Python与网页交互的方式有多种,如使用requests库、Selenium库、BeautifulSoup库、Scrapy框架、Pyppeteer库等。其中,requests库和BeautifulSoup库常用于静态网页数据抓取,Selenium库和Pyppeteer库则用于与动态网页交互。本文将详细介绍这些方法及其应用场景,尤其是如何使用requests库抓取静态网页数据。
一、REQUESTS库
requests库是一个非常流行的HTTP请求库,用于与网页进行交互。它可以发送HTTP请求,并获取网页的HTML代码。以下是使用requests库的基本步骤:
- 安装requests库:
pip install requests
- 导入requests库:
import requests
- 发送HTTP请求:
response = requests.get('https://example.com')
- 获取网页内容:
html_content = response.text
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print('Failed to retrieve the web page.')
使用requests库可以轻松地从网页上抓取数据,但如果需要解析这些数据,还需要结合BeautifulSoup库。
二、BEAUTIFULSOUP库
BeautifulSoup库是一个用于解析HTML和XML文档的库。它能将复杂的HTML文档转换为一个树形结构,方便进行数据提取。以下是使用BeautifulSoup库解析网页内容的基本步骤:
- 安装BeautifulSoup库:
pip install beautifulsoup4
- 导入BeautifulSoup库:
from bs4 import BeautifulSoup
- 创建BeautifulSoup对象:
soup = BeautifulSoup(html_content, 'html.parser')
- 提取数据:
data = soup.find_all('tag')
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
# 提取所有标题
titles = soup.find_all('h1')
for title in titles:
print(title.text)
else:
print('Failed to retrieve the web page.')
三、SELENIUM库
Selenium库是一个自动化测试工具,可以用于模拟浏览器操作,与动态网页进行交互。以下是使用Selenium库的基本步骤:
- 安装Selenium库:
pip install selenium
- 下载浏览器驱动(如ChromeDriver)
- 导入Selenium库:
from selenium import webdriver
- 创建浏览器对象:
driver = webdriver.Chrome('path/to/chromedriver')
- 访问网页:
driver.get('https://example.com')
- 进行交互操作:
element = driver.find_element_by_id('element_id')
from selenium import webdriver
使用Chrome浏览器
driver = webdriver.Chrome('/path/to/chromedriver')
driver.get('https://example.com')
查找元素并进行交互
element = driver.find_element_by_id('element_id')
element.send_keys('Hello World')
element.submit()
获取网页内容
html_content = driver.page_source
print(html_content)
driver.quit()
四、SCRAPY框架
Scrapy框架是一个强大的爬虫框架,适用于大规模爬取数据。以下是使用Scrapy框架的基本步骤:
- 安装Scrapy框架:
pip install scrapy
- 创建Scrapy项目:
scrapy startproject project_name
- 创建爬虫:
scrapy genspider spider_name 'example.com'
- 编写爬虫代码:在spiders目录下的spider_name.py文件中编写爬虫逻辑
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
titles = response.css('h1::text').getall()
for title in titles:
yield {'title': title}
运行爬虫: scrapy crawl example
五、PYPPETEER库
Pyppeteer库是一个用于控制无头浏览器的库,可以用于与动态网页进行交互。以下是使用Pyppeteer库的基本步骤:
- 安装Pyppeteer库:
pip install pyppeteer
- 导入Pyppeteer库:
from pyppeteer import launch
- 创建浏览器对象:
browser = await launch()
- 创建页面对象:
page = await browser.newPage()
- 访问网页:
await page.goto('https://example.com')
- 获取网页内容:
content = await page.content()
import asyncio
from pyppeteer import launch
async def main():
browser = await launch()
page = await browser.newPage()
await page.goto('https://example.com')
content = await page.content()
print(content)
await browser.close()
asyncio.get_event_loop().run_until_complete(main())
六、总结
以上介绍了Python与网页交互的几种常用方法。requests库和BeautifulSoup库适用于静态网页的数据抓取,Selenium库和Pyppeteer库适用于与动态网页的交互,Scrapy框架则适用于大规模的数据爬取。根据具体需求选择合适的工具,可以提高工作效率,节省时间和精力。
Python强大的库和框架使得与网页交互变得非常简单和高效。无论是简单的静态网页数据抓取,还是复杂的动态网页交互,都能找到合适的解决方案。希望本文能为你提供有价值的参考,帮助你更好地进行网页数据抓取和交互操作。
相关问答FAQs:
如何使用Python抓取网页数据?
使用Python抓取网页数据通常可以使用库如requests
和BeautifulSoup
。requests
用于发送HTTP请求获取网页内容,而BeautifulSoup
则可以解析HTML文档,提取所需的数据。首先,通过requests.get()
获取网页内容,然后利用BeautifulSoup
解析HTML结构,提取特定标签或类名的数据。
Python中如何模拟用户在网页上的操作?
可以使用Selenium
库来模拟用户在网页上的操作。Selenium
提供了一个自动化测试工具,可以控制浏览器的行为,如点击按钮、填写表单等。通过配置WebDriver,用户可以编写脚本来自动化浏览器操作,实现与网页的交互。
在Python中如何处理网页响应的JSON数据?
如果网页返回的是JSON格式的数据,可以使用requests
库的.json()
方法轻松处理。在发送请求后,调用响应对象的.json()
方法即可将JSON数据解析为Python字典或列表,方便后续的数据处理和分析。这对于需要从API获取数据的情况尤其有效。
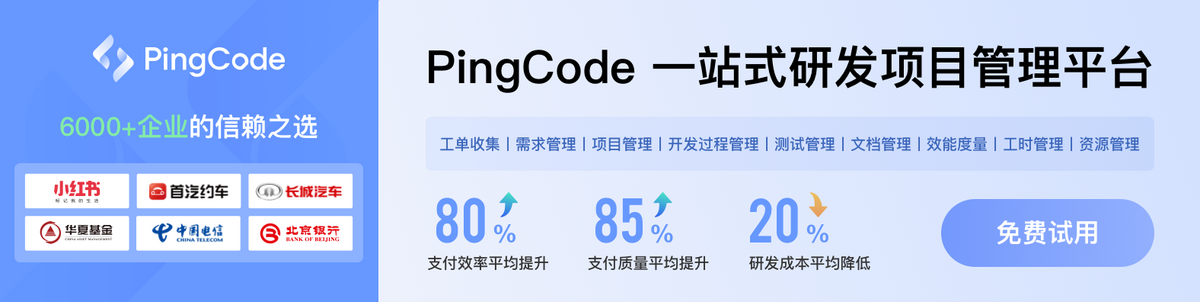