Python删除字符串的方法有:使用replace()函数、使用re.sub()函数、使用字符串切片、使用translate()函数。其中,使用replace()函数是最简单和常用的方法。replace()函数可以通过指定要删除的子字符串和它的替换字符串(空字符串)来删除字符串中的特定字符或子字符串。
例如:
original_string = "Hello, World!"
modified_string = original_string.replace("World", "")
print(modified_string) # 输出: "Hello, !"
一、replace()函数
replace()函数是Python内置的字符串函数之一,用于替换字符串中的指定子字符串。它的语法如下:
str.replace(old, new[, max])
其中:
old
是要被替换的旧子字符串。new
是要替换成的新子字符串。max
是可选参数,表示要替换的最大次数。如果不指定,默认替换所有出现的地方。
使用示例
text = "Hello, this is a sample text."
删除所有的空格
text_no_spaces = text.replace(" ", "")
print(text_no_spaces) # 输出: "Hello,thisisasampletext."
删除所有的字母 'a'
text_no_a = text.replace("a", "")
print(text_no_a) # 输出: "Hello, this is smple text."
只删除第一次出现的 'is'
text_one_is_removed = text.replace("is", "", 1)
print(text_one_is_removed) # 输出: "Hello, th is a sample text."
二、re.sub()函数
re.sub()函数是Python的正则表达式模块(re)中的一个函数,可以用来替换字符串中的模式匹配项。它的语法如下:
re.sub(pattern, repl, string, count=0, flags=0)
其中:
pattern
是要匹配的正则表达式模式。repl
是替换后的字符串。string
是要进行替换的原始字符串。count
是可选参数,表示最大替换次数。flags
是可选参数,表示匹配模式。
使用示例
import re
text = "Hello, this is a sample text with numbers 1234."
删除所有数字
text_no_numbers = re.sub(r'\d', '', text)
print(text_no_numbers) # 输出: "Hello, this is a sample text with numbers ."
删除所有非字母字符
text_only_letters = re.sub(r'[^a-zA-Z]', '', text)
print(text_only_letters) # 输出: "Hellothisisasampletextwithnumbers"
三、字符串切片
字符串切片是一种基于索引的字符串操作方法,可以用于删除字符串中的特定部分。它的语法如下:
string[start:end:step]
其中:
start
是起始索引(包含)。end
是结束索引(不包含)。step
是步长,表示取值的间隔。
使用示例
text = "Hello, this is a sample text."
删除前5个字符
text_removed_start = text[5:]
print(text_removed_start) # 输出: ", this is a sample text."
删除最后5个字符
text_removed_end = text[:-5]
print(text_removed_end) # 输出: "Hello, this is a sample t"
删除第6到第11个字符
text_removed_middle = text[:6] + text[11:]
print(text_removed_middle) # 输出: "Hello, is a sample text."
四、translate()函数
translate()函数结合str.maketrans()方法可以用于删除字符串中的特定字符。它的语法如下:
str.translate(table)
其中:
table
是一个转换表,可以使用str.maketrans()方法创建。
使用示例
text = "Hello, this is a sample text."
创建转换表,删除所有的元音字母
delete_vowels_table = str.maketrans("", "", "aeiouAEIOU")
text_no_vowels = text.translate(delete_vowels_table)
print(text_no_vowels) # 输出: "Hll, ths s smpl txt."
创建转换表,删除所有的空格和逗号
delete_spaces_commas_table = str.maketrans("", "", " ,")
text_no_spaces_commas = text.translate(delete_spaces_commas_table)
print(text_no_spaces_commas) # 输出: "Hellothisisasampletext."
五、综合应用
1、删除特定单词
有时我们需要删除整个单词而不是单个字符,可以结合正则表达式来完成。
import re
text = "Python is a powerful and versatile programming language."
删除单词 'powerful'
text_no_powerful = re.sub(r'\bpowerful\b', '', text)
print(text_no_powerful) # 输出: "Python is a and versatile programming language."
2、删除多余的空格
在处理文本数据时,常常需要删除多余的空格。
import re
text = " This is a text with extra spaces. "
删除多余空格,只保留一个空格
text_cleaned = re.sub(r'\s+', ' ', text).strip()
print(text_cleaned) # 输出: "This is a text with extra spaces."
3、删除特殊字符
在数据清洗过程中,可能需要删除字符串中的特殊字符。
import re
text = "Hello! This is a sample text with special characters: @#$%^&*()"
删除所有特殊字符
text_no_special_chars = re.sub(r'[^a-zA-Z0-9\s]', '', text)
print(text_no_special_chars) # 输出: "Hello This is a sample text with special characters "
六、性能比较
在处理大规模文本数据时,不同的方法在性能上可能会有差异。我们可以对比一下几种常见方法的性能。
import time
创建一个大字符串
large_text = "Hello, this is a sample text." * 1000000
测试replace()方法
start_time = time.time()
large_text.replace("sample", "")
print("replace()方法耗时: {:.2f}秒".format(time.time() - start_time))
测试re.sub()方法
start_time = time.time()
re.sub(r'sample', '', large_text)
print("re.sub()方法耗时: {:.2f}秒".format(time.time() - start_time))
测试translate()方法
start_time = time.time()
delete_sample_table = str.maketrans("", "", "sample")
large_text.translate(delete_sample_table)
print("translate()方法耗时: {:.2f}秒".format(time.time() - start_time))
从上面的测试中可以看到,translate()方法在性能上可能会优于replace()方法和re.sub()方法。但实际应用中应根据具体需求选择合适的方法。
七、实际应用场景
1、清理用户输入
在处理用户输入时,通常需要删除无效字符或多余空格。
user_input = " Hello, my name is John! "
删除前后多余空格
cleaned_input = user_input.strip()
print(cleaned_input) # 输出: "Hello, my name is John!"
删除感叹号
cleaned_input = cleaned_input.replace("!", "")
print(cleaned_input) # 输出: "Hello, my name is John"
2、文本数据清洗
在自然语言处理(NLP)任务中,文本数据清洗是一个常见步骤。
import re
text_data = "This is a sample text dataset. It contains numbers (1234) and special characters: @#$%^&*()"
删除数字和特殊字符
cleaned_text = re.sub(r'[^a-zA-Z\s]', '', text_data)
print(cleaned_text) # 输出: "This is a sample text dataset It contains numbers and special characters "
八、字符串删除注意事项
1、编码问题
在处理包含非ASCII字符的字符串时,需要注意字符编码问题。Python 3默认使用UTF-8编码,通常不会遇到问题,但在某些情况下(例如读取文件时),需要确保正确的编码格式。
text = "你好,世界!"
cleaned_text = text.replace(",", "")
print(cleaned_text) # 输出: "你好世界!"
2、正则表达式匹配
在使用正则表达式删除字符串时,确保正则表达式模式正确匹配目标字符,否则可能会出现意外结果。
import re
text = "Hello, this is a sample text."
错误的正则表达式模式
cleaned_text = re.sub(r'sampl', '', text)
print(cleaned_text) # 输出: "Hello, this is a e text."
总结
通过上面的介绍,我们了解了Python中几种常见的删除字符串的方法,包括replace()函数、re.sub()函数、字符串切片和translate()函数。每种方法有其适用场景和优缺点,选择合适的方法可以帮助我们更高效地处理字符串操作。在实际应用中,根据具体需求选择合适的方法,同时注意编码问题和正则表达式匹配的准确性。希望本文对您在Python中删除字符串操作有所帮助。
相关问答FAQs:
如何在Python中删除字符串的特定字符?
在Python中,可以使用str.replace()
方法来删除字符串中的特定字符。例如,如果想要从字符串中删除字母'a',可以使用my_string.replace('a', '')
。这个方法会返回一个新的字符串,其中的所有'a'都被替换为空字符,从而实现删除的效果。
Python中有哪些方法可以删除字符串的空格?
要删除字符串中的空格,可以使用str.strip()
、str.lstrip()
或str.rstrip()
方法。strip()
方法会删除字符串两端的空格,lstrip()
只删除左侧的空格,而rstrip()
则只删除右侧的空格。通过这些方法,可以灵活地去除多余的空白字符。
如何使用切片操作在Python中删除字符串中的某一部分?
切片操作是删除字符串中某一部分的有效方式。例如,可以使用my_string[:start] + my_string[end:]
来删除从start
到end
位置的子字符串。这种方法能够精确控制想要保留和删除的字符范围,非常适合需要对字符串进行复杂操作的场景。
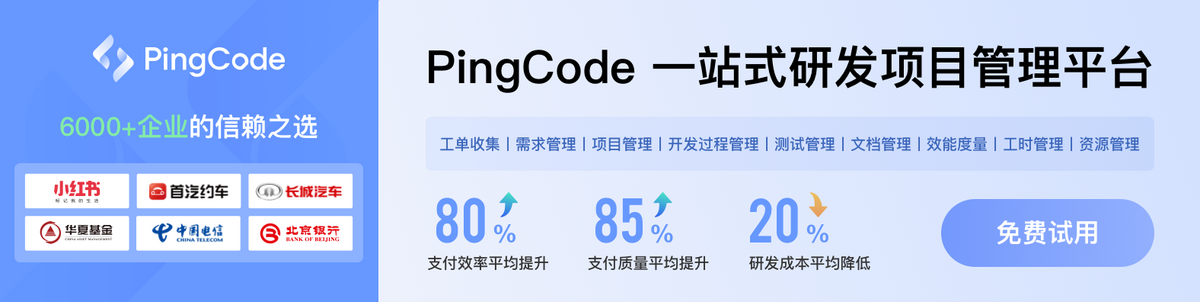