Python程序停留的方法有多种,包括使用time.sleep()
、input()
、threading.Event()
、while
循环、sys.stdin.read()
。其中,time.sleep()
是最常见和简单的方法。
在Python编程中,常常需要让程序在某个点暂时停止并等待某些条件满足后再继续运行。这种需求可以通过以下几种方法来实现:
一、TIME.SLEEP()
time.sleep()
函数是Python中用于让程序暂停执行一段时间的最简单方法。
import time
print("Program started")
time.sleep(5) # 暂停5秒
print("Program resumed")
详细描述:
time.sleep()
函数来自Python的time
模块,它接受一个参数,即暂停的秒数。该函数会将当前线程暂停指定的时间,然后再继续执行后续代码。它主要用于需要在程序中添加延迟的场景,比如控制执行频率、模拟长时间操作等。
二、INPUT()
使用input()
函数可以使程序等待用户输入,从而实现停留效果。
print("Press Enter to continue...")
input() # 等待用户按下Enter键
print("Program resumed")
三、THREADING.EVENT()
threading.Event
对象提供了一种线程间通信的方法,可以让一个线程等待另一个线程的信号。
import threading
event = threading.Event()
def wait_for_event():
print("Waiting for event...")
event.wait() # 等待事件被设置
print("Event received, resuming program")
thread = threading.Thread(target=wait_for_event)
thread.start()
模拟一些操作
import time
time.sleep(3)
设置事件
event.set()
四、WHILE循环
使用while
循环可以让程序在某个条件为False时一直停留。
import time
should_continue = False
def wait_condition():
global should_continue
while not should_continue:
print("Waiting...")
time.sleep(1)
import threading
thread = threading.Thread(target=wait_condition)
thread.start()
模拟一些操作
time.sleep(5)
改变条件
should_continue = True
五、SYS.STDIN.READ()
sys.stdin.read()
可以使程序等待输入,直到用户按下某个键。
import sys
print("Press any key to continue...")
sys.stdin.read(1) # 读取一个字符
print("Program resumed")
六、时间调度
有时,程序需要在特定时间执行某个任务,可以使用调度库如schedule
。
import schedule
import time
def job():
print("Executing scheduled job")
schedule.every(5).seconds.do(job)
while True:
schedule.run_pending()
time.sleep(1)
七、异步编程
在异步编程中,可以使用asyncio
库来实现程序的停留和等待。
import asyncio
async def main():
print("Waiting for 5 seconds...")
await asyncio.sleep(5)
print("Resuming program")
asyncio.run(main())
八、使用信号
在一些高级应用中,可以使用信号来控制程序的执行。
import signal
import time
def handler(signum, frame):
print("Signal received, resuming program")
signal.signal(signal.SIGALRM, handler)
signal.alarm(5) # 5秒后发送SIGALRM信号
print("Waiting for signal...")
signal.pause() # 暂停程序,等待信号
print("Program resumed")
九、阻塞I/O操作
有时,程序会由于I/O操作(如网络或文件操作)而自然地停留。
import requests
print("Fetching data from the internet...")
response = requests.get('https://example.com')
print("Data fetched, resuming program")
十、图形用户界面(GUI)事件循环
在GUI编程中,事件循环使程序在等待用户操作时保持运行状态。
import tkinter as tk
def on_button_click():
print("Button clicked")
root = tk.Tk()
button = tk.Button(root, text="Click me", command=on_button_click)
button.pack()
root.mainloop() # 进入事件循环,程序停留
十一、使用条件变量
条件变量允许一个线程等待另一个线程发出信号。
import threading
condition = threading.Condition()
def wait_for_condition():
with condition:
condition.wait() # 等待条件被通知
print("Condition met, resuming program")
thread = threading.Thread(target=wait_for_condition)
thread.start()
模拟一些操作
import time
time.sleep(5)
通知条件
with condition:
condition.notify()
十二、模拟阻塞操作
在某些情况下,可以模拟阻塞操作来暂停程序。
import time
def blocking_function():
print("Blocking operation started")
time.sleep(5)
print("Blocking operation completed")
blocking_function()
十三、使用事件驱动架构
事件驱动架构使得程序在等待事件时保持响应。
import selectors
import socket
sel = selectors.DefaultSelector()
def accept(sock, mask):
conn, addr = sock.accept()
print('Accepted', conn, 'from', addr)
conn.setblocking(False)
sel.register(conn, selectors.EVENT_READ, read)
def read(conn, mask):
data = conn.recv(1000)
if data:
print('Received', repr(data), 'from', conn)
else:
print('Closing', conn)
sel.unregister(conn)
conn.close()
sock = socket.socket()
sock.bind(('localhost', 12345))
sock.listen(100)
sock.setblocking(False)
sel.register(sock, selectors.EVENT_READ, accept)
while True:
events = sel.select()
for key, mask in events:
callback = key.data
callback(key.fileobj, mask)
十四、使用生成器
生成器可以暂停函数执行并在需要时恢复。
def generator_function():
print("First part")
yield
print("Second part")
gen = generator_function()
next(gen) # 执行到yield
next(gen) # 恢复执行
十五、使用信号量
信号量可以控制多个线程的并发访问。
import threading
semaphore = threading.Semaphore(0)
def worker():
print("Waiting for semaphore")
semaphore.acquire()
print("Semaphore acquired, resuming program")
thread = threading.Thread(target=worker)
thread.start()
模拟一些操作
import time
time.sleep(5)
释放信号量
semaphore.release()
十六、使用资源锁
资源锁(如互斥锁)可以保护共享资源。
import threading
lock = threading.Lock()
def critical_section():
with lock:
print("In critical section")
thread1 = threading.Thread(target=critical_section)
thread2 = threading.Thread(target=critical_section)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
十七、使用队列
队列可以在线程间传递数据和实现同步。
import queue
import threading
q = queue.Queue()
def worker():
item = q.get()
print("Processing item:", item)
q.task_done()
thread = threading.Thread(target=worker)
thread.start()
模拟一些操作
import time
time.sleep(5)
放入队列项
q.put("task")
q.join() # 等待所有队列项被处理
十八、使用回调函数
回调函数可以在特定事件发生时执行。
import time
def callback_function():
print("Callback executed")
def main_function(callback):
print("Main function started")
time.sleep(5)
callback()
print("Main function completed")
main_function(callback_function)
十九、使用协程
协程可以在需要时暂停和恢复执行。
import asyncio
async def coroutine():
print("Coroutine started")
await asyncio.sleep(5)
print("Coroutine resumed")
asyncio.run(coroutine())
二十、使用信号处理
信号处理可以在接收到特定信号时执行代码。
import signal
import time
def handler(signum, frame):
print("Signal received, resuming program")
signal.signal(signal.SIGINT, handler)
print("Waiting for signal...")
signal.pause() # 暂停程序,等待信号
print("Program resumed")
二十一、使用多进程
在多进程编程中,可以使用进程间通信来控制程序执行。
import multiprocessing
import time
def worker(event):
print("Waiting for event")
event.wait()
print("Event received, resuming process")
event = multiprocessing.Event()
process = multiprocessing.Process(target=worker, args=(event,))
process.start()
模拟一些操作
time.sleep(5)
设置事件
event.set()
process.join()
二十二、使用异步I/O
异步I/O可以在等待I/O操作时保持程序响应。
import asyncio
async def fetch_data():
print("Fetching data...")
await asyncio.sleep(5)
print("Data fetched")
asyncio.run(fetch_data())
二十三、使用轮询
轮询可以定期检查某个条件是否满足。
import time
def check_condition():
# 模拟条件检查
return False
print("Polling for condition...")
while not check_condition():
print("Condition not met, waiting...")
time.sleep(1)
print("Condition met, resuming program")
二十四、使用定时器
定时器可以在一定时间后执行代码。
import threading
def timer_callback():
print("Timer expired, resuming program")
timer = threading.Timer(5, timer_callback)
timer.start()
print("Waiting for timer...")
timer.join()
二十五、使用上下文管理器
上下文管理器可以在特定代码块执行前后执行特定操作。
import time
class Timer:
def __enter__(self):
self.start = time.time()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.end = time.time()
print(f"Code block executed in {self.end - self.start} seconds")
with Timer():
time.sleep(5)
总结
通过上述方法,可以根据不同的需求和场景选择合适的方式让Python程序停留。time.sleep()
是最基础且常用的方法,但在更复杂的应用中,可能需要结合线程、事件、信号、异步编程等来实现更高级的控制。
相关问答FAQs:
如何让Python程序在特定位置暂停?
可以使用input()
函数来实现程序的暂停,直到用户按下回车键。这种方法适用于需要等待用户输入的场景。例如,您可以在程序的某个位置添加input("按回车键继续...")
,这样程序就会在此处停留,直到用户完成输入。
Python中是否有内置的暂停功能?
Python本身没有专门的暂停功能,但可以通过使用time
模块中的sleep()
函数来实现。使用sleep(seconds)
可以让程序暂停指定的秒数。例如,time.sleep(5)
将使程序停留5秒钟。这在需要延迟操作时非常有用。
如何在Python程序中实现条件暂停?
可以使用循环和条件语句来实现根据特定条件暂停程序。例如,您可以在程序中设置一个条件,当满足该条件时,程序将等待用户输入以继续执行。这种方法适合需要动态控制程序流程的场景。示例代码如下:
while True:
user_input = input("输入'y'继续,输入'n'暂停:")
if user_input.lower() == 'y':
break
elif user_input.lower() == 'n':
print("程序已暂停,输入'y'继续。")
通过这些方法,您可以灵活地控制Python程序的停留和继续执行。
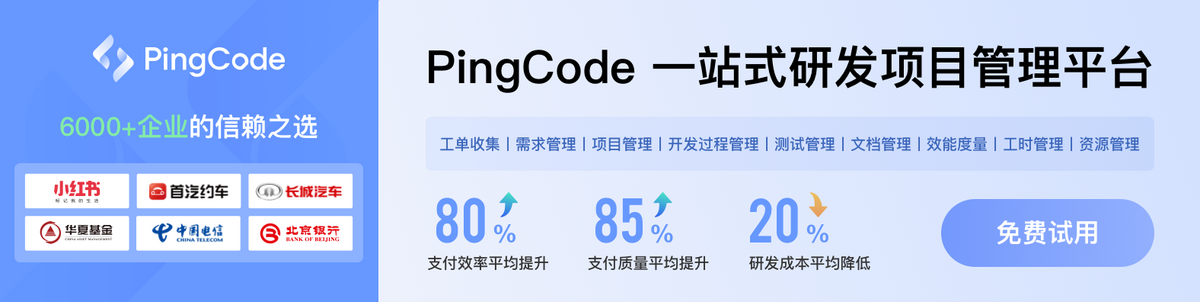