Python判断图片文件的方法包括:使用文件扩展名检查、使用Python内置库检测文件头、通过第三方库如Pillow、使用magic库等方法。接下来我们将详细介绍其中一种方法,即通过第三方库Pillow来判断图片文件。
使用Pillow库检测图片文件
Pillow是Python中一个强大的图像处理库,可以轻松地打开、操作和保存许多不同格式的图像文件。通过Pillow库,我们可以检测一个文件是否是有效的图片文件。
首先,确保你已经安装了Pillow库,可以使用以下命令安装:
pip install pillow
接下来,使用Pillow库判断一个文件是否是图片文件的代码如下:
from PIL import Image
def is_image(file_path):
try:
with Image.open(file_path) as img:
img.verify() # 验证图像文件是否有效
return True
except (IOError, SyntaxError) as e:
return False
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image(file_path):
print(f"{file_path} is a valid image file.")
else:
print(f"{file_path} is not a valid image file.")
在上面的代码中,我们使用Image.open()
函数尝试打开文件,并使用img.verify()
方法验证文件是否是有效的图片文件。如果文件无法打开或者验证失败,将抛出异常,我们捕获异常并返回False
,表示文件不是图片文件。
一、使用文件扩展名检查
判断一个文件是否是图片文件的最简单方法是检查文件的扩展名。常见的图片文件扩展名包括.jpg
、.jpeg
、.png
、.gif
、.bmp
、.tiff
等。虽然这种方法简单,但并不总是可靠,因为文件扩展名可以被随意更改。
import os
def is_image_file(file_path):
image_extensions = ('.jpg', '.jpeg', '.png', '.gif', '.bmp', '.tiff')
return file_path.lower().endswith(image_extensions)
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file(file_path):
print(f"{file_path} is a valid image file based on extension.")
else:
print(f"{file_path} is not a valid image file based on extension.")
二、使用Python内置库检测文件头
文件头(或称魔术数)是文件格式的特征,它位于文件的开头,用于标识文件类型。通过读取文件头的字节数据,可以判断文件是否是图片文件。这种方法比单纯检查扩展名更可靠。
def is_image_file_by_header(file_path):
try:
with open(file_path, 'rb') as file:
header = file.read(10)
if header.startswith(b'\xff\xd8'): # JPEG
return True
elif header.startswith(b'\x89PNG\r\n\x1a\n'): # PNG
return True
elif header.startswith(b'GIF87a') or header.startswith(b'GIF89a'): # GIF
return True
elif header.startswith(b'BM'): # BMP
return True
elif header.startswith(b'II*\x00') or header.startswith(b'MM\x00*'): # TIFF
return True
else:
return False
except IOError:
return False
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file_by_header(file_path):
print(f"{file_path} is a valid image file based on header.")
else:
print(f"{file_path} is not a valid image file based on header.")
三、使用magic库检测文件类型
magic
库是一个用于识别文件类型的库。它基于文件的内容而不是文件扩展名来判断文件类型。首先,确保你已经安装了python-magic
库,可以使用以下命令安装:
pip install python-magic
然后,使用magic
库判断一个文件是否是图片文件的代码如下:
import magic
def is_image_file_by_magic(file_path):
try:
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
return mime_type.startswith('image/')
except IOError:
return False
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file_by_magic(file_path):
print(f"{file_path} is a valid image file based on magic.")
else:
print(f"{file_path} is not a valid image file based on magic.")
四、综合使用多种方法
为了提高判断的准确性,可以综合使用上述多种方法。首先检查文件扩展名,然后使用文件头检查,再使用Pillow库进行验证,最后使用magic库进行确认。以下是综合使用多种方法的代码:
import os
from PIL import Image
import magic
def is_image_file(file_path):
# 检查文件扩展名
image_extensions = ('.jpg', '.jpeg', '.png', '.gif', '.bmp', '.tiff')
if not file_path.lower().endswith(image_extensions):
return False
# 检查文件头
try:
with open(file_path, 'rb') as file:
header = file.read(10)
if not (header.startswith(b'\xff\xd8') or header.startswith(b'\x89PNG\r\n\x1a\n') or
header.startswith(b'GIF87a') or header.startswith(b'GIF89a') or
header.startswith(b'BM') or header.startswith(b'II*\x00') or
header.startswith(b'MM\x00*')):
return False
except IOError:
return False
# 使用Pillow库验证
try:
with Image.open(file_path) as img:
img.verify()
except (IOError, SyntaxError):
return False
# 使用magic库验证
try:
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
if not mime_type.startswith('image/'):
return False
except IOError:
return False
return True
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file(file_path):
print(f"{file_path} is a valid image file.")
else:
print(f"{file_path} is not a valid image file.")
综上所述,通过综合使用文件扩展名检查、文件头检查、Pillow库验证和magic库验证,可以更准确地判断一个文件是否是图片文件。这种方法不仅考虑了文件扩展名,还考虑了文件内容和文件头特征,确保判断的准确性。
五、通过扩展名检测图片文件
判断文件是否是图片文件的一种简单方法是检查文件的扩展名。常见的图片文件扩展名包括:.jpg、.jpeg、.png、.gif、.bmp、.tiff等。虽然这种方法简单,但并不总是可靠,因为文件扩展名可以被随意更改。
def is_image_file_extension(file_path):
image_extensions = ('.jpg', '.jpeg', '.png', '.gif', '.bmp', '.tiff')
return file_path.lower().endswith(image_extensions)
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file_extension(file_path):
print(f"{file_path} is a valid image file based on extension.")
else:
print(f"{file_path} is not a valid image file based on extension.")
六、通过文件头检测图片文件
文件头(或称魔术数)是文件格式的特征,它位于文件的开头,用于标识文件类型。通过读取文件头的字节数据,可以判断文件是否是图片文件。这种方法比单纯检查扩展名更可靠。
def is_image_file_header(file_path):
try:
with open(file_path, 'rb') as file:
header = file.read(10)
if header.startswith(b'\xff\xd8'): # JPEG
return True
elif header.startswith(b'\x89PNG\r\n\x1a\n'): # PNG
return True
elif header.startswith(b'GIF87a') or header.startswith(b'GIF89a'): # GIF
return True
elif header.startswith(b'BM'): # BMP
return True
elif header.startswith(b'II*\x00') or header.startswith(b'MM\x00*'): # TIFF
return True
else:
return False
except IOError:
return False
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file_header(file_path):
print(f"{file_path} is a valid image file based on header.")
else:
print(f"{file_path} is not a valid image file based on header.")
七、通过Pillow库检测图片文件
Pillow是Python中一个强大的图像处理库,可以轻松地打开、操作和保存许多不同格式的图像文件。通过Pillow库,我们可以检测一个文件是否是有效的图片文件。
from PIL import Image
def is_image_file_pillow(file_path):
try:
with Image.open(file_path) as img:
img.verify() # 验证图像文件是否有效
return True
except (IOError, SyntaxError):
return False
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file_pillow(file_path):
print(f"{file_path} is a valid image file based on Pillow.")
else:
print(f"{file_path} is not a valid image file based on Pillow.")
八、通过magic库检测图片文件
magic
库是一个用于识别文件类型的库。它基于文件的内容而不是文件扩展名来判断文件类型。首先,确保你已经安装了python-magic
库,可以使用以下命令安装:
pip install python-magic
然后,使用magic
库判断一个文件是否是图片文件的代码如下:
import magic
def is_image_file_magic(file_path):
try:
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
return mime_type.startswith('image/')
except IOError:
return False
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file_magic(file_path):
print(f"{file_path} is a valid image file based on magic.")
else:
print(f"{file_path} is not a valid image file based on magic.")
九、综合使用多种方法
为了提高判断的准确性,可以综合使用上述多种方法。首先检查文件扩展名,然后使用文件头检查,再使用Pillow库进行验证,最后使用magic库进行确认。以下是综合使用多种方法的代码:
import os
from PIL import Image
import magic
def is_image_file(file_path):
# 检查文件扩展名
image_extensions = ('.jpg', '.jpeg', '.png', '.gif', '.bmp', '.tiff')
if not file_path.lower().endswith(image_extensions):
return False
# 检查文件头
try:
with open(file_path, 'rb') as file:
header = file.read(10)
if not (header.startswith(b'\xff\xd8') or header.startswith(b'\x89PNG\r\n\x1a\n') or
header.startswith(b'GIF87a') or header.startswith(b'GIF89a') or
header.startswith(b'BM') or header.startswith(b'II*\x00') or
header.startswith(b'MM\x00*')):
return False
except IOError:
return False
# 使用Pillow库验证
try:
with Image.open(file_path) as img:
img.verify()
except (IOError, SyntaxError):
return False
# 使用magic库验证
try:
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
if not mime_type.startswith('image/'):
return False
except IOError:
return False
return True
测试代码
file_path = 'path/to/your/image/file.jpg'
if is_image_file(file_path):
print(f"{file_path} is a valid image file.")
else:
print(f"{file_path} is not a valid image file.")
通过综合使用文件扩展名检查、文件头检查、Pillow库验证和magic库验证,可以更准确地判断一个文件是否是图片文件。这种方法不仅考虑了文件扩展名,还考虑了文件内容和文件头特征,确保判断的准确性。
相关问答FAQs:
如何使用Python检测图像文件的格式?
可以使用Python的PIL
(Pillow)库来判断图像文件的格式。通过打开图像文件并查看其格式属性,可以轻松得知文件类型。示例代码如下:
from PIL import Image
def check_image_format(file_path):
try:
with Image.open(file_path) as img:
return img.format
except IOError:
return "不是有效的图像文件"
如何判断图像文件是否损坏?
判断图像文件是否损坏可以通过尝试打开文件并查看是否出现错误来实现。如果文件能够成功打开且没有引发异常,那么文件通常是有效的。使用PIL
库也可以完成这个操作,示例代码如下:
def is_image_corrupted(file_path):
try:
with Image.open(file_path) as img:
img.verify() # 验证图像完整性
return False # 文件未损坏
except (IOError, SyntaxError):
return True # 文件损坏
在Python中,如何获取图像文件的大小和维度?
使用PIL
库不仅可以判断文件格式,还可以获取图像的大小和维度。通过size
属性可以获取图像的宽度和高度。以下是示例代码:
def get_image_size(file_path):
try:
with Image.open(file_path) as img:
return img.size # 返回(宽度,高度)
except IOError:
return "无法读取图像文件"
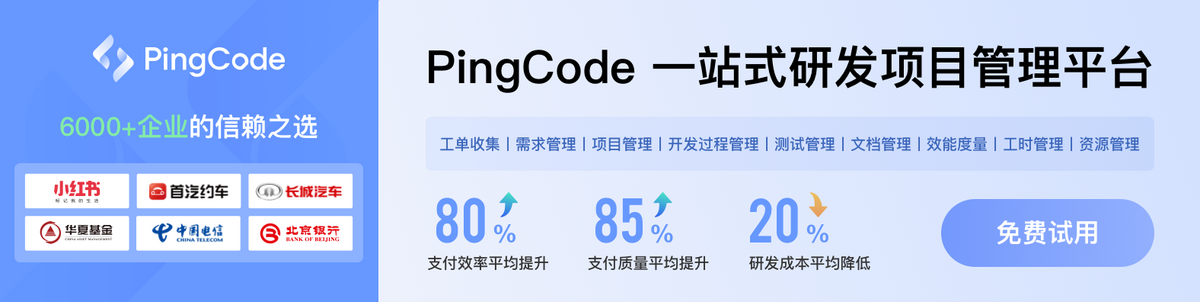