在Python中设置网卡模式可以通过调用操作系统命令或使用特定的网络库来实现。可以使用第三方库如scapy
、调用操作系统命令如ifconfig
或ip
、使用psutil
库来查询网络接口信息。下面将详细描述如何使用这些方法来设置网卡模式,并对其中一个方法展开详细描述。
使用第三方库如scapy
scapy
是一个非常强大的Python库,用于数据包处理,可以用来创建、解析、发送和嗅探网络数据包。虽然scapy
本身不直接提供设置网卡模式的功能,但你可以通过它来检测和操作网卡。
调用操作系统命令如ifconfig或ip
在Linux系统中,设置网卡模式通常通过命令行工具ifconfig
或ip
命令来实现。Python的subprocess
库可以帮助我们调用这些命令。
例如,要将网卡设置为混杂模式,可以使用以下Python代码:
import subprocess
def set_promiscuous_mode(interface):
subprocess.run(['ifconfig', interface, 'promisc'], check=True)
set_promiscuous_mode('eth0')
使用psutil库
psutil
是一个跨平台的Python库,用于系统和进程的监控与管理,虽然它主要用于获取系统信息,但也可以用于查询网络接口信息。
详细描述如何使用ifconfig命令设置网卡模式
在Linux操作系统中,可以通过调用ifconfig
命令来设置网卡模式。下面是详细步骤:
- 安装必要的库
首先,需要确保安装了subprocess
库,这个库通常是Python标准库的一部分,不需要单独安装。
- 检查当前网卡状态
在设置网卡模式之前,可以使用ifconfig
命令来检查当前网卡的状态:
import subprocess
def check_interface(interface):
result = subprocess.run(['ifconfig', interface], capture_output=True, text=True)
print(result.stdout)
check_interface('eth0')
- 设置网卡为混杂模式
混杂模式是网卡的一种工作模式,在这种模式下,网卡可以接收所有通过网络的数据包,无论这些数据包是否发给它。这对于网络监控和嗅探非常有用。
import subprocess
def set_promiscuous_mode(interface):
subprocess.run(['ifconfig', interface, 'promisc'], check=True)
print(f"Interface {interface} set to promiscuous mode.")
set_promiscuous_mode('eth0')
- 关闭混杂模式
如果需要关闭混杂模式,可以使用以下代码:
import subprocess
def unset_promiscuous_mode(interface):
subprocess.run(['ifconfig', interface, '-promisc'], check=True)
print(f"Interface {interface} unset from promiscuous mode.")
unset_promiscuous_mode('eth0')
一、使用第三方库如scapy
scapy
库是一个非常强大的Python库,用于数据包处理和网络分析。虽然它本身不直接提供设置网卡模式的功能,但它可以与操作系统命令结合使用,来检测和操作网卡。
安装scapy
首先,需要安装scapy
库:
pip install scapy
使用scapy进行网络操作
scapy
可以用来嗅探网络数据包,这通常需要将网卡设置为混杂模式。下面是一个简单的示例,演示如何使用scapy
嗅探数据包:
from scapy.all import sniff
def packet_callback(packet):
print(packet.show())
sniff(prn=packet_callback, count=10)
在这个示例中,sniff
函数将捕获并显示前10个数据包。默认情况下,scapy
会将网卡设置为混杂模式以捕获所有通过网络的数据包。
二、调用操作系统命令如ifconfig或ip
在Linux系统中,ifconfig
和ip
命令是最常用的网络配置工具。Python的subprocess
库可以帮助我们调用这些命令来设置网卡模式。
使用ifconfig设置网卡模式
ifconfig
命令用于配置网络接口,可以通过它来设置网卡为混杂模式或关闭混杂模式。
import subprocess
def set_promiscuous_mode(interface):
subprocess.run(['ifconfig', interface, 'promisc'], check=True)
print(f"Interface {interface} set to promiscuous mode.")
def unset_promiscuous_mode(interface):
subprocess.run(['ifconfig', interface, '-promisc'], check=True)
print(f"Interface {interface} unset from promiscuous mode.")
使用ip命令设置网卡模式
ip
命令是ifconfig
命令的现代替代品,功能更强大且更灵活。可以使用以下代码来设置网卡模式:
import subprocess
def set_promiscuous_mode(interface):
subprocess.run(['ip', 'link', 'set', interface, 'promisc', 'on'], check=True)
print(f"Interface {interface} set to promiscuous mode.")
def unset_promiscuous_mode(interface):
subprocess.run(['ip', 'link', 'set', interface, 'promisc', 'off'], check=True)
print(f"Interface {interface} unset from promiscuous mode.")
三、使用psutil库
psutil
是一个跨平台库,用于获取系统信息和进行系统监控。虽然psutil
主要用于获取系统和进程信息,但也可以用于查询网络接口的基本信息。
安装psutil
首先,需要安装psutil
库:
pip install psutil
查询网络接口信息
可以使用psutil
库来获取网络接口的基本信息:
import psutil
def list_network_interfaces():
interfaces = psutil.net_if_addrs()
for interface, addrs in interfaces.items():
print(f"Interface: {interface}")
for addr in addrs:
print(f" Address: {addr.address}")
print(f" Netmask: {addr.netmask}")
print(f" Broadcast: {addr.broadcast}")
list_network_interfaces()
虽然psutil
不直接提供设置网卡模式的功能,但它可以与其他方法结合使用,以实现更复杂的网络配置和监控任务。
四、总结
在Python中设置网卡模式可以通过调用操作系统命令或使用特定的网络库来实现。可以使用第三方库如scapy
、调用操作系统命令如ifconfig
或ip
、使用psutil
库来查询网络接口信息。通过这些方法,可以方便地设置和管理网卡模式,以满足不同的网络配置和监控需求。
相关问答FAQs:
如何在Python中检测网卡的当前模式?
要检测网卡的当前模式,您可以使用psutil
库。首先安装该库,然后通过psutil.net_if_stats()
函数获取网卡状态信息。该函数将返回一个字典,其中包含每个网卡的状态和速度等信息,您可以检查特定网卡的模式。
Python中有哪些库可以用来设置网络接口的模式?
在Python中,有几个库可以用来设置网络接口的模式,如subprocess
和os
库。通过调用系统命令,可以实现更改网络接口的模式。例如,可以使用subprocess.run()
来执行ip
或ifconfig
命令并修改网卡设置。
如何在Python中切换网卡的模式到桥接模式?
切换网卡到桥接模式通常需要管理员权限,您可以使用subprocess
库执行系统命令。在Linux系统中,可以使用命令brctl
来创建一个桥接接口,并将相应的网卡添加到该桥接中。确保在执行这些命令之前,您已经了解了网络配置的相关知识。
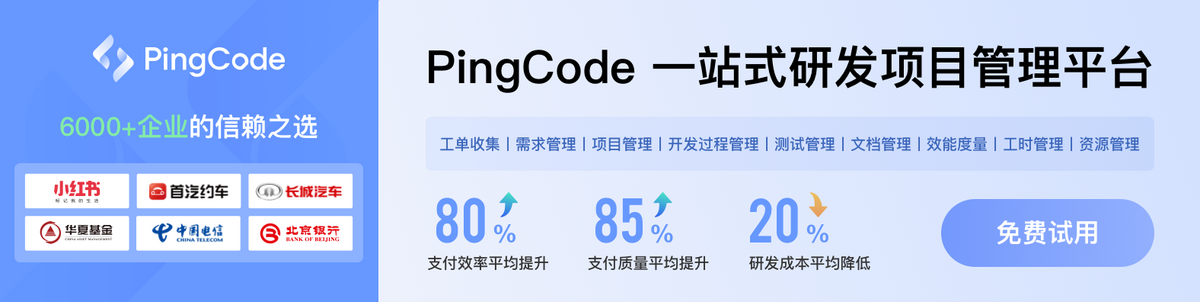