Python设置人工监控的关键在于使用合适的工具和库,如psutil、logging、schedule、Flask等,配置报警机制、日志记录、任务调度等功能。 其中,psutil库提供了系统和进程监控的功能;logging库用于记录日志信息;schedule库用于任务调度;Flask则可以用来创建一个简单的web界面进行监控。下面我们将详细介绍如何使用这些工具来实现Python的人工监控。
一、PSUTIL库的使用
psutil (process and system utilities) 是一个跨平台库,能够轻松实现系统和进程的监控。它可以用来获取CPU、内存、磁盘、网络和传感器的相关信息。通过这些信息,我们可以实现对系统资源的监控。
1.1 安装psutil
要使用psutil库,首先需要安装它。可以通过pip来安装:
pip install psutil
1.2 获取系统信息
psutil库提供了多种方法来获取系统信息。以下是一些常见的使用方法:
import psutil
获取CPU使用率
cpu_usage = psutil.cpu_percent(interval=1)
print(f"CPU Usage: {cpu_usage}%")
获取内存使用情况
memory_info = psutil.virtual_memory()
print(f"Memory Usage: {memory_info.percent}%")
获取磁盘使用情况
disk_usage = psutil.disk_usage('/')
print(f"Disk Usage: {disk_usage.percent}%")
获取网络信息
net_io = psutil.net_io_counters()
print(f"Bytes Sent: {net_io.bytes_sent}, Bytes Received: {net_io.bytes_recv}")
1.3 监控进程
psutil还可以用来监控系统中的进程。我们可以获取进程的详细信息,如CPU、内存、打开的文件等。
# 获取所有进程ID
pids = psutil.pids()
获取某个进程的信息
pid = pids[0] # 以第一个进程为例
process = psutil.Process(pid)
print(f"Process Name: {process.name()}")
print(f"CPU Usage: {process.cpu_percent(interval=1)}%")
print(f"Memory Usage: {process.memory_percent()}%")
二、LOGGING库的使用
日志记录是监控系统中必不可少的一部分。logging库是Python内置的日志记录模块,可以方便地记录和管理日志信息。
2.1 基本配置
首先,我们需要配置日志记录器。以下是一个简单的配置示例:
import logging
配置日志记录器
logging.basicConfig(level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='monitor.log',
filemode='a')
记录日志
logging.info('This is an info message.')
logging.warning('This is a warning message.')
logging.error('This is an error message.')
2.2 日志级别
logging库提供了多种日志级别,每个级别对应不同的严重程度:
- DEBUG: 详细信息,一般只在诊断问题时使用。
- INFO: 信息确认,一般用于记录程序运行的正常信息。
- WARNING: 警告信息,表示程序可能发生问题。
- ERROR: 错误信息,表示程序发生了问题。
- CRITICAL: 严重错误信息,表示程序可能无法继续运行。
2.3 日志处理器和格式器
我们还可以为日志记录器添加多个处理器(Handler)和格式器(Formatter),以实现更灵活的日志记录。
# 创建一个日志记录器
logger = logging.getLogger('monitor')
创建一个控制台处理器
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.DEBUG)
创建一个文件处理器
file_handler = logging.FileHandler('monitor.log')
file_handler.setLevel(logging.INFO)
创建一个格式器
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
console_handler.setFormatter(formatter)
file_handler.setFormatter(formatter)
将处理器添加到日志记录器
logger.addHandler(console_handler)
logger.addHandler(file_handler)
记录日志
logger.debug('This is a debug message.')
logger.info('This is an info message.')
logger.warning('This is a warning message.')
logger.error('This is an error message.')
logger.critical('This is a critical message.')
三、SCHEDULE库的使用
schedule库是一个轻量级的任务调度库,可以用来定时执行任务。通过schedule库,我们可以定期监控系统资源,并记录日志或发送报警信息。
3.1 安装schedule
首先,我们需要安装schedule库:
pip install schedule
3.2 定时任务
schedule库提供了简单的API来定义定时任务。以下是一个示例,定期监控系统资源,并记录到日志文件中:
import schedule
import time
import psutil
import logging
配置日志记录器
logging.basicConfig(level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='monitor.log',
filemode='a')
定义监控任务
def monitor():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
disk_usage = psutil.disk_usage('/')
logging.info(f"CPU Usage: {cpu_usage}%")
logging.info(f"Memory Usage: {memory_info.percent}%")
logging.info(f"Disk Usage: {disk_usage.percent}%")
定时执行监控任务
schedule.every(1).minutes.do(monitor)
运行调度器
while True:
schedule.run_pending()
time.sleep(1)
四、FLASK库的使用
Flask是一个轻量级的web框架,可以用来创建一个简单的web界面,用于展示监控数据或提供控制接口。
4.1 安装Flask
首先,我们需要安装Flask库:
pip install Flask
4.2 创建监控页面
以下是一个简单的Flask应用,展示系统监控数据:
from flask import Flask, jsonify
import psutil
app = Flask(__name__)
@app.route('/monitor')
def monitor():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
disk_usage = psutil.disk_usage('/')
net_io = psutil.net_io_counters()
data = {
'cpu_usage': cpu_usage,
'memory_usage': memory_info.percent,
'disk_usage': disk_usage.percent,
'bytes_sent': net_io.bytes_sent,
'bytes_received': net_io.bytes_recv
}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
运行该应用后,访问http://127.0.0.1:5000/monitor
即可查看系统监控数据。
五、配置报警机制
在监控系统中,当检测到异常情况时,及时报警是非常重要的。我们可以通过发送邮件、短信或其他方式来实现报警机制。
5.1 发送邮件
我们可以使用smtplib库来发送邮件。以下是一个示例,当CPU使用率超过阈值时发送报警邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
import psutil
def send_email(subject, body):
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(sender_email, password)
text = msg.as_string()
server.sendmail(sender_email, receiver_email, text)
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
监控任务
def monitor():
cpu_usage = psutil.cpu_percent(interval=1)
if cpu_usage > 80:
send_email("CPU Usage Alert", f"CPU usage is {cpu_usage}%")
定时执行监控任务
schedule.every(1).minutes.do(monitor)
运行调度器
while True:
schedule.run_pending()
time.sleep(1)
5.2 发送短信
我们可以使用第三方短信服务来发送短信报警。以下是使用twilio库发送短信的示例:
from twilio.rest import Client
import psutil
配置twilio账号信息
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
def send_sms(body):
message = client.messages.create(
body=body,
from_='+1234567890',
to='+0987654321'
)
print(f"Message sent: {message.sid}")
监控任务
def monitor():
cpu_usage = psutil.cpu_percent(interval=1)
if cpu_usage > 80:
send_sms(f"CPU usage is {cpu_usage}%")
定时执行监控任务
schedule.every(1).minutes.do(monitor)
运行调度器
while True:
schedule.run_pending()
time.sleep(1)
六、集成监控系统
综合上述内容,我们可以集成一个完整的监控系统,包括系统资源监控、日志记录、定时任务、报警机制和web界面。
6.1 系统资源监控和日志记录
首先,定义系统资源监控和日志记录的功能:
import psutil
import logging
配置日志记录器
logging.basicConfig(level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='monitor.log',
filemode='a')
def monitor_system():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
disk_usage = psutil.disk_usage('/')
net_io = psutil.net_io_counters()
logging.info(f"CPU Usage: {cpu_usage}%")
logging.info(f"Memory Usage: {memory_info.percent}%")
logging.info(f"Disk Usage: {disk_usage.percent}%")
logging.info(f"Bytes Sent: {net_io.bytes_sent}, Bytes Received: {net_io.bytes_recv}")
6.2 定时任务和报警机制
然后,定义定时任务和报警机制:
import schedule
import time
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(subject, body):
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(sender_email, password)
text = msg.as_string()
server.sendmail(sender_email, receiver_email, text)
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
def monitor_task():
cpu_usage = psutil.cpu_percent(interval=1)
if cpu_usage > 80:
send_email("CPU Usage Alert", f"CPU usage is {cpu_usage}%")
monitor_system()
定时执行监控任务
schedule.every(1).minutes.do(monitor_task)
运行调度器
while True:
schedule.run_pending()
time.sleep(1)
6.3 创建web界面
最后,创建一个简单的web界面来展示监控数据:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/monitor')
def monitor():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
disk_usage = psutil.disk_usage('/')
net_io = psutil.net_io_counters()
data = {
'cpu_usage': cpu_usage,
'memory_usage': memory_info.percent,
'disk_usage': disk_usage.percent,
'bytes_sent': net_io.bytes_sent,
'bytes_received': net_io.bytes_recv
}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
七、总结
通过以上步骤,我们可以使用Python实现一个完整的人工监控系统,包含系统资源监控、日志记录、任务调度、报警机制和web界面。PSUTIL库用于获取系统信息、LOGGING库用于记录日志、SCHEDULE库用于定时任务、FLASK库用于创建web界面,最终实现了一个功能齐全的监控系统。 在实际应用中,我们可以根据具体需求进行调整和优化,以满足不同场景的监控要求。
相关问答FAQs:
如何在Python中实现人工监控的功能?
要在Python中实现人工监控,可以使用多种方法,例如结合OpenCV进行视频流处理,或使用Flask框架搭建一个简单的监控网页。具体步骤包括捕捉视频源,处理图像数据,以及根据需要设置警报或记录功能。通过编写脚本,可以实时监控特定区域,或在检测到异常时发送通知。
Python人工监控需要哪些库或工具?
实现人工监控的常用库包括OpenCV、NumPy和Flask等。OpenCV用于图像处理和视频流捕捉,NumPy用于处理数组和矩阵,Flask则可以帮助搭建Web界面,方便用户查看监控画面。此外,可能还需要其他工具,如FFmpeg用于处理音视频流。
如何确保Python监控系统的稳定性与安全性?
为了确保监控系统的稳定性,建议使用异常处理机制,定期检查视频流的状态并自动重启服务。此外,安全性可以通过加密传输数据、设置访问权限和定期更新系统来加强,确保只有授权用户才能访问监控内容。
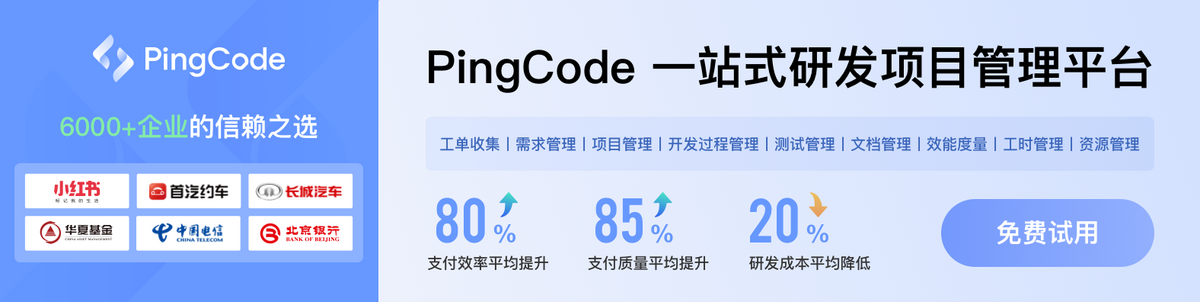