Python可以通过多种方式实现对称处理,包括对称加密、对称矩阵操作和对称递归算法等。常用的方法有使用加密库实现对称加密、利用NumPy进行对称矩阵操作、设计递归函数处理对称结构等。
下面将详细介绍如何使用Python进行对称加密,其他方法在后文中也会详细展开。
对称加密是一种加密技术,其中加密和解密使用相同的密钥。Python中常用的对称加密库是cryptography
。下面是一个使用cryptography
库实现对称加密和解密的例子:
from cryptography.fernet import Fernet
生成密钥
key = Fernet.generate_key()
cipher_suite = Fernet(key)
加密信息
plain_text = b"Hello, this is a secret message."
cipher_text = cipher_suite.encrypt(plain_text)
print(f"Encrypted: {cipher_text}")
解密信息
decrypted_text = cipher_suite.decrypt(cipher_text)
print(f"Decrypted: {decrypted_text}")
在这个例子中,首先生成一个密钥,然后用生成的密钥创建一个Fernet
对象。接着,使用encrypt
方法对明文信息进行加密,最后使用decrypt
方法解密信息。这种方法确保了信息在传输过程中的安全性,因为只有拥有正确密钥的人才能解密信息。
一、对称加密与解密
对称加密是指加密和解密使用相同的密钥,这种方式在数据传输中非常常见,因为它相对高效。Python中可以使用cryptography
库来实现对称加密和解密。
1.1 安装与初始化
首先,安装cryptography
库:
pip install cryptography
然后,在代码中导入所需模块,并生成密钥:
from cryptography.fernet import Fernet
生成密钥
key = Fernet.generate_key()
cipher_suite = Fernet(key)
1.2 加密与解密
生成密钥后,可以使用该密钥对信息进行加密和解密:
# 加密信息
plain_text = b"Hello, this is a secret message."
cipher_text = cipher_suite.encrypt(plain_text)
print(f"Encrypted: {cipher_text}")
解密信息
decrypted_text = cipher_suite.decrypt(cipher_text)
print(f"Decrypted: {decrypted_text}")
在这个例子中,encrypt
方法用于加密明文信息,decrypt
方法用于解密密文信息。这种方法不仅简单,而且有效地保护了数据的安全性。
二、对称矩阵操作
对称矩阵是指一个矩阵与其转置矩阵相等。Python中可以使用NumPy库来创建和操作对称矩阵。
2.1 安装与初始化
首先,安装NumPy库:
pip install numpy
然后,在代码中导入NumPy库:
import numpy as np
2.2 创建对称矩阵
创建一个对称矩阵的方法有很多,可以手动创建,也可以通过算法生成。例如,下面的代码演示了如何创建一个对称矩阵:
# 创建一个随机矩阵
A = np.random.rand(3, 3)
生成对称矩阵
symmetric_matrix = (A + A.T) / 2
print(symmetric_matrix)
在这个例子中,首先创建一个随机矩阵,然后通过将矩阵与其转置矩阵相加,并除以2,生成一个对称矩阵。这种方法确保了生成的矩阵是对称的。
2.3 矩阵操作
创建对称矩阵后,可以进行各种矩阵操作,例如求逆、求特征值等:
# 求矩阵的特征值和特征向量
eigenvalues, eigenvectors = np.linalg.eig(symmetric_matrix)
print(f"Eigenvalues: {eigenvalues}")
print(f"Eigenvectors: {eigenvectors}")
求矩阵的逆
inverse_matrix = np.linalg.inv(symmetric_matrix)
print(f"Inverse Matrix: {inverse_matrix}")
通过这些操作,可以深入了解对称矩阵的性质和应用。
三、对称递归算法
递归是一种在函数中调用自身的方法。对称递归算法是一种特殊的递归算法,其递归调用具有对称性。例如,汉诺塔问题和回文检查就是对称递归算法的典型例子。
3.1 汉诺塔问题
汉诺塔问题是一种经典的递归问题,要求将一堆圆盘从一个柱子移动到另一个柱子,同时满足特定的规则。下面是一个实现汉诺塔问题的Python代码:
def hanoi(n, source, target, auxiliary):
if n == 1:
print(f"Move disk 1 from {source} to {target}")
return
hanoi(n-1, source, auxiliary, target)
print(f"Move disk {n} from {source} to {target}")
hanoi(n-1, auxiliary, target, source)
调用汉诺塔函数
hanoi(3, 'A', 'C', 'B')
在这个例子中,hanoi
函数通过递归调用自身解决汉诺塔问题。这种递归调用具有对称性,因为每次调用都会将问题分解为更小的子问题。
3.2 回文检查
回文是指一个字符串从左到右和从右到左读都是一样的。下面是一个检查字符串是否为回文的递归算法:
def is_palindrome(s):
if len(s) <= 1:
return True
if s[0] != s[-1]:
return False
return is_palindrome(s[1:-1])
调用回文检查函数
print(is_palindrome("racecar")) # True
print(is_palindrome("hello")) # False
在这个例子中,is_palindrome
函数通过递归调用自身检查字符串是否为回文。这种递归调用具有对称性,因为每次调用都会检查字符串的首尾字符。
四、对称图形绘制
对称图形是一种具有对称性质的图形,可以通过编程绘制。Python中可以使用Turtle库绘制对称图形。
4.1 安装与初始化
Turtle库是Python内置的绘图库,无需安装。可以直接在代码中导入Turtle库:
import turtle
4.2 绘制对称图形
下面是一个绘制对称图形的示例代码:
def draw_symmetric_pattern():
turtle.speed(0)
turtle.bgcolor("black")
colors = ["red", "yellow", "blue", "green", "orange", "purple"]
for i in range(360):
turtle.pencolor(colors[i % 6])
turtle.forward(i)
turtle.left(59)
turtle.done()
调用绘制函数
draw_symmetric_pattern()
在这个例子中,draw_symmetric_pattern
函数通过循环和颜色变化绘制对称图形。这种方法可以生成美丽的对称图案。
五、对称数据结构
数据结构是指数据组织、管理和存储的方式。对称数据结构是一种具有对称性质的数据结构,例如二叉树和图。
5.1 二叉树
二叉树是一种每个节点最多有两个子节点的数据结构。下面是一个实现对称二叉树的Python代码:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def is_symmetric(root):
if not root:
return True
return is_mirror(root.left, root.right)
def is_mirror(left, right):
if not left and not right:
return True
if not left or not right:
return False
return (left.value == right.value) and is_mirror(left.left, right.right) and is_mirror(left.right, right.left)
创建对称二叉树
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(2)
root.left.left = TreeNode(3)
root.left.right = TreeNode(4)
root.right.left = TreeNode(4)
root.right.right = TreeNode(3)
调用对称检查函数
print(is_symmetric(root)) # True
在这个例子中,is_symmetric
函数通过递归调用is_mirror
函数检查二叉树是否对称。这种方法可以有效地判断二叉树的对称性。
5.2 图
图是一种由节点和边组成的数据结构。对称图是一种具有对称性质的图。下面是一个实现对称图的Python代码:
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u in self.graph:
self.graph[u].append(v)
else:
self.graph[u] = [v]
if v in self.graph:
self.graph[v].append(u)
else:
self.graph[v] = [u]
def is_symmetric(graph):
for node in graph.graph:
for neighbor in graph.graph[node]:
if node not in graph.graph[neighbor]:
return False
return True
创建对称图
g = Graph()
g.add_edge(1, 2)
g.add_edge(2, 3)
g.add_edge(3, 1)
调用对称检查函数
print(is_symmetric(g)) # True
在这个例子中,is_symmetric
函数通过检查每个节点及其邻居节点是否对称,判断图是否对称。这种方法可以有效地判断图的对称性。
六、对称字符串操作
字符串操作是编程中的常见任务。对称字符串操作是指在字符串操作过程中保持对称性。例如,字符串的反转和回文检查。
6.1 字符串反转
字符串反转是一种简单的对称字符串操作。下面是一个实现字符串反转的Python代码:
def reverse_string(s):
return s[::-1]
调用字符串反转函数
print(reverse_string("hello")) # "olleh"
在这个例子中,reverse_string
函数通过切片操作反转字符串。这种方法简单高效。
6.2 回文检查
回文检查是一种常见的对称字符串操作。下面是一个实现回文检查的Python代码:
def is_palindrome(s):
return s == s[::-1]
调用回文检查函数
print(is_palindrome("racecar")) # True
print(is_palindrome("hello")) # False
在这个例子中,is_palindrome
函数通过比较字符串与其反转字符串,检查字符串是否为回文。这种方法简单直接。
七、对称分形图形
分形图形是一种具有自相似性质的图形。对称分形图形是一种特殊的分形图形,其自相似结构具有对称性。Python中可以使用Matplotlib库绘制对称分形图形。
7.1 安装与初始化
首先,安装Matplotlib库:
pip install matplotlib
然后,在代码中导入Matplotlib库:
import matplotlib.pyplot as plt
import numpy as np
7.2 绘制对称分形图形
下面是一个绘制对称分形图形的示例代码:
def mandelbrot(c, max_iter):
z = c
for n in range(max_iter):
if abs(z) > 2:
return n
z = z*z + c
return max_iter
def mandelbrot_set(xmin, xmax, ymin, ymax, width, height, max_iter):
r1 = np.linspace(xmin, xmax, width)
r2 = np.linspace(ymin, ymax, height)
n3 = np.empty((width,height))
for i in range(width):
for j in range(height):
n3[i,j] = mandelbrot(r1[i] + 1j*r2[j], max_iter)
return (r1, r2, n3)
def display(xmin,xmax,ymin,ymax,width,height,max_iter):
dpi = 80
img_width = dpi * width
img_height = dpi * height
fig, ax = plt.subplots(figsize=(width, height), dpi=dpi)
X, Y, Z = mandelbrot_set(xmin, xmax, ymin, ymax, img_width, img_height, max_iter)
ax.imshow(Z.T, origin='lower', extent=[xmin, xmax, ymin, ymax], cmap='hot')
plt.show()
调用绘制函数
display(-2.0, 1.0, -1.5, 1.5, 10, 10, 256)
在这个例子中,mandelbrot
函数计算Mandelbrot集合的值,mandelbrot_set
函数生成Mandelbrot集合的图像数据,display
函数使用Matplotlib库绘制Mandelbrot集合的图像。这种方法可以生成美丽的对称分形图案。
八、对称图像处理
图像处理是计算机视觉中的重要任务。对称图像处理是一种特殊的图像处理技术,其处理结果具有对称性。例如,图像的对称翻转和对称滤波。
8.1 图像对称翻转
图像对称翻转是一种常见的图像处理操作。下面是一个实现图像对称翻转的Python代码:
from PIL import Image
def flip_image(image_path, output_path):
image = Image.open(image_path)
flipped_image = image.transpose(Image.FLIP_LEFT_RIGHT)
flipped_image.save(output_path)
调用图像对称翻转函数
flip_image("input.jpg", "output.jpg")
在这个例子中,flip_image
函数使用PIL库对图像进行左右翻转。这种方法简单高效。
8.2 图像对称滤波
图像对称滤波是一种特殊的滤波技术,其滤波结果具有对称性。下面是一个实现图像对称滤波的Python代码:
import cv2
import numpy as np
def symmetric_filter(image_path, output_path):
image = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
kernel = np.array([[1, 2, 1], [2, 4, 2], [1, 2, 1]], dtype=np.float32) / 16
filtered_image = cv2.filter2D(image, -1, kernel)
cv2.imwrite(output_path, filtered_image)
调用图像对称滤波函数
symmetric_filter("input.jpg", "output.jpg")
在这个例子中,symmetric_filter
函数使用OpenCV库对图像进行对称滤波。这种方法可以有效地增强图像的对称性。
九、对称信号处理
信号处理是现代通信系统中的重要任务。对称信号处理是一种特殊的信号处理技术,其处理结果具有对称性。例如,对称滤波和对称傅里叶变换。
9.1 对称滤波
对称滤波是一种常见的信号处理操作。下面是一个实现对称滤波的Python代码:
import numpy as np
from scipy.signal import convolve
def symmetric_filter(signal, kernel):
return convolve(signal, kernel, mode='same')
调用对称滤波函数
signal = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
kernel = np.array([1, 2, 1]) / 4
filtered_signal = symmetric_filter(signal, kernel)
print(filtered_signal)
在这个例子中,symmetric_filter
函数使用SciPy库对信号进行对称滤波。这种方法可以有效地平滑信号。
9.2 对称傅里叶变换
傅里叶变换是一种将信号从时域转换到频域的数学变换。对称傅里叶变换是一种特殊的傅里叶变换,其结果具有对称性。下面是一个实现对称傅里叶变换的Python代码:
import numpy as np
import matplotlib.pyplot as plt
def symmetric_fft(signal):
fft_result = np.fft
相关问答FAQs:
如何在Python中实现对称图形的绘制?
在Python中,可以使用多个库来绘制对称图形。例如,使用matplotlib
库可以绘制各种图形。你可以通过定义对称轴并在其两侧绘制相应的图形来实现对称效果。通过设置图形的坐标系,你可以轻松绘制出对称的形状,比如正方形、圆形或多边形。
Python中有哪些库可以用于对称性检测?
在Python中,OpenCV
和scikit-image
是常用的图像处理库,可以用于对称性检测。通过这些库,你可以分析图像的结构,识别出对称的部分,例如使用轮廓检测和特征匹配的方法。结合图像变换技术,可以有效地判断图像的对称性。
如何在Python中生成对称的随机数序列?
生成对称的随机数序列可以使用Python的random
库。你可以生成一组随机数,然后通过简单的数学变换(例如对称轴的翻转)来创建对称的数值。例如,如果生成了一个序列,可以通过反转序列的方式来获得对称的效果。这样可以在数据分析和模拟中使用对称性。
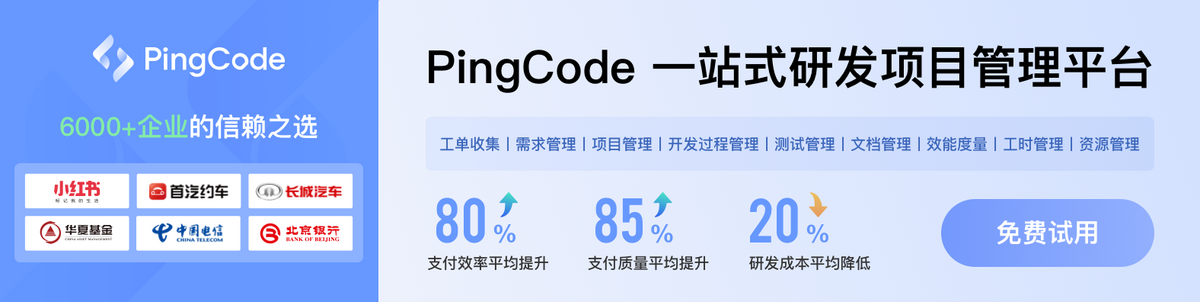