Python线程池创建的方法包括使用ThreadPoolExecutor、Thread类、以及自定义线程池管理器。使用ThreadPoolExecutor是最常见和便捷的方法。
一、使用 ThreadPoolExecutor 创建线程池
ThreadPoolExecutor 是 Python 3.2 引入的一个高效的线程池实现,它隶属于 concurrent.futures 模块。使用 ThreadPoolExecutor 可以方便地管理和调度线程,避免手动管理线程带来的复杂性。
1.1、基本使用方法
ThreadPoolExecutor 可以通过 with 语句来创建和使用线程池,这样可以确保线程池在使用完毕后自动关闭:
from concurrent.futures import ThreadPoolExecutor
def task(arg):
print(f"Task with argument {arg} is running")
with ThreadPoolExecutor(max_workers=5) as executor:
for i in range(10):
executor.submit(task, i)
详细描述:在这个例子中,我们创建了一个最大可容纳 5 个工作线程的线程池。通过 submit
方法将任务提交到线程池中,每个任务会在一个独立的线程中运行。当 with
语句块结束时,线程池会自动关闭并等待所有线程执行完毕。
1.2、设置最大线程数
可以在创建 ThreadPoolExecutor 对象时,指定最大线程数。这个参数决定了线程池中可以同时运行的最大线程数量:
executor = ThreadPoolExecutor(max_workers=10)
1.3、submit 和 map 方法
除了 submit
方法,ThreadPoolExecutor 还提供了 map
方法,可以将一个可迭代对象中的每个元素作为参数传递给任务函数:
def square(x):
return x * x
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(square, range(10))
for result in results:
print(result)
二、使用 Thread 类手动创建线程池
尽管 ThreadPoolExecutor 提供了极大的便利,有时我们可能需要更灵活的控制,可以手动创建线程池。
2.1、创建线程池
手动创建线程池需要自己管理线程的创建和销毁,可以通过创建一个线程类来实现:
import threading
class MyThread(threading.Thread):
def __init__(self, func, args=()):
super().__init__()
self.func = func
self.args = args
def run(self):
self.func(*self.args)
def task(arg):
print(f"Task with argument {arg} is running")
threads = []
for i in range(10):
t = MyThread(task, (i,))
threads.append(t)
t.start()
for t in threads:
t.join()
2.2、管理线程池
可以通过一个管理类来管理线程池中的线程,确保线程池可以被有效地管理和控制:
class ThreadPool:
def __init__(self, num_threads):
self.tasks = []
self.num_threads = num_threads
self.threads = []
def add_task(self, func, args=()):
self.tasks.append((func, args))
def start(self):
for _ in range(self.num_threads):
t = threading.Thread(target=self.worker)
self.threads.append(t)
t.start()
def worker(self):
while self.tasks:
func, args = self.tasks.pop(0)
func(*args)
def wait_completion(self):
for t in self.threads:
t.join()
def task(arg):
print(f"Task with argument {arg} is running")
pool = ThreadPool(5)
for i in range(10):
pool.add_task(task, (i,))
pool.start()
pool.wait_completion()
三、自定义线程池管理器
有时我们需要一个更复杂的线程池管理器,可以自定义一个线程池管理器类,以便更加灵活地管理线程。
3.1、自定义管理器类
通过继承 threading.Thread 类,可以创建一个自定义的线程池管理器类:
import threading
import queue
class CustomThreadPool:
def __init__(self, num_threads):
self.tasks = queue.Queue()
self.num_threads = num_threads
self.threads = []
self._create_threads()
def _create_threads(self):
for _ in range(self.num_threads):
thread = threading.Thread(target=self._worker)
thread.daemon = True
self.threads.append(thread)
thread.start()
def _worker(self):
while True:
func, args = self.tasks.get()
try:
func(*args)
finally:
self.tasks.task_done()
def add_task(self, func, args=()):
self.tasks.put((func, args))
def wait_completion(self):
self.tasks.join()
def task(arg):
print(f"Task with argument {arg} is running")
pool = CustomThreadPool(5)
for i in range(10):
pool.add_task(task, (i,))
pool.wait_completion()
3.2、扩展自定义管理器
自定义线程池管理器可以进一步扩展以支持更多功能,例如动态调整线程数量、任务优先级调度等:
import threading
import queue
class ExtendedThreadPool:
def __init__(self, num_threads):
self.tasks = queue.PriorityQueue()
self.num_threads = num_threads
self.threads = []
self._create_threads()
def _create_threads(self):
for _ in range(self.num_threads):
thread = threading.Thread(target=self._worker)
thread.daemon = True
self.threads.append(thread)
thread.start()
def _worker(self):
while True:
priority, func, args = self.tasks.get()
try:
func(*args)
finally:
self.tasks.task_done()
def add_task(self, func, args=(), priority=1):
self.tasks.put((priority, func, args))
def wait_completion(self):
self.tasks.join()
def task(arg):
print(f"Task with argument {arg} is running")
pool = ExtendedThreadPool(5)
for i in range(10):
pool.add_task(task, (i,), priority=i)
pool.wait_completion()
四、线程池的实际应用场景
线程池在实际编程中有广泛的应用场景,如网络爬虫、数据处理、并行计算等。在这些场景中,使用线程池可以显著提高程序的执行效率。
4.1、网络爬虫
网络爬虫需要同时抓取多个网页内容,使用线程池可以加快抓取速度:
import requests
from concurrent.futures import ThreadPoolExecutor
def fetch_url(url):
response = requests.get(url)
print(f"Fetched {url} with status {response.status_code}")
urls = ["http://example.com", "http://example.org", "http://example.net"]
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(fetch_url, urls)
4.2、数据处理
在大数据处理过程中,可以使用线程池并行处理数据,提高处理效率:
import time
from concurrent.futures import ThreadPoolExecutor
def process_data(data):
time.sleep(1)
print(f"Processed data: {data}")
data_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(process_data, data_list)
4.3、并行计算
线程池可以用于并行计算任务,如矩阵运算、图像处理等:
import numpy as np
from concurrent.futures import ThreadPoolExecutor
def compute_square(x):
return x * x
data = np.random.rand(1000)
with ThreadPoolExecutor(max_workers=10) as executor:
results = list(executor.map(compute_square, data))
print(results)
五、线程池的注意事项
在使用线程池时,有一些注意事项需要考虑,以确保程序的正确性和高效性。
5.1、线程安全
当多个线程访问共享资源时,需要确保线程安全。可以使用线程锁来保护共享资源:
import threading
lock = threading.Lock()
shared_resource = 0
def task():
global shared_resource
with lock:
shared_resource += 1
with ThreadPoolExecutor(max_workers=5) as executor:
for _ in range(1000):
executor.submit(task)
5.2、避免死锁
在使用线程池时,应避免出现死锁的情况。确保每个线程所需的资源能够被及时释放:
import threading
lock1 = threading.Lock()
lock2 = threading.Lock()
def task1():
with lock1:
with lock2:
print("Task 1")
def task2():
with lock2:
with lock1:
print("Task 2")
with ThreadPoolExecutor(max_workers=2) as executor:
executor.submit(task1)
executor.submit(task2)
5.3、资源管理
确保线程池在使用完毕后能够正确释放资源,防止资源泄漏:
from concurrent.futures import ThreadPoolExecutor
def task():
print("Task is running")
with ThreadPoolExecutor(max_workers=5) as executor:
for _ in range(10):
executor.submit(task)
总之,Python线程池提供了一种高效、便捷的方式来管理多线程编程任务。通过使用ThreadPoolExecutor、手动管理线程池或自定义线程池管理器,可以根据不同的需求灵活地处理并发任务。在实际应用中,线程池在网络爬虫、数据处理、并行计算等场景中有着广泛的应用。使用线程池时需要注意线程安全、避免死锁以及正确管理资源,以确保程序的正确性和高效性。
相关问答FAQs:
如何在Python中创建线程池?
要在Python中创建线程池,您可以使用concurrent.futures
模块中的ThreadPoolExecutor
类。首先,导入相关模块,然后创建一个线程池实例,指定最大线程数。通过submit
方法向线程池提交任务,池中的线程将并行执行这些任务。使用with
语句可以确保在任务完成后自动释放资源。
线程池的优势是什么?
线程池的主要优势在于资源的有效管理和重用。当您有多个任务需要并发执行时,使用线程池可以避免频繁创建和销毁线程的开销。这种方法不仅提高了性能,还降低了内存使用。通过线程池,您可以快速启动多个线程,同时保持程序的响应性。
如何处理线程池中的异常?
在使用线程池时,您可以通过Future
对象来处理异常。当任务在执行过程中发生异常时,可以通过调用result()
方法捕获并处理该异常。建议在提交任务时使用try-except
结构,以便对可能的错误进行适当的处理和日志记录,从而提高程序的健壮性。
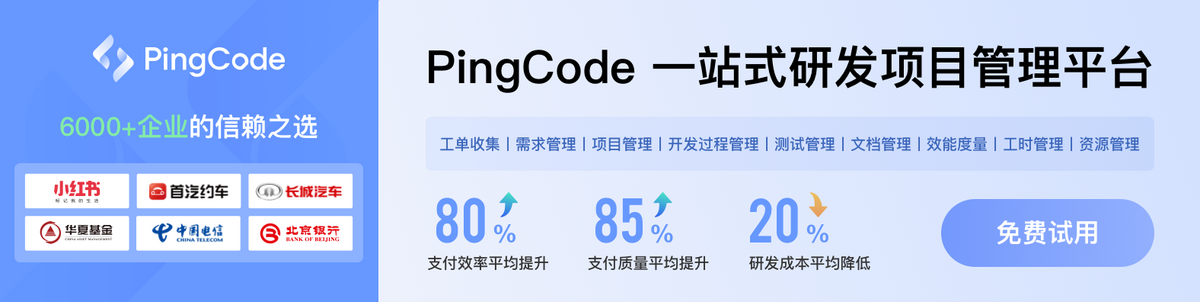