使用Python打开文件进行读写,可以通过多种方式实现,具体方式包括使用内置的open()函数、with语句上下文管理器、以及不同的文件模式。详细步骤如下:使用open()函数、指定文件模式、处理文件异常。 在详细描述中,我们将重点讲解如何使用with语句上下文管理器来确保文件在操作后自动关闭,防止资源泄露。
使用with语句上下文管理器时,打开文件并进行读写操作的代码结构如下:
with open('filename.txt', 'r') as file:
content = file.read()
通过使用with语句,文件在操作完成后会自动关闭,无需显式调用close()方法,这样可以避免因忘记关闭文件而导致的资源泄露问题。
一、使用open()函数
Python内置的open()函数是进行文件操作的最基本方法。它可以打开文件并返回文件对象,这个对象可以用来进行读写操作。open()函数的语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
其中,file是要打开的文件名,mode是文件打开模式。
1、文件模式
文件模式(mode)参数决定了文件的打开方式,常见的模式包括:
- 'r':只读模式(默认)。
- 'w':写模式,会覆盖文件内容。
- 'a':追加模式,在文件末尾追加内容。
- 'b':二进制模式。
- 't':文本模式(默认)。
- 'x':独占写入模式,文件已存在则报错。
- '+':读写模式。
2、示例代码
以下示例展示了如何使用open()函数打开文件进行读写操作:
# 读取文件内容
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
写入文件内容
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
二、使用with语句上下文管理器
使用with语句可以简化文件操作,并确保文件在操作完成后自动关闭。其语法如下:
with open('filename.txt', 'mode') as file:
# 文件操作
1、读取文件
使用with语句读取文件内容的示例如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2、写入文件
使用with语句写入文件内容的示例如下:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
三、处理文件异常
在进行文件读写操作时,可能会遇到文件不存在、权限不足等异常情况。为此,可以使用try-except语句进行异常处理。
1、捕获文件异常
以下示例展示了如何捕获文件异常:
try:
with open('nonexistent.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('File not found')
except PermissionError:
print('Permission denied')
2、处理其他异常
除了FileNotFoundError和PermissionError,还可以处理其他异常:
try:
with open('example.txt', 'r') as file:
content = file.read()
except Exception as e:
print(f'An error occurred: {e}')
四、读写二进制文件
对于二进制文件,如图片、音频等,可以使用'b'模式打开文件。
1、读取二进制文件
以下示例展示了如何读取二进制文件:
with open('image.png', 'rb') as file:
content = file.read()
2、写入二进制文件
以下示例展示了如何写入二进制文件:
with open('output.png', 'wb') as file:
file.write(content)
五、逐行读取文件
对于大文件,可以逐行读取文件内容,以节省内存。
1、使用for循环逐行读取
以下示例展示了如何使用for循环逐行读取文件:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
2、使用readlines()方法逐行读取
以下示例展示了如何使用readlines()方法逐行读取文件:
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
六、读写CSV文件
Python提供了csv模块来方便读写CSV文件。
1、读取CSV文件
以下示例展示了如何读取CSV文件:
import csv
with open('data.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
2、写入CSV文件
以下示例展示了如何写入CSV文件:
import csv
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['Alice', 30])
writer.writerow(['Bob', 25])
七、读写JSON文件
Python提供了json模块来方便读写JSON文件。
1、读取JSON文件
以下示例展示了如何读取JSON文件:
import json
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
2、写入JSON文件
以下示例展示了如何写入JSON文件:
import json
data = {'name': 'Alice', 'age': 30}
with open('output.json', 'w') as file:
json.dump(data, file)
八、读写Excel文件
Python提供了openpyxl和pandas等库来方便读写Excel文件。
1、使用openpyxl读取Excel文件
以下示例展示了如何使用openpyxl读取Excel文件:
from openpyxl import load_workbook
wb = load_workbook('data.xlsx')
sheet = wb.active
for row in sheet.iter_rows(values_only=True):
print(row)
2、使用pandas读取Excel文件
以下示例展示了如何使用pandas读取Excel文件:
import pandas as pd
df = pd.read_excel('data.xlsx')
print(df)
3、使用openpyxl写入Excel文件
以下示例展示了如何使用openpyxl写入Excel文件:
from openpyxl import Workbook
wb = Workbook()
sheet = wb.active
sheet['A1'] = 'Name'
sheet['B1'] = 'Age'
sheet.append(['Alice', 30])
sheet.append(['Bob', 25])
wb.save('output.xlsx')
4、使用pandas写入Excel文件
以下示例展示了如何使用pandas写入Excel文件:
import pandas as pd
data = {'Name': ['Alice', 'Bob'], 'Age': [30, 25]}
df = pd.DataFrame(data)
df.to_excel('output.xlsx', index=False)
九、读写XML文件
Python提供了xml.etree.ElementTree模块来方便读写XML文件。
1、读取XML文件
以下示例展示了如何读取XML文件:
import xml.etree.ElementTree as ET
tree = ET.parse('data.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
2、写入XML文件
以下示例展示了如何写入XML文件:
import xml.etree.ElementTree as ET
root = ET.Element('root')
child = ET.SubElement(root, 'child')
child.set('name', 'Alice')
child.text = '30'
tree = ET.ElementTree(root)
tree.write('output.xml')
十、读写压缩文件
Python提供了zipfile和tarfile模块来方便读写压缩文件。
1、使用zipfile读取压缩文件
以下示例展示了如何使用zipfile读取压缩文件:
import zipfile
with zipfile.ZipFile('data.zip', 'r') as zip_ref:
zip_ref.extractall('extracted')
2、使用zipfile写入压缩文件
以下示例展示了如何使用zipfile写入压缩文件:
import zipfile
with zipfile.ZipFile('output.zip', 'w') as zip_ref:
zip_ref.write('example.txt')
3、使用tarfile读取压缩文件
以下示例展示了如何使用tarfile读取压缩文件:
import tarfile
with tarfile.open('data.tar.gz', 'r:gz') as tar_ref:
tar_ref.extractall('extracted')
4、使用tarfile写入压缩文件
以下示例展示了如何使用tarfile写入压缩文件:
import tarfile
with tarfile.open('output.tar.gz', 'w:gz') as tar_ref:
tar_ref.add('example.txt')
十一、读写配置文件
Python提供了configparser模块来方便读写配置文件。
1、读取配置文件
以下示例展示了如何读取配置文件:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
print(config['DEFAULT']['Server'])
2、写入配置文件
以下示例展示了如何写入配置文件:
import configparser
config = configparser.ConfigParser()
config['DEFAULT'] = {'Server': '127.0.0.1', 'Port': '8080'}
with open('config.ini', 'w') as configfile:
config.write(configfile)
十二、读写日志文件
Python提供了logging模块来方便记录日志信息。
1、配置日志记录
以下示例展示了如何配置日志记录:
import logging
logging.basicConfig(filename='app.log', level=logging.INFO)
logging.info('This is an info message')
2、读取日志文件
日志文件本质上是普通的文本文件,可以使用前面介绍的文本文件读取方法来读取日志文件内容:
with open('app.log', 'r') as file:
content = file.read()
print(content)
十三、读写临时文件
Python提供了tempfile模块来方便创建和操作临时文件。
1、创建临时文件
以下示例展示了如何创建临时文件:
import tempfile
with tempfile.TemporaryFile() as temp_file:
temp_file.write(b'This is a temporary file')
temp_file.seek(0)
content = temp_file.read()
print(content)
2、创建临时目录
以下示例展示了如何创建临时目录:
import tempfile
with tempfile.TemporaryDirectory() as temp_dir:
print(f'Temporary directory: {temp_dir}')
十四、多线程读写文件
在多线程环境下读写文件需要特别注意数据一致性问题。
1、使用线程锁
以下示例展示了如何使用线程锁来确保多线程读写文件时的数据一致性:
import threading
lock = threading.Lock()
def write_to_file(filename, content):
with lock:
with open(filename, 'a') as file:
file.write(content + '\n')
threads = []
for i in range(5):
t = threading.Thread(target=write_to_file, args=('example.txt', f'Thread {i}'))
threads.append(t)
t.start()
for t in threads:
t.join()
2、使用Queue模块
以下示例展示了如何使用Queue模块来实现多线程读写文件:
import threading
import queue
q = queue.Queue()
def worker():
while not q.empty():
filename, content = q.get()
with open(filename, 'a') as file:
file.write(content + '\n')
q.task_done()
for i in range(5):
q.put(('example.txt', f'Thread {i}'))
threads = []
for i in range(3):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
q.join()
for t in threads:
t.join()
通过以上方法,可以灵活地使用Python进行文件读写操作,根据不同的需求选择合适的方式,确保数据的安全和操作的高效。
相关问答FAQs:
如何在Python中打开文件进行读写操作?
在Python中,可以使用内置的open()
函数来打开文件。这个函数有两个主要参数:文件名和模式。常用的模式包括:
'r'
:只读模式,文件必须存在。'w'
:写入模式,文件如果存在则会被覆盖,不存在则会创建。'a'
:追加模式,文件如果存在则在末尾添加内容,不存在则会创建。'r+'
:读写模式,文件必须存在。
示例代码如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这段代码将打开一个名为example.txt
的文件并读取其内容。
如何处理文件打开过程中的异常情况?
在打开文件时,可能会遇到一些异常情况,比如文件不存在或没有权限。可以使用try-except
语句来处理这些问题。例如:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请确认文件名是否正确。")
except PermissionError:
print("没有权限访问该文件。")
这样的处理方式可以提高程序的鲁棒性,避免因错误而导致程序崩溃。
使用with
语句有什么好处?
使用with
语句打开文件可以确保在使用完文件后自动关闭它,这样可以避免内存泄漏和文件占用等问题。即使在文件操作过程中发生异常,with
语句也会确保文件正确关闭。示例代码如下:
with open('example.txt', 'w') as file:
file.write("写入一些内容")
在这个例子中,文件在写入完成后会自动关闭,无需手动调用file.close()
。
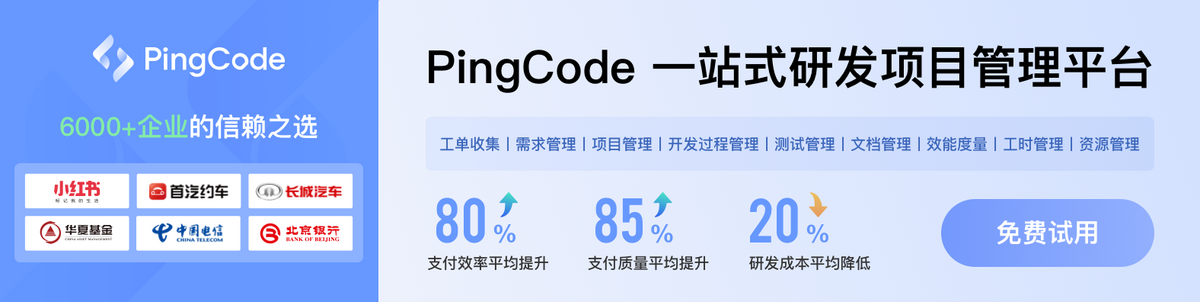