Python加载目标文档的方法有很多种,主要包括使用标准库、使用第三方库、使用网络请求库。其中,标准库包括open()
函数、csv
模块、json
模块等,第三方库包括pandas
、xlrd
、docx
等,网络请求库主要是requests
。例如,使用open()
函数可以轻松读取文本文件,它非常适合处理简单的文本数据。
使用open()函数读取文本文件是一个非常基础且实用的方法。通过open()
函数,你可以轻松地打开文件,读取其内容,并根据需要进行处理。以下是一个简单的例子展示如何使用open()
函数读取一个文本文件:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这个代码段中,open('example.txt', 'r')
打开一个名为“example.txt”的文件,以读取模式('r')打开,并使用with
语句确保文件在读取后自动关闭。file.read()
方法读取文件的全部内容并存储在变量content
中,最后通过print()
函数输出到控制台。
一、使用标准库
1. 使用open()函数
open()
函数是Python内置的文件操作函数之一。通过它,你可以打开、读取和写入文件。以下是一个简单的例子:
# 打开文件
file = open('example.txt', 'r')
读取文件内容
content = file.read()
输出内容
print(content)
关闭文件
file.close()
为了避免手动关闭文件,Python提供了with
语句,它会自动关闭文件:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2. 使用csv模块
csv
模块是Python标准库的一部分,专门用于处理CSV(逗号分隔值)文件。以下是一个简单的例子:
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
你也可以使用DictReader
类来读取CSV文件,并将每一行转换为字典:
import csv
with open('example.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
print(row)
3. 使用json模块
json
模块是Python标准库的一部分,专门用于处理JSON(JavaScript对象表示法)数据。以下是一个简单的例子:
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
二、使用第三方库
1. 使用pandas
pandas
是一个强大的数据分析和操作库。它可以处理各种格式的数据,包括CSV、Excel、SQL等。以下是一个简单的例子:
import pandas as pd
读取CSV文件
df = pd.read_csv('example.csv')
print(df)
读取Excel文件
df = pd.read_excel('example.xlsx')
print(df)
2. 使用xlrd
xlrd
是一个专门用于读取Excel文件的库。以下是一个简单的例子:
import xlrd
打开Excel文件
workbook = xlrd.open_workbook('example.xlsx')
选择工作表
sheet = workbook.sheet_by_index(0)
读取单元格内容
for row in range(sheet.nrows):
print(sheet.row_values(row))
3. 使用docx
docx
是一个专门用于处理Word文档的库。以下是一个简单的例子:
from docx import Document
打开Word文档
doc = Document('example.docx')
读取段落内容
for paragraph in doc.paragraphs:
print(paragraph.text)
三、使用网络请求库
1. 使用requests库
requests
库是一个强大的HTTP请求库,可以用于从网络上获取文档。以下是一个简单的例子:
import requests
发送GET请求
response = requests.get('https://example.com/example.txt')
读取响应内容
content = response.text
print(content)
2. 使用BeautifulSoup
BeautifulSoup
是一个专门用于解析HTML和XML文档的库,通常与requests
库一起使用。以下是一个简单的例子:
import requests
from bs4 import BeautifulSoup
发送GET请求
response = requests.get('https://example.com')
解析HTML文档
soup = BeautifulSoup(response.text, 'html.parser')
提取标题
title = soup.title.text
print(title)
四、使用数据库
有时候,目标文档的数据可能存储在数据库中。Python提供了多种方式来连接和操作数据库。
1. 使用sqlite3
sqlite3
是Python标准库中的一个模块,用于与SQLite数据库进行交互。以下是一个简单的例子:
import sqlite3
连接到SQLite数据库
conn = sqlite3.connect('example.db')
创建游标
cursor = conn.cursor()
执行查询
cursor.execute('SELECT * FROM example_table')
获取查询结果
rows = cursor.fetchall()
for row in rows:
print(row)
关闭连接
conn.close()
2. 使用SQLAlchemy
SQLAlchemy
是一个功能强大的ORM(对象关系映射)库,可以与多种数据库交互。以下是一个简单的例子:
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
创建数据库引擎
engine = create_engine('sqlite:///example.db')
创建会话
Session = sessionmaker(bind=engine)
session = Session()
查询数据库
results = session.execute('SELECT * FROM example_table')
for row in results:
print(row)
五、使用云存储服务
有时候,目标文档可能存储在云端,例如Amazon S3、Google Cloud Storage等。Python提供了多种库来与这些云存储服务进行交互。
1. 使用boto3
boto3
是Amazon Web Services(AWS)的Python SDK,可以用于与Amazon S3进行交互。以下是一个简单的例子:
import boto3
创建S3客户端
s3 = boto3.client('s3')
下载文件
s3.download_file('mybucket', 'example.txt', 'local_example.txt')
读取文件内容
with open('local_example.txt', 'r') as file:
content = file.read()
print(content)
2. 使用google-cloud-storage
google-cloud-storage
是Google Cloud Storage的Python客户端库。以下是一个简单的例子:
from google.cloud import storage
创建客户端
client = storage.Client()
获取桶
bucket = client.get_bucket('mybucket')
下载文件
blob = bucket.blob('example.txt')
blob.download_to_filename('local_example.txt')
读取文件内容
with open('local_example.txt', 'r') as file:
content = file.read()
print(content)
六、处理不同编码的文件
在读取文档时,有时候需要处理不同的文件编码。Python的open()
函数允许你指定文件的编码。以下是一个简单的例子:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
你也可以使用chardet
库来自动检测文件的编码:
import chardet
读取文件的二进制内容
with open('example.txt', 'rb') as file:
raw_data = file.read()
检测文件编码
result = chardet.detect(raw_data)
encoding = result['encoding']
使用检测到的编码读取文件内容
with open('example.txt', 'r', encoding=encoding) as file:
content = file.read()
print(content)
七、处理大文件
在处理大文件时,直接读取整个文件到内存中可能会导致内存不足。为了避免这种情况,你可以按行或按块读取文件。以下是一些例子:
1. 按行读取文件
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
2. 按块读取文件
def read_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'r') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
yield chunk
for chunk in read_in_chunks('example.txt'):
print(chunk)
八、处理二进制文件
有时候,目标文档可能是二进制文件,例如图片、音频、视频等。Python的open()
函数允许你以二进制模式打开文件。以下是一个简单的例子:
# 读取二进制文件
with open('example.png', 'rb') as file:
binary_data = file.read()
print(binary_data)
写入二进制文件
with open('copy_example.png', 'wb') as file:
file.write(binary_data)
九、使用Pathlib模块
pathlib
模块是Python 3.4引入的一个面向对象的文件系统路径操作模块。它提供了更加方便和直观的文件操作方法。以下是一些例子:
1. 读取文件内容
from pathlib import Path
创建Path对象
file_path = Path('example.txt')
读取文件内容
content = file_path.read_text()
print(content)
2. 迭代目录中的文件
from pathlib import Path
创建Path对象
dir_path = Path('.')
迭代目录中的文件
for file_path in dir_path.iterdir():
if file_path.is_file():
print(file_path)
十、总结
在Python中加载目标文档的方法有很多种,包括使用标准库、第三方库、网络请求库、数据库、云存储服务等。根据具体需求选择合适的方法,可以高效地处理不同类型的文档和数据。无论是处理文本文件、CSV文件、JSON文件、Excel文件、Word文档,还是与数据库和云存储服务交互,Python提供了丰富的工具和库,能够满足各种场景的需求。通过灵活运用这些方法,你可以轻松地加载和处理目标文档,从而实现更复杂的数据操作和分析任务。
相关问答FAQs:
如何在Python中打开和读取文档文件?
在Python中打开和读取文档文件可以通过内置的open()
函数实现。该函数允许你指定文件路径和模式(如读取、写入等)。例如,使用with open('document.txt', 'r') as file:
可以安全地打开文档并在读取后自动关闭文件。使用file.read()
可以读取整个文件内容,而file.readlines()
则可以逐行读取文件内容。
Python支持哪些文档格式的加载?
Python支持多种文档格式的加载,包括文本文件(.txt)、CSV文件(.csv)、JSON文件(.json)以及更复杂的格式如Microsoft Word(.docx)和PDF(.pdf)。对于每种格式,通常都有相应的库可供使用,例如pandas
用于CSV和Excel文件,json
模块用于JSON文件,python-docx
用于Word文档,以及PyPDF2
用于PDF文件。
如何处理加载文档时可能出现的错误?
在加载文档时,可能会遇到文件未找到、权限错误或格式不正确等问题。为了处理这些错误,可以使用try...except
语句来捕获异常。例如,使用try:
来尝试打开文件,若出现FileNotFoundError
或PermissionError
等异常,则可以在except
中处理相应的错误信息,确保程序能够优雅地应对这些问题而不崩溃。
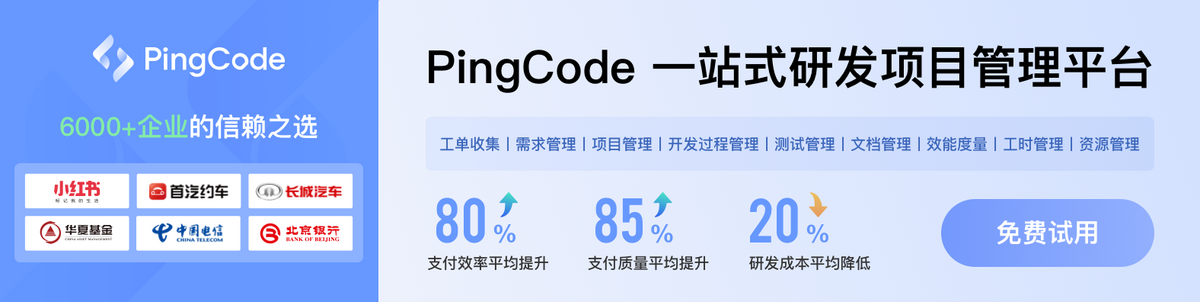