Python提取文本特征的方法有:词袋模型(Bag of Words)、TF-IDF(Term Frequency-Inverse Document Frequency)、词向量(Word Embeddings)、主题模型(Topic Modeling)、N-gram模型(N-gram Model)。 其中,词袋模型是一种简单而有效的文本表示方法,通过统计文本中每个词出现的次数来表示文本特征,适用于多数常见的文本分类任务。
一、词袋模型(Bag of Words)
词袋模型(Bag of Words)是一种常见的文本表示方法,它通过统计文本中每个词出现的频率来表示文本特征。词袋模型简单且直观,常用于文本分类和信息检索任务。
1.1 词袋模型的基本概念
词袋模型的基本思想是将文本表示为一个词的集合(即“词袋”),忽略词的顺序。具体做法是:
- 创建一个词汇表,其中包含所有文本中出现的不同词。
- 对于每个文本,统计每个词在该文本中出现的次数,生成一个词频向量。
1.2 词袋模型的实现
在Python中,可以使用scikit-learn
库中的CountVectorizer
类来实现词袋模型。以下是一个示例代码:
from sklearn.feature_extraction.text import CountVectorizer
示例文本数据
corpus = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
创建CountVectorizer对象
vectorizer = CountVectorizer()
拟合模型并转换文本数据为词频向量
X = vectorizer.fit_transform(corpus)
输出词汇表
print("Vocabulary:", vectorizer.vocabulary_)
输出词频向量
print("Word Frequency Vectors:\n", X.toarray())
二、TF-IDF(Term Frequency-Inverse Document Frequency)
TF-IDF是一种常用的文本特征提取方法,用于衡量一个词在文档中的重要性。它结合了词频(Term Frequency)和逆文档频率(Inverse Document Frequency),在一定程度上减少了常见词对文本表示的影响。
2.1 TF-IDF的基本概念
- 词频(TF):表示词在文档中出现的频率,计算方法为某个词在文档中出现的次数除以文档中的总词数。
- 逆文档频率(IDF):表示词在整个语料库中的稀有程度,计算方法为语料库中文档总数除以包含该词的文档数,然后取对数。
TF-IDF值是词频和逆文档频率的乘积,用来衡量词的重要性。
2.2 TF-IDF的实现
在Python中,可以使用scikit-learn
库中的TfidfVectorizer
类来实现TF-IDF。以下是一个示例代码:
from sklearn.feature_extraction.text import TfidfVectorizer
示例文本数据
corpus = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
创建TfidfVectorizer对象
vectorizer = TfidfVectorizer()
拟合模型并转换文本数据为TF-IDF向量
X = vectorizer.fit_transform(corpus)
输出词汇表
print("Vocabulary:", vectorizer.vocabulary_)
输出TF-IDF向量
print("TF-IDF Vectors:\n", X.toarray())
三、词向量(Word Embeddings)
词向量是一种将词表示为低维向量的技术,能够捕捉词之间的语义关系。常用的词向量模型包括Word2Vec、GloVe和FastText。
3.1 Word2Vec
Word2Vec是一种基于神经网络的词向量模型,能够学习到词的语义关系。它有两种训练方法:CBOW(Continuous Bag of Words)和Skip-gram。
在Python中,可以使用gensim
库来训练和使用Word2Vec模型。以下是一个示例代码:
from gensim.models import Word2Vec
示例文本数据
sentences = [
["this", "is", "the", "first", "document"],
["this", "document", "is", "the", "second", "document"],
["and", "this", "is", "the", "third", "one"],
["is", "this", "the", "first", "document"]
]
训练Word2Vec模型
model = Word2Vec(sentences, vector_size=100, window=5, min_count=1, workers=4)
获取词向量
word_vector = model.wv['document']
print("Word Vector for 'document':\n", word_vector)
3.2 GloVe
GloVe(Global Vectors for Word Representation)是一种基于全局共现矩阵的词向量模型,能够捕捉词之间的全局共现信息。
在Python中,可以使用gensim
库来加载预训练的GloVe词向量。以下是一个示例代码:
import gensim.downloader as api
加载预训练的GloVe词向量
glove_vectors = api.load("glove-wiki-gigaword-100")
获取词向量
word_vector = glove_vectors['document']
print("Word Vector for 'document':\n", word_vector)
四、主题模型(Topic Modeling)
主题模型是一种用于从文本数据中发现隐藏主题的技术,常用的主题模型包括LDA(Latent Dirichlet Allocation)和LSA(Latent Semantic Analysis)。
4.1 LDA(Latent Dirichlet Allocation)
LDA是一种生成模型,用于发现文档中的隐藏主题。它假设每个文档都是若干主题的混合,而每个主题是若干词的混合。
在Python中,可以使用gensim
库来实现LDA模型。以下是一个示例代码:
from gensim import corpora
from gensim.models import LdaModel
示例文本数据
documents = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
预处理文本数据
texts = [[word for word in document.lower().split()] for document in documents]
创建词汇表
dictionary = corpora.Dictionary(texts)
将文本数据转换为文档-词频矩阵
corpus = [dictionary.doc2bow(text) for text in texts]
训练LDA模型
lda_model = LdaModel(corpus, num_topics=2, id2word=dictionary, passes=15)
输出主题
topics = lda_model.print_topics(num_words=4)
for topic in topics:
print(topic)
4.2 LSA(Latent Semantic Analysis)
LSA是一种基于奇异值分解(SVD)的主题模型,用于发现文档和词之间的潜在语义结构。
在Python中,可以使用scikit-learn
库中的TruncatedSVD
类来实现LSA模型。以下是一个示例代码:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.decomposition import TruncatedSVD
示例文本数据
corpus = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
创建TF-IDF向量
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(corpus)
训练LSA模型
lsa = TruncatedSVD(n_components=2)
X_lsa = lsa.fit_transform(X)
输出LSA结果
print("LSA Components:\n", lsa.components_)
五、N-gram模型(N-gram Model)
N-gram模型是一种基于词序列的文本表示方法,通过将文本分割成连续的N个词的序列来捕捉词的顺序信息。
5.1 N-gram模型的基本概念
N-gram模型的基本思想是将文本分割成连续的N个词的序列。例如,对于文本“this is a test”,可以生成如下N-gram序列:
- Unigram(1-gram):["this", "is", "a", "test"]
- Bigram(2-gram):["this is", "is a", "a test"]
- Trigram(3-gram):["this is a", "is a test"]
5.2 N-gram模型的实现
在Python中,可以使用CountVectorizer
或TfidfVectorizer
类,并通过设置ngram_range
参数来实现N-gram模型。以下是一个示例代码:
from sklearn.feature_extraction.text import CountVectorizer
示例文本数据
corpus = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
创建CountVectorizer对象,设置ngram_range参数
vectorizer = CountVectorizer(ngram_range=(1, 2))
拟合模型并转换文本数据为N-gram词频向量
X = vectorizer.fit_transform(corpus)
输出词汇表
print("Vocabulary:", vectorizer.vocabulary_)
输出N-gram词频向量
print("N-gram Word Frequency Vectors:\n", X.toarray())
结论
文本特征提取是自然语言处理中的关键步骤,不同的方法适用于不同的任务。词袋模型和TF-IDF简单且高效,适用于多数文本分类任务;词向量能够捕捉词的语义关系,适用于更复杂的NLP任务;主题模型能够发现文档中的隐藏主题,适用于文本聚类和主题分析;N-gram模型能够捕捉词的顺序信息,适用于序列标注任务。选择合适的方法能够提高文本处理的效果。
相关问答FAQs:
如何使用Python提取文本特征?
在Python中,提取文本特征通常可以通过自然语言处理(NLP)库来实现,如NLTK、spaCy或Scikit-learn。首先,可以使用分词技术将文本分割成单个词或词组。接着,常用的方法包括TF-IDF(词频-逆文档频率)、词袋模型和Word2Vec等。这些方法可以帮助将文本数据转化为数值形式,便于机器学习模型进行训练和分析。
使用哪些库可以方便地进行文本特征提取?
多种Python库可以用来提取文本特征,其中最常用的包括NLTK、spaCy、Scikit-learn和Gensim。NLTK提供了丰富的文本处理工具,spaCy则以其高效的文本分析而闻名。Scikit-learn专注于机器学习,提供了TF-IDF等特征提取方法,而Gensim则擅长于处理主题建模和Word2Vec等任务。
在提取文本特征时,如何选择合适的方法?
选择合适的特征提取方法主要取决于具体任务的需求。例如,如果目标是进行文本分类,TF-IDF或词袋模型可能更为合适;而如果需要理解词语之间的语义关系,Word2Vec或BERT等深度学习模型可能更有效。考虑数据的规模、复杂性以及模型的需求,将有助于做出更合理的选择。
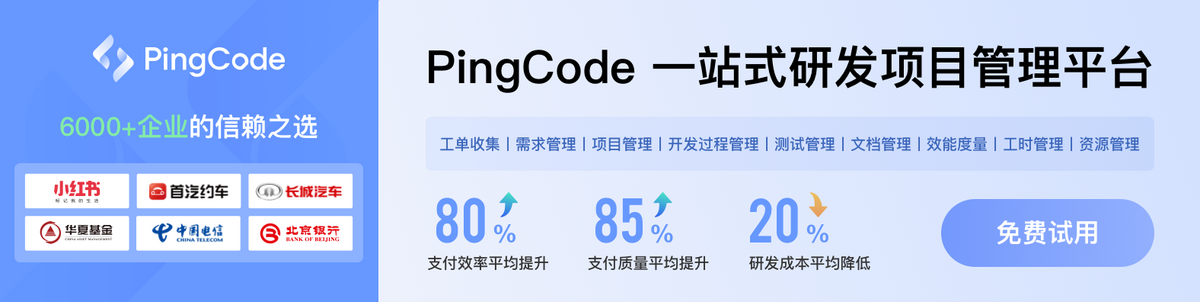