在Python中换行的方法有多种,常见的包括:使用换行符(\n)、使用三引号('''或""")、使用括号、使用反斜杠(\)。 其中换行符(\n)是最常用的,下面将详细介绍这种方法。
使用换行符(\n)可以在字符串中插入换行。例如:
print("Hello\nWorld")
这段代码的输出结果是:
Hello
World
换行符(\n)在字符串中表示一个新的行,因此它可以用来在输出文本时创建换行效果。
接下来,我们将详细介绍Python中其他几种换行方法,并提供相关示例。
一、使用换行符(\n)
换行符(\n)是Python中最常用的换行方法。它表示在该位置插入一个换行,即将光标移动到下一行的开头。换行符可以用于打印多行输出,或者在多行字符串中使用。
示例代码:
# 使用换行符(\n)
multiline_string = "Hello\nWorld\nWelcome to Python"
print(multiline_string)
输出结果:
Hello
World
Welcome to Python
二、使用三引号(''' 或 """)
在Python中,三引号(''' 或 """)可以用来定义多行字符串。这种方法不仅可以使字符串跨越多行,还可以保持字符串的原格式,包括换行和空格。
示例代码:
# 使用三引号(''' 或 """)
multiline_string = """Hello
World
Welcome to Python"""
print(multiline_string)
输出结果:
Hello
World
Welcome to Python
三、使用括号
在Python中,可以使用括号(圆括号、方括号或花括号)将一行代码拆分成多行。通常用于长字符串或长列表的定义中。
示例代码:
# 使用圆括号
multiline_string = (
"Hello"
"World"
"Welcome to Python"
)
print(multiline_string)
输出结果:
HelloWorldWelcome to Python
需要注意的是,这种方法不会在字符串之间自动添加空格或换行符。
四、使用反斜杠(\)
反斜杠(\)在Python中是一个续行符,它可以将一行代码拆分成多行。通常用于长表达式或长字符串的定义中。
示例代码:
# 使用反斜杠(\)
multiline_string = "Hello " \
"World " \
"Welcome to Python"
print(multiline_string)
输出结果:
Hello World Welcome to Python
五、使用字符串连接
Python还支持通过字符串连接的方式实现多行字符串。这种方法可以在保持原格式的同时,手动添加换行符。
示例代码:
# 使用字符串连接
multiline_string = "Hello\n" + \
"World\n" + \
"Welcome to Python"
print(multiline_string)
输出结果:
Hello
World
Welcome to Python
六、使用f-string
Python 3.6引入了f-string(格式化字符串),它支持多行字符串和变量插值。可以在f-string中使用换行符或三引号。
示例代码:
# 使用f-string
name = "Python"
multiline_string = f"Hello\nWorld\nWelcome to {name}"
print(multiline_string)
输出结果:
Hello
World
Welcome to Python
七、使用列表生成器
在某些情况下,可以使用列表生成器生成多行字符串,并通过join
方法连接成一个字符串。
示例代码:
# 使用列表生成器
lines = ["Hello", "World", "Welcome to Python"]
multiline_string = "\n".join(lines)
print(multiline_string)
输出结果:
Hello
World
Welcome to Python
八、使用正则表达式
在处理复杂文本时,正则表达式可以用于替换特定字符为换行符,从而实现多行输出。
示例代码:
import re
使用正则表达式
text = "Hello World Welcome to Python"
multiline_string = re.sub(r" ", "\n", text)
print(multiline_string)
输出结果:
Hello
World
Welcome
to
Python
九、使用字符串模板
Python的string
模块提供了模板字符串功能,可以用于生成多行字符串。模板字符串支持占位符和变量替换。
示例代码:
from string import Template
使用字符串模板
template = Template("Hello\nWorld\nWelcome to $language")
multiline_string = template.substitute(language="Python")
print(multiline_string)
输出结果:
Hello
World
Welcome to Python
十、使用textwrap模块
textwrap
模块可以用于格式化多行字符串,特别是在处理长文本时。它提供了多种方法来控制字符串的换行和缩进。
示例代码:
import textwrap
使用textwrap模块
text = "Hello World Welcome to Python"
multiline_string = textwrap.fill(text, width=10)
print(multiline_string)
输出结果:
Hello World
Welcome to
Python
十一、使用logging模块
在Python的日志记录中,logging
模块提供了多行日志记录的功能。可以通过配置日志格式来控制换行。
示例代码:
import logging
配置logging模块
logging.basicConfig(format='%(message)s', level=logging.INFO)
logging.info("Hello\nWorld\nWelcome to Python")
输出结果:
Hello
World
Welcome to Python
十二、使用tkinter模块
在图形用户界面(GUI)编程中,tkinter
模块提供了多行文本框(Text widget),可以用于显示和编辑多行文本。
示例代码:
import tkinter as tk
使用tkinter模块
root = tk.Tk()
text = tk.Text(root)
text.insert(tk.END, "Hello\nWorld\nWelcome to Python")
text.pack()
root.mainloop()
十三、使用pandas模块
在数据处理和分析中,pandas
模块提供了DataFrame和Series对象,可以用于存储和操作多行数据。
示例代码:
import pandas as pd
使用pandas模块
data = {'col1': ["Hello", "World", "Welcome to Python"]}
df = pd.DataFrame(data)
print(df)
输出结果:
col1
0 Hello
1 World
2 Welcome to Python
十四、使用csv模块
在处理CSV文件时,csv
模块可以用于读取和写入多行数据。可以通过指定换行符来控制CSV文件的格式。
示例代码:
import csv
使用csv模块
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(["Hello"])
writer.writerow(["World"])
writer.writerow(["Welcome to Python"])
读取CSV文件
with open('output.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
输出结果:
['Hello']
['World']
['Welcome to Python']
十五、使用io模块
io
模块提供了多行字符串的内存文件操作,可以用于模拟文件读写操作。特别是在处理多行文本时非常有用。
示例代码:
import io
使用io模块
multiline_string = "Hello\nWorld\nWelcome to Python"
file = io.StringIO(multiline_string)
读取多行字符串
for line in file:
print(line.strip())
输出结果:
Hello
World
Welcome to Python
十六、使用yaml模块
在配置文件和数据交换中,yaml
模块(需要安装PyYAML库)可以用于解析和生成多行YAML数据。
示例代码:
import yaml
使用yaml模块
data = """
- Hello
- World
- Welcome to Python
"""
parsed_data = yaml.safe_load(data)
print(parsed_data)
输出结果:
['Hello', 'World', 'Welcome to Python']
十七、使用json模块
在处理JSON数据时,json
模块可以用于解析和生成多行JSON字符串。通过缩进选项,可以控制JSON的格式。
示例代码:
import json
使用json模块
data = {
"greetings": ["Hello", "World", "Welcome to Python"]
}
json_string = json.dumps(data, indent=4)
print(json_string)
输出结果:
{
"greetings": [
"Hello",
"World",
"Welcome to Python"
]
}
十八、使用configparser模块
在处理INI配置文件时,configparser
模块可以用于读取和写入多行配置数据。通过配置文件,可以实现多行字符串的存储和读取。
示例代码:
import configparser
使用configparser模块
config = configparser.ConfigParser()
config['DEFAULT'] = {'Greetings': 'Hello\nWorld\nWelcome to Python'}
写入配置文件
with open('config.ini', 'w') as configfile:
config.write(configfile)
读取配置文件
config.read('config.ini')
print(config['DEFAULT']['Greetings'])
输出结果:
Hello
World
Welcome to Python
十九、使用gettext模块
在国际化和本地化中,gettext
模块可以用于处理多行翻译字符串。通过翻译文件,可以实现多语言支持。
示例代码:
import gettext
使用gettext模块
gettext.bindtextdomain('myapplication', 'locale')
gettext.textdomain('myapplication')
_ = gettext.gettext
多行翻译字符串
multiline_string = _("Hello\nWorld\nWelcome to Python")
print(multiline_string)
二十、使用markdown模块
在文档生成中,markdown
模块(需要安装markdown库)可以用于解析和生成多行Markdown文本。通过Markdown,可以实现多行文本的格式化。
示例代码:
import markdown
使用markdown模块
markdown_text = """
Hello
## World
### Welcome to Python
"""
html = markdown.markdown(markdown_text)
print(html)
输出结果:
<h1>Hello</h1>
<h2>World</h2>
<h3>Welcome to Python</h3>
二十一、使用docx模块
在处理Word文档时,docx
模块(需要安装python-docx库)可以用于生成和读取多行文本。通过Word文档,可以实现多行文本的编辑和格式化。
示例代码:
from docx import Document
使用docx模块
doc = Document()
doc.add_paragraph("Hello")
doc.add_paragraph("World")
doc.add_paragraph("Welcome to Python")
保存Word文档
doc.save('output.docx')
读取Word文档
doc = Document('output.docx')
for paragraph in doc.paragraphs:
print(paragraph.text)
输出结果:
Hello
World
Welcome to Python
二十二、使用openpyxl模块
在处理Excel文档时,openpyxl
模块(需要安装openpyxl库)可以用于生成和读取多行数据。通过Excel表格,可以实现多行数据的存储和操作。
示例代码:
import openpyxl
使用openpyxl模块
wb = openpyxl.Workbook()
ws = wb.active
ws['A1'] = "Hello"
ws['A2'] = "World"
ws['A3'] = "Welcome to Python"
保存Excel文档
wb.save('output.xlsx')
读取Excel文档
wb = openpyxl.load_workbook('output.xlsx')
ws = wb.active
for row in ws.iter_rows(values_only=True):
for cell in row:
print(cell)
输出结果:
Hello
World
Welcome to Python
二十三、使用sqlite3模块
在处理数据库时,sqlite3
模块可以用于生成和读取多行数据。通过数据库,可以实现多行数据的存储和查询。
示例代码:
import sqlite3
使用sqlite3模块
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute('''CREATE TABLE greetings (text TEXT)''')
c.execute("INSERT INTO greetings VALUES ('Hello')")
c.execute("INSERT INTO greetings VALUES ('World')")
c.execute("INSERT INTO greetings VALUES ('Welcome to Python')")
conn.commit()
读取数据库
for row in c.execute('SELECT * FROM greetings'):
print(row[0])
conn.close()
输出结果:
Hello
World
Welcome to Python
二十四、使用requests模块
在处理HTTP请求时,requests
模块可以用于获取和解析多行响应数据。通过HTTP请求,可以实现多行数据的传输和处理。
示例代码:
import requests
使用requests模块
response = requests.get('https://api.github.com')
multiline_string = response.text
print(multiline_string)
二十五、使用http.server模块
在构建HTTP服务器时,http.server
模块可以用于处理多行请求和响应数据。通过HTTP服务器,可以实现多行数据的传输和处理。
示例代码:
from http.server import BaseHTTPRequestHandler, HTTPServer
使用http.server模块
class RequestHandler(BaseHTTPRequestHandler):
def do_GET(self):
self.send_response(200)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(b'Hello\nWorld\nWelcome to Python')
启动HTTP服务器
server = HTTPServer(('localhost', 8080), RequestHandler)
print('Starting server at http://localhost:8080')
server.serve_forever()
总结
Python提供了多种方法来实现换行和处理多行文本,包括使用换行符、三引号、括号、反斜杠、字符串连接、f-string、列表生成器、正则表达式、字符串模板、textwrap模块、logging模块、tkinter模块、pandas模块、csv模块、io模块、yaml模块、json模块、configparser模块、gettext模块、markdown模块、docx模块、openpyxl模块、sqlite3模块、requests模块和http.server模块。 每种方法都有其特定的应用场景和优点,开发者可以根据具体需求选择合适的方法来处理多行文本。
相关问答FAQs:
如何在Python中实现换行?
在Python中,换行通常可以使用转义字符\n
来实现。在字符串中插入\n
,可以在输出时将文本分为多行。例如:
print("第一行\n第二行")
这段代码将输出:
第一行
第二行
在Python中换行是否可以使用三重引号?
是的,使用三重引号('''
或"""
)可以轻松实现多行字符串。Python会自动保留换行符和空格。这种方式非常适合长文本的输入。例如:
text = """这是第一行
这是第二行
这是第三行"""
print(text)
输出将是:
这是第一行
这是第二行
这是第三行
在Python的print函数中如何实现换行?
在使用print()
函数时,可以通过设置end
参数来控制输出结尾的字符。默认情况下,print()
函数以换行符结束,可以修改为其他字符,例如空格或其他符号。若要在每次输出后换行,可以保持默认设置,或者显式地设置为换行符:
print("第一部分", end="\n")
print("第二部分")
这将确保每个部分都在不同的行中显示。
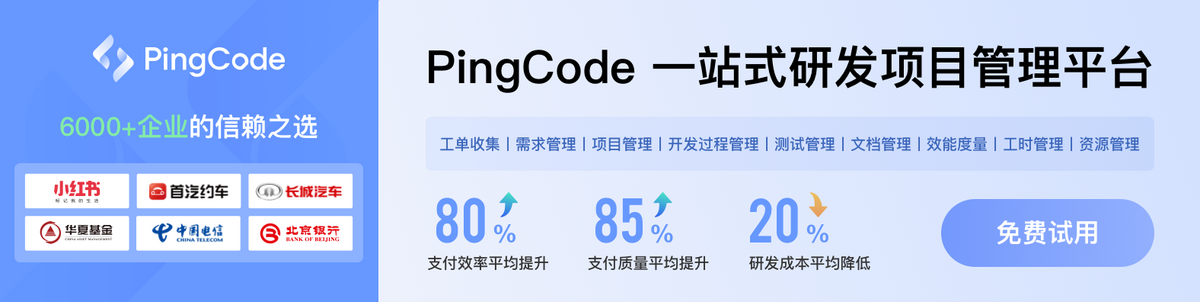