Python调用多线程的方法有Threading模块、concurrent.futures模块、Queue模块,其中Threading模块是最常用的。
一、Threading模块
Threading模块是Python标准库中的一个模块,专门用于多线程编程。它提供了Thread类,可以方便地创建和管理线程。
1. 创建和启动线程
在Threading模块中,可以通过创建Thread类的实例来创建线程。Thread类的构造函数接受两个主要参数:target和args。target参数指定要在线程中执行的函数,args参数是传递给该函数的参数。
import threading
def my_function(arg1, arg2):
print(f"Argument 1: {arg1}, Argument 2: {arg2}")
创建线程
thread = threading.Thread(target=my_function, args=(10, 20))
启动线程
thread.start()
等待线程执行完毕
thread.join()
在上述示例中,我们创建了一个线程,并将my_function函数传递给它。然后,通过调用start方法启动线程。最后,使用join方法等待线程执行完毕。
2. 使用继承创建线程
除了直接创建Thread对象外,还可以通过继承Thread类来创建线程。这种方法使代码更加面向对象。
import threading
class MyThread(threading.Thread):
def __init__(self, arg1, arg2):
super().__init__()
self.arg1 = arg1
self.arg2 = arg2
def run(self):
print(f"Argument 1: {self.arg1}, Argument 2: {self.arg2}")
创建线程实例
thread = MyThread(10, 20)
启动线程
thread.start()
等待线程执行完毕
thread.join()
二、concurrent.futures模块
concurrent.futures模块提供了更高级的接口来管理线程和进程。它提供了ThreadPoolExecutor类,用于创建和管理线程池。
1. 使用ThreadPoolExecutor
ThreadPoolExecutor类提供了一个线程池,可以方便地提交多个任务并获取其结果。
from concurrent.futures import ThreadPoolExecutor
def my_function(arg):
return arg * 2
创建线程池
with ThreadPoolExecutor(max_workers=4) as executor:
# 提交任务
future = executor.submit(my_function, 10)
# 获取结果
result = future.result()
print(result)
在上述示例中,我们创建了一个ThreadPoolExecutor对象,并将最大线程数设置为4。然后,使用submit方法提交任务,并使用result方法获取结果。
2. 使用map方法
ThreadPoolExecutor还提供了map方法,可以方便地将同一个函数应用到多个输入上,并返回结果。
from concurrent.futures import ThreadPoolExecutor
def my_function(arg):
return arg * 2
创建线程池
with ThreadPoolExecutor(max_workers=4) as executor:
results = executor.map(my_function, [1, 2, 3, 4, 5])
for result in results:
print(result)
三、Queue模块
Queue模块提供了线程安全的队列类,可以方便地在线程之间传递数据。常用的队列类包括Queue、LifoQueue和PriorityQueue。
1. 使用Queue类
Queue类表示先进先出(FIFO)队列。可以使用put方法将数据放入队列,使用get方法从队列中取出数据。
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f"Produced: {i}")
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed: {item}")
创建队列
q = queue.Queue()
创建生产者线程
producer_thread = threading.Thread(target=producer, args=(q,))
创建消费者线程
consumer_thread = threading.Thread(target=consumer, args=(q,))
启动线程
producer_thread.start()
consumer_thread.start()
等待生产者线程结束
producer_thread.join()
发送结束信号
q.put(None)
等待消费者线程结束
consumer_thread.join()
在上述示例中,我们创建了一个队列,并分别创建了生产者线程和消费者线程。生产者线程将数据放入队列,消费者线程从队列中取出数据并处理。
2. 使用LifoQueue类
LifoQueue类表示后进先出(LIFO)队列。使用方法与Queue类类似。
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f"Produced: {i}")
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed: {item}")
创建LIFO队列
q = queue.LifoQueue()
创建生产者线程
producer_thread = threading.Thread(target=producer, args=(q,))
创建消费者线程
consumer_thread = threading.Thread(target=consumer, args=(q,))
启动线程
producer_thread.start()
consumer_thread.start()
等待生产者线程结束
producer_thread.join()
发送结束信号
q.put(None)
等待消费者线程结束
consumer_thread.join()
在上述示例中,我们将Queue类替换为LifoQueue类,其余代码保持不变。
3. 使用PriorityQueue类
PriorityQueue类表示优先级队列,可以按照优先级顺序处理数据。可以通过传递元组(优先级,数据)来指定优先级。
import threading
import queue
def producer(q):
for i in range(5):
q.put((5 - i, i))
print(f"Produced: {i}")
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed: {item[1]}")
创建优先级队列
q = queue.PriorityQueue()
创建生产者线程
producer_thread = threading.Thread(target=producer, args=(q,))
创建消费者线程
consumer_thread = threading.Thread(target=consumer, args=(q,))
启动线程
producer_thread.start()
consumer_thread.start()
等待生产者线程结束
producer_thread.join()
发送结束信号
q.put(None)
等待消费者线程结束
consumer_thread.join()
在上述示例中,我们使用PriorityQueue类创建了优先级队列,并将数据作为元组(优先级,数据)放入队列。
四、线程同步
在多线程编程中,可能会遇到多个线程同时访问共享资源的问题。为了避免数据竞争和不一致,可以使用线程同步机制。Python提供了多种线程同步机制,包括锁(Lock)、条件变量(Condition)、事件(Event)和信号量(Semaphore)。
1. 使用锁(Lock)
锁是一种最简单的同步机制,可以确保在同一时间只有一个线程访问共享资源。
import threading
lock = threading.Lock()
shared_resource = 0
def increment():
global shared_resource
with lock:
shared_resource += 1
print(shared_resource)
threads = []
for _ in range(5):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在上述示例中,我们使用锁确保在同一时间只有一个线程访问shared_resource变量。
2. 使用条件变量(Condition)
条件变量是一种高级的同步机制,可以在某个条件成立时通知一个或多个线程。
import threading
condition = threading.Condition()
shared_resource = 0
def increment():
global shared_resource
with condition:
shared_resource += 1
print(shared_resource)
condition.notify_all()
def wait_for_threshold(threshold):
with condition:
while shared_resource < threshold:
condition.wait()
print(f"Threshold reached: {shared_resource}")
increment_thread = threading.Thread(target=increment)
wait_thread = threading.Thread(target=wait_for_threshold, args=(5,))
wait_thread.start()
increment_thread.start()
increment_thread.join()
wait_thread.join()
在上述示例中,wait_for_threshold函数会等待shared_resource达到指定的阈值,然后打印消息。
3. 使用事件(Event)
事件是一种简单的同步机制,可以在线程之间发送信号。
import threading
event = threading.Event()
def wait_for_event():
print("Waiting for event to be set")
event.wait()
print("Event set")
def set_event():
print("Setting event")
event.set()
wait_thread = threading.Thread(target=wait_for_event)
set_thread = threading.Thread(target=set_event)
wait_thread.start()
set_thread.start()
wait_thread.join()
set_thread.join()
在上述示例中,wait_for_event函数会等待事件被设置,然后打印消息。
4. 使用信号量(Semaphore)
信号量是一种复杂的同步机制,可以控制对共享资源的访问。
import threading
semaphore = threading.Semaphore(2)
def access_resource():
with semaphore:
print("Accessing resource")
time.sleep(1)
print("Resource released")
threads = []
for _ in range(5):
thread = threading.Thread(target=access_resource)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在上述示例中,我们使用信号量控制对共享资源的访问,每次允许最多两个线程访问资源。
五、线程局部数据
在多线程编程中,有时需要为每个线程维护独立的数据。Python提供了threading.local类,可以方便地实现线程局部数据。
import threading
thread_local_data = threading.local()
def process_data(value):
thread_local_data.value = value
print(f"Thread {threading.current_thread().name}: {thread_local_data.value}")
threads = []
for i in range(5):
thread = threading.Thread(target=process_data, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在上述示例中,我们使用threading.local类为每个线程维护独立的value变量。
六、守护线程
守护线程是一种特殊的线程,它的生命周期依赖于主线程。在主线程结束时,所有守护线程会自动终止。
import threading
import time
def daemon_thread():
while True:
print("Daemon thread running")
time.sleep(1)
daemon = threading.Thread(target=daemon_thread)
daemon.daemon = True
daemon.start()
time.sleep(3)
print("Main thread ending")
在上述示例中,我们创建了一个守护线程,并在主线程结束时自动终止守护线程。
七、线程池
线程池是一种高效的线程管理机制,可以重用线程,减少线程创建和销毁的开销。Python的concurrent.futures模块提供了ThreadPoolExecutor类,用于创建和管理线程池。
from concurrent.futures import ThreadPoolExecutor
def task(value):
print(f"Processing {value}")
with ThreadPoolExecutor(max_workers=4) as executor:
futures = [executor.submit(task, i) for i in range(10)]
for future in futures:
future.result()
在上述示例中,我们使用ThreadPoolExecutor创建了一个线程池,并提交了10个任务。
八、GIL(全局解释器锁)
Python的GIL(全局解释器锁)是一个限制多线程并发执行的机制。由于GIL的存在,Python的多线程在CPU密集型任务中无法充分利用多核CPU的优势。为了解决这个问题,可以使用多进程代替多线程。
from multiprocessing import Process
def cpu_bound_task(value):
print(f"Processing {value}")
processes = []
for i in range(10):
process = Process(target=cpu_bound_task, args=(i,))
processes.append(process)
process.start()
for process in processes:
process.join()
在上述示例中,我们使用多进程代替多线程,解决了GIL的限制。
九、总结
通过本文的介绍,我们学习了Python中调用多线程的多种方法,包括Threading模块、concurrent.futures模块和Queue模块。我们还学习了线程同步机制、线程局部数据、守护线程、线程池和GIL。在实际应用中,可以根据具体需求选择合适的多线程编程方法和同步机制,以提高程序的并发性能。
相关问答FAQs:
如何在Python中实现多线程的基本步骤是什么?
在Python中实现多线程的基本步骤包括导入threading
模块,创建一个线程类或定义一个线程函数,然后使用threading.Thread
来实例化线程对象。接下来,调用线程对象的start()
方法来启动线程,最后使用join()
方法来确保主线程等待所有子线程完成。
Python中的多线程适合哪些类型的任务?
Python的多线程非常适合I/O密集型任务,例如网络请求、文件读写和数据库操作等。这类任务通常会在等待I/O操作完成时使线程处于闲置状态,因此可以通过多线程来提高程序的并发性。然而,对于CPU密集型任务,Python的全局解释器锁(GIL)可能会限制多线程的性能提升,这种情况下可以考虑使用多进程。
如何在Python中处理多线程中的异常?
在Python的多线程中,处理异常可以通过在线程函数内部使用try...except
语句来捕获异常,这样可以防止线程意外终止。如果需要在主线程中获取子线程的异常信息,可以考虑使用队列(queue.Queue
)来传递异常信息,或者将异常信息存储在一个共享的变量中,以便在主线程中进行检查和处理。
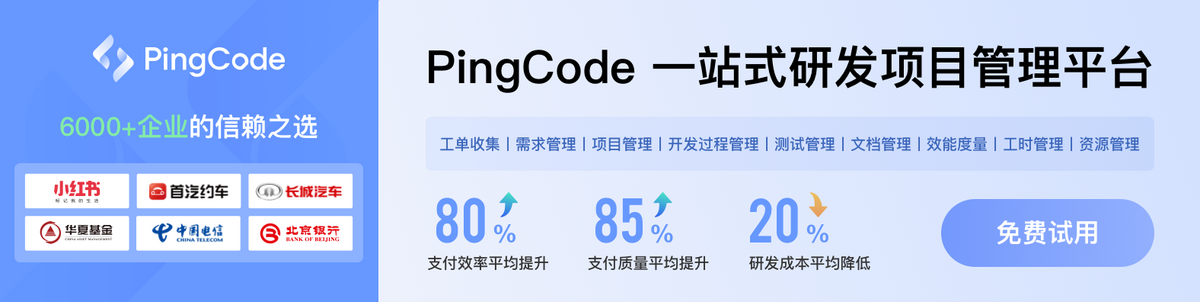