在Python中,可以通过使用requests库、beautifulsoup库、time库来获得网页计时。我们可以使用requests库来发送HTTP请求获取网页内容,然后用beautifulsoup库进行解析,最后用time库来记录请求的时间。以下是其中一个方法的详细描述。
使用time库来记录请求的时间。首先,我们需要导入相关库,然后使用time库的time()函数在发起请求前后记录时间差,以计算获取网页所花费的时间。
import requests
from bs4 import BeautifulSoup
import time
def get_webpage_time(url):
# 记录开始时间
start_time = time.time()
# 发起HTTP请求
response = requests.get(url)
# 记录结束时间
end_time = time.time()
# 计算时间差
time_taken = end_time - start_time
# 检查请求是否成功
if response.status_code == 200:
print(f"Successfully fetched the webpage in {time_taken} seconds")
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return time_taken
示例使用
url = "https://www.example.com"
get_webpage_time(url)
这种方法简单有效,能够准确地记录获取网页所花费的时间,可以应用于需要记录网页加载时间的各种场景。
一、使用requests库获取网页内容
requests库是Python中最常用的HTTP库之一,能够方便地发送HTTP请求并获取响应。我们可以使用requests库来获取网页内容。
1、安装requests库
在使用requests库之前,我们需要先安装它。可以使用以下命令来安装requests库:
pip install requests
2、发送HTTP请求
使用requests库发送HTTP请求非常简单。我们可以使用requests.get()方法来发送GET请求,并获取响应内容。以下是一个示例代码:
import requests
def fetch_webpage(url):
response = requests.get(url)
if response.status_code == 200:
print("Successfully fetched the webpage")
return response.text
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None
url = "https://www.example.com"
content = fetch_webpage(url)
print(content)
在这个示例中,我们使用requests.get()方法发送GET请求,并检查响应的状态码是否为200(表示请求成功)。如果请求成功,我们会返回网页的内容,否则会打印错误信息。
二、使用BeautifulSoup解析网页内容
BeautifulSoup是一个用于解析HTML和XML文档的Python库,能够方便地从网页中提取数据。我们可以使用BeautifulSoup来解析获取到的网页内容。
1、安装BeautifulSoup库
在使用BeautifulSoup库之前,我们需要先安装它。可以使用以下命令来安装BeautifulSoup库:
pip install beautifulsoup4
2、解析网页内容
使用BeautifulSoup解析网页内容非常简单。我们可以将获取到的网页内容传递给BeautifulSoup,然后使用各种方法来提取所需的数据。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
def fetch_and_parse_webpage(url):
response = requests.get(url)
if response.status_code == 200:
print("Successfully fetched the webpage")
soup = BeautifulSoup(response.text, 'html.parser')
return soup
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None
url = "https://www.example.com"
soup = fetch_and_parse_webpage(url)
print(soup.prettify())
在这个示例中,我们使用BeautifulSoup将获取到的网页内容解析为一个BeautifulSoup对象。然后,我们可以使用各种方法来提取所需的数据,例如使用soup.find()、soup.find_all()等方法。
三、使用time库记录请求时间
time库是Python标准库中的一个模块,提供了各种与时间相关的功能。我们可以使用time库来记录HTTP请求的时间。
1、记录请求时间
我们可以使用time库的time()函数来记录请求的时间。time()函数返回当前时间的时间戳(以秒为单位)。以下是一个示例代码:
import requests
import time
def fetch_webpage_with_time(url):
start_time = time.time()
response = requests.get(url)
end_time = time.time()
time_taken = end_time - start_time
if response.status_code == 200:
print(f"Successfully fetched the webpage in {time_taken} seconds")
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return time_taken
url = "https://www.example.com"
time_taken = fetch_webpage_with_time(url)
print(f"Time taken: {time_taken} seconds")
在这个示例中,我们在发送HTTP请求前后分别记录时间戳,并计算时间差,以此来记录请求所花费的时间。
四、结合requests、BeautifulSoup和time库
我们可以将requests、BeautifulSoup和time库结合起来,构建一个功能完整的脚本,用于获取网页内容、解析网页数据并记录请求时间。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
import time
def fetch_and_parse_webpage_with_time(url):
start_time = time.time()
response = requests.get(url)
end_time = time.time()
time_taken = end_time - start_time
if response.status_code == 200:
print(f"Successfully fetched the webpage in {time_taken} seconds")
soup = BeautifulSoup(response.text, 'html.parser')
return soup, time_taken
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None, time_taken
url = "https://www.example.com"
soup, time_taken = fetch_and_parse_webpage_with_time(url)
print(f"Time taken: {time_taken} seconds")
print(soup.prettify())
在这个示例中,我们将requests、BeautifulSoup和time库结合起来,首先记录请求的时间,然后获取网页内容并解析网页数据,最后打印请求所花费的时间和解析后的网页内容。
五、处理异常情况
在实际应用中,我们可能会遇到各种异常情况,例如网络连接失败、请求超时等。我们可以使用try-except语句来处理这些异常情况,确保脚本能够正常运行。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
import time
def fetch_and_parse_webpage_with_time(url):
try:
start_time = time.time()
response = requests.get(url, timeout=10)
end_time = time.time()
time_taken = end_time - start_time
if response.status_code == 200:
print(f"Successfully fetched the webpage in {time_taken} seconds")
soup = BeautifulSoup(response.text, 'html.parser')
return soup, time_taken
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None, time_taken
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None, None
url = "https://www.example.com"
soup, time_taken = fetch_and_parse_webpage_with_time(url)
if time_taken is not None:
print(f"Time taken: {time_taken} seconds")
if soup is not None:
print(soup.prettify())
在这个示例中,我们使用try-except语句来捕获可能的异常情况,例如网络连接失败、请求超时等。如果发生异常情况,我们会打印错误信息,并返回None。
六、优化和扩展
在实际应用中,我们可能需要对脚本进行优化和扩展,以满足不同的需求。以下是一些优化和扩展的建议:
1、使用多线程或异步方式提高效率
如果需要同时获取多个网页的内容,我们可以使用多线程或异步方式来提高效率。以下是一个使用多线程的示例代码:
import requests
from bs4 import BeautifulSoup
import time
from concurrent.futures import ThreadPoolExecutor
def fetch_and_parse_webpage_with_time(url):
try:
start_time = time.time()
response = requests.get(url, timeout=10)
end_time = time.time()
time_taken = end_time - start_time
if response.status_code == 200:
print(f"Successfully fetched the webpage in {time_taken} seconds")
soup = BeautifulSoup(response.text, 'html.parser')
return soup, time_taken
else:
print(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None, time_taken
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None, None
def fetch_multiple_webpages(urls):
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(fetch_and_parse_webpage_with_time, urls)
return list(results)
urls = ["https://www.example.com", "https://www.example.org", "https://www.example.net"]
results = fetch_multiple_webpages(urls)
for soup, time_taken in results:
if time_taken is not None:
print(f"Time taken: {time_taken} seconds")
if soup is not None:
print(soup.prettify())
在这个示例中,我们使用ThreadPoolExecutor来并行获取多个网页的内容,从而提高效率。
2、增加日志记录
在实际应用中,增加日志记录可以帮助我们更好地了解脚本的运行情况。我们可以使用logging库来记录日志信息。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
import time
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def fetch_and_parse_webpage_with_time(url):
try:
start_time = time.time()
response = requests.get(url, timeout=10)
end_time = time.time()
time_taken = end_time - start_time
if response.status_code == 200:
logging.info(f"Successfully fetched the webpage in {time_taken} seconds")
soup = BeautifulSoup(response.text, 'html.parser')
return soup, time_taken
else:
logging.error(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None, time_taken
except requests.exceptions.RequestException as e:
logging.error(f"An error occurred: {e}")
return None, None
url = "https://www.example.com"
soup, time_taken = fetch_and_parse_webpage_with_time(url)
if time_taken is not None:
logging.info(f"Time taken: {time_taken} seconds")
if soup is not None:
logging.info(soup.prettify())
在这个示例中,我们使用logging库记录了各种日志信息,包括成功获取网页的时间、失败的状态码和异常信息等。
3、支持更多HTTP方法
在某些情况下,我们可能需要使用POST、PUT、DELETE等其他HTTP方法来获取或提交数据。我们可以在脚本中增加对更多HTTP方法的支持。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
import time
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def fetch_and_parse_webpage_with_time(url, method='GET', data=None):
try:
start_time = time.time()
if method == 'GET':
response = requests.get(url, timeout=10)
elif method == 'POST':
response = requests.post(url, data=data, timeout=10)
else:
logging.error(f"Unsupported HTTP method: {method}")
return None, None
end_time = time.time()
time_taken = end_time - start_time
if response.status_code == 200:
logging.info(f"Successfully fetched the webpage in {time_taken} seconds")
soup = BeautifulSoup(response.text, 'html.parser')
return soup, time_taken
else:
logging.error(f"Failed to fetch the webpage. Status code: {response.status_code}")
return None, time_taken
except requests.exceptions.RequestException as e:
logging.error(f"An error occurred: {e}")
return None, None
url = "https://www.example.com"
soup, time_taken = fetch_and_parse_webpage_with_time(url, method='POST', data={'key': 'value'})
if time_taken is not None:
logging.info(f"Time taken: {time_taken} seconds")
if soup is not None:
logging.info(soup.prettify())
在这个示例中,我们增加了对POST方法的支持,并可以通过传递method参数来指定使用的HTTP方法。
通过以上方法,我们可以使用Python脚本来获取网页计时,并根据需要进行优化和扩展。无论是单个网页的获取,还是多个网页的并行获取,都可以通过合理的设计和优化来提高效率和可靠性。
相关问答FAQs:
如何在Python中获取网页加载时间?
要获取网页的加载时间,可以使用requests
库配合time
模块。通过记录请求前后的时间戳,可以计算出网页的加载时间。例如:
import requests
import time
start_time = time.time()
response = requests.get('http://example.com')
end_time = time.time()
loading_time = end_time - start_time
print(f"网页加载时间: {loading_time:.2f}秒")
使用Python监控网页加载时间的工具有哪些?
除了手动编写代码,您还可以使用一些库或工具,如Selenium
、BeautifulSoup
和Pyppeteer
。这些工具提供了更为全面的功能,可以模拟浏览器行为,并获取更详细的加载时间统计信息。
如何优化网页以减少加载时间?
优化网页加载时间的策略包括压缩图像、使用内容分发网络(CDN)、减少HTTP请求、优化CSS和JavaScript等。使用工具如Google PageSpeed Insights
可以帮助您识别并改善网页性能,从而有效提升加载速度。
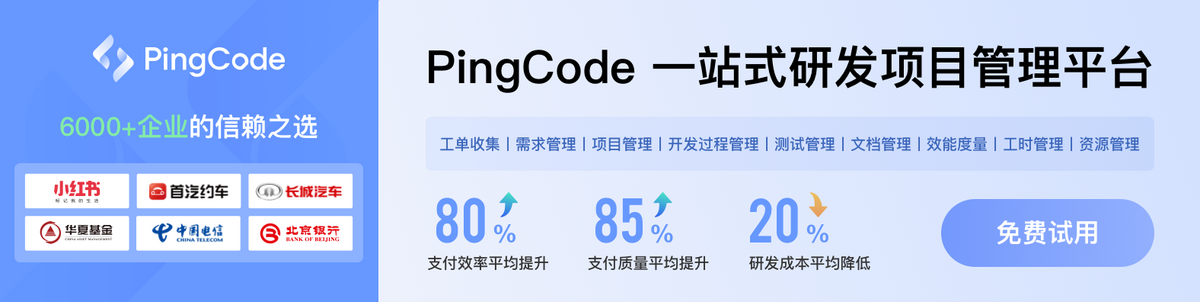