在Python中创建对象的方法包括使用类构造函数、通过类继承创建子类对象、使用工厂函数等。使用类构造函数、通过类继承创建子类对象、使用工厂函数是创建对象的常见方法。下面将详细描述如何使用类构造函数来创建对象。
使用类构造函数创建对象
类构造函数是定义类时的一个特殊方法(__init__
),它在创建对象时自动被调用,用于初始化对象的属性。以下是一个简单的示例:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
print(f"Car: {self.year} {self.make} {self.model}")
创建对象
my_car = Car('Toyota', 'Corolla', 2020)
my_car.display_info()
在这个示例中,定义了一个名为Car
的类,并在其构造函数__init__
中初始化了三个属性:make
、model
和year
。创建Car
类的对象时,需要传递这些属性的值。然后,可以调用display_info
方法来显示对象的信息。
正文内容
一、使用类构造函数
类构造函数是创建对象的基础方法。它可以帮助我们在类实例化时初始化对象的属性。以下详细介绍如何使用类构造函数创建复杂对象。
class Person:
def __init__(self, name, age, gender):
self.name = name
self.age = age
self.gender = gender
def introduce(self):
print(f"Hi, I'm {self.name}, a {self.age}-year-old {self.gender}.")
创建对象
person1 = Person('Alice', 28, 'female')
person1.introduce()
在这个例子中,我们创建了一个Person
类,并在__init__
方法中初始化了name
、age
和gender
三个属性。创建对象时,需要传递这些属性的初始值。然后,可以调用introduce
方法来输出个人介绍。
使用类构造函数的优势在于,它使对象的初始化过程变得直观且易于管理。当类的属性较多时,可以通过构造函数一次性传递所有必要的参数,从而避免后续逐一设置属性的繁琐操作。
二、通过类继承创建子类对象
类继承是面向对象编程中的一个重要概念,通过继承,可以创建新的类(子类),并且这些子类可以继承父类的属性和方法。继承不仅可以减少代码的冗余,还可以增强代码的可维护性和可扩展性。
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclass must implement this method")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
def speak(self):
print(f"{self.name} says Woof! I am a {self.breed}")
class Cat(Animal):
def __init__(self, name, color):
super().__init__(name)
self.color = color
def speak(self):
print(f"{self.name} says Meow! I am a {self.color} cat")
创建子类对象
dog = Dog('Buddy', 'Golden Retriever')
cat = Cat('Whiskers', 'black')
dog.speak()
cat.speak()
在这个示例中,定义了一个基类Animal
,并在其子类Dog
和Cat
中重写了speak
方法。通过调用super().__init__(name)
,子类可以继承并初始化父类的属性。创建子类对象时,可以分别传递子类特有的属性值。
通过类继承创建子类对象的优势在于,子类可以继承并扩展父类的功能,从而实现代码的复用和扩展。例如,在上面的示例中,Dog
和Cat
类都继承了Animal
类的name
属性,并各自实现了特有的speak
方法。
三、使用工厂函数
工厂函数是一种设计模式,用于创建对象的函数。它可以根据输入参数的不同,创建并返回不同类型的对象。工厂函数的主要优点是,它使对象创建过程更加灵活和可定制。
class Shape:
def draw(self):
raise NotImplementedError("Subclass must implement this method")
class Circle(Shape):
def draw(self):
print("Drawing a circle")
class Square(Shape):
def draw(self):
print("Drawing a square")
class Triangle(Shape):
def draw(self):
print("Drawing a triangle")
def shape_factory(shape_type):
if shape_type == 'circle':
return Circle()
elif shape_type == 'square':
return Square()
elif shape_type == 'triangle':
return Triangle()
else:
raise ValueError(f"Unknown shape type: {shape_type}")
使用工厂函数创建对象
shape1 = shape_factory('circle')
shape2 = shape_factory('square')
shape3 = shape_factory('triangle')
shape1.draw()
shape2.draw()
shape3.draw()
在这个示例中,定义了一个基类Shape
,并在其子类Circle
、Square
和Triangle
中实现了draw
方法。工厂函数shape_factory
根据输入参数shape_type
的不同,创建并返回相应的形状对象。
使用工厂函数的优势在于,它使对象的创建过程更加灵活和可定制。工厂函数可以根据需要动态地创建不同类型的对象,而无需在客户端代码中显式调用类构造函数。
四、使用元类
元类(metaclass)是用于创建类的“类”。在Python中,类也是对象,而元类就是创建这些类的类。通过使用元类,可以在类创建过程中进行一些自定义操作。
class Meta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=Meta):
def __init__(self, value):
self.value = value
def show_value(self):
print(f"Value: {self.value}")
创建对象
obj = MyClass(10)
obj.show_value()
在这个示例中,定义了一个元类Meta
,并在__new__
方法中添加了创建类时的自定义操作。MyClass
类使用Meta
作为其元类,因此在创建MyClass
类时,会打印一条消息。创建MyClass
类的对象时,可以正常初始化和使用其方法。
使用元类的优势在于,它使类的创建过程更加灵活和可定制。通过元类,可以在类创建过程中添加自定义操作,从而实现一些高级功能。
五、使用数据类(dataclass)
数据类(dataclass)是Python 3.7引入的新特性,用于简化类定义过程。数据类通过装饰器@dataclass
来定义,并自动生成初始化方法、比较方法等。
from dataclasses import dataclass
@dataclass
class Point:
x: int
y: int
def move(self, dx: int, dy: int):
self.x += dx
self.y += dy
创建对象
point = Point(10, 20)
print(point)
point.move(5, -10)
print(point)
在这个示例中,使用@dataclass
装饰器定义了一个Point
类,并自动生成了__init__
和__repr__
方法。创建对象时,可以直接传递属性值,并使用生成的方法。
使用数据类的优势在于,它简化了类定义过程,减少了样板代码的编写。数据类自动生成的初始化方法和比较方法,使得类定义更加简洁和易于维护。
六、使用命名元组(namedtuple)
命名元组(namedtuple)是Python标准库中的一个类工厂函数,用于创建具有命名字段的不可变对象。命名元组可以像普通元组一样使用,但具有更高的可读性和更好的结构化数据表示。
from collections import namedtuple
定义命名元组
Person = namedtuple('Person', ['name', 'age', 'gender'])
创建对象
person = Person(name='Bob', age=30, gender='male')
print(person)
print(person.name, person.age, person.gender)
在这个示例中,使用namedtuple
定义了一个Person
类,并通过命名字段创建对象。命名元组提供了元组的所有特性,同时增加了字段名的可读性。
使用命名元组的优势在于,它提供了一种简单且高效的方式来定义不可变对象。命名元组的字段名使得代码更具可读性和可维护性。
七、使用类型提示(type hint)
类型提示是一种用于声明变量、参数和返回值类型的语法。类型提示不会改变代码的运行行为,但可以提供额外的信息供类型检查器和IDE使用,以提高代码的可读性和可靠性。
class Employee:
def __init__(self, name: str, id: int, department: str):
self.name = name
self.id = id
self.department = department
def get_details(self) -> str:
return f"Employee: {self.name}, ID: {self.id}, Department: {self.department}"
创建对象
employee = Employee('John Doe', 123, 'Engineering')
print(employee.get_details())
在这个示例中,使用类型提示声明了Employee
类的属性类型和方法返回类型。类型提示可以帮助开发者理解代码的预期输入输出类型,并在开发过程中提供类型检查和自动补全功能。
使用类型提示的优势在于,它提高了代码的可读性和可靠性。类型提示可以帮助开发者在编写和维护代码时避免类型错误,并提供更好的开发体验。
八、使用单例模式
单例模式是一种设计模式,确保一个类只有一个实例,并提供全局访问点。单例模式通常用于需要全局唯一实例的场景,如配置管理器、日志记录器等。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self, value):
self.value = value
创建对象
singleton1 = Singleton('First Instance')
singleton2 = Singleton('Second Instance')
print(singleton1.value)
print(singleton2.value)
print(singleton1 is singleton2)
在这个示例中,使用__new__
方法实现了单例模式。在创建对象时,如果实例不存在,则创建一个新实例;如果实例已存在,则返回已有实例。无论创建多少次对象,始终只有一个实例。
使用单例模式的优势在于,它确保一个类只有一个实例,并提供全局访问点。单例模式适用于需要全局唯一实例的场景,避免了重复创建实例带来的资源浪费和状态不一致问题。
九、使用对象池
对象池是一种设计模式,用于重用对象而不是每次都创建新对象。对象池通常用于频繁创建和销毁对象的场景,以提高性能和资源利用率。
class ObjectPool:
def __init__(self, create_func, max_size=10):
self._create_func = create_func
self._pool = []
self._max_size = max_size
def get(self):
if self._pool:
return self._pool.pop()
return self._create_func()
def release(self, obj):
if len(self._pool) < self._max_size:
self._pool.append(obj)
创建对象池
def create_connection():
return {'connection': 'db_connection'}
pool = ObjectPool(create_connection)
获取和释放对象
conn1 = pool.get()
print(conn1)
pool.release(conn1)
conn2 = pool.get()
print(conn2)
在这个示例中,定义了一个ObjectPool
类,用于管理对象的创建和重用。对象池通过get
方法获取对象,通过release
方法释放对象,从而实现对象的重用。
使用对象池的优势在于,它提高了性能和资源利用率。对象池适用于频繁创建和销毁对象的场景,减少了对象创建和销毁带来的开销。
十、使用组合模式
组合模式是一种设计模式,用于将对象组合成树形结构,以表示“部分-整体”的层次结构。组合模式使得客户端可以以一致的方式处理单个对象和对象组合。
class Component:
def operation(self):
raise NotImplementedError("Subclass must implement this method")
class Leaf(Component):
def operation(self):
print("Leaf operation")
class Composite(Component):
def __init__(self):
self._children = []
def add(self, component):
self._children.append(component)
def remove(self, component):
self._children.remove(component)
def operation(self):
print("Composite operation")
for child in self._children:
child.operation()
创建对象
leaf1 = Leaf()
leaf2 = Leaf()
composite = Composite()
composite.add(leaf1)
composite.add(leaf2)
composite.operation()
在这个示例中,定义了一个Component
基类,并在其子类Leaf
和Composite
中实现了operation
方法。Composite
类可以包含多个子组件,并在operation
方法中依次调用它们的operation
方法。
使用组合模式的优势在于,它使得客户端可以以一致的方式处理单个对象和对象组合。组合模式适用于需要表示“部分-整体”层次结构的场景,如图形界面、组织结构等。
十一、使用策略模式
策略模式是一种设计模式,用于定义一系列算法,将每个算法封装起来,并使它们可以相互替换。策略模式使得算法可以在不影响客户端的情况下发生变化。
class Strategy:
def execute(self):
raise NotImplementedError("Subclass must implement this method")
class ConcreteStrategyA(Strategy):
def execute(self):
print("Executing strategy A")
class ConcreteStrategyB(Strategy):
def execute(self):
print("Executing strategy B")
class Context:
def __init__(self, strategy: Strategy):
self._strategy = strategy
def set_strategy(self, strategy: Strategy):
self._strategy = strategy
def execute_strategy(self):
self._strategy.execute()
创建对象
context = Context(ConcreteStrategyA())
context.execute_strategy()
context.set_strategy(ConcreteStrategyB())
context.execute_strategy()
在这个示例中,定义了一个Strategy
基类,并在其子类ConcreteStrategyA
和ConcreteStrategyB
中实现了execute
方法。Context
类使用Strategy
对象,并通过execute_strategy
方法执行当前策略。
使用策略模式的优势在于,它使得算法可以在不影响客户端的情况下发生变化。策略模式适用于需要动态选择和切换算法的场景,如排序算法、加密算法等。
十二、使用观察者模式
观察者模式是一种设计模式,用于定义对象间的一对多依赖关系,使得当一个对象状态发生改变时,其依赖者能够自动收到通知并更新。观察者模式通常用于事件处理系统。
class Observer:
def update(self, message):
raise NotImplementedError("Subclass must implement this method")
class ConcreteObserver(Observer):
def update(self, message):
print(f"Received message: {message}")
class Subject:
def __init__(self):
self._observers = []
def add_observer(self, observer: Observer):
self._observers.append(observer)
def remove_observer(self, observer: Observer):
self._observers.remove(observer)
def notify_observers(self, message):
for observer in self._observers:
observer.update(message)
创建对象
subject = Subject()
observer1 = ConcreteObserver()
observer2 = ConcreteObserver()
subject.add_observer(observer1)
subject.add_observer(observer2)
subject.notify_observers("Hello, Observers!")
在这个示例中,定义了一个Observer
基类,并在其子类ConcreteObserver
中实现了update
方法。Subject
类管理观察者列表,并通过notify_observers
方法通知所有观察者。
使用观察者模式的优势在于,它使得对象间的依赖关系变得松散耦合,便于扩展和维护。
相关问答FAQs:
如何在Python中定义一个类以便创建对象?
在Python中,定义一个类是创建对象的第一步。你可以使用class
关键字来定义一个类,类中可以包含属性和方法。例如:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return "Woof!"
这个例子中,我们创建了一个Dog
类,包含初始化方法和一个叫的行为。通过调用这个类,我们就可以创建多个对象。
对象创建后如何访问其属性和方法?
一旦你定义了一个类并创建了对象,就可以通过对象名来访问其属性和方法。例如:
my_dog = Dog("Buddy", 3)
print(my_dog.name) # 输出:Buddy
print(my_dog.bark()) # 输出:Woof!
通过my_dog.name
可以访问对象的name
属性,而my_dog.bark()
则调用了该对象的bark
方法。
在Python中,可以创建多个对象吗?
当然可以。在Python中,你可以根据同一个类创建多个对象,每个对象都有独立的属性。例如:
dog1 = Dog("Bella", 2)
dog2 = Dog("Charlie", 4)
print(dog1.name) # 输出:Bella
print(dog2.age) # 输出:4
每个对象都可以拥有不同的属性值,完全独立于其他对象。这种特性使得Python非常适合面向对象的编程。
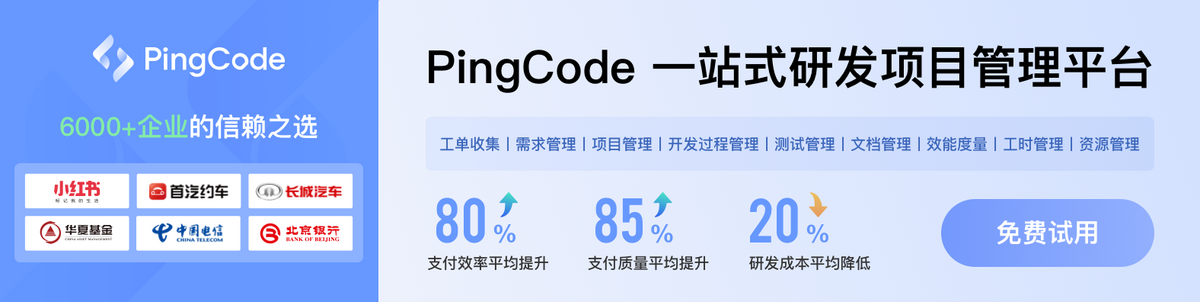