OpenWeatherMap API来获取实时天气数据。下面将详细介绍如何使用这些方法来获取天气信息。
一、通过使用Requests库发送HTTP请求
使用requests库发送HTTP请求是获取网页内容的第一步。requests库是Python中最常用的HTTP库之一,提供了简单的API来发送HTTP请求并获取响应内容。以下是一个基本的示例:
import requests
def get_weather_data(city):
url = f"http://example.com/weather/{city}"
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
return None
city = 'Beijing'
weather_data = get_weather_data(city)
print(weather_data)
在这个示例中,我们通过构建一个URL并发送HTTP GET请求来获取指定城市的天气数据。
二、使用BeautifulSoup库解析HTML
获取网页内容后,下一步就是解析HTML以提取所需的数据。BeautifulSoup是一个强大的HTML和XML解析库,适用于从HTML文档中提取数据。以下是一个示例:
from bs4 import BeautifulSoup
import requests
def get_weather_data(city):
url = f"http://example.com/weather/{city}"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
weather_info = soup.find('div', class_='weather-info')
return weather_info.text
else:
return None
city = 'Beijing'
weather_data = get_weather_data(city)
print(weather_data)
在这个示例中,我们使用BeautifulSoup解析HTML,并找到包含天气信息的特定HTML元素。
三、从公开的天气API获取数据
使用公开的天气API是获取天气数据最可靠的方式。OpenWeatherMap是一个广泛使用的天气API,提供了丰富的天气数据。要使用OpenWeatherMap API,您需要注册一个API密钥。以下是一个示例:
import requests
def get_weather_data(city, api_key):
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
if response.status_code == 200:
return response.json()
else:
return None
api_key = 'your_api_key'
city = 'Beijing'
weather_data = get_weather_data(city, api_key)
print(weather_data)
在这个示例中,我们使用OpenWeatherMap API获取指定城市的天气数据,并将响应内容解析为JSON格式。
四、解析API返回的数据
API返回的数据通常是JSON格式,我们可以使用Python的json库来解析这些数据。以下是一个详细的示例:
import requests
import json
def get_weather_data(city, api_key):
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
if response.status_code == 200:
return response.json()
else:
return None
def parse_weather_data(data):
if data:
main = data.get('main', {})
weather = data.get('weather', [{}])[0]
temperature = main.get('temp')
weather_description = weather.get('description')
return {
'temperature': temperature,
'description': weather_description
}
else:
return None
api_key = 'your_api_key'
city = 'Beijing'
weather_data = get_weather_data(city, api_key)
parsed_data = parse_weather_data(weather_data)
print(parsed_data)
在这个示例中,我们定义了一个parse_weather_data函数来解析API返回的数据,并提取温度和天气描述等信息。
五、处理异常和错误
在实际应用中,可能会遇到各种异常和错误。我们需要添加适当的错误处理机制,以确保程序的鲁棒性。以下是一个示例:
import requests
import json
def get_weather_data(city, api_key):
try:
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
return None
def parse_weather_data(data):
if data:
main = data.get('main', {})
weather = data.get('weather', [{}])[0]
temperature = main.get('temp')
weather_description = weather.get('description')
return {
'temperature': temperature,
'description': weather_description
}
else:
return None
api_key = 'your_api_key'
city = 'Beijing'
weather_data = get_weather_data(city, api_key)
parsed_data = parse_weather_data(weather_data)
print(parsed_data)
在这个示例中,我们使用try-except块来捕获并处理可能的异常,并在发生错误时打印错误信息。
六、将天气数据保存到文件
有时,我们可能需要将获取的天气数据保存到文件中,以便后续处理。以下是一个示例:
import requests
import json
def get_weather_data(city, api_key):
try:
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
return None
def parse_weather_data(data):
if data:
main = data.get('main', {})
weather = data.get('weather', [{}])[0]
temperature = main.get('temp')
weather_description = weather.get('description')
return {
'temperature': temperature,
'description': weather_description
}
else:
return None
def save_weather_data(city, data):
with open(f'{city}_weather.json', 'w') as json_file:
json.dump(data, json_file)
api_key = 'your_api_key'
city = 'Beijing'
weather_data = get_weather_data(city, api_key)
parsed_data = parse_weather_data(weather_data)
if parsed_data:
save_weather_data(city, parsed_data)
print(f"Weather data for {city} saved to {city}_weather.json")
在这个示例中,我们定义了一个save_weather_data函数来将解析后的天气数据保存到JSON文件中。
七、定时获取天气数据
为了定期获取和记录天气数据,我们可以使用Python的定时任务库,比如schedule。以下是一个示例:
import requests
import json
import schedule
import time
def get_weather_data(city, api_key):
try:
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
return None
def parse_weather_data(data):
if data:
main = data.get('main', {})
weather = data.get('weather', [{}])[0]
temperature = main.get('temp')
weather_description = weather.get('description')
return {
'temperature': temperature,
'description': weather_description
}
else:
return None
def save_weather_data(city, data):
with open(f'{city}_weather.json', 'w') as json_file:
json.dump(data, json_file)
def fetch_and_save_weather_data(city, api_key):
weather_data = get_weather_data(city, api_key)
parsed_data = parse_weather_data(weather_data)
if parsed_data:
save_weather_data(city, parsed_data)
print(f"Weather data for {city} saved to {city}_weather.json")
api_key = 'your_api_key'
city = 'Beijing'
schedule.every().hour.do(fetch_and_save_weather_data, city, api_key)
while True:
schedule.run_pending()
time.sleep(1)
在这个示例中,我们使用schedule库每小时调用一次fetch_and_save_weather_data函数,以定期获取和保存天气数据。
八、发送天气数据通知
为了在天气数据更新时发送通知,我们可以使用第三方服务,比如Twilio发送短信通知。以下是一个示例:
import requests
import json
from twilio.rest import Client
def get_weather_data(city, api_key):
try:
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
return None
def parse_weather_data(data):
if data:
main = data.get('main', {})
weather = data.get('weather', [{}])[0]
temperature = main.get('temp')
weather_description = weather.get('description')
return {
'temperature': temperature,
'description': weather_description
}
else:
return None
def send_sms_notification(weather_data, to_phone, from_phone, twilio_sid, twilio_auth_token):
client = Client(twilio_sid, twilio_auth_token)
message = f"Current weather: {weather_data['description']}, Temperature: {weather_data['temperature']}K"
message = client.messages.create(
body=message,
from_=from_phone,
to=to_phone
)
return message.sid
api_key = 'your_api_key'
city = 'Beijing'
to_phone = '+1234567890'
from_phone = '+0987654321'
twilio_sid = 'your_twilio_sid'
twilio_auth_token = 'your_twilio_auth_token'
weather_data = get_weather_data(city, api_key)
parsed_data = parse_weather_data(weather_data)
if parsed_data:
sms_sid = send_sms_notification(parsed_data, to_phone, from_phone, twilio_sid, twilio_auth_token)
print(f"Notification sent with SID: {sms_sid}")
在这个示例中,我们使用Twilio发送短信通知,以便在天气数据更新时通知用户。
九、总结
通过上述步骤,我们可以使用Python爬虫和API获取天气信息。使用requests库发送HTTP请求、使用BeautifulSoup解析HTML、使用公开的天气API获取数据,并结合错误处理、文件保存、定时任务和通知发送等功能,可以构建一个完整的天气数据获取和处理系统。
无论是用于个人项目还是商业应用,掌握这些技巧将大大提高您的数据采集和处理能力。希望本文能够帮助您更好地理解和应用Python爬虫获取天气信息的方法。
相关问答FAQs:
如何使用Python爬虫获取实时天气数据?
要获取实时天气数据,您可以使用Python中的requests库来发送HTTP请求,从天气API或天气网站获取数据。具体步骤包括选择合适的API(例如OpenWeatherMap或WeatherAPI),注册并获取API密钥,构造API请求,最后解析返回的JSON数据并提取所需的天气信息。
在爬取天气数据时需要注意哪些法律和道德问题?
在爬取天气数据时,务必遵循网站的使用条款和条件,确保不违反任何法律法规。许多网站提供API供开发者合法使用数据,优先选择这些方式。此外,避免频繁请求同一页面,以免对网站造成负担或被封禁。
Python爬虫获取天气数据的常见错误和解决方案是什么?
常见错误包括请求超时、解析失败和数据格式错误。为解决这些问题,可以设置适当的请求超时、使用BeautifulSoup或lxml库进行HTML解析,并确保对返回数据进行格式检查。此外,调试时可以打印出请求的URL和返回的状态码,以便快速定位问题。
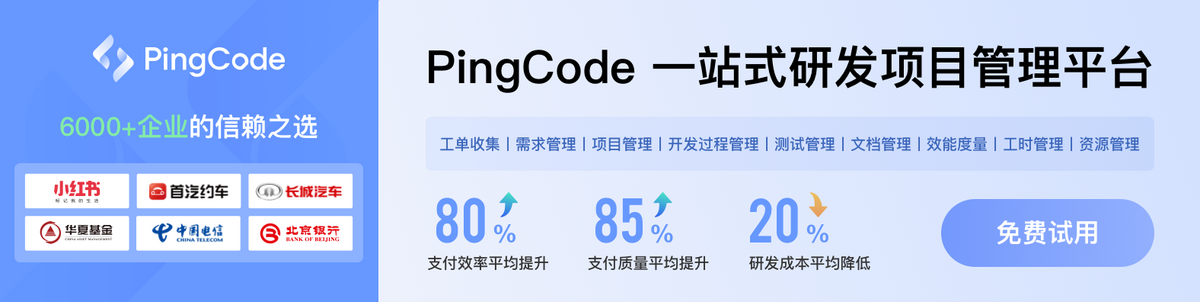