在Python中,合并多个plot的方法包括使用subplot、组合多个plot对象、使用GridSpec进行更复杂的布局等。其中,使用subplot是最常见和简单的方法之一。下面将详细展开其中一种方法——使用subplot:
使用subplot合并图形时,可以在一个画布上绘制多个子图,通过plt.subplot()
函数来实现。这个函数的参数指定了图形的行列数量以及当前的子图位置。例如,plt.subplot(2, 1, 1)
表示在一个包含2行1列的布局中的第一个子图上绘图。通过这种方式,我们可以方便地在同一个画布上绘制多个图形。下面是一个具体的代码示例:
import matplotlib.pyplot as plt
import numpy as np
数据准备
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
创建一个图形窗口
plt.figure(figsize=(10, 8))
第一个子图
plt.subplot(2, 1, 1) # 2行1列的布局中的第一个子图
plt.plot(x, y1, label='sin(x)')
plt.title('Sin(x)')
plt.legend()
第二个子图
plt.subplot(2, 1, 2) # 2行1列的布局中的第二个子图
plt.plot(x, y2, label='cos(x)')
plt.title('Cos(x)')
plt.legend()
显示图形
plt.tight_layout() # 调整子图间距
plt.show()
在这个例子中,我们使用了plt.subplot()
来创建两个子图,并分别绘制了sin(x)
和cos(x)
的图形。通过调整plt.figure()
的参数,还可以设置图形窗口的大小,以便更好地显示多个子图。
接下来,我们将更详细地探讨其他方法和技巧,以便在Python中合并和管理多个plot。
一、使用subplot
1、基本用法
使用subplot
可以在一个图形窗口内创建多个子图。例如,可以创建一个2×2的网格布局,并在每个子图中绘制不同的图形。
import matplotlib.pyplot as plt
import numpy as np
创建数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
创建一个图形窗口
plt.figure(figsize=(12, 10))
第一个子图
plt.subplot(2, 2, 1) # 2行2列的布局中的第一个子图
plt.plot(x, y1, 'r-', label='sin(x)')
plt.title('Sin(x)')
plt.legend()
第二个子图
plt.subplot(2, 2, 2) # 2行2列的布局中的第二个子图
plt.plot(x, y2, 'g-', label='cos(x)')
plt.title('Cos(x)')
plt.legend()
第三个子图
plt.subplot(2, 2, 3) # 2行2列的布局中的第三个子图
plt.plot(x, y3, 'b-', label='tan(x)')
plt.title('Tan(x)')
plt.legend()
第四个子图
plt.subplot(2, 2, 4) # 2行2列的布局中的第四个子图
plt.plot(x, y4, 'm-', label='exp(x)')
plt.title('Exp(x)')
plt.legend()
调整子图间距
plt.tight_layout()
显示图形
plt.show()
2、共享坐标轴
在某些情况下,您可能希望子图共享相同的坐标轴,以便更容易进行比较。可以使用sharex
和sharey
参数来实现这一点。
fig, axs = plt.subplots(2, 2, figsize=(12, 10), sharex=True, sharey=True)
第一个子图
axs[0, 0].plot(x, y1, 'r-', label='sin(x)')
axs[0, 0].set_title('Sin(x)')
axs[0, 0].legend()
第二个子图
axs[0, 1].plot(x, y2, 'g-', label='cos(x)')
axs[0, 1].set_title('Cos(x)')
axs[0, 1].legend()
第三个子图
axs[1, 0].plot(x, y3, 'b-', label='tan(x)')
axs[1, 0].set_title('Tan(x)')
axs[1, 0].legend()
第四个子图
axs[1, 1].plot(x, y4, 'm-', label='exp(x)')
axs[1, 1].set_title('Exp(x)')
axs[1, 1].legend()
调整子图间距
plt.tight_layout()
显示图形
plt.show()
通过设置sharex=True
和sharey=True
,所有子图将共享相同的x轴和y轴。这对于需要在相同尺度上比较多个图形的数据特别有用。
二、使用组合多个plot对象
1、基本组合方法
除了使用subplot外,还可以通过组合多个plot对象来合并多个图形。例如,可以在同一个图形上叠加多条线。
import matplotlib.pyplot as plt
import numpy as np
数据准备
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
创建一个图形窗口
plt.figure(figsize=(10, 6))
绘制多条线在同一个图形上
plt.plot(x, y1, label='sin(x)')
plt.plot(x, y2, label='cos(x)')
plt.plot(x, y3, label='tan(x)')
添加标题和图例
plt.title('Multiple Plots on the Same Axes')
plt.legend()
显示图形
plt.show()
2、使用不同的绘图样式
在同一个图形上绘制多条线时,可以使用不同的绘图样式来区分它们。Matplotlib提供了多种绘图样式和颜色选项。
import matplotlib.pyplot as plt
import numpy as np
数据准备
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
创建一个图形窗口
plt.figure(figsize=(10, 6))
绘制多条线并使用不同的样式和颜色
plt.plot(x, y1, 'r--', label='sin(x)') # 红色虚线
plt.plot(x, y2, 'g-.', label='cos(x)') # 绿色点划线
plt.plot(x, y3, 'b:', label='tan(x)') # 蓝色点线
添加标题和图例
plt.title('Multiple Plots with Different Styles')
plt.legend()
显示图形
plt.show()
通过使用不同的线型、颜色和标记,可以使图形更加清晰易读。
三、使用GridSpec进行更复杂的布局
1、基本用法
GridSpec是Matplotlib中的一个模块,允许创建更复杂和灵活的布局。可以通过指定行和列的数量以及每个子图所占的网格单元来创建复杂的布局。
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
import numpy as np
数据准备
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
创建一个图形窗口
plt.figure(figsize=(12, 10))
创建GridSpec对象
gs = gridspec.GridSpec(3, 3)
第一个子图
ax1 = plt.subplot(gs[0, :]) # 第0行,所有列
ax1.plot(x, y1, label='sin(x)')
ax1.set_title('Sin(x)')
ax1.legend()
第二个子图
ax2 = plt.subplot(gs[1, :-1]) # 第1行,前2列
ax2.plot(x, y2, label='cos(x)')
ax2.set_title('Cos(x)')
ax2.legend()
第三个子图
ax3 = plt.subplot(gs[1:, -1]) # 第1行和第2行,最后1列
ax3.plot(x, y3, label='tan(x)')
ax3.set_title('Tan(x)')
ax3.legend()
第四个子图
ax4 = plt.subplot(gs[2, 0]) # 第2行,第一列
ax4.plot(x, y4, label='exp(x)')
ax4.set_title('Exp(x)')
ax4.legend()
调整子图间距
plt.tight_layout()
显示图形
plt.show()
通过使用GridSpec,可以创建具有不同大小和位置的子图,从而实现更复杂的布局。
2、嵌套子图
有时,您可能需要在一个子图内嵌套其他子图。可以使用GridSpec和add_subplot
方法来实现这一点。
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
import numpy as np
数据准备
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
创建一个图形窗口
plt.figure(figsize=(12, 10))
创建GridSpec对象
gs0 = gridspec.GridSpec(1, 2)
第一个子图(包含嵌套子图)
gs00 = gridspec.GridSpecFromSubplotSpec(3, 1, subplot_spec=gs0[0])
ax1 = plt.subplot(gs00[0])
ax1.plot(x, y1, label='sin(x)')
ax1.set_title('Sin(x)')
ax1.legend()
ax2 = plt.subplot(gs00[1])
ax2.plot(x, y2, label='cos(x)')
ax2.set_title('Cos(x)')
ax2.legend()
ax3 = plt.subplot(gs00[2])
ax3.plot(x, y3, label='tan(x)')
ax3.set_title('Tan(x)')
ax3.legend()
第二个子图
ax4 = plt.subplot(gs0[1])
ax4.plot(x, y4, label='exp(x)')
ax4.set_title('Exp(x)')
ax4.legend()
调整子图间距
plt.tight_layout()
显示图形
plt.show()
通过这种方式,可以在一个图形内创建嵌套子图,从而实现更复杂的布局和展示效果。
四、使用seaborn和pandas进行多图合并
1、使用seaborn进行多图合并
Seaborn是基于Matplotlib的高级绘图库,提供了更简洁的接口和更美观的默认设置。可以使用seaborn的FacetGrid
来合并多个图形。
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
创建数据
x = np.linspace(0, 10, 100)
data = pd.DataFrame({
'x': np.tile(x, 3),
'y': np.concatenate([np.sin(x), np.cos(x), np.tan(x)]),
'function': ['sin'] * 100 + ['cos'] * 100 + ['tan'] * 100
})
使用FacetGrid
g = sns.FacetGrid(data, col='function')
g.map(plt.plot, 'x', 'y')
显示图形
plt.show()
2、使用pandas进行多图合并
Pandas的DataFrame对象也提供了方便的方法来绘制和合并多个图形。可以使用plot
方法并设置subplots=True
来实现。
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
创建数据
x = np.linspace(0, 10, 100)
df = pd.DataFrame({
'x': x,
'sin': np.sin(x),
'cos': np.cos(x),
'tan': np.tan(x)
})
使用pandas进行多图合并
df.set_index('x').plot(subplots=True, layout=(2, 2), figsize=(12, 10), title='Multiple Plots using Pandas')
调整子图间距
plt.tight_layout()
显示图形
plt.show()
通过使用seaborn和pandas,可以更加方便地进行数据可视化和多个图形的合并。
五、使用plotly进行交互式图形合并
1、基本用法
Plotly是一个强大的交互式绘图库,可以创建交互式的图形和仪表盘。可以使用make_subplots
来创建多个子图,并使用add_trace
方法将图形添加到指定的子图中。
import plotly.graph_objects as go
from plotly.subplots import make_subplots
import numpy as np
创建数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
创建子图
fig = make_subplots(rows=2, cols=2, subplot_titles=('Sin(x)', 'Cos(x)', 'Tan(x)', 'Exp(x)'))
添加图形到子图
fig.add_trace(go.Scatter(x=x, y=y1, name='sin(x)'), row=1, col=1)
fig.add_trace(go.Scatter(x=x, y=y2, name='cos(x)'), row=1, col=2)
fig.add_trace(go.Scatter(x=x, y=y3, name='tan(x)'), row=2, col=1)
fig.add_trace(go.Scatter(x=x, y=y4, name='exp(x)'), row=2, col=2)
更新布局
fig.update_layout(title='Multiple Plots using Plotly', showlegend=False)
显示图形
fig.show()
2、创建交互式仪表盘
Plotly还可以与Dash结合使用,创建交互式的仪表盘。下面是一个简单的示例,展示如何创建一个包含多个图形的交互式仪表盘。
import dash
import dash_core_components as dcc
import dash_html_components as html
import plotly.graph_objects as go
import numpy as np
创建数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
初始化Dash应用
app = dash.Dash(__name__)
创建图形
fig = make_subplots(rows=2, cols=2, subplot_titles=('Sin(x)', 'Cos(x)', 'Tan(x)', 'Exp(x)'))
fig.add_trace(go.Scatter(x=x, y=y1, name='sin(x)'), row=1, col=1)
fig.add_trace(go.Scatter(x=x, y=y2, name='cos(x)'), row=1, col=2)
fig.add_trace(go.Scatter(x=x, y=y3, name='tan(x)'), row=2, col=1)
fig.add_trace(go.Scatter(x=x, y=y4, name='exp(x)'), row=2, col=2)
fig.update_layout(title='Multiple Plots using Plotly and Dash', showlegend=False)
创建布局
app.layout = html.Div([
html.H1('Interactive Dashboard'),
dcc.Graph(figure=fig)
])
运行应用
if __name__ == '__main__':
app.run_server(debug=True)
通过使用Plotly和Dash,可以创建强大的交互式图形和仪表盘,方便用户进行数据探索和分析。
六、总结
在Python中,合并多个plot的方法有很多,主要包括使用subplot、组合多个plot对象、使用GridSpec进行更复杂的布局,以及使用seaborn、pandas和plotly进行多图合并。每种方法都有其独特的优势和适用场景,可以根据实际需求选择合适的方法。
1、使用subplot
subplot是最常见和简单的方法,通过在一个画布上创建多个子图,可以方便地进行图形合并和布局调整。
2、组合多个plot对象
通过在同一个图形上叠加多条线,可以快速实现图形的合并和展示。使用不同的绘图样式可以使图形更加清晰。
3、使用GridSpec进行更复杂的布局
GridSpec允许创建更加复杂和灵活的布局,通过指定行列数量和每个子图所占的网格单元,可以实现更复杂
相关问答FAQs:
如何在Python中合并多个绘图?
在Python中,可以使用Matplotlib库来合并多个绘图。通过使用subplot
函数或GridSpec
模块,可以在同一图形窗口中创建多个子图,从而实现合并绘图的效果。此外,还可以通过figure
和add_subplot
方法来更灵活地控制每个子图的布局和大小。
是否可以在同一图中绘制不同类型的图形?
是的,Python的Matplotlib库允许在同一个图中绘制不同类型的图形。例如,可以将线图与散点图结合在一起,或者在柱状图上叠加折线图。这种方式可以通过在同一个Axes对象中调用不同的绘图方法来实现,使得数据的展示更为丰富和直观。
如何调整合并图形中的子图间距和大小?
在合并多个图形时,可以使用subplots_adjust
函数来调整子图之间的间距。通过设置参数如left
, right
, top
, bottom
, wspace
和hspace
,可以精确控制子图的布局。此外,在创建子图时,还可以设置每个子图的大小,以确保它们在视觉上的和谐与平衡。
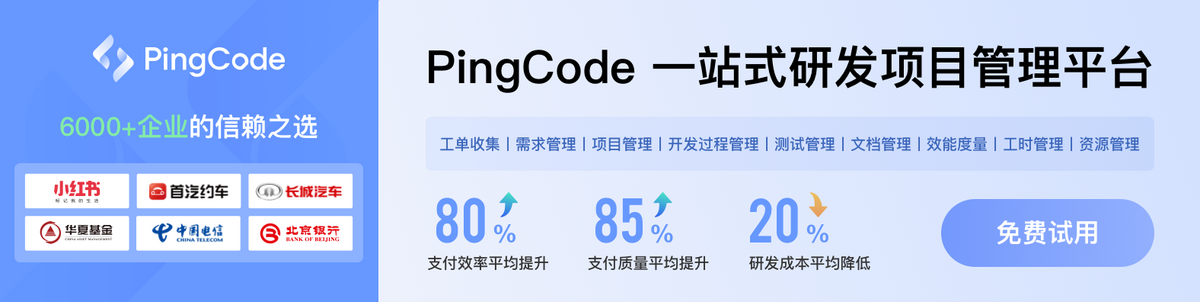