要用Python读取HTML文件,可以使用多种方法,如使用内置的urllib
库、使用requests
库、使用BeautifulSoup
进行HTML解析。其中,BeautifulSoup
是一个非常强大的HTML解析库,它可以让你轻松地提取、导航和修改HTML文档。下面将详细介绍如何使用这些方法读取HTML文件。
使用requests
库是最常见的方式之一,因为它简单且功能强大。requests
库允许你发送HTTP请求,并轻松地获取响应内容。以下是如何使用requests
库读取HTML文件的详细步骤:
首先,你需要安装requests
库,可以使用以下命令:
pip install requests
然后,可以使用以下代码来读取HTML文件:
import requests
url = 'https://www.example.com'
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve HTML content. Status code: {response.status_code}")
这里,requests.get(url)
发送一个GET请求到指定的URL,并返回一个响应对象。response.text
包含了HTML文件的内容。
一、使用urllib
库读取HTML
Python内置的urllib
库也是一个非常强大的工具,特别适合进行简单的HTTP请求。urllib
库包含了几个模块,如urllib.request
、urllib.error
、urllib.parse
和urllib.robotparser
。其中,urllib.request
模块允许你打开和读取URL。
示例代码:
import urllib.request
url = 'https://www.example.com'
with urllib.request.urlopen(url) as response:
html_content = response.read().decode('utf-8')
print(html_content)
这里,urllib.request.urlopen(url)
打开一个URL,并返回一个响应对象。response.read()
读取响应内容,而decode('utf-8')
将字节数据解码为字符串。
二、使用BeautifulSoup
解析HTML
BeautifulSoup
是一个非常强大的HTML解析库,可以让你轻松地提取、导航和修改HTML文档。首先,你需要安装BeautifulSoup
及其依赖库lxml
或html.parser
。
安装命令:
pip install beautifulsoup4 lxml
示例代码:
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'lxml')
# 示例:提取所有的链接
for link in soup.find_all('a'):
print(link.get('href'))
else:
print(f"Failed to retrieve HTML content. Status code: {response.status_code}")
在这个示例中,首先使用requests
库获取HTML内容,然后使用BeautifulSoup
解析HTML。soup.find_all('a')
会找到所有的<a>
标签,并通过link.get('href')
提取每个链接的href
属性。
三、使用lxml
解析HTML
lxml
是另一个强大的HTML解析库,特别适合处理复杂的HTML文档。你可以使用lxml
库来解析HTML,并结合XPath进行高效的元素定位和提取。
安装命令:
pip install lxml
示例代码:
import requests
from lxml import html
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.content
tree = html.fromstring(html_content)
# 示例:提取所有的链接
links = tree.xpath('//a/@href')
for link in links:
print(link)
else:
print(f"Failed to retrieve HTML content. Status code: {response.status_code}")
在这个示例中,首先使用requests
库获取HTML内容,然后使用lxml
库解析HTML。tree.xpath('//a/@href')
会找到所有的<a>
标签,并提取它们的href
属性。
四、使用selenium
库读取动态生成的HTML
有些网页是通过JavaScript动态生成内容的,这时需要使用selenium
库来模拟浏览器行为,获取完整的HTML内容。selenium
库可以自动化浏览器操作,并获取动态生成的HTML。
安装命令:
pip install selenium
你还需要下载相应的浏览器驱动程序,例如ChromeDriver,并将其路径添加到系统环境变量中。
示例代码:
from selenium import webdriver
url = 'https://www.example.com'
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get(url)
获取页面源码
html_content = driver.page_source
print(html_content)
关闭浏览器
driver.quit()
在这个示例中,首先使用webdriver.Chrome
启动一个Chrome浏览器实例,并访问指定的URL。driver.page_source
会获取当前页面的完整HTML内容。
五、使用pyppeteer
库读取动态生成的HTML
pyppeteer
是一个Node.js的puppeteer
库的Python移植版本,它也可以用于处理动态生成的HTML。与selenium
类似,pyppeteer
库可以模拟浏览器行为,并获取完整的HTML内容。
安装命令:
pip install pyppeteer
示例代码:
import asyncio
from pyppeteer import launch
async def get_html(url):
browser = await launch()
page = await browser.newPage()
await page.goto(url)
html_content = await page.content()
print(html_content)
await browser.close()
url = 'https://www.example.com'
asyncio.get_event_loop().run_until_complete(get_html(url))
在这个示例中,首先使用launch
函数启动一个浏览器实例,并访问指定的URL。page.content()
会获取当前页面的完整HTML内容。
六、使用Scrapy
框架进行网页抓取
Scrapy
是一个非常强大的网页抓取框架,适合处理复杂的抓取任务。它内置了很多有用的功能,如请求调度、数据解析和存储等。
安装命令:
pip install scrapy
示例代码:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://www.example.com']
def parse(self, response):
# 提取所有链接
for link in response.css('a::attr(href)').getall():
yield {'link': link}
运行爬虫
from scrapy.crawler import CrawlerProcess
process = CrawlerProcess()
process.crawl(ExampleSpider)
process.start()
在这个示例中,定义了一个名为ExampleSpider
的爬虫类,继承自scrapy.Spider
。start_urls
定义了起始URL,parse
方法用于解析响应内容,并提取所有的链接。
七、使用mechanize
库读取HTML
mechanize
是一个用于模拟浏览器行为的库,可以处理表单提交、重定向和cookie等。它适合处理需要模拟用户交互的网页抓取任务。
安装命令:
pip install mechanize
示例代码:
import mechanize
url = 'https://www.example.com'
br = mechanize.Browser()
br.set_handle_robots(False) # 忽略robots.txt
br.open(url)
html_content = br.response().read().decode('utf-8')
print(html_content)
在这个示例中,首先创建一个mechanize.Browser
实例,并打开指定的URL。br.response().read()
读取响应内容,并将字节数据解码为字符串。
八、使用requests-html
库读取动态生成的HTML
requests-html
是一个非常强大的库,结合了requests
库和pyppeteer
库的功能。它不仅可以处理静态HTML,还可以处理动态生成的HTML。
安装命令:
pip install requests-html
示例代码:
from requests_html import HTMLSession
url = 'https://www.example.com'
session = HTMLSession()
response = session.get(url)
等待JavaScript加载完成
response.html.render()
html_content = response.html.html
print(html_content)
在这个示例中,首先创建一个HTMLSession
实例,并发送一个GET请求到指定的URL。response.html.render()
会等待JavaScript加载完成,并获取完整的HTML内容。
九、使用html5lib
解析HTML
html5lib
是一个完全符合HTML5规范的解析库,它可以将HTML解析为一个DOM树结构,适合处理复杂的HTML文档。
安装命令:
pip install html5lib
示例代码:
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html5lib')
# 示例:提取所有的链接
for link in soup.find_all('a'):
print(link.get('href'))
else:
print(f"Failed to retrieve HTML content. Status code: {response.status_code}")
在这个示例中,首先使用requests
库获取HTML内容,然后使用BeautifulSoup
结合html5lib
解析HTML。soup.find_all('a')
会找到所有的<a>
标签,并通过link.get('href')
提取每个链接的href
属性。
十、使用pyquery
解析HTML
pyquery
是一个类似于jQuery的解析库,它提供了一种非常简洁的方式来解析和操作HTML文档。
安装命令:
pip install pyquery
示例代码:
import requests
from pyquery import PyQuery as pq
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
doc = pq(html_content)
# 示例:提取所有的链接
for link in doc('a'):
print(link.attrib['href'])
else:
print(f"Failed to retrieve HTML content. Status code: {response.status_code}")
在这个示例中,首先使用requests
库获取HTML内容,然后使用pyquery
解析HTML。doc('a')
会找到所有的<a>
标签,并通过link.attrib['href']
提取每个链接的href
属性。
十一、使用html.parser
解析HTML
Python内置的html.parser
模块提供了一种简单的方式来解析HTML文档。它适合处理简单的HTML解析任务。
示例代码:
from html.parser import HTMLParser
class MyHTMLParser(HTMLParser):
def handle_starttag(self, tag, attrs):
if tag == 'a':
for attr in attrs:
if attr[0] == 'href':
print(attr[1])
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
parser = MyHTMLParser()
parser.feed(html_content)
else:
print(f"Failed to retrieve HTML content. Status code: {response.status_code}")
在这个示例中,定义了一个名为MyHTMLParser
的类,继承自HTMLParser
。在handle_starttag
方法中,检查是否为<a>
标签,并提取href
属性。
十二、使用feedparser
库解析RSS和Atom
feedparser
是一个专门用于解析RSS和Atom feed的库。如果你需要从RSS或Atom feed中提取内容,可以使用这个库。
安装命令:
pip install feedparser
示例代码:
import feedparser
url = 'https://www.example.com/rss'
feed = feedparser.parse(url)
for entry in feed.entries:
print(entry.title)
print(entry.link)
在这个示例中,使用feedparser.parse(url)
解析RSS feed,并迭代feed.entries
来提取每个条目的标题和链接。
总结
通过上述方法,可以使用Python读取和解析HTML文件。根据具体需求和HTML文档的复杂程度,可以选择合适的工具和库来完成任务。对于简单的静态HTML解析,可以使用requests
结合BeautifulSoup
或lxml
;对于动态生成的HTML,可以使用selenium
或pyppeteer
;对于复杂的抓取任务,可以使用Scrapy
框架。无论选择哪种方法,都可以根据实际需求灵活应用,确保高效、准确地提取所需信息。
相关问答FAQs:
如何使用Python读取HTML文件中的内容?
可以使用Python的内置库,如open()
函数直接读取HTML文件的内容。如果需要解析和提取特定的HTML元素,推荐使用BeautifulSoup
库。该库提供了强大的解析功能,能够轻松地提取标签、文本和属性。以下是一个简单的示例:
from bs4 import BeautifulSoup
# 读取HTML文件
with open('example.html', 'r', encoding='utf-8') as file:
content = file.read()
# 使用BeautifulSoup解析内容
soup = BeautifulSoup(content, 'html.parser')
print(soup.prettify()) # 格式化输出HTML内容
有哪些Python库可以用来读取和解析HTML?
Python中有多个库可用于读取和解析HTML,包括但不限于:
BeautifulSoup
:用于解析HTML和XML文档,支持多种解析器。lxml
:一个高效的XML和HTML解析库,适合处理大型文件。html.parser
:Python自带的HTML解析器,适合简单的解析任务。requests
:虽然主要用于发送HTTP请求,但也能轻松获取网页内容,配合BeautifulSoup
使用效果更佳。
在读取HTML时,如何处理编码问题?
读取HTML文件时,确保使用正确的编码格式非常重要。常见的编码格式包括UTF-8和ISO-8859-1。使用open()
函数时,可以通过encoding
参数指定编码。例如:
with open('example.html', 'r', encoding='utf-8') as file:
content = file.read()
若不确定文件的编码,可以尝试使用chardet
库自动检测编码。这将有助于避免因编码错误导致的内容读取问题。
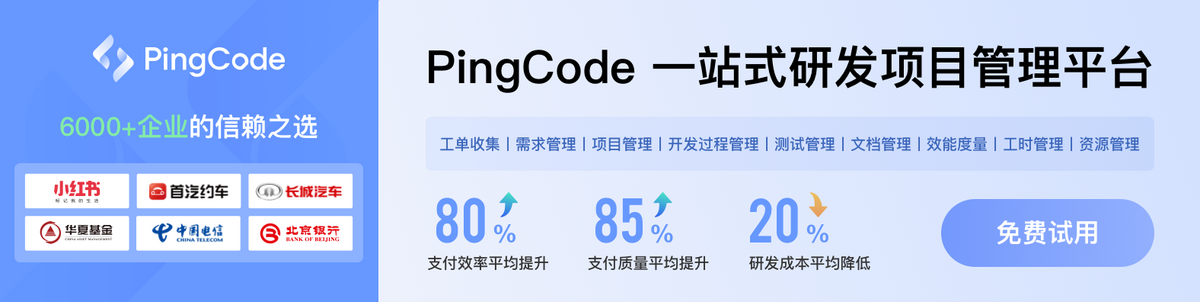