在Python中,关闭子窗体的方式主要取决于所使用的GUI框架。常见的GUI框架包括Tkinter、PyQt和wxPython等。要关闭子窗体,可以使用destroy()方法、close()方法、Destroy()方法。其中,destroy()方法在Tkinter中使用,close()方法在PyQt中使用,Destroy()方法在wxPython中使用。
详细描述其中一个方法:
在Tkinter中使用destroy()方法:
在Tkinter中,子窗体是通过Toplevel()类创建的。要关闭这个子窗体,可以调用子窗体实例的destroy()方法。比如,如果创建了一个子窗体实例为child_window,那么可以通过child_window.destroy()来关闭这个子窗体。这种方法会销毁子窗体及其所有子组件,使其从内存中删除。
接下来,我们将详细介绍如何在不同的Python GUI框架中关闭子窗体。
一、Tkinter中的子窗体管理
1、创建和关闭子窗体
在Tkinter中,子窗体通常通过Toplevel()类来创建。以下是一个简单的示例,展示了如何创建和关闭子窗体:
import tkinter as tk
def open_child_window():
child_window = tk.Toplevel(root)
child_window.title("Child Window")
close_button = tk.Button(child_window, text="Close", command=child_window.destroy)
close_button.pack()
root = tk.Tk()
root.title("Main Window")
open_button = tk.Button(root, text="Open Child Window", command=open_child_window)
open_button.pack()
root.mainloop()
在这个示例中,我们创建了一个主窗体,并在其中放置了一个按钮。点击按钮会打开一个子窗体,在子窗体中放置一个关闭按钮,点击关闭按钮即可关闭子窗体。
2、管理多个子窗体
如果需要管理多个子窗体,可以将子窗体实例保存到列表或字典中。例如:
import tkinter as tk
child_windows = []
def open_child_window():
child_window = tk.Toplevel(root)
child_window.title(f"Child Window {len(child_windows) + 1}")
close_button = tk.Button(child_window, text="Close", command=lambda: close_child_window(child_window))
close_button.pack()
child_windows.append(child_window)
def close_child_window(window):
window.destroy()
child_windows.remove(window)
root = tk.Tk()
root.title("Main Window")
open_button = tk.Button(root, text="Open Child Window", command=open_child_window)
open_button.pack()
root.mainloop()
在这个示例中,我们使用一个列表child_windows来保存所有打开的子窗体实例。关闭子窗体时,将其从列表中移除。
二、PyQt中的子窗体管理
1、创建和关闭子窗体
在PyQt中,子窗体通常通过QWidget或QDialog类来创建。以下是一个简单的示例,展示了如何创建和关闭子窗体:
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QWidget, QVBoxLayout
def open_child_window():
child_window = QWidget()
child_window.setWindowTitle("Child Window")
close_button = QPushButton("Close", child_window)
close_button.clicked.connect(child_window.close)
layout = QVBoxLayout()
layout.addWidget(close_button)
child_window.setLayout(layout)
child_window.show()
app = QApplication([])
main_window = QMainWindow()
main_window.setWindowTitle("Main Window")
open_button = QPushButton("Open Child Window", main_window)
open_button.clicked.connect(open_child_window)
main_window.setCentralWidget(open_button)
main_window.show()
app.exec_()
在这个示例中,我们创建了一个主窗体,并在其中放置了一个按钮。点击按钮会打开一个子窗体,在子窗体中放置一个关闭按钮,点击关闭按钮即可关闭子窗体。
2、管理多个子窗体
如果需要管理多个子窗体,可以将子窗体实例保存到列表或字典中。例如:
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QWidget, QVBoxLayout
child_windows = []
def open_child_window():
child_window = QWidget()
child_window.setWindowTitle(f"Child Window {len(child_windows) + 1}")
close_button = QPushButton("Close", child_window)
close_button.clicked.connect(lambda: close_child_window(child_window))
layout = QVBoxLayout()
layout.addWidget(close_button)
child_window.setLayout(layout)
child_window.show()
child_windows.append(child_window)
def close_child_window(window):
window.close()
child_windows.remove(window)
app = QApplication([])
main_window = QMainWindow()
main_window.setWindowTitle("Main Window")
open_button = QPushButton("Open Child Window", main_window)
open_button.clicked.connect(open_child_window)
main_window.setCentralWidget(open_button)
main_window.show()
app.exec_()
在这个示例中,我们使用一个列表child_windows来保存所有打开的子窗体实例。关闭子窗体时,将其从列表中移除。
三、wxPython中的子窗体管理
1、创建和关闭子窗体
在wxPython中,子窗体通常通过wx.Frame或wx.Dialog类来创建。以下是一个简单的示例,展示了如何创建和关闭子窗体:
import wx
class ChildWindow(wx.Frame):
def __init__(self, parent, title):
super(ChildWindow, self).__init__(parent, title=title, size=(300, 200))
panel = wx.Panel(self)
close_button = wx.Button(panel, label="Close", pos=(100, 70))
close_button.Bind(wx.EVT_BUTTON, self.on_close)
def on_close(self, event):
self.Destroy()
class MainWindow(wx.Frame):
def __init__(self, parent, title):
super(MainWindow, self).__init__(parent, title=title, size=(300, 200))
panel = wx.Panel(self)
open_button = wx.Button(panel, label="Open Child Window", pos=(70, 70))
open_button.Bind(wx.EVT_BUTTON, self.on_open)
def on_open(self, event):
child_window = ChildWindow(None, "Child Window")
child_window.Show()
app = wx.App(False)
main_window = MainWindow(None, "Main Window")
main_window.Show()
app.MainLoop()
在这个示例中,我们创建了一个主窗体,并在其中放置了一个按钮。点击按钮会打开一个子窗体,在子窗体中放置一个关闭按钮,点击关闭按钮即可关闭子窗体。
2、管理多个子窗体
如果需要管理多个子窗体,可以将子窗体实例保存到列表或字典中。例如:
import wx
class ChildWindow(wx.Frame):
def __init__(self, parent, title):
super(ChildWindow, self).__init__(parent, title=title, size=(300, 200))
panel = wx.Panel(self)
close_button = wx.Button(panel, label="Close", pos=(100, 70))
close_button.Bind(wx.EVT_BUTTON, self.on_close)
def on_close(self, event):
self.Destroy()
class MainWindow(wx.Frame):
def __init__(self, parent, title):
super(MainWindow, self).__init__(parent, title=title, size=(300, 200))
self.child_windows = []
panel = wx.Panel(self)
open_button = wx.Button(panel, label="Open Child Window", pos=(70, 70))
open_button.Bind(wx.EVT_BUTTON, self.on_open)
def on_open(self, event):
child_window = ChildWindow(None, f"Child Window {len(self.child_windows) + 1}")
child_window.Show()
self.child_windows.append(child_window)
app = wx.App(False)
main_window = MainWindow(None, "Main Window")
main_window.Show()
app.MainLoop()
在这个示例中,我们使用一个列表child_windows来保存所有打开的子窗体实例。
四、总结
在不同的Python GUI框架中,关闭子窗体的方法略有不同。在Tkinter中,可以使用destroy()方法;在PyQt中,可以使用close()方法;在wxPython中,可以使用Destroy()方法。无论使用哪种方法,都可以通过调用子窗体实例的相应方法来关闭子窗体。此外,为了更好地管理多个子窗体,可以将子窗体实例保存到列表或字典中,并在关闭子窗体时从中移除。希望这些示例和技巧能帮助你更好地管理Python中的子窗体。
相关问答FAQs:
如何在Python中关闭Tkinter创建的子窗体?
在Tkinter中,可以使用destroy()
方法来关闭子窗体。首先,你需要获取子窗体的引用,然后调用该方法。例如,如果你的子窗体变量名为child_window
,你可以使用child_window.destroy()
来关闭它。
关闭子窗体时需要注意哪些事项?
关闭子窗体时,确保没有未保存的数据或正在进行的操作。可以在关闭之前弹出确认对话框,询问用户是否确认关闭,这样可以避免数据丢失的情况。
是否可以在关闭子窗体时执行特定的操作?
当然可以。你可以在调用destroy()
之前执行特定的操作,例如保存数据或释放资源。可以通过定义一个函数来处理这些操作,然后在关闭子窗体时调用该函数,确保所有必要的步骤都已完成。
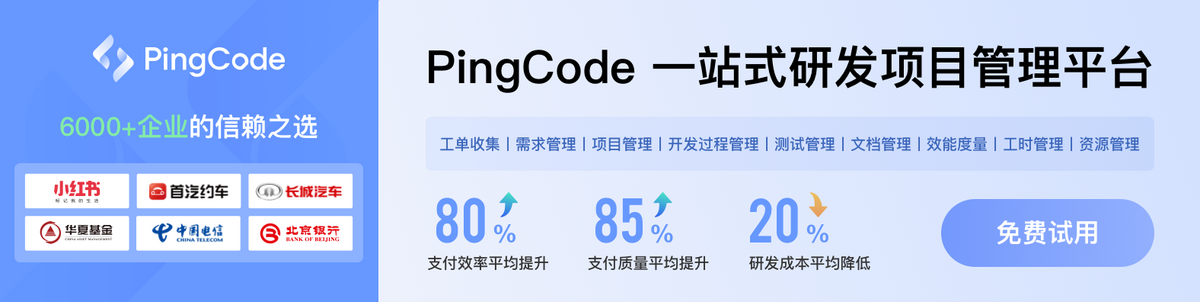